Write a program to input marital status and gender and print the insurance amount as per the given table.
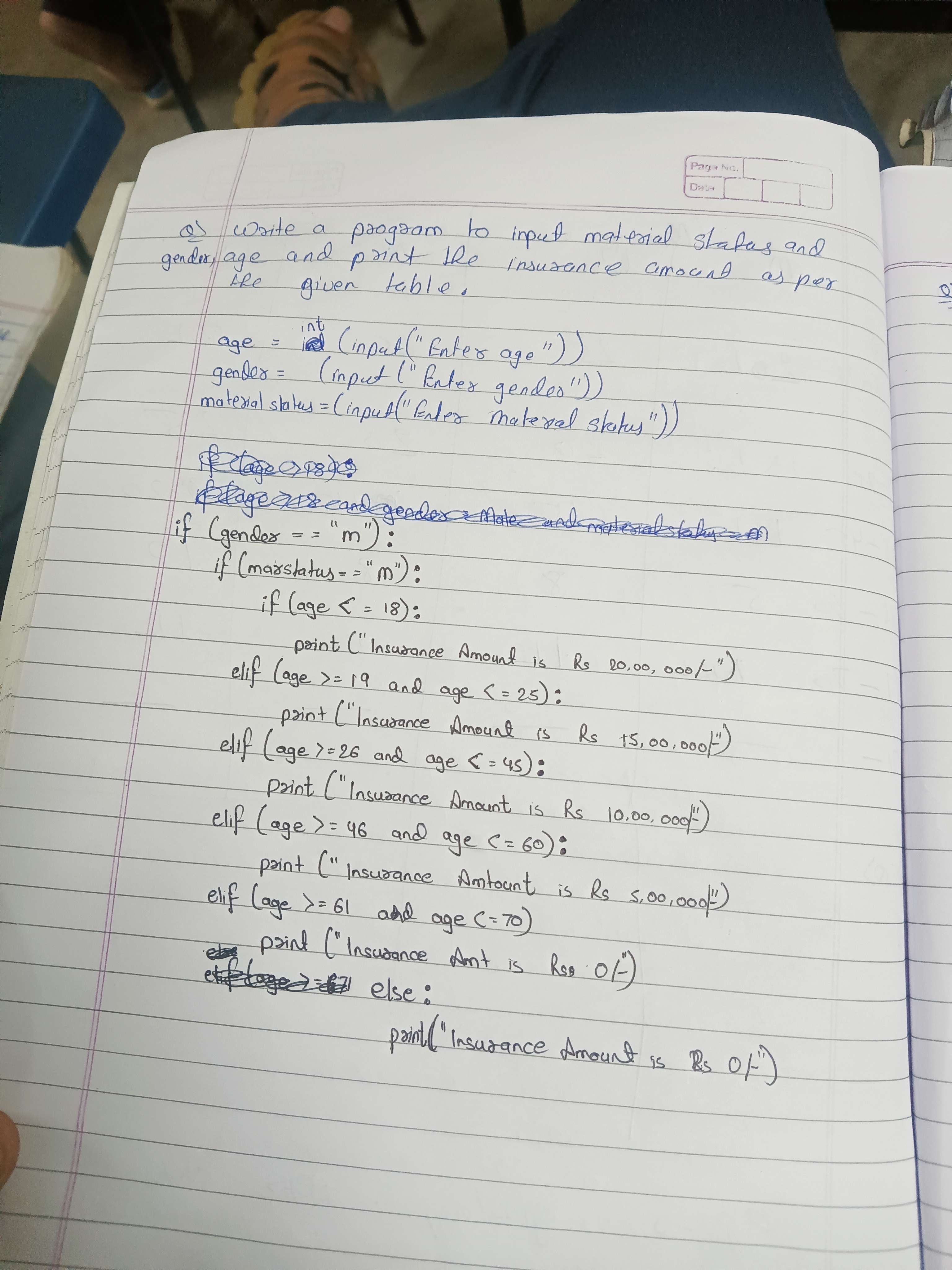
Understand the Problem
The question is asking to write a program that takes input for marital status, age, and gender, and then determines and prints the insurance amount based on these inputs according to a given table.
Answer
The program calculates the insurance amount based on input criteria using conditional statements.
Answer for screen readers
The program calculates insurance amounts based on age, gender, and marital status by using input checks with conditional statements.
Steps to Solve
- Input Collection Collect the required input values from the user.
- Use
input()
function to gather theage
,gender
, andmarital_status
.
age = int(input("Enter age: "))
gender = input("Enter gender (m/f): ")
marital_status = input("Enter marital status (m/u): ")
- Determine Insurance Amount based on Conditions Create a series of conditional statements to determine the insurance amount based on the provided input.
- Use
if
,elif
, andelse
statements to check conditions based ongender
,marital_status
, andage
.
- Example Conditional Structure Here's an example structure of the conditions, checking gender first, then marital status, and finally age:
if gender == 'm':
if marital_status == 'm': # married
if age < 18:
print("Insurance Amount is Rs 20,00,000/-")
elif age >= 19 and age <= 25:
print("Insurance Amount is Rs 15,00,000/-")
elif age >= 26 and age <= 45:
print("Insurance Amount is Rs 10,00,000/-")
elif age >= 46 and age <= 60:
print("Insurance Amount is Rs 5,00,000/-")
elif age > 61 and age < 70:
print("Insurance Amount is Rs 3,00,000/-")
else:
print("Insurance Amount is Rs 0/-")
else: # unmarried
print("Insurance Amount is Rs 0/-")
- Finalizing the Program Ensure that all paths in your conditions are covered, and provide appropriate outputs for each case. This makes sure that your program runs smoothly and gives the right outputs based on the inputs.
The program calculates insurance amounts based on age, gender, and marital status by using input checks with conditional statements.
More Information
This program utilizes conditional logic to assess multiple criteria that lead to specific outputs. It highlights logical thinking in programming, especially when handling user inputs and conditions.
Tips
- Forgetting to convert input types properly, especially when reading age as an integer.
- Not properly nesting conditions, which can lead to incorrect outputs.
- Ignoring edge cases for ages or mistyping variables.
AI-generated content may contain errors. Please verify critical information