6 - Lists Tuples Sets (1).pptx
Document Details
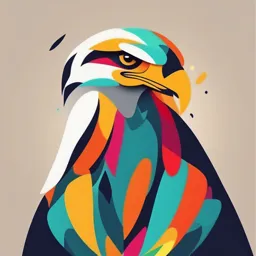
Uploaded by InvulnerableYellow2190
2019
Tags
Full Transcript
Fall 2019 Computational Thinking with @cse_bennett @csebennett Programming Python Collections/Sequences (Arrays) List is a collection which is ordered and changeable. Allows duplicate members. Tuple...
Fall 2019 Computational Thinking with @cse_bennett @csebennett Programming Python Collections/Sequences (Arrays) List is a collection which is ordered and changeable. Allows duplicate members. Tuple is a collection which is ordered and unchangeable. Allows duplicate members. Set is a collection which is unordered and unindexed. No duplicate members. Dictionary is a collection which is unordered, changeable and indexed. No duplicate members. Mutable Data Types: Data types in python where the value assigned to a variable can be changed Immutable Data Types: Data types in python where the value assigned to a variable cannot be changed Python has a great built-in list type named "list". List literals are written within square brackets [ ]. Lists work similarly to strings -- use the len() function and square brackets [ ] to access data, with the first element at index 0. colors = ['red', 'blue', 'green'] Lists print(colors) print(colors) ## red ## green print(len(colors)) ## 3 Elements are indexed from 0 to n-1 , where n is number of elements in the list. Example: [1, 2, 3], ['one', 'two', 'three’], ['apples', 50, True] An empty list is denoted by an empty pair of square brackets, []. Negative indexing, i.e, -1 and -2 refers to the last and second last items. Ex. lst[- 1]=3, lst[-2] = 2. Range Search : lst[0:2] = [1, 2] List Methods Some Common List Methods: list.append(elem) -- adds a single element to the end of the list. Common error: does not return the new list, just modifies the original. list.insert(index, elem) -- inserts the element at the given index, shifting elements to the right. list.extend(list2) adds the elements in list2 to the end of the list. Using + or += on a list is similar to using extend(). list.index(elem) -- searches for the given element from the start of the list and returns its index. Throws a ValueError if the element does not appear (use "in" to check without a ValueError). list.remove(elem) -- searches for the first instance of the given element and removes it (throws ValueError if not present) list.sort() -- sorts the list in place (does not return it). (The sorted() function shown later is preferred.) list.reverse() -- reverses the list in place (does not return it) list.pop(index) -- removes and returns the element at the given index. Returns the rightmost element if index is omitted (roughly the opposite of append()). List Modification Operations in Python Colab Link: https://colab.research.google.com/drive/1oreu8OTv_ROnYTnDUXYSaArGHnxLsa8e?usp= sharing MCQs 1. What would be the range of index values for a list of 10 elements? (a) 0–9 (b) 0–10 (c) 1–10 2. Which one of the following is NOT a common operation on lists? (a) access (b) replace (c) interleave (d) append (e) insert (f ) delete 3. Which of the following would be the resulting list after inserting the value 50 at index 2? (a) (b) (c) MCQs: Answers 1. What would be the range of index values for a list of 10 elements? (a) 0–9 (b) 0–10 (c) 1–10 2. Which one of the following is NOT a common operation on lists? (a) access (b) replace (c) interleave (d) append (e) insert (f ) delete 3. Which of the following would be the resulting list after inserting the value 50 at index 2? (a) (b) (c) Tuples A tuple is an immutable linear data structure. Thus, in contrast to lists, once a tuple is defined, it cannot be altered. Tuples are denoted by parentheses instead of square brackets. Example: nums = (10, 20, 30) student = ('John Smith', 48, 'Computer Science’, 3.42) Tuples of one element must include a comma following the element. Otherwise, the parenthesized element will not be made into a tuple. An empty tuple is represented by a set of empty parentheses, (). Tuple Items Tuple items are ordered, unchangeable, and allow duplicate values. Tuple items are indexed, the first item has index , the second item has index etc. Ordered When we say that tuples are ordered, it means that the items have a defined order, and that order will not change. Unchangeable Tuples are unchangeable, meaning that we cannot change, add or remove items after the tuple has been created. Allow Duplicates Since tuples are indexed, they can have items with the same value. Colab Link: https://colab.research.google.com/drive/16U04OB-sBUHh2B_84YRManw2ruIMBsmK?usp= sharing What will happen? What Will Happen ? Sets Sets are used to store multiple items in a single variable. A set is a collection which is unordered, unchangeable*, and unindexed. * Note: Set items are unchangeable, but you can remove items and add new items. thisset = {"apple", "banana", "cherry"} print(thisset) Set Items Set items are unordered, unchangeable, and do not allow duplicate values. Unordered Unordered means that the items in a set do not have a defined order. Set items can appear in a different order every time you use them, and cannot be referred to by index or key. Unchangeable Set items are unchangeable, meaning that we cannot change the items after the set has been created. Once a set is created, you cannot change its items, but you can remove items and add new items. Duplicates Not Allowed Sets cannot have two items with the same value. Colab Link: https://colab.research.google.com/drive/1_XEHA0sSAhwl8bGDiWgR8yuVigvmBtoh?usp=s haring MCQs 1. Which of the following sequence 4. For lst = [4, 2, 9, 1], what is the result of types is a mutable type? the following operation, lst.insert(2, 3) ? a) strings b) lists c) tuples a) [4, 2, 3, 9, 1] b) [4, 3 ,2, 9, 1] c) [4, 2, 9, 2, 1] 2. Which of the following is true? a) Lists and tuples are denoted by the use of square brackets. 5. Which of the following is the correct way b) Lists are denoted by use of to denote a tuple of one element? square brackets and tuples are a) b) (6) c) [6,] denoted by the use of d) (6,) parentheses. c) Lists are denoted by use of parentheses and tuples are 6. Which of the following set of operations denoted by the use of square can be applied to any sequence? brackets. a) len(s), s[i], s+w (concatenation) 3. Lists and tuples must each contain b) max(s), s[i], sum(s) at least one element. c) len(s), s[i], s.sort() a) True b) False MCQs: Answers 1. Which of the following sequence 4. For lst = [4, 2, 9, 1], what is the result of types is a mutable type? the following operation, lst.insert(2, 3) ? a) strings b) lists c) tuples a) [4, 2, 3, 9, 1] b) [4, 3 ,2, 9, 1] c) [4, 2, 9, 2, 1] 2. Which of the following is true? a) Lists and tuples are denoted by the use of square brackets. 5. Which of the following is the correct way b) Lists are denoted by use of to denote a tuple of one element? square brackets and tuples a) b) (6) c) [6,] are denoted by the use of d) (6,) parentheses. c) Lists are denoted by use of parentheses and tuples are 6. Which of the following set of operations denoted by the use of square can be applied to any sequence? brackets. a) len(s), s[i], s+w (concatenation) 3. Lists and tuples must each contain b) max(s), s[i], sum(s) at least one element. c) len(s), s[i], s.sort() a) True b) False