What will be the output of the following C code?
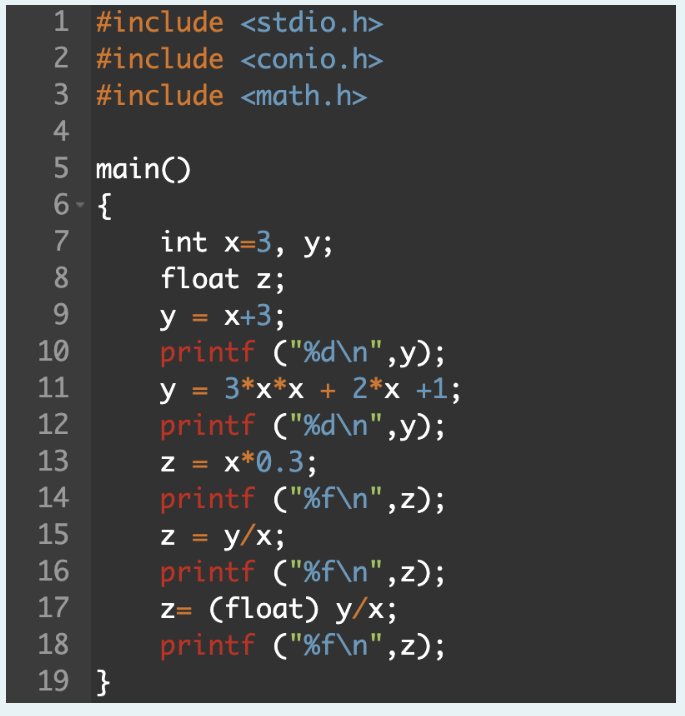
Understand the Problem
The question is asking us to analyze the given C code, particularly focusing on the values of the variables used and printed at different stages of execution. We need to trace through the code step by step to find out the output produced by the printf statements.
Answer
``` 6 34 0.900000 11.333333 11.333333 ```
Answer for screen readers
The output of the program will be:
6
34
0.900000
11.333333
11.333333
Steps to Solve
-
Initialize the variables
The code starts by initializing the variables:
-
int x = 3;
-
int y;
(uninitialized at this point) -
float z;
(also uninitialized)
-
-
Assign the value to y
The next line executes
y = x + 3;
, which assigns the value ofy
:- Since
x
is 3, $$ y = 3 + 3 = 6 $$
- Since
-
Print the value of y
Now the first
printf
executes:printf("%d\n", y);
- This will print
6
.
- This will print
-
Update the value of y
Next,
y
is updated: $$ y = 3xx + 2*x + 1 $$- Substituting
x
(3): $$ y = 333 + 2*3 + 1 = 27 + 6 + 1 = 34 $$
- Substituting
-
Print the updated value of y
The second
printf
executes:printf("%d\n", y);
- This will print
34
.
- This will print
-
Calculate z with a multiplication
Next, we compute
z
: $$ z = x * 0.3 $$- Substituting
x
(3): $$ z = 3 * 0.3 = 0.9 $$
- Substituting
-
Print the value of z
The third
printf
executes:printf("%f\n", z);
- This will print
0.900000
.
- This will print
-
Update z based on y and x
The code updates
z
: $$ z = y / x $$- With
y
=34 andx
=3: $$ z = 34 / 3 \approx 11.3333 $$
- With
-
Print the new value of z
The fourth
printf
is executed:printf("%f\n", z);
- This will print approximately
11.333333
.
- This will print approximately
-
Cast y to float and update z
Finally,
z
is updated to: $$ z = (float) y / x $$- Calculating again using
y
=34 andx
=3: $$ z = \frac{34}{3} \approx 11.3333 $$
- Calculating again using
-
Print the casted new value of z
The last
printf
executes:printf("%f\n", z);
- This will again print approximately
11.333333
.
- This will again print approximately
The output of the program will be:
6
34
0.900000
11.333333
11.333333
More Information
This program demonstrates variable initialization, arithmetic operations, and type casting in C. It serves as an example of both integer and floating-point arithmetic.
Tips
- Forgetting to initialize variables: Uninitialized variables can lead to unpredictable results.
-
Confusion between
%d
and%f
: Using the wrong format specifier for data types can cause incorrect output or runtime errors.
AI-generated content may contain errors. Please verify critical information