Write a C program to perform multiplication on a 3D array.
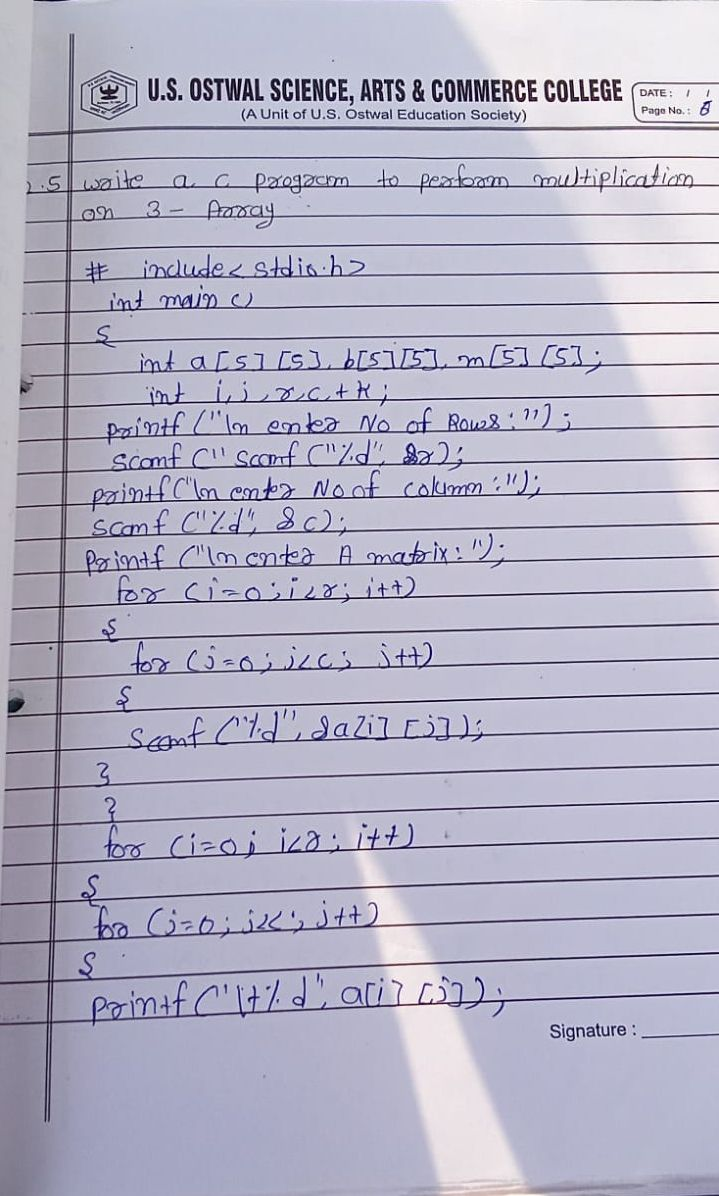
Understand the Problem
The question is asking to write a C program that performs multiplication on a 3D array. It outlines necessary components such as input for the number of rows and columns, and the structure for implementing the multiplication operation in code.
Answer
Complete C program example for 3D array multiplication.
Here is a complete C program to perform multiplication on a 3D array: #include <stdio.h> int main() { int a[5][5][5], b[5][5][5], m[5][5][5]; int i, j, k, r, c, d; printf("Enter No of Rows (up to 5):"); scanf("%d", &r); printf("Enter No of Columns (up to 5):"); scanf("%d", &c); printf("Enter Depth (up to 5):"); scanf("%d", &d); printf("Enter A matrix:"); for (i = 0; i < r; i++) { for (j = 0; j < c; j++) { for (k = 0; k < d; k++) { scanf("%d", &a[i][j][k]); } } } printf("Enter B matrix:"); for (i = 0; i < r; i++) { for (j = 0; j < c; j++) { for (k = 0; k < d; k++) { scanf("%d", &b[i][j][k]); } } } for (i = 0; i < r; i++) { for (j = 0; j < c; j++) { for (k = 0; k < d; k++) { m[i][j][k] = a[i][j][k] * b[i][j][k]; } } } printf("Resultant matrix m after multiplication:\n"); for (i = 0; i < r; i++) { for (j = 0; j < c; j++) { for (k = 0; k < d; k++) { printf("%d ", m[i][j][k]); } printf("\n"); } printf("\n"); } return 0; }
Answer for screen readers
Here is a complete C program to perform multiplication on a 3D array: #include <stdio.h> int main() { int a[5][5][5], b[5][5][5], m[5][5][5]; int i, j, k, r, c, d; printf("Enter No of Rows (up to 5):"); scanf("%d", &r); printf("Enter No of Columns (up to 5):"); scanf("%d", &c); printf("Enter Depth (up to 5):"); scanf("%d", &d); printf("Enter A matrix:"); for (i = 0; i < r; i++) { for (j = 0; j < c; j++) { for (k = 0; k < d; k++) { scanf("%d", &a[i][j][k]); } } } printf("Enter B matrix:"); for (i = 0; i < r; i++) { for (j = 0; j < c; j++) { for (k = 0; k < d; k++) { scanf("%d", &b[i][j][k]); } } } for (i = 0; i < r; i++) { for (j = 0; j < c; j++) { for (k = 0; k < d; k++) { m[i][j][k] = a[i][j][k] * b[i][j][k]; } } } printf("Resultant matrix m after multiplication:\n"); for (i = 0; i < r; i++) { for (j = 0; j < c; j++) { for (k = 0; k < d; k++) { printf("%d ", m[i][j][k]); } printf("\n"); } printf("\n"); } return 0; }
More Information
This program multiplies two 3D arrays element-wise and stores the result in a third 3D array.
Tips
Ensure that the dimensions of the arrays are compatible for element-wise multiplication.
Sources
- C Program to Multiply Two Matrices Using Multi-dimensional Arrays - programiz.com
- Multidimensional Arrays in C - 2D and 3D Arrays - GeeksforGeeks - geeksforgeeks.org
AI-generated content may contain errors. Please verify critical information