C Programming: Initializing Arrays of Structs PDF
Document Details
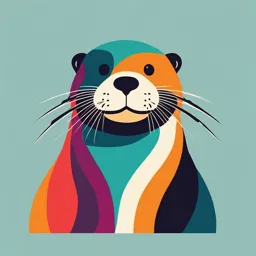
Uploaded by LuxuryAbundance
Algonquin College
Tags
Summary
This document provides examples and explanations on initializing arrays of structures in C programming. It details various methods for initializing arrays of structures. The examples given showcase different types of data and methods for initialization.
Full Transcript
CST8234 – C Programming Initializing Arrays of Structs Initializing Variables For simple data types, this is intuitive long nStudents = 50; char *strPrompt = “Welcome!” int nCourse = 3; 2 Initializing Arrays Additionally, many hav...
CST8234 – C Programming Initializing Arrays of Structs Initializing Variables For simple data types, this is intuitive long nStudents = 50; char *strPrompt = “Welcome!” int nCourse = 3; 2 Initializing Arrays Additionally, many have seen/used approaches to initialize array char *cards[] = { “AC”, “2C”, “3C”, “4C”, … , “QS”, “KS” }; char SUITS[] = { ‘C’, ‘D’, ‘H’, ‘S’ }; Note the size of the array is inferred automatically from the number of elements in the array 52 elements in cards 4 elements in SUITS I.e., You don’t need to necessarily specify char SUITS = { ‘C’, ‘D’, ‘H’, ‘S’ }; 3 Initializing Arrays If you specify a length that is different from the size of the initialization char inHand = { 0 }; The last provided value (e.g., 0) will be replicated for all the remaining elements. 4 Initializing Structs You can likewise initialize structs, where each element in the initialization is in the same order as the members of the struct typedef struct { char *rank; char suit; int isDealt; void (*pfuncEffect)( Card *pTopCard ); } Card; Card myCard = { “2”, ‘C’, 0, &drawTwoCards }; Note the multiple data types in the initializer… char *, char, int, and a function pointer 5 Initializing Arrays of Structs Just wrap one initializer in another typedef struct { char *rank; char suit; int isDealt; void (*pfuncEffect)( Card* pTopCard ); } Card; Card hand[] = { { “2”, ‘C’, 0, &drawTwoCards }, { “2”, ‘D’, 0, &drawTwoCards }, { “2”, ‘H’, 0, &drawTwoCards }, { “2”, ‘S’, 0, &drawTwoCards }, { “Q”, ‘S’, 0, &drawFiveCards }, { “8”, ‘C’, 0, &changeSuit }, { “3”, ‘S’, 0, NULL }, { “4”, ‘S’, 0, NULL } }; 6