finalbook.pdf
Document Details
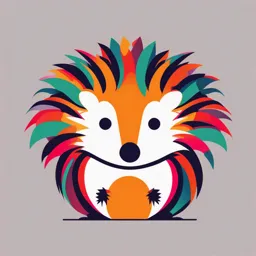
Uploaded by FasterNeptune
Tags
Related
- Computer Programming 1 - Introduction to C++ PDF
- bsc-3-sem-computer-science-ad-1833-s-2023.pdf
- Computer Science - XI - Part I PDF
- 22316 Object-Oriented Programming using C++ Sample Question Paper PDF
- Lecture 1: Introduction to C++ Programming and Computer Science PDF
- Intro to Computer Science - For Loop & Control Structures PDF
Full Transcript
1 Structures and Pointers W e started writing C++ programs in Significant Learning Outcomes Class X...
1 Structures and Pointers W e started writing C++ programs in Significant Learning Outcomes Class XI for solving problems. Almost all problems are related to After the completion of this chapter, the the processing of different types of data. Last learner year we came across only elementary data items identifies the need of user-defined such as integers, fractional numbers, characters data types and uses structures to represent grouped data. and strings. We used variables to refer to these creates structure data types and data and the variables are declared using basic accesses elements to refer to the data types of C++. We know that all data is data items. not of fundamental (basic) types; rather many uses nested structures to represent of them may be composed of elementary data data consisting of elementary data items. No programming language can provide items and grouped data items. data types for all kinds of data. So develops C++ programs using programming languages provide facility to structure data types for solving real define new data types, as desired by the users. life problems. In this chapter, we will discuss such a user- explains the concept of pointer and defined data type named structure. This uses pointer with the operators & chapter also discusses a new kind of variable and *. known as pointer. The concept of pointers is a compares the two types of memory typical feature of languages like C, C++. It allocations and uses dynamic helps to access memory locations by specifying operators new and delete. illustrates the operations on the memory addresses directly, which makes pointers and predicts the outputs. execution faster. A good understanding of this establishes the relationship concept will help us to design data structure between pointer and array. applications and system level programs. uses pointers to handle strings. Last year you might have used GNU Compiler explains the concept of self Collection (GCC) with Geany IDE or Turbo referential structures. C++ IDE for developing C++ programs. Computer Science - XII GCC differs from Turbo C++ IDE in the structure of source code, the way of including header files, the size of int and short, etc. In this chapter the concepts are presented based on GCC. 1.1 Structures Now-a-days students, employees, professionals, etc. wear identity cards issued by institutions or organisations. Figure 1.1 shows the identity card of a student. The first column of Table 1.1 contains some of the data printed on the card. You try to fill up the second column with the appropriate C++ data types discussed in Class XI. Data C++ data type 12345 Sneha S. Raj 20/02/1997 O+ve Snehanilayam, Gandhi Nagar, Chemmanavattom, Fig.1.1: ID card of a student Pin 685 531 Table 1.1: Data and C++ data types You may use short or int for admission number (12345), char array for name (Sneha S. Raj), blood group (O +ve) and even address. Sometimes you may not be able to identify the most appropriate data types for date of birth and address. Let us consider the data 20/02/1997 and analyse the composition of this data. It is composed of three data items namely day number (10), month number (02) and year (1997). In some cases, the name of the month may be used instead of month number. Address can also be viewed as a composition of data items such as house number/name, place, district, state and PIN code. Even the entire details on the identity card can be considered as a single unit of data. Such data is known as grouped data (or aggregate data or compound data). C++ provides facility to define new data types by which such aggregate or grouped data can be represented. The data types defined by user to represent data of aggregate nature are generally known as user-defined data types. Structure is a user-defined data type of C++ to represent a collection of logically related data items, which may be of different types, under a common name. We learnt array in Class XI, to refer to a collection of data of the same type. But structure 8 1. Structures and Pointers can represent a group of different types of data under a common name. Let us discuss how a structure is defined in C++ and elements are referenced. 1.1.1 Structure definition While solving problems, the data to be processed may be of grouped type as mentioned above. We have to define a suitable structure to represent such grouped data. For that, first we have to identify the elementary data items that constitute the grouped data. Then we have to adopt the following syntax to define the structure. struct structure_tag { data_type variable1; data_type variable2;...................;...................; data_type variableN; }; In the above syntax, struct is the keyword to define a structure, structure_tag (or structure_name ) is an identifier and variable1 , variable2 , …, variableN are identifiers to represent the data items constituting the grouped data. The identifier used as the structure tag or structure name is the new user- defined data type. It has a size like any other data types and it can be used to declare variables. This data type can also be used to specify the arguments of functions and as the return type of functions. The variables specified within the pair of braces are known as elements of the structure. The data types preceded by these elements may be basic data types or user-defined data types. These data types determine the size of the structure. Now let us define a structure to represent dates of the format 20/02/1997 (as seen in the ID card). We can see that this format of date is constituted by three integers which can be represented by int data type of C++. The following is the structure definition for this format of date: struct date { int dd; int mm; int yy; }; 9 Computer Science - XII Here, date is the structure tag (structure name), and dd, mm and yy are the elements of the structure date, all of them are of int type. If we want to specify the month as a string (like January instead of 1), the definition can be modified as: struct strdate { int day; char month; // name of month is a string int year; }; While writing programs for solving problems, some of the data involved may be logically related in one way or the other. In such cases, the concept of structure data type can be utilised effectively to combine the data under a common name to represent data compactly. For example, the student details such as admission number, name, group, fee, etc. are logically related and hence a structure can be defined as follows: struct student { int adm_no; char name; char group; float fee; }; Now, try to define separate structures yourself to represent address and blood group. Blood group consists of group name and rh value. We know that the details of an employee may consist of employee code, name, gender, designation and salary. Define a suitable structure Let us do to represent these details. We have discussed the way of defining a structure type data. Can we now store data in it? No, it is simply a data type definition. Using this data type we should declare a variable to store data. 1.1.2 Variable declaration and memory allocation As in the case of basic data items, a variable is required to refer to a group of data. Once we have defined a structure data type, variable is declared using the following syntax: struct structure_tag var1, var2,..., varN; OR structure_tag var1, var2,..., varN; 10 1. Structures and Pointers In the syntax, structure_tag is the name of the structure and var1, var2,..., varN are the structure variables. Let us declare variables to store some dates using the structure date and fulldate. date dob, today; OR struct date dob, today; strdate adm_date, join_date; We know that variable declaration statement causes memory allocation as per the size of the data type. What will be the size of a structure data type? Since it is user- defined, the size depends upon the definition of the structure. The definition of date shows that its variables require 12 bytes each because it contains three int type elements (size of int in GCC is 4 bytes). The memory allocation for the variable join_date of strdate type is shown in Figure 1.2. join_date Fig. 1.2: Memory allocation for a structure variable The size of int type in GCC is 4 bytes and in Turbo IDE, it is 2 bytes. In Figure 1.2, the elements day and year are provided with 4 bytes of memory since we follow GCC. Since this much memory is not required for storing values in these elements, it is better to replace int with short. The variable join_date consists of three elements day, month and year. These elements require 4 bytes, 10 bytes and 4 bytes, respectively and hence the memory space for join_date is 18 bytes. Now you find the size of the structure student defined earlier. Also write the C++ statement to declare a variable to refer to the details of a student and draw the layout of memory allocated to this Let us do variable. A structure variable can be declared along with the definition also, as shown below: struct complex { short real; short imaginary; }c1, c2; This structure, named complex can represent complex numbers. The identifiers c1 and c2 are two structure variables, each of which can be used to refer to a 11 Computer Science - XII complex number. If we declare structure variables along with the definition, structure tag (or structure name) can be avoided. The following statement declares structure variables along with the definition. struct { int a, b, c; }eqn_1, eqn_2; There is a limitation in this type of definition cum declaration. If we want to declare variables, to define functions, or to specify arguments using this structure later in the program, it is not possible since there is no tag to refer. The above structure also shows that if the elements (or members) of the structure are of the same type, they can be specified in a single statement. Variable initialisation During the declaration of variables, they can be assigned with some values. This is true in the case of structure variables also. When we declare a structure variable, it can be initialised as follows: structure_tag variable={value1, value2,..., valueN}; For example, the details of a student can be stored in a variable during its declaration itself as shown below: student s={3452, "Vaishakh", "Science", 270.00}; The values will be assigned to the elements of structure variable s in the order of their position in the definition. So, care should be given to the order of appearance of the values. The above statement allocates 38 bytes of memory space for variable s, and assigns the values 3452, "Vaishakh", "Science" and 270.00 to the elements adm_no, name, group and fee of s, respectively. If we do not provide values for all the elements, the given values will be assigned to the elements on First Come First Served (FCFS) basis. The remaining elements will be assigned with 0 (zero) or '\0' (null character) depending on numeric or string. A structure variable can be assigned with the values of another structure variable. But both of them should be of the same structure type. The following is a valid assignment: student st = s; This statement initialises the variable st with the values available in s. Figure 1.3 shows this assignment: 12 1. Structures and Pointers s Fig. 1.3: Structure assignment While defining a structure, the elements specified in it cannot be assigned with initial values. Though the elements are specified using the syntax of variable declaration, memory is not allocated for the structure definition statement. Hence, values cannot be assigned to them. The structure definition can be considered as the blue print of a house. It shows the number of rooms, each with a specific name and size. But nothing can be stored in these rooms. The total space of the building will be the sum of the spaces of all rooms in the house. Any number of houses can be constructed based on this plan. All of them will be the same in terms of number of rooms and space, but each house will be given different name. Structure definition is the blue print and structure variables are the realisation of the blue print (similar to the construction of houses based on the blue print). Each variable can store data in its elements (similar to the placing of furniture, house-hold items and residents in rooms). 1.1.3 Accessing elements of structure We know that array is a collection of elements and these elements are accessed using the subscripts. Structure is also a collection of elements and C++ allows the accessibility to the elements individually. The period symbol (.) is provided as the operator for this purpose and it is named dot operator. The dot operator (.) connects a structure variable and its element using the following syntax: structure_variable.element_name In programs, the operations on the structure data can be expressed only by referring the elements of the structure. The following are some examples for accessing the elements: today.dd = 10; strcpy(adm_date.month, "June"); cin >> s1.adm_no; cout >s.te; tot_score=s.ce+s.pe+s.te; cout