Microprocessor Architecture, Programming, and Applications with the 8085 PDF
Document Details
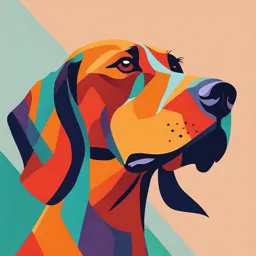
Uploaded by ConsummateBlueLaceAgate4112
Ramesh Gaonkar
Tags
Summary
This textbook covers microprocessor architecture, programming, and applications with the 8085. It details microprocessors, microcomputers, and assembly language, including concepts like binary operations, machine language, and mnemonics.
Full Transcript
# Microprocessor-Based Systems: Hardware and Interfacing ## Chapter 1: Microprocessors, Microcomputers, and Assembly Language ### Introduction - The microprocessor controls processes and turns devices on/off, as a programmable logic device. - It is often used as a computing unit for a computer. -...
# Microprocessor-Based Systems: Hardware and Interfacing ## Chapter 1: Microprocessors, Microcomputers, and Assembly Language ### Introduction - The microprocessor controls processes and turns devices on/off, as a programmable logic device. - It is often used as a computing unit for a computer. - The microprocessor is a programmable integrated device with decision-making capability that is similar to a Computer's central processing unit (CPU) - It is used in a wide range of products called microprocessor-based products or systems. - When a microprocessor is embedded in a larger system, it can function as a standalone unit controlling processes, or a CPU in a computer called a microcomputer. - The chapter concludes a block diagram of a temperature control system as an application of the microprocessor-based system. ### The Microprocessor Operates in Binary - Binary numbers are represented as bits, 0 and 1. - Each microprocessor has a fixed set of instructions called machine language. - Machine language is difficult for humans to communicate in, therefore they abbreviate the instructions calling them mnemonics. ### Objectives - Draw a block diagram of a microprocessor-based system and explain functions of each component: microprocessor, memory, and I/O. - Explain the terms SSI, MSI, and LSI. - Define the terms bit, byte, word, instruction, software, and hardware. - Explain the difference between machine language and the assembly language of a computer. - Explain the terms low-level and high-level languages. - Explain the advantages of assembly language over high-level languages. - Define the term ASCII code, and explain the relationship between the binary code and alphanumeric characters. - Define the term operating system. - List the components of a typical personal computer. - Draw a block diagram of a microprocessor-controlled temperature system and identify the function of each component. ### 1.1 Microprocessors - Microprocessors are multipurpose, programmable, clock-driven, register-based electronic devices. - The device reads instructions from memory, accepts data, processes the data, and returns results. - At an elementary level, the device can be compared to the human brain which processes information according to an instruction stored in memory. - The microprocessor is much less complex than the human brain. - A typical programmable machine consists of four components: microprocessor, memory, input, and output. ### 1.1.1 Organization of a Microprocessor-Based System - A microprocessor-based system consists of: - Microprocessor - I/O (input/output) - Memory (read/write memory and read-only memory) - All of these components are organized around a common communication path called a bus. - The microprocessor is a clock-driven semiconductor device consisting of electronic logic circuits. - It is capable of various computing functions and making decisions. - The microprocessor is similar to the CPU, but includes all the logic circuitry on one chip. - The microprocessor can be divided into three segments: - Arithmetic/logic unit (ALU) - Register array - Control unit ### 1.1.2 Memory - Memory stores binary information as instructions and data. - The processor reads instructions and data from memory and performs operations in the ALU. - Results can be transferred to the output section for display, or stored in memory for later use. - The two types of memory are: - Read-only memory (ROM), and - Read/write memory (R/WM), also known as RAM - The ROM is used for storage of programs that do not need alteration. - The R/WM is used to store user programs and data, and the information can be easily read and altered. ### 1.1.3 Input/Output - I/O communicates with the outside world. - The two types of devices are: - Input devices (inputting data and instructions), such as a keyboard, and - Output devices (displaying data), such as a video screen. - The system bus is a communication path between the microprocessor and peripherals. - The processor communicates with only one peripheral at a time, and the timing is provided by the control unit. ### 1.1.4 How Does the Microprocessor Work? - The processor reads and executes one instruction at a time that is already stored in the R/W memory. - The process can be compared to assembling a radio kit, where each instruction is followed in sequence to complete the task. ### 1.1.5 Summary of Important Concepts - The microprocessor: - Reads instructions from memory. - Communicates with all peripherals (memory and I/O) using the system bus. - Controls the timing of information flow. - Performs the computing tasks specified in a program. - The memory: - Stores binary information called instructions and data. - Provides instructions and data to the microprocessor on request. - Stores results and data for the microprocessor. - The input device: - Enters data and instructions under the control of a program. - The output device: - Accepts data from the microprocessor as specified in a program. - The bus: - Carries bits between the microprocessor and memory and I/O. ## 1.2 Microprocessor Instruction Set and Computer Languages ### 1.2.1 Machine Language - The number of bits in a computer's word is fixed, and words are formed through various combinations of bits. - The microprocessor design engineer selects combinations of bit patterns and gives a meaning to each combination. - Each combination is called an instruction. - Instructions are made up of one word or several words. - The set of instructions designed into the machine makes up its machine language - a binary language composed of 0s and 1s. - The microprocessor determines a computer's machine language because it executes the operations of a microprocessor-based system. ### 1.2.2 8085 Machine Language - The 8085 is a microprocessor with an 8-bit word length. - Instruction set is designed by using various combinations of the eight bits. - The 8085 is an improved version of the 8080A processor. - An instruction is a binary pattern entered through an input device that commands the microprocessor to perform a specific function. - The 8085 has 246 bit patterns which amounts to 74 different instructions for performing various operations. - These 74 different instructions are called its instruction set, and this binary language with a predetermined instruction set is called the 8085 machine language. ### 1.2.3 8085 Assembly Language - While instructions can be written in hexadecimal code, it is difficult to understand programs in this form. - Manufacturers have devised symbolic codes for each instruction, called a mnemonic, which means mindful, or a memory aid. - The mnemonic for a particular instruction consists of letters that suggest the operation. - The 8085 microprocessor uses mnemonics to represent bit patterns. For example, the mnemonic *INR A* represents the binary code 0011 1100, and similar mnemonics suggest additional actions, such as addition, subtraction, and more. - The complete set of 8085 mnemonics is called the 8085 assembly language, and programs written in these mnemonics are called assembly language programs. ### 1.2.4 ASCII Code - ASCII (American Standard Code for Information Interchange) is a 7-bit code with 128 combinations used to communicate with a computer in alphabetic letters and decimal numbers. - The code is assigned to letters, decimal numbers, symbols, and machine commands. - The code is used in microcomputer systems, keyboards, video screens, and printers. - Extended ASCII is an 8-bit code with 256 combinations and is used by recent computers. ### 1.2.5: Writing and Executing an Assembly Language Program - To manually write and execute an assembly language program on a single-board computer with a Hex keyboard for input and LEDs for output, the steps are: - Write instructions in mnemonics obtained from the instruction set. - Find the hexadecimal machine code for each instruction by searching through the instruction set. - Enter the program into user memory in sequential order. - Execute the program using the Hex keyboard as input and the result is displayed by LEDs as output. ### 1.2.6 High-Level Languages - Machine-independent programming languages are called **high-level languages**. - High-level languages have their own sets of rules and use symbols and conventions from English. - Examples of high-level languages are: - BASIC - PASCAL - C - C++ - Java - Programs written in high-level languages can be run on different microprocessors without needing to be re-written. ### 1.2.7 Operating Systems - Operating systems oversee all operations of the computer. - They transfer information, store programs on disk, communicate with peripherals, and manage the interaction between the computer and its peripherals. - The functional relationship between the operating system and the hardware is shown in a block diagram. - The operating system is closest to the hardware and application programs are farthest. - When a computer turns on, the operating system is in charge of the system. - Windows is a graphical user interface (GUI) operating system. ## Chapter 1.3: From Large Computers to Single-Chip Microcontrollers - The range of computers available extends from million-dollar mainframe Cray computers to $1000 personal computers. - Regardless of their purpose, these computers all include the basic components shown in Figure 1.3. - There are three categories of computers: - **Large computers:** These are multi-user, multitasking computers designed for complex, scientific and engineering calculations, and handling records for large corporations and government agencies. The range of these computers includes mainframes and supercomputers. - **Medium-size computers:** These computers were designed to meet the needs of small colleges, factories, and medium-size businesses. - **Microcomputers:** These computers are single users and are designed for personal use. These computers are categorized by their size and portability. ### 1.3.1 Large Computers - Large Computers include: - Mainframes: These computers have a word length of 32 to 64 bits, can address megabytes of memory, and handle various peripherals and users. Examples of mainframes are the IBM System/390 series and Fujitsu GS8800. - Supercomputers: These are high-performance, high-speed computers capable of executing billions of instructions per second, such as the Cray-2 and Y-MP. These are used for research. ### 1.3.2 Medium-Size Computers - Medium-size computers are designed to meet the needs of small colleges, factories, and businesses, and are called **minicomputers**. ### 1.3.3 Microcomputers - Microcomputers are single-user systems designed for personal use. - The computers are typically small and lightweight, and can be classified by their size and portability. - Examples of microcomputers include: - **Laptops:** A portable computer that has a flat screen, a hard disk, and USB connectors for flash drives. - **Notebooks:** A portable computer that is smaller than a laptop and weighs less than five pounds. - **Tablets:** A mobile computer smaller than a notebook. - **Workstations:** High-performance cousins of the personal computers that are used for engineering and scientific tasks. ### 1.3.4 Single-Board Microcomputers - Single-board microcomputers are designed with a single chip, which includes a microprocessor, memory, and signal lines to connect I/Os. - They are used primarily for instructional purposes, such as evaluating the performance of a given microprocessor. ## 1.4 Application: Microprocessor Controlled Temperature System (MCTS) - Using the concepts from this chapter, a typical microprocessor-controlled temperature system will sense room temperature, display it on a liquid crystal display (LCD) panel, and turn on/off a fan and heater based on the set point. ### 1.4.1 System Hardware - The system includes: - Microprocessor - Memory - Temperature sensor - Fan - Heater - LCD panel for display ### 1.4.2 System Software (Programs) - The program that runs the system is called a monitor program or system software. - This program is stored in ROM (or EPROM) and is divided into subtasks written as independent modules that execute in sequence. ## Summary - **Computer Structure** - **Digital Computer:** A programmable machine that processes binary data, traditionally represented by five components: CPU, ALU, control unit, memory, input, and output. - **CPU:** The central processing unit, includes arithmetic/logic unit, registers, instruction decoder, and the control unit. - **ALU:** Performs arithmetic and logic operations. - **Scale of Integration (SSI, MSI, LSI, VLSI, SLSI):** The process of designing circuits on a single chip. - **Microcomputers:** - **Microprocessor (MPU):** A semiconductor device manufactured by using the LSI technique. - **Microprocessor-based product:** A process or product that uses a microprocessor. - **Microcontroller:** A device that includes microprocessor, memory, and I/O signal lines on a single chip. - **Microcomputer:** A computer designed using a microprocessor as its CPU. - **Computer Languages:** - **Bit:** A binary digit 0 or 1. - **Byte:** A group of eight bits. - **Nibble:** A group of four bits. - **Word:** A group of bits the computer recognizes and processes at a time. - **Instruction:** A command in binary that is recognized and executed by the computer. - **Mnemonic:** A combination of letters to suggest an instruction. - **Program:** A set of instructions. - **Machine Language:** The binary medium of communication with a computer. - **Assembly Language:** A medium of communication with a computer in which programs are written in mnemonics. - **Low-Level Language:** Machine-dependent languages. - **High-Level Languages:** Machine-independent languages. - **Source Code:** A program written in English-like words. - **Compiler:** A program that translates high-level code into machine language. - **Interpreter:** A program that translates one statement at a time. - **Assembler:** A computer program that translates assembly language into machine code. - **Manual Assembly:** Translating machine code manually. - **ASCII:** A 7-bit alphanumeric code, used for communication between devices. - **Extended ASCII:** An 8-bit code that includes additional characters. - **Operating System:** A set of programs that manage interaction between hardware and software. - **Monitor Program:** A program that interprets input from a keyboard and converts it into binary. ## Looking Ahead - Chapter 2 introduces 8085 assembly language programming and provides an overview of the 8085 instruction set. - Chapter 3 expands on the architectural concepts of microcomputers. - Chapter 4 focuses on architecture details of the 8085 microprocessor and memory interfacing. - Chapter 5 covers I/O interfacing. ## Questions and Problems 1. List the components of a computer. 2. Explain the functions of each component of a computer. 3. What is a microprocessor? What is the difference between a microprocessor and a CPU? 4. Explain the difference between a microprocessor and a microcomputer. 5. Explain these terms: SSI, MSI, and LSI. 6. Define bit, byte, word, and instruction. 7. How many bytes make a word of 32 bits? 8. Specify the number of registers in a 2K memory chip. 9. Calculate the number of registers in a 64K memory board. 10. Explain the difference between the machine language and the assembly language of the 8085 microprocessor. 11. What is an assembler? 12. What are low- and high-level languages? 13. Explain the difference between a compiler and an interpreter. 14. What are the advantages of an assembly language in comparison with high-level languages? 15. What is an ASCII code? 16. Identify the difference between the ASCII and the extended ASCII codes. 17. Find the ASCII codes for the letters "A," "Z," and "m" from the ASCII table in Appendix E. 18. What is an operating system? 19. Identify the following peripherals as input or output: scanner, digital camera, printer, keyboard, mouse.