COMPROG NOTES.pdf
Document Details
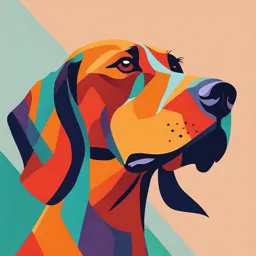
Uploaded by CleanMiracle7675
University of Santo Tomas
Tags
Related
- OOP - JAVA Object-Oriented Programming PDF
- Topic 1.6 Running Program with Objects PDF
- CS0070 Object-Oriented Programming Module 1 PDF
- Object Oriented Programming (2nd Year 1st Sem) PDF
- Introduction to Java Programming and Data Structures (2019) by Y. Daniel Liang - PDF
- VILLASAN Object-Oriented Programming PDF
Full Transcript
CHAPTER 3 OBJECTS AND REFERENCE VARIABLES Reference variables - stores the address of the object containing the data. In Java, variables such as str are called reference variables. More formally, reference variables are variables that store the address of a memo...
CHAPTER 3 OBJECTS AND REFERENCE VARIABLES Reference variables - stores the address of the object containing the data. In Java, variables such as str are called reference variables. More formally, reference variables are variables that store the address of a memory space. In Java, any variable declared using a class (such as the variable str) is a reference variable. String object - instance of a class. The memory space 2500, where the string (literal) "Java Programming" is stored, is called a String object. Instances of the class String - String objects Instantiating an object of a class - Using the operator new to create a class object Operator new - used to instantiate an object of a class “string variable refVar” - pertains to the address stored in refVar “Object/string refVar” - pertains to the object whose address is stored in refVar String objects are immutable, meaning that once they are created, they cannot be changed. However you can declare another statement with the same variable and a new object, but the address of the allocated memory space will be different from that in the first statement. Garbage collection - once you declare another statement with the same variable and a new object, the memory space of that old object will be reclaimed by the Java system for later use. To manually do garbage collection, the statement is: System.gc(); Primitive type variables (int, double, char) - store data directly into their own memory space Non-primitive type variables (String) - store the address of an object’s memory space, into their own memory space USING PREDEFINED CLASSES AND METHODS IN A PROGRAM Method - is a collection of instructions. Distinguished from (reference) variables by the presence of parentheses Predefined classes - a class that is already defined and available for use without the need for the programmer to create it from scratch. Java comes with a wealth of of classes called predefined classes. (Ex: String, Math) Class libraries - predefined classes organized as a collection of packages Package - contains several classes (Ex. java.lang, java.util). You need to know the name of the package containing the class and import this class from the package in the program. Class/es - contain several methods (Ex. Scanner) TWO TYPES OF METHODS Static - can be used using the name of the class containing the method. (Ex: Every method of the class Math is a static method. Therefore, you can use every method of the class Math using the name of the class, which is Math.) Non-static - MATH CLASS Ex. Math.pow(x,y) where x is the base and y is the exponent Math.pow(2,3) = 2^3 = 8 Parameters -The numbers x and y used in the method pow Method call - An expression such as Math.pow(2, 3). Causes the code attached to the method pow to execute Methods that have no parameters must have empty parentheses (Ex: nextInt()) DOT BETWEEN CLASS (OBJECT) NAME AND CLASS MEMBER: A PRECAUTION Member access operator - The operator dot (.) in Java Dot - separates the class variable name, that is, the object name from the method. For example, console is the name of a (reference) variable, and nextInt is the name of a method. (console.nextInt();) CLASS STRING Index - Position of a character in a string (Ex. “Hello”, the index of character ‘H’ is 0, ‘e’ is 1, and so on and so forth) General expression to use a String method StringVariable.StringMethodName(parameters) sentence.length(); //=21 But the label for the last character is 20 because the counting starts with 0 Commonly Used String Methods char charAt(int index) OUTPUT: the character at the position specified by index Ex. sentence.charAt(3) returns ‘g’ int indexOf(char ch) OUTPUT: index of the FIRST occurrence of the character specified by ch If the specified character does not appear in the string OUTPUT: -1 Ex. sentence.indexOf(‘J’) returns 17 sentence.indexOf(‘a’) returns 5 int indexOf(char ch, int pos) OUTPUT: index of the first occurrence of the character specified ch. The parameter pos specific where to begin the search. If the specified character does not appear in the string OUTPUT: -1 Ex. sentence.indexOf(‘a’, 10) returns 18 int indexOf(String str) OUTPUT: index of the first occurrence of the string specified by str If the string specified by str does not appear in the string OUTPUT: -1 Ex. sentence.indexOf(“with”) returns 12 sentence.indexOf(“ing” returns 8 int indexOf(String str, int pos) OUTPUT: Returns the index of the first occurrence of the String specified by str. The parameter pos specific where to begin the search. If the string specified by str does not appear in the string OUTPUT: -1 Ex. sentence.indexOf("a", 10) returns 18 sentence.indexOf("Pr", 10) returns -1 String concat(String str) OUTPUT: Returns the string that is this string concatenated with str Ex. String sentence = "Programming with Java"; System.out.println(""+sentence.concat(" is fun.")); Returns the string “Programming with Java is fun.” int compareTo(String str) Compares two strings character by character OUTPUTS: Returns a negative value if this string is less than str Returns 0 if this string is same as str Returns a positive value if this string is greater than str Ex. String name = "Lisa Johnson"; String sentence = "Programming with Java"; String sentence2 = "Programming with Java"; // System.out.println(""+name.compareTo(sentence)); Returns -4 System.out.println(""+sentence.compareTo(name)); Returns 4 System.out.println(""+sentence.compareTo(sentence2)); Returns 0 Boolean equals(String str) OUTPUT: Returns true if this string is same as str Ex. System.out.println(""+sentence.equals(sentence2)); Returns true String replace(char charToBeReplaced, char charReplacedWith) OUTPUT: the string in which every occurrence of charToBeReplaced is replaced with charReplacedWith Ex. sentence.replace('a', '*') returns the string “Progr*mming with J*v*” String substring(int beginIndex) OUTPUT: the string which is a substring of this string beginning at beginIndex until the end of the string. Ex. sentence.substring(12) returns the string "with Java" String substring(int beginIndex, int endIndex) OUTPUT: Returns the string which is a substring of this string beginning at beginIndex until endIndex – 1 Ex. System.out.println(""+sentence.substring(0,13)); Returns “Programming w” Basta memorize m n yan puta FORMATTING OUTPUT WITH PRINTF Float values - 6 decimal places Double values - 15 decimal places formatString - string specifying the format of the output argumentList - list of arguments that consists of constant values, variables, or expressions. If more than one argument, arguments are separated with commas Format specifiers - ung mga %.2f, %d ganern %[argument_index$][flags][width][.precision]conversion PARSING NUMERIC STRINGS Numeric string - string consisting of only an integer or a floating point number, optionally preceded by a minus sign. Ex. “6723”, “-823”, “321.43”, “-356.258” Converting numeric string to their equivalent numeric form for addition or multiplication String to int Integer.parsInt(“6723”) = 6723 Integer.parseInt(“-823”) = -823 String to float Float.parseFloat(“34.56”) =34.56 Float.parseFloat("-542.97") = -542.97 String to double Double.parseDouble("345.78") = 345.78 Double.parseDouble("-782.873") = -782.873 CHAPTER 4 CONTROL STRUCTURES A computer can process a program in one of three ways - In sequence - By making a selection or a choice (branch) - By repetition, executing a statement over and over (loop) Two most common control structures Selection - program executes particular statements depending on one or more conditions Repetition - program repeats particular statements a certain number of times depending on one or more conditions Branch - altering flow of program by making a selection or choice Loop - altering flow of program execution by the repetition of statement(s) RELATIONAL OPERATORS Logical (boolean) expression - expression that has a value of either true or false Logical (boolean) values - values true and false Relational operator - operator that allows you to make comparisons in a program. Equality operator - determines whether two expressions are equal, == Assignment operator - assigns the value of an expression to a variable, = SELECTION: if and if-else One-way selection if(logical expression) Statement Conditional - also called logical expression Action statement - statement following the logical expression CONDITIONAL (? :) (optional) Conditional operator (? :) is a ternary operator SYNTAX: Expression ? expression2 : expression3 (called a conditional expression) If expression 1 is true, it outputs expression2, otherwise, it outputs expression3 SWITCH STRUCTURES The expression is also called a selector