Given the variables int x and int y declare and initialize a string named values that is of the format x hyphen y. Example: '22-13' (Without spaces)
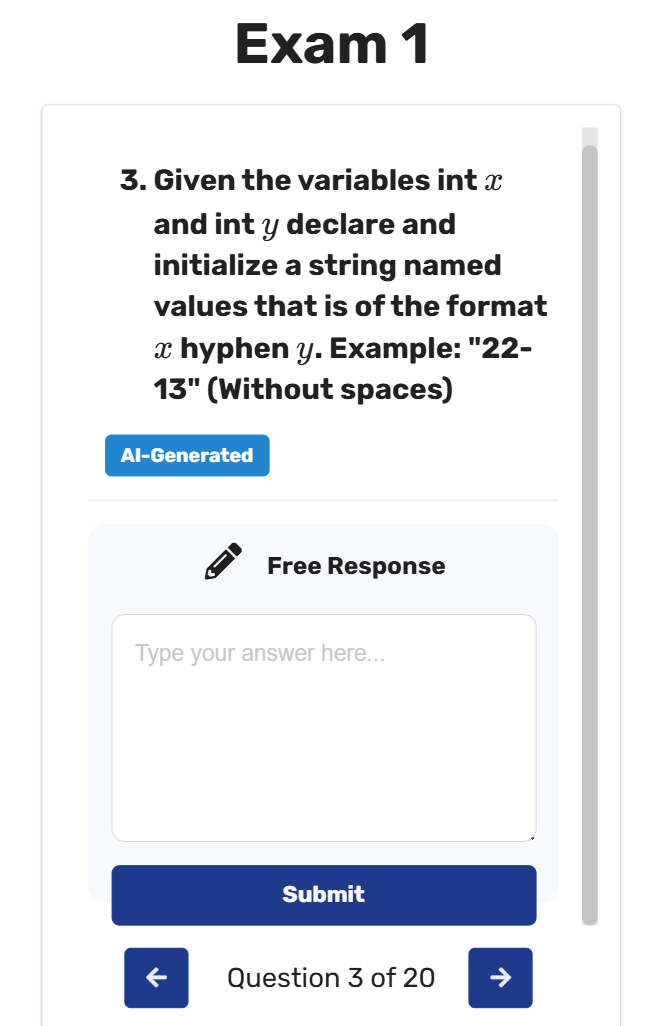
Understand the Problem
The question is asking to declare and initialize a string variable named 'values' that combines two integer variables, x and y, into a specific format using a hyphen without spaces. This involves basic string manipulation and declaration in a programming context.
Answer
The string declaration and initialization can be summarized as: ```java String values = String.valueOf(x) + "-" + String.valueOf(y); ```
Answer for screen readers
The code to declare and initialize the string variable values
is:
int x = 22; // Example value
int y = 13; // Example value
String values = String.valueOf(x) + "-" + String.valueOf(y);
Steps to Solve
-
Declare the integer variables First, ensure that you have declared the integer variables
x
andy
and assigned them values. For example:int x = 22; int y = 13;
-
Convert integers to strings You need to convert the integer values of
x
andy
into strings. This can generally be done using theString.valueOf()
method in Java:String strX = String.valueOf(x); String strY = String.valueOf(y);
-
Concatenate the strings with a hyphen Use the
+
operator to concatenate the string representations ofx
andy
, including a hyphen between them:String values = strX + "-" + strY;
-
Finalize the string At this point,
values
will hold the desired string format. For example, it will contain "22-13" if x is 22 and y is 13:System.out.println(values); // Outputs: 22-13
The code to declare and initialize the string variable values
is:
int x = 22; // Example value
int y = 13; // Example value
String values = String.valueOf(x) + "-" + String.valueOf(y);
More Information
In this example, if x
is 22 and y
is 13, the value of the string values
will be "22-13". This demonstrates basic string concatenation in Java.
Tips
- Forgetting to convert integers to strings before concatenation can lead to errors. Always ensure you convert using methods like
String.valueOf()
. - Missing the hyphen in the concatenation step will result in an incorrect format.
AI-generated content may contain errors. Please verify critical information