Unit III Array - PDF
Document Details
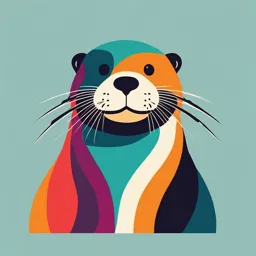
Uploaded by BonnySheep
First Year Engineering Department
Ms. Dhanashree S. Tayade
Tags
Summary
This document, titled 'Unit III Array,' provides an overview of arrays in C programming.It discusses array declarations, initializations, and offers illustrative examples, making it valuable for students of computer science learning about fundamental data structures. The document is not an exam paper.
Full Transcript
UNIT III Array Ms. Dhanashree S. Tayade, M.E. (Computer Science and Engineering), PhD pursuing Assistant Professor First Year Engineering Department Outline Arrays: What are Arrays? A Simple Program using Array, Array Initialization, Array Element...
UNIT III Array Ms. Dhanashree S. Tayade, M.E. (Computer Science and Engineering), PhD pursuing Assistant Professor First Year Engineering Department Outline Arrays: What are Arrays? A Simple Program using Array, Array Initialization, Array Elements in Memory, Passing Array Elements to a Function Multidimensional Array: Two Dimensional Arrays, initializing a Two-Dimensional Array, Memory Map of a Two-Dimensional Array Passing 2 D Array to a Function. Array An array is defined as the fixed-size collection of similar data items stored at contiguous memory locations. Arrays are the derived data type in C programming language which can store the primitive type of data such as int, char, double, float, etc. Array It also has the capability to store the collection of derived data types, such as pointers, structure, etc. The array is the simplest data structure where each data element can be randomly accessed by using its index number. C array is beneficial if you have to store similar elements. Properties of Array Each element of an array is of same data type and carries the same size, i.e., int = 4 bytes. Elements of the array are stored at contiguous memory locations where the first element is stored at the smallest memory location. Elements of the array can be randomly accessed since we can calculate the address of each element of the array with the given base address and the size of the data element. Array Declaration Syntax data_type array_name[array_size]; Example int marks; Here, int is the data_type, marks are the array_name, & 5 is the array_size. Array Initialization When the array is declared or allocated memory, the elements of the array contain some garbage value. So, we need to initialize the array to some meaningful value. Syntax: data_type array_name [size] = {value1, value2,... valueN}; e.g. int arr= {2,4,8,12,16}; Array Initialization Array Initialization with Declaration int num = { 2, 4, 12, 5, 45, 5 } ; Array Initialization with Declaration without Size int n[ ] = { 2, 4, 12, 5, 45, 5 } ; Array Initialization after Declaration (Using Loops) for (int i = 0; i < n; i++) { array_name[i] = value; } C Array: Declaration with Initialization The C array can be initialize at the time of declaration. Let's see the code. int marks={20,30,40,50,60}; In such case, there is no requirement to define the size. So it may also be written as the following code. int marks[ ]={20,30,40,50,60}; Example- To declare and initialize the array in C #include int main() { int i=0; int marks={20,30,40,50,60}; //declaration and initialization of array //traversal of array Output for(i=0;i= 0; i--) int main() { { printf("%d\n", arr[i]); int i,n,arr; } return 0; printf("Enter the size of array: "); } scanf("%d",&n); Output printf("Enter the elements: "); Enter the size of array: 5 Enter the elements: // taking input and storing it in an array 1 2 Displaying array elements in for( i = 0; i < n; i++) { 3 reversed order : scanf("%d", &arr[i]); 4 5 5 4 } 3 printf("Displaying array elements in 2 reversed order : \n"); 1 Example -Program to calculate sum of array in C #include Output void main() Sum of array is 55. { int array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; int sum=0, i; for(i = 0; i