Week 10 Exercises - C Pointer File Operations PDF
Document Details
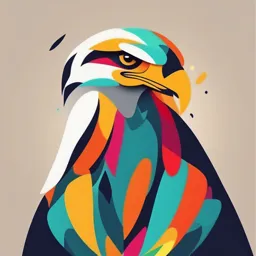
Uploaded by SnazzyEarthArt8280
Istanbul Atlas Üniversitesi
Tags
Summary
This document covers exercises on file operations in C, detailing functions like fopen, fclose, fprintf, for creating, opening, reading, writing, and closing files. It also includes explanations and examples.
Full Transcript
Exercises Pointer File Operations File Operations | C Files I/O: Create, Open, Read, Write and Close a File A File can be used to store a large volume of function purpose persistent data. Like many other languages ‘C’ Creating a file or opening an existing pr...
Exercises Pointer File Operations File Operations | C Files I/O: Create, Open, Read, Write and Close a File A File can be used to store a large volume of function purpose persistent data. Like many other languages ‘C’ Creating a file or opening an existing provides following file management functions, fopen () file 1. Creation of a file fclose () Closing a file 2. Opening a file fprintf () Writing a block of data to a file fscanf () Reading a block data from a file 3. Reading a file 4. Writing to a file getc () Reads a single character from a file 5. Closing a file putc () Writes a single character to a file Following are the most important file management getw () Reads an integer from a file functions available in ‘C,’ putw () Writing an integer to a file Sets the position of a file pointer to a fseek () specified location Returns the current position of a file ftell () pointer Sets the file pointer at the beginning of rewind () a file How to Create a File File Whenever you want to work with a file, the first step is to Mod Description create a file. A file is nothing but space in a memory e where data is stored. Open a file for reading. If a file is in reading To create a file in a ‘C’ program following syntax is used, r mode, then no data is deleted if a file is already present on a system. Open a file for writing. If a file is in writing mode, then a new file is created if a file doesn’t exist at all. If a file is already present w on a system, then all the data inside the file is truncated, and it is opened for writing purposes. Open a file in append mode. If a file is in append mode, then fopen is a standard function which is used to open a file. a the file is opened. The content within the file If the file is not present on the system, then it is created doesn’t change. and then opened. r+ open for reading and writing from beginning If a file is already present on the system, then it is directly opened using this function. w+ open for reading and writing, overwriting a file a+ open for reading and writing, appending to file Example: fopen() You can specify the path where you want to create your file How to Close a file One should always close a file whenever the operations on file are The fclose function takes a file pointer as an over. It means the contents and links to argument. The file associated with the file pointer is the file are terminated. This prevents then closed with the help of fclose function. It returns accidental damage to the file. 0 if close was successful and EOF (end of file) if there is an error has occurred while file closing. ‘C’ provides the fclose function to perform file closing operation. The After closing the file, the same file pointer can also syntax of fclose is as follows, be used with other files. In ‘C’ programming, files are automatically close when the program is terminated. Closing a file manually by writing fclose function is a good programming practice. Writing to a File In C, when you write to a file, newline characters ‘\n’ must be explicitly added. The stdio library offers the necessary functions to write to a file: fputc(char, file_pointer): It writes a character to the file pointed to by file_pointer. fputs(str, file_pointer): It writes a string to the file pointed to by file_pointer. fprintf(file_pointer, str, variable_lists): It prints a string to the file pointed to by file_pointer. The string can optionally include format specifiers and a list of variables variable_lists. The program below shows how to perform writing to a file: fputc() Function: fputs () Function: The above program writes a single character into the fputc_test.txt file until it reaches the next line symbol “\n” which 1.(fopen part) In the above program, we have created and indicates that the sentence was successfully written. The process is to opened a file called fputs_test.txt in a write mode. take each character of the array and write it into the file. 2.(fputs parts)After we do a write operation using fputs() function by writing three different strings Code Explanation 3.(fclose part)Then the file is closed using the fclose 1.In the above program, we have created and opened a file called function. fputc_test.txt in a write mode and declare our string which will be written into the file. 2.(for loop part)We do a character by character write operation using for loop and put each character in our file until the “\n” character is encountered then the file is closed using the fclose function. fprintf()Function: 1.(fopen part)In the above program we have created and opened a file called fprintf_test.txt in a write mode. 2.(fprintf)After a write operation is performed using fprintf() function by writing a string, then the file is closed using the fclose function. Reading data from a File - I Explain the END OF FILE (EOF) with a C Program There are three different functions dedicated to reading data from a file The End of the File (EOF) indicates the end of input. In C programming, End of File (EOF) is a special marker used to indicate that there fgetc(file_pointer): It returns the next character are no more data to be read from a file or input stream. It is particularly important from the file pointed to by the file pointer. When when dealing with file I/O and standard input operations. the end of the file has been reached, the EOF is Key Points about EOF: sent back. 1.Definition: EOF is a condition that indicates the end of a data stream. It is not a character fgets(buffer, n, file_pointer): It reads n-1 but a signal that no more data is available for reading. characters from the file and stores the string in a 2.EOF Value: In C, EOF is defined as a constant in the header file. It is typically buffer in which the NULL character ‘\0’ is represented by the integer value -1. This value is returned by functions like appended as the last character. getchar(), fgetc(), and fscanf() when they reach the end of a file or input stream. fscanf(file_pointer, conversion_specifiers, 3.Usage: variable_adresses): It is used to parse and EOF is commonly used in loops to read data until there is no more data to analyze data. It reads characters from the file and read. For example, when reading from a file or standard input, you can check assigns the input to a list of variable pointers for EOF to terminate the reading process. 4.Functions that Return EOF: variable_adresses using conversion specifiers. Functions such as getchar(), fgetc(), and fscanf() return EOF when they reach Keep in mind that as with scanf, fscanf stops the end of the input stream or encounter an error. reading a string when space or newline is encountered. Reading data from a File - II 1.(fopen + fgets parts)In the above program, we have opened the file called “fprintf_test.txt” which was previously written using fprintf() function, and it contains “Learning C with course” string. We read it using the fgets() function which reads line by line where the buffer size must be enough to handle the entire line. 2.(fopen+fscanf+prints parts)We reopen the file to reset the pointer file to point at the beginning of the file. Create various strings variables to handle each word separately. Print the variables to see their contents. The fscanf() is mainly used to extract and parse data from a file. 3.(fopen part)Reopen the file to reset the pointer file to point at the beginning of the file. Read data and print it from the file character by character using getc() function until the EOF statement is encountered 4.(fclose part)After performing a reading operation file using different variants, we again closed the file using the fclose function. Exercises: Program to compare the lengths of two entered words A program that checks the case of each character in a string and converts it to uppercase if it is lowercase and to lowercase if it is Write the Fizz Buzz game in C programming language. uppercase (Example - Input: MerHabA, Output: mERhABa ) Program to compare the lengths of two entered words. A program that takes the transpose of a matrix (transforming columns to rows and rows to columns) Program to compare whether two numbers entered from the keyboard (between 1 and 5) are the same. Print a program that prints whether a number entered from outside is positive or negative to the screen using a method Code perfect numbers in C language. Print the cube of all numbers starting from 1 to the number entered Program to find the most frequent letter/word count in a string from outside to the screen (Letter/Word Frequency). For example, Print the code that takes the average of all numbers starting from 1 to the number entered from outside using a method the most repeated letter for the word «hello» is «a». A program that prints and adds positive odd numbers starting from 1 Program to find the most frequent letter and count in a text to the screen as many as the number entered from outside file. A program that finds the ECO of two numbers entered Program to find the number of words in a text file. A program that finds the number of "numbers" in the text entered Program to remove repeated numbers in a sorted array. from the keyboard For example, Print a program that finds the number of spaces, number of letters, input: mySortedArray={1,1,1,2,2,3,4,5,5} reverse spelling, and number of words in the text entered from the output: mySortedArray={1,2,3,4,5} keyboard using a method Program to sort the numbers entered from the keyboard from From the keyboard Print the code that creates a triangle formation, smallest to largest/largest to smallest and remove repeated increasing the numbers from 1 up to the entered number, and a numbers. decreasing triangle formation afterwards (Sample output is given For example, below) input 6, 13, 1, 5, 8, 13, 1 output :1, 5, 6, 8, 13 Let's Take a Look! (Extra) Examine the algorithms below, try to understand what they are used for, how they work, how they approach problems, etc. and try to code. Some sample links: -https://www.geeksforgeeks.org/searching-algorithms/ -To access other algorithms from the menu on the left in the link: https://www.programiz.com/dsa/bubble-sort Sorting Algorithms: Bubble Sort, Selection Sort, Insertion Sort, Merge Sort, Quick Sort, Heap Sort etc. Search Algorithms: Linear Search, Binary Search, Depth-First Search (DFS), Breadth-First Search (BFS), Largest element in an Array, Second largest element in an array, Largest three in an array, Fibonacci Search etc. Graph Algorithms: Dijkstra's Algorithm (Shortest Path), Bellman-Ford Algorithm (Shortest Path), Kruskal's Algorithm (Minimum Spanning Tree), Prim's Algorithm (Minimum Spanning Tree) etc. Tree Algorithms: Binary Search Tree (BST), AVL Tree (Balanced Tree), Heap (Priority Queue) Data Structure Algorithms: Hashing, Trie, Union-Find (Disjoint Set) etc. Dynamic Programming Algorithms: Fibonacci Numbers, Longest Common Subsequence (LCS), Knapsack Problem, Red-Black Trees etc. Non-Sorting Algorithms: Linear Search, Radix Sort, Bucket Sort, Counting Sort etc. Artificial Intelligence, Classification and Clustering Algorithms: Minimax Algorithm (Game Trees), K-Means Clustering, SVM (Support Vecor Machine), Naive-Bayes Genetic Algorithms (Genetic Algorithms), Neural Networks (Artificial Neural Networks), LLM (Large Language Models) etc. Optimization Algorithms: Simulated Annealing, Genetic Algorithms, Particle Swarm Optimization Other known-prominent ones: LIFO (Last in First Out), FIFO (First in First Out), Traveling Salesman Algorithm, Ant Colony Algorithm, Greedy Algorithm, Dijkstra's Algorithm (Shortest Path), Kruskal's Algorithm (Minimum Spanning Tree), Prim's Algorithm (Minimum Spanning Tree), Huffman Coding (Optimal Prefix Codes) etc.