Methods, Math, String, Java PDF
Document Details
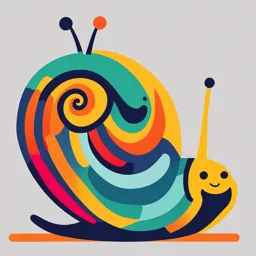
Uploaded by SatisfiedDandelion6498
Tags
Summary
This presentation covers methods in Java, focusing on Math, String, and Boolean types. It details the use of string literals, static methods, relational operators, and logical operators. The document includes numerous examples to illustrate the usage of Java methods.
Full Transcript
Methods Objectives Use Math methods Use string methods Understand boolean type Build your own Java methods Define parameters and pass arguments to them Distinguish between class and instance methods Build a method library...
Methods Objectives Use Math methods Use string methods Understand boolean type Build your own Java methods Define parameters and pass arguments to them Distinguish between class and instance methods Build a method library 2 Java’s Math class Provides a set of math functions Part of Java.lang, so you don’t have to import Static methods (no need to create a Math guy) Math methods most often take double arguments Math class constants: Math.E and Math.PI Example: compute volume of a sphere given radius – Formula: volume = 4/3 x PI x radius3 – Implementation: double volume = 4.0 / 3.0 * Math.PI * Math.pow(radius, 3.0); 3 Math Class 4 Math Class 5 The String Type Used to store a sequence of characters Example: String lastName = "Cat"; Different handles may refer to one String object String literal: 0 or more characters between “ and ” – Escape sequences can also be used in String literals String handle can be set to null or empty string 6 The String Type (continued) Instance method: message sent to an object Class method: message sent to a class – Indicated by the word static Java API lists a rich set of String operations Example of instance method sent to String object – char lastInit = lastName.charAt(0); Example of class method sent to String class – String PI_STR = String.valueOf(Math.PI); 7 The String Type (continued) 8 The String Type (continued) 9 The String Type (continued) Concatenation – Joins String values using the + operator – At least one operand must be a String type An illustration of concatenation – String word = "good"; word = word + "bye"; – Second statement refers to a new object – Garbage collector disposes of the de-referenced object +=: the concatenation-assignment shortcut – Example: word += "bye"; 10 The boolean Type Holds one of two values: true (1) or false (0) boolean (logical) expressions – Control flow of execution through a program Relational operators (, =, ==, !=) – Compare two operands and return a boolean value – May also be used to build simple logical expressions Example of a simple boolean expression – boolean seniorStatus = age >= 65; – Produces true if age >= 65; otherwise false 11 The boolean Type (continued) 12 The boolean Type (continued) 13 The boolean Type (continued) Logical operators (&&, ||, and !) – Used to build compound logical expressions Example of a compound logical expression – boolean liqWater; // declare boolean variable liqWater = 0.0 < wTemp && wTemp < 100.0; – wTemp must be > 0 and < 100 to produce true Truth table – Relates combinations of operands to each operator – Shows value produced by each logical operation 14 The boolean Type (continued) 15