Introduction to 8085 Assembly Language Programming PDF
Document Details
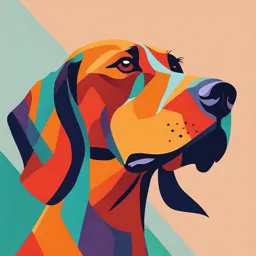
Uploaded by ConsummateBlueLaceAgate4112
Tags
Related
- Assembly Language Programming ACSL PDF
- Lecture 2 Assembly Language Programming 2024-2025 PDF
- CSC 204 - Assembly Language - Chapter 04 - Fall 2024-2025 PDF
- CPE 409 Assembly Language Programming PDF
- Chapter 5 - Basic Concepts of Assembly Language PDF
- Microprocessor Architecture, Programming, and Applications with the 8085 PDF
Summary
This textbook chapter introduces 8085 assembly language programming. It covers the 8085 microprocessor, including its hardware and programming model, instruction classification, and data formats. The chapter also explains how to write, assemble, and execute a simple program, including examples and programming concepts.
Full Transcript
# Introduction to 8085 Assembly Language Programming ## Chapter 2 - The 8085 Microprocessor ### Objectives - Explain the various functions of the registers in the 8085 programming model. - Define the term _flag_ and explain how the flags are affected. - Explain the terms _operation code_ (opcode)...
# Introduction to 8085 Assembly Language Programming ## Chapter 2 - The 8085 Microprocessor ### Objectives - Explain the various functions of the registers in the 8085 programming model. - Define the term _flag_ and explain how the flags are affected. - Explain the terms _operation code_ (opcode) and _operand_ and illustrate these terms by writing instructions. - Classify the instructions in terms of their word size and specify the number of memory registers required to store the instructions in memory. - List the five categories of the 8085 instruction set. - Define and explain the term _addressing mode_. - Write logical steps to solve a simple programming problem. - Draw a flowchart from the logical steps of a given programming problem. - Translate the flowchart into mnemonics and convert the mnemonics into Hex code for a given programming problem. ## The 8085 Programming Model ### 2.1.1 - 8085 Hardware Model - The hardware model has two major segments: an arithmetic/logic unit (ALU) and a set of registers. - The ALU performs arithmetic and logical operations and stores the result in the accumulator. - Flags are set or reset to reflect the results of ALU operations. - There are three buses: - a 16-bit unidirectional address bus, - an 8-bit bidirectional data bus, - a control bus. ### 2.1.2 - 8085 Programming Model - The programming model consists of segments of the ALU and the registers. - The model includes six registers, one accumulator, and one flag register, and two 16-bit registers: the stack pointer and the program counter. #### Registers: - Six general-purpose registers (B, C, D, E, H, and L) to store 8-bit data. - Can be combined into register pairs (BC, DE, and HL) to perform 16-bit operations. #### Accumulator: - An 8-bit register that is part of the ALU and performs arithmetic and logical operations. - Stores the result of an operation and is also identified as register A. #### Flags: - Five flip-flops that are set or reset after an operation according to the results in the accumulator and other registers. - These flags are Zero (Z), Carry (CY), Sign (S), Parity (P), and Auxiliary Carry (AC) flags. ## 2.2 - Instruction Classification - An instruction is a binary pattern designed inside a microprocessor to perform a specific function. - The 8085 instruction set determines what functions the microprocessor can perform. - Instructions are classified into five functional categories: data transfer (copy), arithmetic, logical, branching, and machine-control. ### 2.2.1 - The 8085 Instruction Set #### Data Transfer (Copy) Operations - Copies data from a source to a destination without modifying the contents of the source. - Types: - Between registers - Specific data byte to a register or a memory location - Between a memory location and a register - Between an I/O device and the accumulator #### Arithmetic Operations - Perform arithmetic operations such as addition, subtraction, increment, and decrement. - Types: - Addition - Subtraction - Increment/Decrement #### Logical Operations - Perform various logical operations with the contents of the accumulator. - Types: - AND, OR, Exclusive-OR - Rotate - Compare - Complement #### Branching Operations - Alter the sequence of program execution either conditionally or unconditionally. - Types: - Jump - Call, Return, and Restart #### Machine Control Operations - Control machine functions such as Halt, Interrupt, or do nothing. ### 2.2.2 - Review of the 8085 Operations - Data manipulation functions are summarized in four functions: - Copying data - Performing arithmetic operations - Performing logical operations - Testing for a given condition and altering the program sequence ## 2.3 - Instruction, Data Format, and Storage - An instruction is a command to the microprocessor to perform a given task on specified data. - Each instruction has two parts: - opcode: the task to be performed - operand: the data to be operated on (8-bit or 16-bit data, an internal register, a memory location, or an 8-bit or 16-bit address). ### 2.3.1 - Instruction Word Size - Classified according to word size (or byte size) - 1-byte instructions - 2-byte instructions - 3-byte instructions ### 2.3.2 - Opcode Format - Operation codes are identified with specific codes: - Registers - Register pairs ### 2.3.3 - Data Format - The 8085 processes binary numbers, but the real world operates in decimal numbers and languages of alphabets and characters. - Data formats: ASCII, BCD, signed integers, and unsigned integers ### 2.3.4 - Instruction and Data Storage: Memory - Memory is a storage of binary bits, with each register storing 8 bits. - Memory has: two address lines, eight data lines, and three timing or control signals (Read, Write, and Chip Select). ## 2.4 How to Write, Assemble, and Execute a Simple Program - A program is a sequence of instructions written to tell a computer to perform a specific function. - To write a program, divide a given problem into small steps in terms of the operations the 8085 can perform, then translate these steps into instructions. - Flowchart: a block diagram representing the steps in a program sequence. - Assembly Language Program: the program translated into mnemonics, converted into Hex code, and then to binary code and stored in memory. ### 2.4.1 - Illustrative Program: Adding Two Hexadecimal Numbers - Steps: - Load the numbers into the registers - Add the numbers - Display the sum at the output port ### 2.4.2 - How Does a Microprocessor Differentiate Between Data and Instruction Code? - The microprocessor is a sequential machine that executes instructions from memory, one code after another, at the speed of its clock. - The first byte fetched is interpreted as an opcode. - If there is an error in entering the code (for example, if a data byte is skipped), the microprocessor will interpret the next code as an opcode, and the program will execute in sequence. ## 2.5 - Overview of the 8085 Instruction Set - The 8085 microprocessor instruction set has 74 operation codes that result in 246 instructions. - The set includes all the 8080A instructions plus two additional instructions (SIM and RIM, related to serial I/O). ## 2.6 - Writing and Hand Assembling a Program - In previous sections, we discussed the 8085 instructions, the number of bytes per instruction, the relationship between the number of bytes of an instruction and memory registers, and the processor's computing capability, and we pulled together all these concepts in a simple illustrative program. ### 2.6.1 - Illustrative Program: Subtracting Two Hexadecimal Numbers and Storing the Result in Memory - Steps: - Copy two data bytes into processor registers - Subtract B from A - Save the result in memory - Terminate the program ### 2.6.2 - How Does a Microprocessor Differentiate Between Data and Instruction Code? - The microprocessor is a sequential machine. As soon as a microprocessor-based system is turned on, it begins the execution of the code in memory. The execution continues in sequence, one code after another (one memory location after another) at the speed of its clock until the system is turned off (or the clock stops). If an unconditional loop is set up in a program, the execution will continue until the system is either reset or turned off. Now a puzzling question is: How does the microprocessor differentiate between a code and data when both are binary numbers? The answer lies in the fact that the microprocessor interprets the first byte it fetches as an opcode. When the 8085 is reset, its program counter is cleared to 0000H, and it fetches the first code from the location 0000H. In the example of the previous section, we tell the processor that our program begins at location 2000H. The first code it fetches is 3EH. When it decodes that code, it knows that it is a two-byte instruction. Therefore, it assumes that the second code, 32H, is a data byte. If we forget to enter 32H and enter the next code, 06H, instead, the 8085 will load 06H in the accumulator, interpret the next code, 48H, as an opcode, and continue the execution in sequence. As a consequence, we may encounter a totally unexpected result. ## 2.7 - Common Errors - Similar errors happen in writing assembly language instructions as in learning a new language (misspelling, mispronouncing, not using the correct syntax, etc.) ## 2.8 - Summary - The 8085 microprocessor operations are classified into five major groups: data transfer, arithmetic, logic, branch, and machine control. - An instruction has two parts: opcode and operand. - The instruction set is classified in three groups according to the word size: 1-, 2-, or 3-byte instructions. - To write an assembly language program, divide the given problem into small steps in terms of the microprocessor operations, translate these steps into assembly language instructions, and then translate them into the 8085 machine code. ## 2.9 - Questions and Programming Assignments - A number of questions and assignments are given to test the reader’s understanding of the material presented in this chapter. This document has been converted from a scanned image using Optical Character Recognition (OCR) and may contain errors as there are some images that OCR cannot recognize.