Write PL/SQL block to display students who have a grade of A. Write PL/SQL block to delete all students who are older than 18 years. Write PL/SQL block to retrieve the Name and Gra... Write PL/SQL block to display students who have a grade of A. Write PL/SQL block to delete all students who are older than 18 years. Write PL/SQL block to retrieve the Name and Grade of all students. Write PL/SQL block to sort Student id in reverse order. Write PL/SQL block to calculate factorial of numbers. Write PL/SQL block to print 9, 18, 27, 36, 45, ..., 90 number series.
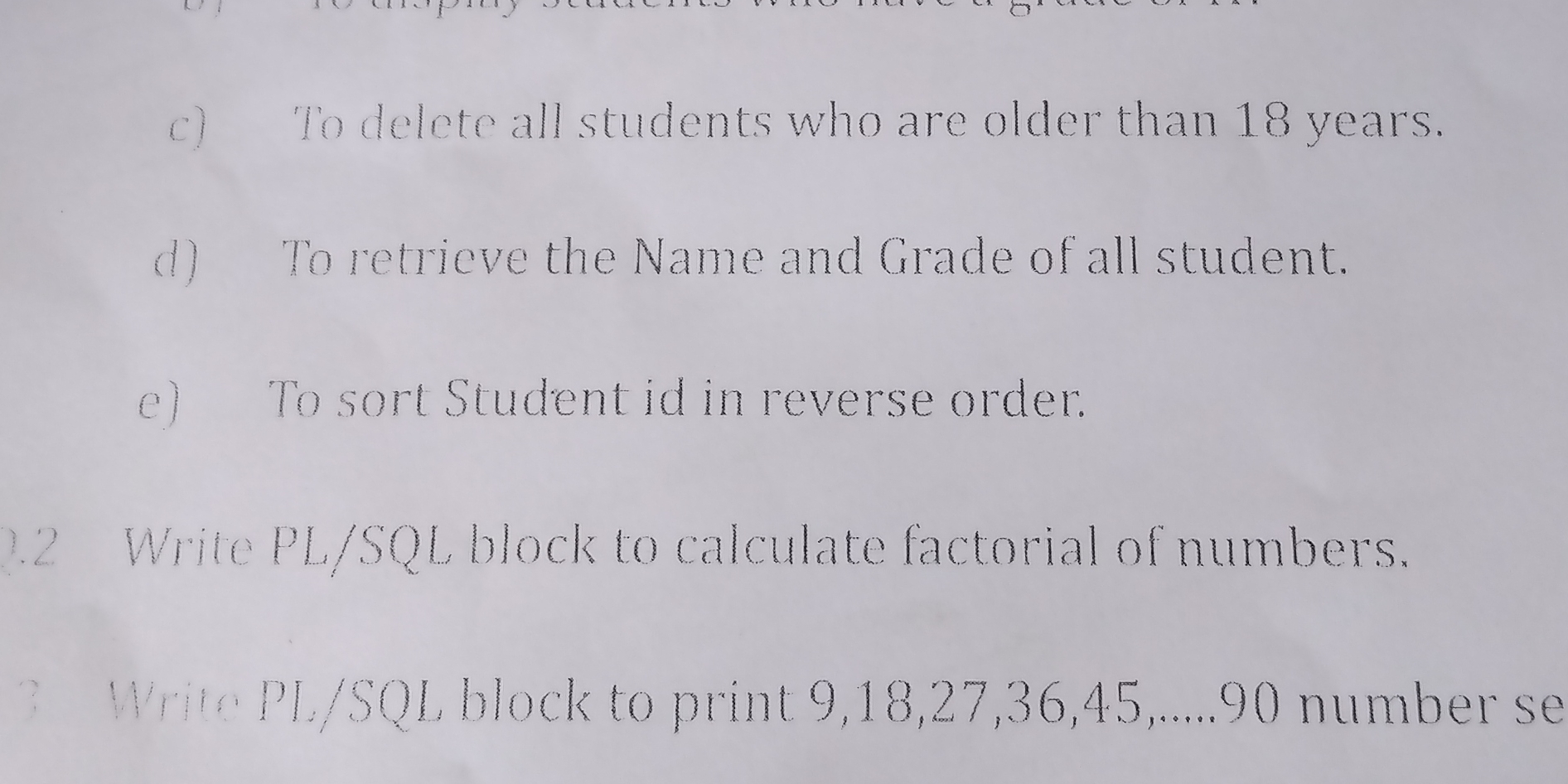
Understand the Problem
The question is asking for PL/SQL blocks to perform various database operations, including deleting students based on age, retrieving specific attributes of students, sorting IDs, and calculating factorials of numbers. Each point outlines a specific task to be accomplished using PL/SQL.
Answer
PL/SQL blocks provided for displaying, deleting, retrieving, sorting, factorials, and series printing as requested.
Here are the PL/SQL blocks for each task:
- Display students with grade 'A':
DECLARE
BEGIN
FOR student IN (SELECT * FROM Students WHERE Grade = 'A')
LOOP
dbms_output.put_line('ID: ' || student.ID || ', Name: ' || student.Name);
END LOOP;
END;
- Delete students older than 18:
BEGIN
DELETE FROM Students WHERE Age > 18;
COMMIT;
END;
- Retrieve Name and Grade of all students:
DECLARE
BEGIN
FOR student IN (SELECT Name, Grade FROM Students)
LOOP
dbms_output.put_line('Name: ' || student.Name || ', Grade: ' || student.Grade);
END LOOP;
END;
- Sort Student ID in reverse order:
DECLARE
BEGIN
FOR student IN (SELECT * FROM Students ORDER BY ID DESC)
LOOP
dbms_output.put_line('ID: ' || student.ID || ', Name: ' || student.Name);
END LOOP;
END;
- Calculate factorial of numbers:
DECLARE
n NUMBER := 5; --for example
fact NUMBER := 1;
BEGIN
FOR i IN 1..n LOOP
fact := fact * i;
END LOOP;
dbms_output.put_line('Factorial of ' || n || ' is ' || fact);
END;
- Print 9, 18, 27, ..., 90 series:
DECLARE
i NUMBER;
BEGIN
i := 9;
WHILE i <= 90 LOOP
dbms_output.put_line(i);
i := i + 9;
END LOOP;
END;
Answer for screen readers
Here are the PL/SQL blocks for each task:
- Display students with grade 'A':
DECLARE
BEGIN
FOR student IN (SELECT * FROM Students WHERE Grade = 'A')
LOOP
dbms_output.put_line('ID: ' || student.ID || ', Name: ' || student.Name);
END LOOP;
END;
- Delete students older than 18:
BEGIN
DELETE FROM Students WHERE Age > 18;
COMMIT;
END;
- Retrieve Name and Grade of all students:
DECLARE
BEGIN
FOR student IN (SELECT Name, Grade FROM Students)
LOOP
dbms_output.put_line('Name: ' || student.Name || ', Grade: ' || student.Grade);
END LOOP;
END;
- Sort Student ID in reverse order:
DECLARE
BEGIN
FOR student IN (SELECT * FROM Students ORDER BY ID DESC)
LOOP
dbms_output.put_line('ID: ' || student.ID || ', Name: ' || student.Name);
END LOOP;
END;
- Calculate factorial of numbers:
DECLARE
n NUMBER := 5; --for example
fact NUMBER := 1;
BEGIN
FOR i IN 1..n LOOP
fact := fact * i;
END LOOP;
dbms_output.put_line('Factorial of ' || n || ' is ' || fact);
END;
- Print 9, 18, 27, ..., 90 series:
DECLARE
i NUMBER;
BEGIN
i := 9;
WHILE i <= 90 LOOP
dbms_output.put_line(i);
i := i + 9;
END LOOP;
END;
More Information
These PL/SQL blocks handle specific operations related to student data and mathematical calculations, demonstrating key programming constructs like loops and conditionals.
Tips
Ensure correct use of loops and conditions. Order by DESC for reverse sorting.
AI-generated content may contain errors. Please verify critical information