Strings and Arrays PDF
Document Details
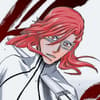
Uploaded by Kennzueee
Our Lady of Fatima University
Tags
Related
- ADP262S-Prac 8-Exception-2024 PDF, Cape Peninsula University of Technology, 17 Apr 2024
- Java Programming Fifth Edition - Characters, Strings, and the StringBuilder PDF
- Java Programming Fifth Edition: Characters, Strings, and StringBuilder - PDF
- Java Strings Learning Sheet - Programming 1 PDF
- Java Minor Assignment 2 (B.Sc. III Sem) PDF
- Core Java Programming Intake 45 - Dec 2024 PDF
Summary
This document details the creation and manipulation of strings and arrays in Java programming. It explains different ways to create and use strings, including string literals and constructors, with examples for each method. It also discusses one-dimensional and multi-dimensional arrays in Java and provides examples of how to declare and initialize them.
Full Transcript
IT2402 Strings and Arrays Strings (Oracle, 2023) Strings are a sequence of characters widely used in Java programming. Java provides the String class using java.lang to create and manipulate strings. They are considered objects in Java and have their speci...
IT2402 Strings and Arrays Strings (Oracle, 2023) Strings are a sequence of characters widely used in Java programming. Java provides the String class using java.lang to create and manipulate strings. They are considered objects in Java and have their specific methods for working on strings. String Index: Each character in a string has a specific position wherein the position of the first character starts at index 0. The length of a string can be defined based on the number of characters used. For example, the length of the "Hello World" string is 11. String "Hello World" Character in the string 'H' 'e' 'l' 'l' 'o' ' ' 'W' 'o' 'r' 'l' 'd' Index 0 1 2 3 4 5 6 7 8 9 10 Creating Strings A String can be written in two (2) ways: by directly assigning a string literal to a String object and by using the new keyword and String constructor to create a String object. For the example below, a direct assignment of a string literal to a String object is achieved. String greeting = "Hello World!"; The "Hello World!" used in the string is considered a string literal. A string literal is a series of characters found enclosed in double quotes and is considered constants. Whenever the compiler encounters a string literal, the compiler creates a String object with its value, in this case, Hello World!. String literals appear as an output exactly as entered. Another example with a string literal in strName: String greeting = "Hello World!"; String strName; strName = "Harley"; For the example below, a String object is created using the new keyword and a constructor. String strGreet = new String("Hello World!"); A constructor is a block of code that initializes a newly created object. The String class has 13 constructors that provide the initial value of the string using different sources. Some of the most commonly used constructors in the String class include the following: 1. String() – the default constructor to create an empty string. Syntax: String s = new String(); It creates a String object in the heap area with no value. The heap area contains all objects created and serves as a memory for objects and classes. 2. String(String str) – the most common String constructor used in Java. It creates a new String object in the heap area and stores the given value in it. Syntax, using a specified string str: String st = new String(String str); 07 Handout 1 *Property of STI Page 1 of 9 IT2402 3. String(char chars[]) – used to create a String object and store the array of characters in it. Syntax to create a String object using a specified array of characters: String str = new String(char chars[]); For example: char chars[] = {'e', 'f', 'g', 'h'}; String s3 = new String(chars); The object reference variable (s3) has the address of the value stored in the heap area. In a program code: package stringPrograms; public class History { public static void main(String[ ] args) { char chars[] = {'h', 'i', 's', 't', 'o','r','y'}; String s = new String(chars); System.out.println(s); } } In this sample program, a package was used with stringPrograms as the package name. A package is a specified named collection of classes in Java. Just like with import, it must be declared at the beginning of the source file. Packages are used to organize the many classes created when creating an application. Since the variable s contains the address of the value stored in the heap area, the output of this code is history. 4. String(char chars [], int startIndex, int count) – used to create and initialize a String object with a subrange of a character array. The startIndex argument defines the index at which the subrange begins, while count specifies the number of characters to be copied. Syntax to create a String object using a specified subrange of characters: String str = new String(char chars [], int startIndex, int count); For example: char chars[] = { 'p', 'e', 'a', 'c', 'e', 'f', 'u', 'l'}; String str = new String(chars, 2, 3); The object str contains the address of the value 'ace' since the starting index is 2 and the total number of characters to be copied (count) is 3. In a program code: package stringPrograms; public class Sign { public static void main(String[ ] args) { char chars[] = {'p', 'e', 'a', 'c', 'e', 'f', 'u', 'l'}; String s = new String(chars, 0, 5); System.out.println(s); } } 07 Handout 1 *Property of STI Page 2 of 9 IT2402 The output of this program is peace since the starting index starts at 0 and the total number of characters to be copied is 5. 5. String(byte byteArr[]) – used to create a new String object by decoding the given array of bytes such as by decoding ASCII values according to the system’s default character set. Syntax: String str = new String(byte byteArr[]); Program example: Decoding Unicode values. package stringPrograms; public class ByteArray { public static void main(String[] args) { byte b[] = {70, 114, 101, 101, 100, 111, 109}; // Range of bytes: -128 to 127. These byte values will be converted into corresponding characters. String s = new String(b); System.out.println(s); // Output: Freedom } } This program results in Freedom as 70 = F, 114 = r, 101 = e, 111 = o, and 109 = m. 6. String(byte byteArr[], int startIndex, int count) – this constructor also creates a new String object by decoding the ASCII values of the current string using the system’s default character. The concept of startIndex and count in the 4th String constructor also applies here. Syntax: String str = new String(byte byteArr[], int startIndex, int count); Program example: package stringPrograms; public class ByteArray { public static void main(String[] args) { byte b[] = {66, 82, 65, 86, 69, 82, 89}; // Range of bytes: -128 to 127. String s = new String(b, 1, 4); //RAVE System.out.println(s); } } This program results in RAVE as 82 = R, 65 = A, 86 = V, and 69 = E. String Methods The String class has various methods used to perform operations on Strings. The methods below can only create and return a new String containing the result of the operation. charAt() This method returns the character present in the specified index. Syntax: string.charAt (int index) ; Example: class Main { public static void main(String[] args) { String strA = "Java Programming"; System.out.println(strA.charAt(5)); // returns character at index 5 } } // Output: P 07 Handout 1 *Property of STI Page 3 of 9 IT2402 trim() This method removes the leading (starting) and trailing(ending) whitespaces from a string. Syntax: string.trim(); Example: class Main { public static void main(String[] args) { String strA = " Program with care "; System.out.println(strA.trim()); } } // Output: Program with care replace() This method changes the matching occurrences of a text or character in the string with the new text or character. Syntax: string.replace(char oldChar, char newChar); Example: class Main { public static void main(String[] args) { String strA = "batballing is a crime"; System.out.println(strA.replace('b', 'c')); //replace b with c } } // Output: catcalling is a crime equals() This method returns true if two strings are identical and false if otherwise. Syntax: string.equals(String str); Example: class Main { public static void main(String[] args) { String strA = "Learn Java"; String strB = "Learn Java"; String strC = "Learn Kolin"; boolean result; result = strA.equals(strB); // comparing strA with strB System.out.println(result); // true result = strA.equals(strC); // comparing strA with strC System.out.println(result); // false result = strC.equals(strA); // comparing strC with strA System.out.println(result); // false } } In this code, strA and strB are equal; thus, strA.equals(strB) returns true. On the other hand, strA and strC are not equal, resulting in returning false for strA.equals(strC) and strC.equals(strA). 07 Handout 1 *Property of STI Page 4 of 9 IT2402 toLowerCase() This method changes all characters in the string to lowercase or minuscule characters. Syntax: string.toLowerCase(); Example: class Main { public static void main(String[] args) { String strA = "BREATHE"; System.out.println(strA.toLowerCase());// convert to lowercase letters } } // Output: breathe Conversely, the method toUpperCase() can be used to convert all characters in the string to uppercase or majuscule characters. string.toUpperCase() is used as syntax. length() This method returns the number of characters in a string. Syntax: string.length(); Example: class Main { public static void main(String[] args) { String strA = "Kindness is free"; int length = strA.length(); // returns the length of strA System.out.println(strA.length());// Output: 16 } } concat() This method returns a new string based on two (2) concatenated or joined strings. Syntax: string.concat(String str); Example: class Main { public static void main(String[] args) { String strA = "Java "; String strB = "Programming"; // concatenate strA and strB System.out.println(strA.concat(strB)); // "Java Programming" // concatenate strB and strA System.out.println(strB.concat(strA)); // "ProgrammingJava " } } 07 Handout 1 *Property of STI Page 5 of 9 IT2402 indexOf() This method returns the index or position of the first occurrence of the specified character/substring within the string. To look for the index of a character, the syntax is string.indexOf(int ch, int fromIndex); ch refers to the character whose starting index is to be found while fromIndex is optional and if passed, the ch character is searched starting from this index. To look for the index of a substring within the string, the syntax is string indexOf(String str, int fromIndex); str refers to the string whose starting index is to be found while fromIndex, is also optional; if passed, the str string is searched starting from this index. Example without fromIndex: class Main { public static void main(String[] args) { String strA = "It gets better"; int result; result = strA.indexOf('g'); // getting index of character 'g' System.out.println(result); // Output: 3 } } Example with fromIndex: class Main { public static void main(String[] args) { String strA = "It gets better"; int result; // getting the index of character 't' // search starts at index 6 result = strA.indexOf('t', 6); System.out.println(result); // 10 // getting the index of "gets" // search starts at index 7 result = strA.indexOf("gets", 7); System.out.println(result); // -1 } } The first occurrence of 't' in the "It gets better" string is at index 2. However, the index of third 't' is returned when strA.indexOf('t', 6) is used. It is because the search starts at index 6. The "gets" string is in the "It gets better" string. However, strA.indexOf("gets", 7) returns -1 (string not found). It is because the search starts at index 7 and there is no "gets" in " better". compareTo() This method compares or matches two (2) strings based on the dictionary order. This comparison is based on the Unicode value of each character in the string. Syntax: string.compareTo(String str); 07 Handout 1 *Property of STI Page 6 of 9 IT2402 Example: class Main { public static void main(String[] args) { String strA = "Learn Java"; String strB = "Learn Java"; String strC = "Learn Kolin"; int result; result = strA.compareTo(strB); // comparing strA with strB System.out.println(result); // 0 result = strA.compareTo(strC); // comparing strA with strC System.out.println(result); // -1 result = strC.compareTo(strA); // comparing strC with strA System.out.println(result); // 1 } } In this example, strA and strB are equal, hence strA.compareTo(strB) returns 0. Moreover, strA comes before strC in the dictionary order. Thus, strA.compareTo(strC) returns negative, and strC.compareTo(strA) returns positive. Arrays (Agarwal & Bansal, 2023) An array represents data in a connected space in the computer memory. It is a collection of elements (a fixed number of variables) of the same data type referenced by a common name. Here are the considerations for determining an array: o The elements in the array are determined by the index o All elements in an array must have the same data types o The elements of an array can be referred to by an array name and an index An example of how arrays work: int[] num = new int ; The example declares and creates the array num consisting of six (6) elements in type int. Knowing the indices start at 0, the elements are accessed as follows: num, num, num, num, num, and num. When an array is created, Java automatically initializes the elements of numeric arrays as initialized to 0. num num num num num num num 0 0 0 0 0 0 Like any other primitive data type variable, an array can also be initialized with specific values when declared. For example, the following Java statement declares an array variable named kilograms of the value of type double with five (5) elements and initializes these elements to specific values: double kg[] = {2.65, 3.92, 1.85, 11.53, 14.57}; The initializer list contains initial values placed between braces and separated by commas. The elements are accessed: kg kg kg kg kg kg 2.65 3.92 1.85 11.35 14.57 07 Handout 1 *Property of STI Page 7 of 9 IT2402 Arrays must be declared first and then initialized to create and use an array in Java. The following is an example of different ways to declare array variables and how to assign and access each element. public class ArrayExample { public static void main(String[] args) { int[] ageList = new int; ageList = 23; //initialize the array elements using assignment operator ageList = 32; ageList = 43; ageList = 26; ageList = 34; ageList = 57; double kilograms[] = {2.65, 3.92, 1.85, 11.53, 14.57}; //initial values } } There are two (2) types of arrays: one-dimensional and multi-dimensional. One-dimensional Array A single or one-dimensional array is a list of the same typed variables. Here are other syntaxes and their examples that can be used in declaring a one-dimensional array: Syntax Example Data type [] variable_name; int []i; Data type variable name[]; int i[]; Data type [] variable_name, variable_name; int [] i, j; Datatype… variable_name; int…i; Table 1. One-dimensional array After declaring the variables for the array, it must be created in the memory. This can be done by using the new operator. new int ; This statement assigns eight (8) connecting memory i = new int ; locations of the type int to the variables i and j. j = new int ; The array can store eight (8) elements. Initializing this array can be done using a for loop: for(int var=0; var