Programming in C PDF
Document Details
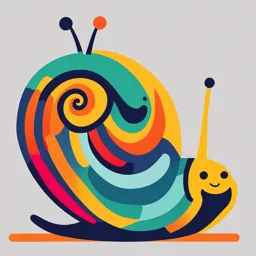
Uploaded by AttentiveAlgorithm
Tags
Summary
This document provides an overview of programming in C, including different programming languages, types of programming language, and explanations of programming paradigms. It also discusses the structure of a C program and detailed explanations.
Full Transcript
Programming Language Program Language A program is a set of instructions that help computer to perform tasks. Programs are executed by processor. The languages that are used to write a program or set of instructions for computer are called "Programming languages". A programming language is a...
Programming Language Program Language A program is a set of instructions that help computer to perform tasks. Programs are executed by processor. The languages that are used to write a program or set of instructions for computer are called "Programming languages". A programming language is a language used to write computer programs, which instruct a computer to perform some kind of computation, and/or organize the flow of control between external devices (such as a printer, a robot, or any peripheral). A programming language is a computer language that is used by programmers (developers) to communicate with computers. It is a set of instructions written in any specific language ( C, C++, Java, Python) to perform a specific task. Types of Programming Language 1. Low-level programming language Low-level language is machine-dependent (0s and 1s) programming language. The processor runs low- level programs directly without the need of a compiler or interpreter, so the programs written in low-level language can be run very fast. i. Machine Language i. Machine language is lowest level of programming language. ii. It handles binary data i.e. 0’s and 1’s. It directly interacts with system. iii. Machine language is difficult for human beings to understand as it comprises combination of 0’s and 1’s. There is software which translate programs into machine level language. Examples include operating systems like Linux, UNIX, Windows, etc. iv. In this language, there is no need of compilers and interpreters for conversion and hence the time consumption is less. However, it is not portable and non-readable to humans. v. The advantage of machine language is that it helps the programmer to execute the programs faster than the high-level programming language. i. Assembly Language Assembly language (ASM) is also a type of low-level programming language that is designed for specific processors. It represents the set of instructions in a symbolic and human-understandable form (known as mnemonics. It uses short mnemonic codes for instructions and allows the programmer to introduce names for blocks of memory that hold data. One might thus write “add pay, total” instead of “0110101100101000” for an instruction that adds two numbers. Assembly language is designed to be easily translated into machine language. It uses an assembler to convert the assembly language to machine language. High-level programming language High-level programming language (HLL) is designed for developing user-friendly software programs and websites. This programming language requires a compiler or interpreter to translate the program into machine language (execute the program). The main advantage of a high-level language is that it is easy to read, write, and maintain. Structure of C program Documentation section Preprocessor section Definition section Global declaration Main function User defined functions Documentation section (Comment) It includes the statement specified at the beginning of a program, such as a program's name, date, description, and title. It is represented as: //name of a program Preprocessor section The preprocessor section contains all the header files used in a program. It informs the system to link the header files to the system libraries. It is given by: #include #include Define section The define section comprises of different constants declared using the define keyword. It is given by: #define a = 2 Global declaration The global section comprises of all the global declarations in the program. It is given by: float num = 2.54; Main function main() is the first function to be executed by the computer. It is necessary for a code to include the main(). It is like any other function available in the C library. Parenthesis () are used for passing parameters (if any) to a function. The main function is declared as: int main() Or void main() Local declarations The variable that is declared inside a given function or block refers to as local declarations. main() { int i = 2; } User defined functions The user defined functions specified the functions specified as per the requirements of the user. For example, color(), sum(), division(), etc. Programming Paradigm The term programming paradigm refers to a style of programming. Paradigm can also be termed as method to solve some problem or do some task. Programming paradigms are not languages or tools. They're more like a set of ideals and guidelines. Each paradigm advocates a specific way to organize the program code. Each language has a unique programming style that implements a specific programming paradigm. Each programming paradigm advocates a set of principles, ideas, design concepts and rules that defines the manner in which the program code is written and organized. Types of Programming Paradigm Imperative Programming Functional Procedural Programming Programming Programming Paradigm Declarative Object Oriented programming Programming Imperative Programming It consists of sets of detailed instructions that are given to the computer to execute in a given order. It's called "imperative" because as programmers we dictate exactly what the computer has to do, in a very specific way. Imperative programming focuses on describing how a program operates, step by step. It is one of the oldest programming paradigm. Procedural programming paradigm Procedural programming is a derivation of imperative programming, adding to it the feature of functions (also known as "procedures" or "subroutines"). In procedural programming, the user is encouraged to subdivide the program execution into functions, as a way of improving modularity and organization. Example: C, Pascal etc Object oriented programming The program is written as a collection of classes and object which are meant for communication. The smallest and basic entity is object and all kind of computation is performed on the objects only. More emphasis is on data rather procedure. It can handle almost all kind of real life problems which are today in scenario. Example: C++, Java, python, ruby etc. Advantages: Data security Inheritance Code reusability Flexible and abstraction is also present Declarative Programming Declarative programming is all about hiding away complexity and bringing programming languages closer to human language and thinking. It's the direct opposite of imperative programming in the sense that the programmer doesn't give instructions about how the computer should execute the task, but rather on what result is needed. This is the only difference between imperative (how to do) and declarative (what to do) programming paradigms. Functional programming paradigms Functional programming takes the concept of functions a little bit further. In functional programming, functions are treated as first-class citizens, meaning that they can be assigned to variables, passed as arguments, and returned from other functions. The key principle of this paradigms is the execution of series of mathematical functions. Data are loosely coupled to functions. The function hide their implementation. Example: Scala, F# etc Language Translator Computer programs are generally written in high-level languages (like C++, Python, and Java). A language processor, or language translator, is a computer program that convert source code from one programming language to another language or to machine code (also known as object code). They also find errors during translation. Types of Language Translator Compiler Assembler Interpreter Compiler A compiler is a translator used to convert high-level programming language (source code) to low-level programming language (object Code). It converts the whole program in one session (all at a time). The source code is translated to object code successfully if it is free of errors. The compiler specifies the errors at the end of the compilation with line numbers when there are any errors in the source code. The object program can be executed number of times without translating it again. It is different for each processor. Compilers are also different for each platform. Example: C, C++ Interpreter Just like a compiler, is a translator used to convert high-level programming language to low-level programming language. An Interpreter translates a single statement of the source program into machine code and executes immediately before moving on to the next line. If there is an error in the statement, the interpreter terminates its translating process at that statement and displays an error message. It is faster than a compiler. They are used as debugging tools because interpreters can only run one piece of code at a time. Example: Pearl, python etc. Assembler Assemblers translate a program written in assembly language into machine language. The assembler is basically able to convert these mnemonics in binary code. Example: Fortran Assembly Program (FAP), Macro Assembly Program (MAP), Symbolic Optimal Assembly Program (SOAP)