c-Lecture-2024-2025-.pptx
Document Details
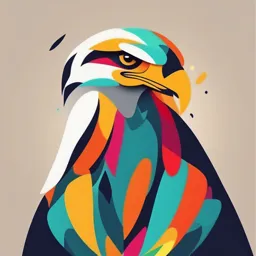
Uploaded by SimplifiedSaturn
Tags
Full Transcript
PROGRAMMING IN C# Shalaw Rasul Hassan Chapter : 1 Basic Structure of C# Program Learning objective What is C#.NET? Advantages of using the.NET Framework from the C# point of view. Different Types of applications are developed using C#.NET. What is the visual studio? What...
PROGRAMMING IN C# Shalaw Rasul Hassan Chapter : 1 Basic Structure of C# Program Learning objective What is C#.NET? Advantages of using the.NET Framework from the C# point of view. Different Types of applications are developed using C#.NET. What is the visual studio? What is a console application? How to Create a console application using visual studio? Understanding the Basic Structure of a C# Program. Importing section Namespace Declaration Class Declaration Main() method What is C#.NET? C#.NET is one of the Microsoft Programming Languages to work with the.NET Framework,.NET Core, or.NET to develop different kinds of applications such as Web, Console, Windows, Restful Services, etc. It is the most powerful programming language among all programming languages available in the.NET framework because it contains all the features of C++, VB.NET, and JAVA, and also has some additional features. As we progress in this course, you will come to know the additional features. C#.NET is a completely Object-Oriented Programming Language. It means it supports all 4 OOPs Principles i.e. Abstraction, Encapsulation, Inheritance, and Polymorphism. Different Types of Applications are Developed using C#.NET. Windows Applications Web Applications Restful Web Services SOAP Based Services Console Applications Class Library, ETC. What is the Visual Studio? Visual Studio is one of the Microsoft IDE tools. Using this tool, we can develop, build, compile, publish and run applications with the.NET framework. This tool provides some features such as Editor Compiler Interpreters, and Many More What is a Console Application? A console application is an application that can be run in the command prompt. For any beginner on.NET it is the first step to learning the C# Language. The Console Applications contain a similar user interface to the Operating systems like MS-DOS, UNIX, etc. The Console Application is known as the CUI application because in this application we completely work with the CUI environment. These applications are similar to C or C++ applications. Console applications do not provide any GUI facilities like the Mouse Pointer, Colors, Buttons, Menu Bars, etc. What is a Console Application? A console application is an application that can be run in the command prompt. For any beginner on.NET it is the first step to learning the C# Language. The Console Applications contain a similar user interface to the Operating systems like MS-DOS, UNIX, etc. The Console Application is known as the CUI application because in this application we completely work with the CUI environment. These applications are similar to C or C++ applications. Console applications do not provide any GUI facilities like the Mouse Pointer, Colors, Buttons, Menu Bars, etc. Chapter : 1 Decision Making & Looping The While Statement The process of repeatedly executing a Entry based loop block of statements is known as looping. Is an entry-controlled loop statement. The test condition is evaluated and if the condition is true, then the body of the loop is executed. Test False cond Syntax: initialization; while(test condition) True { Body of the Body of the Loop… loop 3 } Example class DowhileTest { public static void Main ( ) { int two,count,y; two = 2; count=1; System.Console. WriteLine("Multi plication Table \ n"); while(count b ) ? a : b ; return ( x ); } } class NestTesting { public static void Main( ) { Nesting next = new Nesting ( ) ; next.Largest ( 100, 200 ) ; } } 22 Method Parameters For managing the process of passing values & getting back the results, C# employs four kinds of parameters. Value Parameters Reference Parameters Output Parameters Parameter Arrays 23 Pass By Value By default, method using System; parameters are passed by class PassByValue value. { static void change (int m) When a method is invoked, the value of actual parameters { are assigned to the m = m+10; corresponding formal } parameters. public static void Main( ) { Any changes to formal int x = 100; parameters does not affect the change (x); actual parameters. Console.Wri teLine("x =" There are 2 copies of + variables when passed by x); 24 value. } } Pass By Reference We can force the value parameters to be using System; passed by reference. class PassByRef { Use ref keyword. static void Swap ( ref int x, ref int y ) { This does not create a new storage int temp = x; location. x = y; y = temp; It represents the same storage location as the actual parameter. } public static void Main( ) When a formal parameter is declared as ref, the corresponding actual argument in { the method invocation must be declared as int m = 100; ref. int n = 200; Console.WriteLine("Before Swapping;"); Used when we want to change the Console.WriteLine("m = " + m); values of variables in the calling method. Console.WriteLine("n = " + n); Swap (ref m , ref n ); Console.WriteLine("After Swapping;"); Console.WriteLine("m = " + m); Console.WriteLine("n = " + n); 25 } } The Output Parameters Used to pass results back to the calling method. Declare the parameters with an out keyword. It does not create a new storage location. When a formal parameter is declared as out, the corresponding actual argument in the method invocation must also be declared as out. using System; class Output { static void Square ( int x, out int y ) { y = x * x; } public static void Main( ) { int m; //need not be initialized Square ( 10, out m ); Console.WriteLine("m = " + m); } 26 } Variable Argument Lists We can define methods that can using System; handle variable number of class Params arguments using parameter arrays. { static void Parameter arrays are declared using Parray the keyword params (params int [ ] The parameter arrays should be a one- arr) dimensional arrays. { Console.Write("array elements are:"); foreach ( int i in arr) Console.Write(" " + i); Console.WriteLine( ); } public static void Main( ) //call 1 { Parray ( ) ; //call 2 int [ ] x = { 11, 22, 33 }; Parray ( 100, 200 ) ;//call 3 Parray ( x) ; } 27 } Method Overloading Enables us to create more than one Console.WriteLine(add(312L,22L,21)); method with the same name, but } with the different parameter lists & static int add(int a,int b) different definitions. { return(a+b); Required when methods are required } to perform similar tasks but using static float add(float a,float b) different input parameters. { return(a+b); Example } static long add(long a,long b,int c) using System; { class Overloading return(a+b+c); { } public static void Main() } { Console.WriteLine(add(2,3)); Console.WriteLine(add(2.6F,3.1F)); 2 8 Chapter : 3 Handling Arrays Introduction Array is a group of contiguous or related data items that share a common name. A particular value is indicated by writing a number called index number or subscript in brackets after the array name. Example : marks The complete set of values is referred to as an array. The individual values are called elements. 30 One-Dimensional Arrays A list of items can be given one variable name using only one subscript & such a variable is called a one-dimensional array. Declaration of Arrays: Syntax: type[] arrayname; Example: int[] counter; float[] marks; int[] x,y; Creation of Arrays: Syntax: arrayname = new type[size]; Example: counter=new int; marks=new float; Combination: int[] counter=new int; Initialization of Arrays: Syntax: arrayname[subscript]=value; Example: marks=60; marks=70; 31 int[] counter={10,20,30,40,50}; int len=c.Length; //Returns Length of Array Exercise Write a program to sort an array of 5 number taking from user as input. 32 Two-Dimensional Arrays Allows to store table of values. Example: v[4,5]; Each dimension of the array is indexed from zero to its maximum size minus one. First index specifies the row & second index specifies the column within that row. Declaration: int[,] myArray; Creation: myArray=new int[3,4]; Combination: int[,] myArray=new int[3,4]; Initialization: int[,] n={{0,0,0}, 33 {1,1,1}}; Example using System; class MulTable { static int ROWS = 5; static int COLUMNS = 10; public static void Main( ) { int[,] product =new int[ROWS,COLUMNS]; int i,j; for (i=1; i