MIPS Case Study: Leaf Procedure Example (Lecture 12)
Document Details
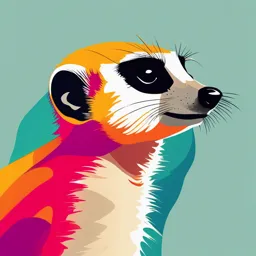
Uploaded by CuteWatermelonTourmaline
Kangwon National University
Tags
Summary
These notes cover MIPS (Microprocessor Architecture) assembly language, focusing on leaf procedures and how the stack is used. Examples show how variables that don't fit in registers are handled, and clear1(int array[], int size) examples are included, illustrating the process of clearing an array by setting all elements to 0.
Full Transcript
Leaf Procedure Example C code: int leaf_example(int g, h, i, j) { int f; f = (g + h) – (i + j) return f; } Arguments g, …, j in $a0, …, $a3 f in $s0 (hence, need to save $s0 on stack) Result in $v0...
Leaf Procedure Example C code: int leaf_example(int g, h, i, j) { int f; f = (g + h) – (i + j) return f; } Arguments g, …, j in $a0, …, $a3 f in $s0 (hence, need to save $s0 on stack) Result in $v0 1 Leaf Procedure Example (cont’d) Stack “grows” from Compiled MIPS code: high address to low address leaf_example: addi $sp, $sp, -4 sw $s0, 0($sp) Save $s0 on stack add $t0, $a0, $a1 add $t1, $a2, $a3 sub $s0, $t0, $t1 Procedure body add $v0, $s0, $zero Result lw $s0, 0($sp) addi $sp, $sp, 4 Restore $s0 jr $ra Return 2 Stack and $sp in Leaf Procedure Example Contents of Register $s0 3 Non-leaf Procedures Procedures that call other procedures For nested call, caller needs to save on the stack Its return address Any arguments and temporaries needed after the call Restore from the stack after call Example C code: int fact (int n) Argument n in $a0 { Result in $v0 if (n < 1) return 1; else return n * fact(n-1); } 4 Non-leaf Procedures (cont’d) int fact (int n) { if (n < 1) return 1; else return n * fact(n-1); Compiled MIPS code } fact: addi $sp, $sp, -8 // adjust stack for 2 items sw $ra, 4($sp) // save return address sw $a0, 0($sp) // save argument slti $t0, $a0, 1 // test for n < 1 beq $t0, $zero, L1 addi $v0, $zero, 1 // if so, result is 1 addi $sp, $sp, 8 // pop 2 items from stack jr $ra // and return L1: addi $a0, $a0, -1 // else decrement n jal fact // recursive call lw $a0, 0($sp) // restore original n lw $ra, 4($sp) // and return address addi $sp, $sp, 8 // pop 2 items from stack mul $v0, $a0, $v0 // multiply to get result jr $ra // and return 5 Local Data on the Stack Stack is also used to store variables that are local to procedure but do not fit in registers E.g., local arrays or structures The segment of the stack containing a procedure’s saved registers and local variables is its procedure frame (aka activation record) The frame pointer ($fp) points to the first word of the frame of a procedure – providing a stable “base” register for the procedure $fp is initialized using $sp on a call and $sp is restored using $fp on a return 6 Memory Layout Text: program code Static data: global variables e.g., static variables in C, constant arrays and strings $gp initialized to address allowing ±offsets into this segment Dynamic data: heap e.g., malloc in C, new in Java Stack: automatic storage 7 Example C Program 8 From Program to Process 9 Arrays vs. Pointers Array indexing involves Multiplying index by element size Adding to array base address Pointers correspond directly to memory addresses Can avoid indexing complexity clear1(int array[], int size) { int i; Example : Clearing an array for (i = 0; i < size; i += 1) array[i] = 0; } move $t0,$zero # i = 0 loop1: sll $t1,$t0,2 # $t1 = i * 4 add $t2,$a0,$t1 # $t2 = &array[i] sw $zero, 0($t2) # array[i] = 0 addi $t0,$t0,1 # i = i + 1 slt $t3,$t0,$a1 # $t3 = (i < size) bne $t3,$zero,loop1 # if (…) # goto loop1 10 Reading Recommended Chapter 2 2.2 Operations of the Computer Hardware 2.3 Operand of the Computer Hardware 2.5 Representing Instructions in the Computer 2.7 Instructions for Making Decisions 2.8 Supporting Procedures in Computer Hardware Appendix A-2 A.2 Assemblers A.3 Linkers A.4 Loading A.5 Memory Usage A.6 Procedure Call Convention 11