Programming II Course 8 - Special Keywords 2024 PDF
Document Details
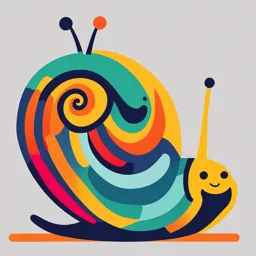
Uploaded by GenialAntigorite2219
Transilvania University of Brașov
2024
Bianca Iacob
Tags
Summary
This document is a set of lecture notes on C++ programming keywords, focusing on const, constexpr, inline, =default, =delete, noexcept, and auto. It includes explanations, syntax, examples, and advantages/disadvantages of each keyword.
Full Transcript
Programming II Course 8 - Special Keywords Bianca Iacob Transilvania University of Brasov 2024 Bianca Iacob Transilvania University of Brasov 2024 1 / 52 Contents 1 const 2 constexpr...
Programming II Course 8 - Special Keywords Bianca Iacob Transilvania University of Brasov 2024 Bianca Iacob Transilvania University of Brasov 2024 1 / 52 Contents 1 const 2 constexpr 3 inline 4 =default 5 =delete 6 noexcept 7 auto Bianca Iacob Transilvania University of Brasov 2024 2 / 52 const const Bianca Iacob Transilvania University of Brasov 2024 3 / 52 const const The const keyword is used to define a constant value that cannot be changed during program execution. It means that once we declare a variable as constant in a program, the value of the variable will be fixed and will never be changed. If we try to change the value of the const variable, it displays an error message in the program. Bianca Iacob Transilvania University of Brasov 2024 4 / 52 const The const keyword can be associated with: a variable a method a pointer an object of a class Bianca Iacob Transilvania University of Brasov 2024 5 / 52 const Const variable We must initialize a constant variable from the moment of declaration. => otherwise we will get a compile error. int main() { const int a = 10; const float b = 3.6; const std::string c = “abc”; } Bianca Iacob Transilvania University of Brasov 2024 6 / 52 const Const method When a function is declared as const, it can be called on any type of object, const object as well as non-const objects. Whenever an object is declared as const, it must be initialized at the time of declaration. class Base { public: void myMethod() const; }; Bianca Iacob Transilvania University of Brasov 2024 7 / 52 const Const parameter The variable x cannot be changed inside the method myMethod. class Base { public: void myMethod(const MyObj& x); }; Bianca Iacob Transilvania University of Brasov 2024 8 / 52 const Const return type The return type is constant and will return a constant integer value. class Base { public: const int myMethod(); }; Bianca Iacob Transilvania University of Brasov 2024 9 / 52 const Const data members To initialize the const value using the constructor, we need to use the initializer list. class Base{ private: const int x; public: Base(int a) : x(a){} }; Bianca Iacob Transilvania University of Brasov 2024 10 / 52 const Const pointer Pointers can be declared as const in three situations: 1 Pointer to a constant variable const data_type* variable_name; 2 Constant pointer to a variable data_type* const variable_name; 3 Constant pointer to a constant variable const data_type* const variable_name; Bianca Iacob Transilvania University of Brasov 2024 11 / 52 const Const object The constructor and destructor can modify the data members of the object, other methods of the class cannot. int main() { const MyClass obj; } Bianca Iacob Transilvania University of Brasov 2024 12 / 52 constexpr constexpr Bianca Iacob Transilvania University of Brasov 2024 13 / 52 constexpr constexpr It was added in C++11. The main idea is to improve the performance of programs by performing calculations at compile time. Bianca Iacob Transilvania University of Brasov 2024 14 / 52 constexpr constexpr can only call other constexpr functions. constexpr functions cannot return void type. Any constexpr variable must be initialized at declaration time. Bianca Iacob Transilvania University of Brasov 2024 15 / 52 constexpr constexpr can only return literal types: void scalar types references arrays of void, scalar types or references A class that has a trivial destructor and one or more constexpr constructors that are not move or copy constructors. Additionally, all non-static data members and base classes must be literal types Bianca Iacob Transilvania University of Brasov 2024 16 / 52 constexpr Example int main() { constexpr int x = 3; constexpr float y = 3.2; constexpr char z = 'z'; return 0; } Bianca Iacob Transilvania University of Brasov 2024 17 / 52 constexpr Example constexpr int dimensiune(int x, int y) { return x*y; } int main() { int v[dimensiune(1,3)] = {1, 2, 3}; return 0; } Bianca Iacob Transilvania University of Brasov 2024 18 / 52 constexpr Example class Base { public: Base(int x) : x(x) { cout