Class 8 Python Chapter 8 PDF
Document Details
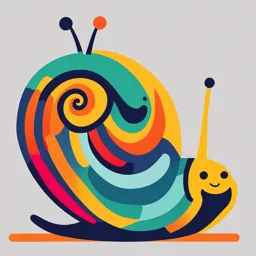
Uploaded by GlimmeringAsteroid
Tags
Related
Summary
This document introduces Python functions, explaining their features, components, and types, including built-in and user-defined functions. It provides examples and explanations. The document covers basic Python concepts for beginners.
Full Transcript
# INTRODUCING PYTHON FUNCTIONS It is always advisable to perform a larger task after dividing it down into a set of manageable tasks. This division enables us to manage the task in a more organised manner. In a programming language, functions help us break our program into smaller pieces, or modu...
# INTRODUCING PYTHON FUNCTIONS It is always advisable to perform a larger task after dividing it down into a set of manageable tasks. This division enables us to manage the task in a more organised manner. In a programming language, functions help us break our program into smaller pieces, or modules. In a programming language, functions help organize a program. Like any other programming language, Python also allows us to use functions in a program. A function can be defined as a block of reusable code that performs a specific task. This concept is the foundation upon which the procedural programming concept operates. # FEATURES OF FUNCTION Functions are the basis of procedural programming. Some important features of functions are: - A program is divided into small modules and each module performs some specific task. Each module can be called as per the requirement. - We can call a function as many times as required. This saves the programmer time and effort to rewrite the same code again. Therefore, it also reduces the length of the program. - Dividing a larger program into smaller functions makes the program more manageable. It makes error detection easy and makes the code efficient, both in terms of time and memory. # COMPONENTS OF PYTHON FUNCTION A Python function consists of the following components: - **Name of the function:** A function name should be unique and easy to correlate with the task it will perform. We can have functions of the same name with different parameters. - **Arguments:** The input given to the functions is referred to as arguments. A function can or cannot have any arguments. - **Statements:** The statements are the executable instructions that the function can perform. - **Return Value:** A function may or may not return a value. # TYPES OF FUNCTIONS IN PYTHON We have mentioned earlier that one of the strengths of the Python language is that Python functions are easy to define and use. Python functions can be categorized into built-in functions and user-defined functions. Let us discuss about them in detail. ## Built-In Functions The `print()` and `input()` belong to the category of built-in functions. We also have other built-in functions like `range()`, `type()`, etc. The main difference between these two categories is that built-in functions do not require to be written by us whereas a user-defined function has to be developed by the user at the time of writing a program. ## User-defined Functions User-defined functions are created by the user according to the needs of the program. Once the user defines a function, the user can call it in the same way as the built-in functions. User-defined functions are divided into various categories based on their parameters and return type. The functions that do not take any parameters and do not return any values are called type 1 functions. Type 2 functions take parameters but do not return anything. The type 3 functions take parameters and return values. Let us understand this with the help of an example: #### Program 1: To add two numbers using all the three types of user-defined functions ```python # Type1 Function: def add1(): a = int(input("Enter the first number: ")) b = int(input("Enter the second number: ")) c = a + b print("The sum of both the numbers is", c) # Type2 Function: def add2(a, b): c = a + b print("The sum of both the numbers is", c) # Type3 Function: def add3(a, b): c = a + b return (c) print("Calling Type1 function") add1() print("Calling Type2 Function") add2(12, 16) print("Calling Type 3 function") result = add3(20, 30) print(result) ``` #### Creating a Function We can create a function in the following ways: - **Defining a Function:** We use the `def` keyword to begin the function definition. - **Naming a Function:** Provide a meaningful name to your function. - **Supply Parameters:** The parameters (separated by commas) are given in the parenthesis following the name of the function. These are basically the input values we pass to the function. - **Body of the Function:** The body of the function contains Python statements that make our function perform the required task. Syntax of creating a function is: ```python def <name of the function> (list of parameters) <body> ``` #### Calling a Function A function can be called anytime from other functions or from the command prompt after the definition. For calling a function, we type the function name and pass the parameters. For example: #### Program 2: To call a function ```python def my_function(): print("Hello") my_function() ``` #### Program 3: To print a string by calling a function ```python # Function Example def hello(name): print("Hello " + name + "! How do you do?") # Calling Function by passing parameter hello('Orange') ``` # STRING A sequence of characters that is enclosed or surrounded by single (') or double (") quotes is known as a string. The sequence may include a letter, number, special characters or a backslash. Python treats single quotes as double quotes. #### Example of a string: ```python str = "This is Shweta's pen." print(str) ``` #### Creating Strings Strings can be created by enclosing characters inside single or double quotes. It is like assigning a value to a variable. #### Program 4: To create a string ```python first_name = "Aadya" last_name = "Kaushal" str = " " # Empty string: It does not hold any value and # displays an empty space when printed. ``` ## Multiline Strings A multiline string in Python begins and ends with either three single quotes or three double quotes. Quotes, tabs, or newlines in between the “triple quotes” are considered part of the string. For example, ```python ress = """ = 44A, Street No 1 Kailash Colony New Delhi """ ``` ## Escape Sequences With Strings Escape sequences or characters are used to insert special characters that are invalid in Python. These are special characters and to insert them in a string, we must escape them with a backslash character in front of them. An escape sequence is a sequence of characters that does not represent itself when used inside a character or string. It is typically used to specify actions such as carriage returns and tab movements. The backslash (\) is a special character and is also known as the escape character in Python. It is used to represent white space characters, for example, `\t` for tab, `\n` for new line, and `\r` is for carriage return. #### Program 5: To print special symbols using escape sequences ```python print("I love programming in\n Python") ``` #### Program 6: To print multi line strings ```python print('''A sequence of characters is called a string. Strings are used by programming languages to manipulate text such as words and sentences.''') ``` # TRAVERSING A STRING Traversing means visiting each element and processing it as required by the program. We can access the characters of a string one at a time using indexing. There are two ways of indexing a list: - **Index with positive integers:** Index from left starts with 0. - **Index with negative integers:** Index from right starts with -1. Syntax for traversing a string: `<name of the string> [index]` #### Program 7: To access different elements of a string ```python name = "Orange" print (name[0]) print(name[-1]) ``` # STRING BUILT-IN FUNCTIONS Python includes the following built-in functions to manipulate strings: - **`len()`:** The `len()` function calculates and returns the length of a string supplied as an argument. Syntax of using `len()` function is: `len(string_name)` - **`lower()`:** The `lower()` function converts all uppercase letters to lowercase. Syntax of using `lower()` function is: `string_name.lower()` - **`upper()`:** The `upper()` function converts all lowercase letters to uppercase. Syntax of using `upper()` function is: `string_name.upper()` - **`capitalize()`:** The `capitalize()` function returns a string with the first character in capital. Syntax of using `capitalize()` function is: `string_name.capitalize()` #### Program 10: To use various string built-in functions ```python str = "Hello" print (len(str)) print("HELLO".lower()) print("hello".upper()) print("hello".capitalize()) ``` # LIST In Python, a list is a type of container that is used to store a list of values of any type. One can store an integer, string as well as objects in a single list. Each element in a list is assigned an index number. The first index is 0, the second index is 1, the third is 2, and so on. ## Creating a List A list can be created by inserting the elements in square brackets []. The elements in the list are separated by a comma. #### Program 11: To create a list ```python a = [10, 20, 30, 40, 50] print(a) ``` Let us study some of the ways through which we can create different types of lists. ## Empty List An empty list in Python is created using []. #### Program 12: To create an empty list ```python a = [] print(a) ``` ## Mixed Data Type List Mixed data type list can be created by placing values of different data types such as integers, strings, double, etc. #### Program 13: To create a mixed data type list ```python a = ['Ravi', 'Bhatt', 12, 13, 14] print(a) ``` ## Nested List A list can have a list in itself as an element. #### Program 14: To create a nested list ```python a = ['Lakshaya', 'Rohit', 10, 20, [1,2,3], 7] print(a) ``` ## Accessing List Items We use an index number to access the list items just like strings. Use the index operator [ ] to access the elements of a list. #### Program 15: To access the different elements of a list ```python List1 = [1, 2, 3, 11, 12, 13, 'Computer', 40, 'Science'] print (List1[2]) print (List1[6]) print (List1[-2]) ``` # FUNCTIONS Let us study some of the functions we can use with lists. ## append() Function The `append()` function inserts the object passed to it at the end of the list. Syntax of using `append()` function is: ```python list_name.append(item) ``` #### Program 16: To add an element to a list using append() function ```python a = [1, 2, 3, 4, 5] a.append(6) print(a) ``` ## extend() Function Unlike the `append()` function, we can only add one element at a time. For adding more than one element, we use the `extend()` function. Syntax of using `extend()` function is: ```python list_name.extend(iterable value) ``` #### Program 17: To add elements to an existing list using extend() function ```python a = [1, 2, 3, 4, 5] a.extend([6, 7, 8, 9]) print(a) ``` ## del() Function We use the `del()` function to remove a sublist (start: stop: step) or a whole list of elements. Syntax for using `del()` function is: ```python del list_name(start : stop : step) ``` #### Program 18: To delete elements from the list ```python a = [1, 2, 3, 4, 5, 6, 7] del a[1:3:2] print(a) ``` #### Program 19: To delete the whole list ```python a= [1, 2, 3, 4, 5, 6, 7] del a ``` ## I KNOW - A sequence of characters that are enclosed or surrounded by a single (') or double (") quotes is known as a string. - A list is a type of container that is used to store a list of values of any type.