Chapter2_IntroductionToPythonProgramming_Part5.pptx
Document Details
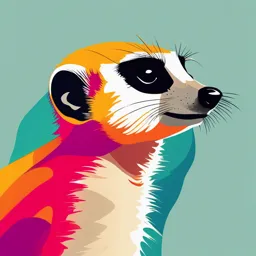
Uploaded by ConstructiveWave
Full Transcript
Chapter 2 Introduction to Python Programming CSC1402 Learning Objectives Python IDEs Define Python Statements Create variables to store data for later use. Use arithmetic operators and comparison operators and understand their pr...
Chapter 2 Introduction to Python Programming CSC1402 Learning Objectives Python IDEs Define Python Statements Create variables to store data for later use. Use arithmetic operators and comparison operators and understand their precedence. Become familiar with built-in data types. Use single-, double- and triple-quoted stings. Use built-in function print to display text. Use built-in function input to prompt the user to enter data at the keyboard and get that data for use in the program. Convert text to integer values with built-in function int. Use comparison operators and the if statement to decide whether to execute a statement or group of statements. Use built-in function type to get an object’s type. Learn about errors in Python Introduction Python is a popular high programming language. It was created by Guido van Rossum, and released in 1991 Python is a high-level language intended to be relatively straightforward for humans to read and write Python's syntax is pretty simple and easy to learn According to Wikipedia definition,” Python is an interpreted high-level, general-purpose, programming language” What is an IDE An integrated development environment (IDE) is a software application that helps programmers develop software code efficiently. IDE key features: Code Editor: A text editor with features like syntax highlighting, code completion, and indentation to make writing code easier and more efficient. Compiler/Interpreter: Built-in tools to compile or interpret the code, allowing developers to run their programs directly within the IDE. Debugger: Tools to help identify and fix errors in code Version Control Integration: Support for version control systems to manage code changes and collaborate with other developers. It increases developer productivity by combining capabilities such as software editing, building and testing in an easy-to-use application. Python IDEs Many IDEs are available for Python: IPython, IDLE, Spyder, PyCharm and Jupyter Notebook. Jupyter Notebook Jupyter Notebook is a web application that allows users to create and share documents that contain code, equations, visualizations, and narrative text. Python Statements A Python statement is an instruction that the Python interpreter can execute There are different types of statements in Python language as Assignment statements, Conditional statements, Looping statements Python has simple and compound statements. A simple statement is a construct that occupies a single logical line like an assignment statement. A compound statement is a construct that occupies multiple logical lines, such as a for loop or a conditional statement. An expression is a simple statement that produces and returns a value. All expressions are statements, not all statements are expressions Variables Variables are containers for storing data values. Creating Variables Python has no command for declaring a variable. A variable is created the moment you first assign a value to it Variable names are case-sensitive Variables Variable Name is called an identifier A variable can have a short name (like x and y) or a more descriptive name (age,carname, total_volume) Rules for Python variables: A variable name must start with a letter or the underscore character A variable name cannot start with a number A variable name can only contain alpha-numeric characters and underscores (A- z, 0-9, and _ ) Variable names are case-sensitive (age, Age and AGE are three different variables) Reserved Keywords Reserved keywords are words in a programming language that have predefined meanings. They are used to develop programming instructions. Reserved keywords cannot be used as identifiers for other elements. Words that Python reserves for its language features. Using a keyword as an identifier causes a SyntaxError: def, while, etc.. Operators An operator is a character or set of characters that can be used to perform the desired operation on the operands. Programming languages typically have operators built in as part of their syntax. In many languages, including Python, you can also create your own operator or modify the behavior of existing ones, which is a powerful and advanced feature to have. This operation can act on one or more operands. If the operation involves a single operand, then the operator is unary. If the operator involves two operands, then the operator is binary. Many different types of operators supported by Python Arithmetic Operators Arithmetic operators are those operators that allow you to perform arithmetic operations on numeric values Grouping Expressions with Parentheses Parentheses group Python expressions, as they do in algebraic expressions Operator Precedence Rules Expressions in parentheses evaluate first, so parentheses may force the order of evaluation to occur in any sequence you desire. Parentheses have the highest level of precedence. In expressions with nested parentheses, such as (a / (b - c)), the expression in the innermost parentheses (that is, b - c) evaluates first. Exponentiation operations evaluate next. If an expression contains several exponentiation operations, Python applies them from right to left. Multiplication, division and modulus operations evaluate next. If an expression contains several multiplication, true-division, floor-division and modulus operations, Python applies them from left to right. Addition and subtraction operations evaluate last. If an expression contains several addition and subtraction operations, Python applies them from left to right. Redundant Parentheses The parentheses are redundant (unnecessary) if removing them yields the same result. You can use redundant parentheses to group subexpressions to make the expression clearer. Assignment Operators Assignment operators are used to assign the values to the variables. Comparison Operators Comparison operators are used to compare compatible values Boolean Operators Boolean operators are used to combine logical expressions. Check Questions 1- Evaluate the expression 3 * (4-5) with and without parenthesis. Are the parenthesis redundant. Data Types Each value in Python has a type that indicates the kind of data the value represents. Function A function is a block of code designed to perform a specific task. Functions help organize code, promote reusability, and improve readability A function runs when it is called. A function can return data as a result. A function performs a task when you call it by writing its name, followed by parentheses, (). The parentheses contain the function’s argument—the data that the function needs to perform its task. Built-in versus custom functions Create a custom function using the def keyword Built-in Type Function Python’s type built-in function determines a value’s type. Displaying Output to the user: Function Print The built-in print function displays its argument(s) as a line of text: 'Welcome to Python!' is a string—a sequence of characters enclosed in single quotes (‘) May enclose in double quotes (“) When print completes its task, it positions the screen cursor at the beginning of the next line. Printing Many Lines of Text with One Statement When a backslash (\) appears in a string, it’s known as the escape character. The backslash is called an escape character in programming because it alters the interpretation of the character that follows it. Essentially, it "escapes" the normal behavior of that character. The backslash and the character immediately following it form an escape sequence. For example, \n represents the newline character escape sequence, which tells print to move the output cursor to the next line. Escape Sequences Printing the Value of an Expression Calculations can be performed in print statements: Including Quotes in Strings In a string delimited by single quotes, you may include double-quote characters: not single quotes a syntax error, which is a violation of Python’s language rules—in this case, a single quote inside a single-quoted string. It displays information about the line of code that caused the syntax error and points to the error with a ^ symbol. It also displays the message SyntaxError: invalid syntax. Including Quotes in Strings A string delimited by double quotes may include single quote characters: not double quotes Including Quotes in Strings Using escape sequences Using Triple-Quoted String Getting Input from the User: Function Input The built-in input function requests and obtains user input : First, input displays its string argument—called a prompt—to tell the user what to type and waits for the user to respond. Lamiae was typed Function input then returns (that is, gives back) those characters as a string that the program can use. Here we assigned that string to the variable name. Getting Input from the User: Function Input Function input Always Returns a String Getting an Integer from the User Convert the string to an integer using the built-in int function: Practice Problem Write a Python code that prompts the user for two numbers and displays their sum and product Comments Comments are used to document code and to improve readability. Comments also help other programmers read and understand the code. They do not cause the computer to perform any action when the code executes. Comments start with a # or comments between ‘ ‘ ‘ ‘ ‘ ‘ Decision Making: The if Statement and Comparison Operators A condition is a Boolean expression with the value True or False. True and False are keywords True and False are each capitalized Comparison Operators Errors in operators A syntax error occurs when any of the operators ==, !=, >= and = and and =