What will be the output of the following program: class Test { public static void main(String[] args) { int x = 20; String sup = (x < 15) ? "small" : (x < 22) ? "tiny" : "huge"; Sy... What will be the output of the following program: class Test { public static void main(String[] args) { int x = 20; String sup = (x < 15) ? "small" : (x < 22) ? "tiny" : "huge"; System.out.println(sup); } } Options: A. small B. tiny C. huge D. Compilation fails
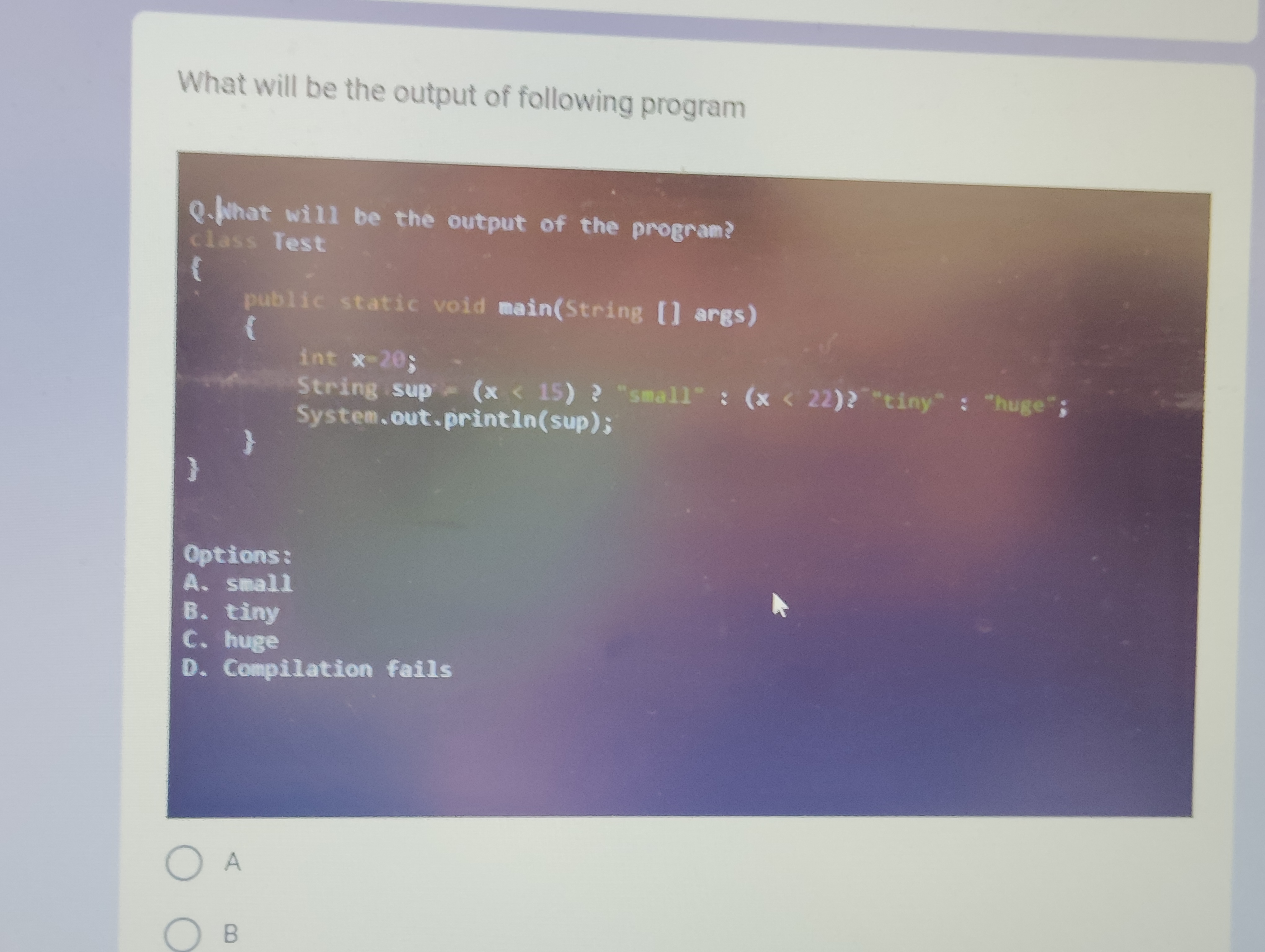
Understand the Problem
The question is asking for the output of a Java program that uses a conditional (ternary) operator to determine the value of a string based on the value of an integer. We need to analyze the code to evaluate which string the program will output when executed.
Answer
The output will be "tiny".
Answer for screen readers
The output of the program will be "tiny".
Steps to Solve
-
Identify the integer value
The code initializes an integer variable:
int x = 20;
-
Analyze the conditional operation
The ternary operation structure is:
String sup = (x < 15) ? "small" : (x < 22) ? "tiny" : "huge";
Here, we check the conditions starting from the left. The first condition checks if $x < 15$.
-
Evaluate the first condition
Since $x = 20$, we evaluate:
$$ x < 15 \text{ evaluates to false.} $$ -
Evaluate the second condition
Next, since the first condition is false, we move to the next condition which checks if $x < 22$:
$$ x < 22 \text{ evaluates to true.} $$ -
Set the string value based on the conditions
Since the second condition is true, the string value becomes "tiny". Thus:
sup = "tiny";
-
Output the result
The program will output the value ofsup
:
System.out.println(sup);
The output of the program will be "tiny".
More Information
In this Java code, the ternary operator is used to set a string based on the evaluation of conditions involving an integer variable. The evaluated conditions dictate the string assigned to the sup
variable.
Tips
- Misunderstanding the order of evaluation in nested ternary operations can lead to incorrect values being assigned.
- Failing to correctly evaluate Boolean expressions could also lead to confusion. Always check each condition sequentially.