Java Programming Summary PDF
Document Details
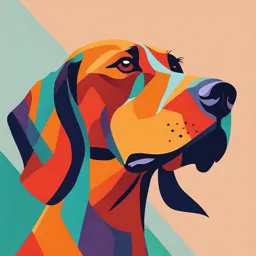
Uploaded by FamousDeStijl3725
KFU
Tags
Summary
This document is a summary of three chapters on Java programming. It covers fundamental concepts like classes, objects, and methods. The text explains different data types and operators, and how to use input/output to handle user interactions. It outlines Java's application, including the Java Virtual Machine.
Full Transcript
# Chapter 1: Introduction to Java - Java is the most widely used. - Software: the instructions we write. - Hardware: the computers. - Performing a task requires a method. - **Method:** Houses the program statements (hidden from the user). - **Class:** Houses the methods. - Reuse th...
# Chapter 1: Introduction to Java - Java is the most widely used. - Software: the instructions we write. - Hardware: the computers. - Performing a task requires a method. - **Method:** Houses the program statements (hidden from the user). - **Class:** Houses the methods. - Reuse the class to build many objects, it saves time and efforts, reliable and effective systems. - An object has attributes, part of the object's class, specified by the class's Instance Variable. - Java is used to develop Large-scale applications. - **Java Class Libraries:** 1. Rich collections of existing classes and methods. 2. Known as the: Java API (Application Programming Interface). - **Integrated Development Environments (IDEs):** - Provide tools that support the process. - Editors for writing, editing, debuggers for logic errors. - Popular IDEs: - Eclipse - netBeans - TextPad - CPU doesn’t understand java. - Machinecode consists of 0s and 1s. - Java is a high Prog lang: (readable instructions) - So Java-Programs must be converted to machine code by Compiler. ## Problems of Conversion: 1. Each CPU has its own machine code. 2. Each PC has its own Platform (CPU + Operating system) ## To Solve the Problem: Java-byte code. ## Java virtual machine (JVM): - Make byte code understandable. - JVM is developed by Sun, free, Not independent. ## Java Application: A computer Program that executes when you use the Java command to launch the JVM. - **Comments:** Ignored by the compiler. - `//` end of line comment - `/* Traditional comment */` All text is ignored. - **Javadoc Comments:** Delimited by `/**` and `*/` (use in HTML format) - **Blank lines** and **whitespaces** are ignored. - **Every Java Program consists a class.** - **Main method** is the Starting Point of every Java application. - **Java class** contain one or more method. - **Void:** The method will not return any information. - **String:** Called character String or string literal. - **White space** in the string are not ignored. - **Strings** can't span multiple lines of code. ## Newline Characters Whitespace ## Back Slash: escape Character. `\n` ## Printf: comma-separated list %s %d %f # Chapter 2: Data and Operators - Data in the memory called variables. - **Import:** Help the compiler to locate the class in the program. - Rich set of classes. - **Classes** are grouped into **Packages** - Java API - **Variable deceleration Statement** `Scanner input = New Scanner(System.in);` - **Scanner:** To read data - Data come from many sources, user, file. - **Equals sign =:** The variable should be initialized with the result. - **New:** Create an object - **System.in:** To read bytes information typed by the user. (the Scanner translates these bytes) - **System:** Is a class, from package `java.lang`. - **Operator =:** Is a binary operator, it has two operands. - `*`: Multiplication - `%`: Remainder after division - `/`: Integer division - **Condition:** True or False - **if selection:** To make a decision - **Equality operators:** `==` and `!=` - **Relational operators:** `>`, `<`, `>=`, `<=` # Chapter 3: Objects and Classes - Objects are attributes: Reusable software components. - Object referred to instance of its class. - **Method call:** Tells method of the object to perform its task. - **Keyword Public:** Is an access modifier (available). - **First line in the method called Method header.** - **Static method (such as Main) is special, it can be called without creating an object. ** - **I can’t call method from another class i create object from that class.** ## Why Java is an extensible language? - I can declare new class types as needed. - **Constructor:** Class name with() (call), Similar to a method used when the object is created. - **Any class can contain Main method.** - **JVM invoke the main method** - `javac GradeBook.java GradeBookTest.java` - `javac *.java` ## Class Name - Attribute <- UML diagram - Methods ## Parameter: Additional information a method needs to perform a task. - (Method Call need arguments) - one (int) ←→ more (int, int) - **Scanner method nextLine:** Read until the end of the line (Press Enter). - **Scanner method next:** Reads one word (Press whitespace to Submit). - **Number of arguments = Number of Parameters.** - **Type ~ = Type ~ ** - e.g. `Add(a,b);` ← Parameters - `Add(2,3);` ← Arguments (actual values) ## Attributes called (fields) - **Instance Variable declared Private. access modifier** - data hiding - **Set and get method used to access instance variables** - Local variables are Not automatically initialized - **set:** assign values. - **get:** obtain values from private instance variables - **References:** to store the locations of objects in memory.