Lecture 4.2_ Iteration II.pdf
Document Details
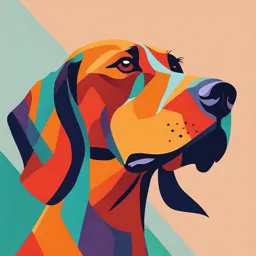
Uploaded by GenerousChrysoprase
La Trobe University
Tags
Full Transcript
Lecture 4.2 Iteration II Lecture Overview 1. For Loops 2. Example Program: FizzBuzz 3. Aggregation 2 1. For Loops 3 For Loops 4 While Loops vs. For Loops ◎ Previously we saw how while loops can be used to perform iteration in Python. ○ The while loop is controlled by a condition. ◎ A com...
Lecture 4.2 Iteration II Lecture Overview 1. For Loops 2. Example Program: FizzBuzz 3. Aggregation 2 1. For Loops 3 For Loops 4 While Loops vs. For Loops ◎ Previously we saw how while loops can be used to perform iteration in Python. ○ The while loop is controlled by a condition. ◎ A common use for iteration is to perform an action for each item in a sequence. ○ e.g. Add numbers from 1 to 10, print all customers. ◎ Python provides for loops as a more convenient way of iterating over a sequence. 5 While Loops vs. For Loops # While loop i = 0 while i < 10: print(i) # For loop for i in range(10): print(i) i = i + 1 ◎ Both code snippets print numbers from 0 to 9. ◎ The for loop version is better. ○ More concise. ○ Easier to read (once we learn about range). ○ Less error prone (can't forget to increment i). 6 For Loops for Variable in Sequence: Statement ◎ While there are items left in the sequence: ○ Assign the next item in the sequence to the iteration variable. ○ Execute the statements in the for block. 7 For Loops ◎ For loops share a lot in common with while loops. ○ You can use continue and break statements to finish early. ○ You can nest them. ○ You must follow the same indentation rules. 8 For Loops 9 For Loops: range () function 10 For Loops- range () function 11 Writing a Python Range ◎ Let's say you have a sequence in mind that you want to express using a Python range. ◎ Firstly you need to check whether this is possible: ○ Are all of the numbers unique integers? ○ Is the sequence an arithmetic sequence (i.e. do items in the sequence increase/decrease by a constant amount)? 12 Valid Range Sequences Valid Range Sequences 🗸 1, 2, 3, 4, 5 🗸 8, 10, 12 🗸 6, 3, 0, -3 🗸 100 Invalid Range Sequences ✗ 1, 4, 9, 16, 25 ✗ 1.1, 2.1, 3.1 ✗ 5, 5, 5, 5 13 Steps For Writing a Python Range 1. Think of the first number in the sequence. This is m. 2. Think of the difference between the second and first item in the sequence. This is step. 3. Think of the last number in the sequence, then add step to it. This is n. The Python code for the sequence is: range(m, n, step) 14 Simplifying Python Ranges ◎ In some instances you can simplify the range. range(m, n, step) ○ If step is 1, you can omit it. ○ If step is 1 and m is 0, you can omit them both. ◎ For example: ○ range(6, 9, 1) simplifies to range(6, 9) ○ range(0, 3, 1) simplifies to range(3) 15 Examples: Writing a Python Range ◎ 0, 1, 2, 3, 4 ○ range(0, 5, 1), or simply range(5) ◎ 1, 2, 3 ○ range(1, 4, 1), or simply range(1, 4) ◎ 0, 2, 4, …, t ○ range (0, t + 2, 2) ◎ y, y-1, y-2, …, y-x ○ range(y, (y-x)-1, -1) 16 Examples: For Loops: 17 Examples: For Loops 18 Examples: For Loops 19 Check Your Understanding Q. Fill in the blank using a Python range such that the program output is 9, 8, 7, 6. for i in ANSWER: print(i) 20 Check Your Understanding Q. Fill in the blank using a Python range such that the program output is 9, 8, 7, 6. A. range(9, 5, -1) 1. The first value is 9. ➢ m=9 2. The first two values are 9, 8. ➢ step = 8 - 9 = -1 3. The last value is 6. ➢ n = 6 + step = 5 for i in ANSWER: print(i) 21 2. Example Program: FizzBuzz 22 What is FizzBuzz? ◎ FizzBuzz is a programming problem often used during software developer job interviews. ◎ Tests knowledge of: ○ Selection and iteration control structures, ○ Boolean expressions, and ○ The modulo operator. ◎ We have learnt about each of these things, so we are now equipped to tackle FizzBuzz! 23 Task Definition Task Definition Write a program that prints the numbers from 1 to 100. But for multiples of three print "Fizz" instead of the number and for the multiples of five print "Buzz". For numbers which are multiples of both three and five print "FizzBuzz". Source: https://imranontech.com/2007/01/24/using-fizzbuzz-to-find-developers-who-grok-coding/ 24 Example Output ◎ The first 16 lines of the expected program output are shown here. 1 2 Fizz 4 Buzz Fizz 7 8 Fizz Buzz 11 Fizz 13 14 FizzBuzz 16 ... 25 Printing Numbers from 1 to 100 ◎ To begin with, let's ignore the fizzing/buzzing and focus on simply printing numbers from 1 to 100. ◎ We know that we can iterate over these numbers using a for loop and a Python range. ◎ How can we express the sequence of numbers from 1 to 100 (inclusive) using a Python range? range(1, 101) 26 Printing Numbers from 1 to 100 for i in range(1, 101): print(i) ◎ The above code will print all integers from 1 to 100, inclusive. 27 Fizzing and Buzzing ◎ Let's now consider the full task definition: Write a program that prints the numbers from 1 to 100. But for multiples of three print "Fizz" instead of the number and for the multiples of five print "Buzz". For numbers which are multiples of both three and five print "FizzBuzz". ◎ We can identify that the program should select one of four different outputs for each number. ○ The number itself (this is the "default" option). ○ "Fizz" (multiple of 3). ○ "Buzz" (multiple of 5). ○ "FizzBuzz" (multiple of 3 and 5). 28 Fizzing and Buzzing ◎ We will need to use a selection structure with four branches. for i in range(1, 101): if Condition 1: print(Output 1) elif Condition 2: print(Output 2) elif Condition 3: print(Output 3) else: print(i) # The "default" option. 29 Fizzing and Buzzing ◎ We are testing conditions based on whether the number is a multiple of other numbers (3 and/or 5). ◎ Some number x is a multiple of another number y if and only if x can be divided by y with no remainder. ○ Recall that the modulo operator calculates the remainder. ○ Hence x is a multiple of y if and only if x % y == 0. 30 Fizzing and Buzzing ◎ Let's pair each output with its condition: ○ multiples of three print "Fizz" ◉ Condition: i % 3 == 0 ◉ Output: "Fizz“ ○ multiples of five print "Buzz" ◉ Condition: i % 5 == 0 ◉ Output: "Buzz“ ○ multiples of both three and five print "FizzBuzz" ◉ Condition: i % 3 == 0 and i % 5 == 0 ◉ Output: "FizzBuzz" 31 First Solution Attempt (Incorrect) for i in range(1, 101): if i % 3 == 0: print("Fizz") elif i % 5 == 0: print("Buzz") elif i % 3 == 0 and i % 5 == 0: print("FizzBuzz") else: print(i) ◎ Can you spot the bug in the code? 32 First Solution Attempt (Incorrect) ◎ The conditions are tested in order of appearance. ◎ Any value of i which satisfies the "FizzBuzz" condition will satisfy the "Fizz" condition. ◎ Since the "Fizz" condition is tested first, the program will output "Fizz" instead of "FizzBuzz". for i in range(1, 101): if i % 3 == 0: print("Fizz") elif i % 5 == 0: print("Buzz") elif i % 3 == 0 and i % 5 == 0: print("FizzBuzz") else: print(i) 33 Second Solution Attempt (Correct) ◎ The bug can be fixed by reordering the branches. ◎ Importantly the most specific condition is tested first. for i in range(1, 101): if i % 3 == 0 and i % 5 == 0: print("FizzBuzz") elif i % 3 == 0: print("Fizz") elif i % 5 == 0: print("Buzz") else: print(i) 34 Check Your Understanding Q. Does the order of the two elif branches matter for getting the correct output? for i in range(1, 101): if i % 3 == 0 and i % 5 == 0: print("FizzBuzz") elif i % 3 == 0: print("Fizz") elif i % 5 == 0: print("Buzz") else: print(i) 35 Check Your Understanding Q. Does the order of the two elif branches matter for getting the correct output? A. No. A value of i which does not satisfy the FizzBuzz condition can never satisfy both the Fizz and Buzz conditions. Therefore the ordering of these conditions does not matter. for i in range(1, 101): if i % 3 == 0 and i % 5 == 0: print("FizzBuzz") elif i % 3 == 0: print("Fizz") elif i % 5 == 0: print("Buzz") else: print(i) 36 3. Aggregation 37 Aggregation ◎ Aggregation is the process of combining values to produce a single summary value. ◎ Some common aggregations include: ○ Sum, ○ Average (mean), and ○ Maximum value. ◎ Using a for loop is a convenient way of aggregating. 38 Aggregation Pattern ◎ A general pattern for aggregation is: 1. Define one (or more) summary variables, using suitable initial values. 2. Write a for loop to update the summary variable(s) using each item in the sequence. 3. Use the summary variable(s) to calculate the final result. ◎ Step 3 is not always necessary---in some cases the summary variable itself is the result. 39 Aggregation: Sum ◎ This program sums the numbers from 1 to 9. total = 0 for x in range(1, 10): total = total + x print(total) ◎ Summary variable: total. ◎ We keep track of the running total as each item in the sequence is considered. 40 Aggregation: Average (Mean) ◎ This program averages the numbers from 1 to 9. ◎ Summary variables: total and count. total = 0 count = 0 for x in range(1, total = total count = count average = total / 10): + x + 1 count print(average) ◎ We keep track of both the total sum and the count, then calculate the average at the end by dividing. 41 Aggregation: Maximum ◎ This program finds the maximum value from a list. ○ More about lists in a future lecture. maximum = 0 for x in [3, 1, 8, 4]: if x > maximum: maximum = x print(maximum) ◎ Summary variable: maximum. ◎ An if statement is used to conditionally update maximum whenever a larger value is encountered. 42 Count ◎ This program counts the number of values greater than 5 in a list. count = 0 for x in [4, 9, 5, 8, 9]: if x > 5: count = count + 1 print(count) ◎ Summary variable: count. ◎ The count is incremented for each item which meets the if statement condition. 43 Custom Aggregation ◎ With a little bit of thinking you can devise your own custom aggregations. ◎ You need to: 1. Define the sequence to aggregate. 2. Identify summary variable(s). 3. Identify the repeated operation(s) which builds up the summary variable(s). 4. Use the summary variable(s) to calculate the final result (not always necessary). 44 Custom Aggregation Task Definition Write a program which concatenates all single-digit numbers into one long string surrounded by square brackets and prints the result. 1. The sequence we are iterating over is 0, 1, …, 9. 2. The summary variable is the string being built. 3. The repeated operation is converting the item to a string and concatenating it with the summary variable. 4. The final result is the summary variable in square brackets. 45 Custom Aggregation s = '' for x in range(10): s = s + str(x) print('[‘ + s + ']’) #output >>> [0123456789] 1. The sequence we are iterating over is 0, 1, …, 9. 2. The summary variable is the string being built. 3. The repeated operation is converting the item to a string and concatenating it with the summary variable. 4. The final result is the summary variable in square brackets. 46 Custom Aggregation ◎ If you are still unsure about how this code works, think about what it looks like with the loop "unrolled". ◎ Recall that the str function converts a value to a string. ○ e.g. str(0) gives '0' s = '' for x in range(10): s = s + str(x) print('[‘ + s + ']') s = s = s = ... s = '' s + str(0) s + str(1) s + str(9) print('[‘ + s + ']') 47 Check Your Understanding Q. Which program correctly calculates the product of values between 1 and 10 (inclusive)? # Program A. prod = 1 for x in range(1, 11): prod = prod * x print(prod) # Program B. prod = 0 for x in range(1, 11): prod = prod * x print(prod) # Program C. prod = 1 for x in range(1, 11): x = prod * x print(prod) 48 Check Your Understanding Q. Which program correctly calculates the product of values between 1 and 10 (inclusive)? A. Program A. ◎ Program B uses an inappropriate initial value and will output 0. ◎ Program C does not update the summary variable. # Program A. prod = 1 for x in range(1, 11): prod = prod * x print(prod) # Program B. prod = 0 for x in range(1, 11): prod = prod * x print(prod) # Program C. prod = 1 for x in range(1, 11): x = prod * x print(prod) 49 Nested Loops 50 Nested Loops 51 Nested Loops 52 Summary 53 In This Lecture We... ◎ Iterated over ranges of numbers using for loops. ◎ Used selection and iteration to solve a common coding interview question. ◎ Performed aggregation using for loops. 54 Next Lecture We Will... ◎ Discover how functions allow us to use and write reusable chunks of code. 55 Thanks for your attention! The slides and lecture recording will be made available on LMS. The “Cordelia” presentation template by Jimena Catalina is licensed under CC BY 4.0. PPT Acknowledgement: Dr Aiden Nibali, CS&IT LTU. 56