COSC 114 Python Strings - Fall 2024 PDF
Document Details
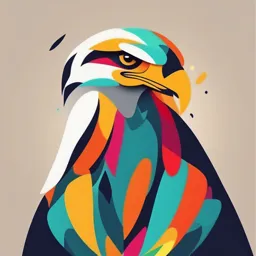
Uploaded by SmarterOnyx1408
Khalifa University
2024
COSC
Fatma Mohamed
Tags
Summary
These notes cover Python strings, including creation, access, slicing, length, concatenation, and various string functions and methods. Examples and exercises are included to demonstrate use. The content is from Fall 2024.
Full Transcript
Course Topic 9 Strings COSC 114 Introduction to Computing – Python Fatma Mohamed Fall 2024 Dr. Shakti Singh Strings A string in Python is a sequence of characters. Python strings can be created with single, double, or triple quotes. Triple quotes can be used for...
Course Topic 9 Strings COSC 114 Introduction to Computing – Python Fatma Mohamed Fall 2024 Dr. Shakti Singh Strings A string in Python is a sequence of characters. Python strings can be created with single, double, or triple quotes. Triple quotes can be used for multiline strings. The str() function converts the specified value into a string. number1='21' number2=str(31) Output : print(number1,number2) 21 31 Dr. Shakti Singh |2 Strings We can simultaneously declare and define a string by creating a variable of string type. This can be done in several ways which are as follows: greeting="Hello" hello= greeting seeyou=str('Bye') print(greeting,hello,seeyou) Output : Hello Hello Bye Dr. Shakti Singh |3 String Access String characters are accessed using indexes. If n is the total number of characters in the string, then the index of the first element is 0 while the index of the last element is n-1 mystr="Hello" Output : print(mystr,mystr,mystr,mystr,mystr) H e l l o mystr="Hello Bye" Output : print(mystr,mystr,mystr,mystr,mystr) H e l e Dr. Shakti Singh |4 String Access Negative Indexing : Negative indexing means start from the end. -1 refers to the last character, -2 refers to the second last character, and so on… mystr="Hello Bye" print(mystr[-1],mystr[-2],mystr[-3],mystr[-4],mystr[-5]) Output : e y B o Dr. Shakti Singh |5 String Access and Length The range of indexes can be specified by specifying where the range begins and ends. To access characters in strings, square brackets are used to slice along with the index(es) to get value(s) stored at that index(es) – Slicing mystr="Hello Bye" Output : print(mystr[1:4]) ell print(mystr[-4:-1]) By print(mystr[:3]) Hel print(mystr[3:]) lo Bye The length of the string can be determined by using len() function. mystr="Hello Bye" Output : print(len(mystr)) 9 Dr. Shakti Singh |6 String Access and Length In the slice operation, you can specify an optional third argument as the stride, which refers to the number of characters to move forward after the first character is retrieved from the string. By default the value of stride is 1 mystr="Hello Bye" Output : print(mystr[1:9]) # default stride=1 ello Bye print(mystr[1:9:1]) # stride=1 ello Bye print(mystr[1:9:2]) # stride=2, skips every second character el y print(mystr[1:9:5]) # stride=5, skips every fifth character eB print(mystr[::2]) # stride=2, no beginning or end HloBe print(mystr[-9:-1:3]) # stride=3, negative indexing HlB What is the output of eyB olleH print(mystr[::-1])? Dr. Shakti Singh |7 Strings – check To check if character(s) exists in the string use in keyword mystr="Hello Bye" Output : print('o' in mystr) True print('By' in mystr) True print('h' in mystr) False print('ello' not in mystr) False mystr="Hello Bye" Output : print ('Yes') if 'Bye' in mystr else print('No') Yes Dr. Shakti Singh |8 Strings – Traversing Output : H is in position 0 e is in position 1 mystr="Hello Bye" l is in position 2 count=0 l is in position 3 for i in mystr: o is in position 4 print(i, "is in position",count) is in position 5 count+=1 B is in position 6 y is in position 7 e is in position 8 Dr. Shakti Singh |9 Strings – Traversing Display the same output but using the len() function. Output : H is in position 0 e is in position 1 l is in position 2 l is in position 3 o is in position 4 is in position 5 mystr="Hello Bye" B is in position 6 i=0 y is in position 7 while i Error uabcvabcwabc4 Dr. Shakti Singh |14 Exercise – Example For the string "Python is my favorite subject," create a program that outputs the string without the letter "y" and any spaces. inp = 'Python is my favorite subject' out = '' for i in inp: if i != 'y' and i != ' ': out += i print(out) inp = 'Python is my favorite subject' for i in inp: lst=[i for i in inp if i != 'y' and i != ' '] out= ''.join(lst) print(out) Output (for both codes): Pthonismfavoritesubject Dr. Shakti Singh |15 Strings – Multiplication mystr='Hello' Output : print(mystr*3) HelloHelloHello mystr='Hello' Output : print((mystr+" ")*3) Hello Hello Hello mystr1="Hello" mystr2="Bye" print((mystr1*2+mystr2)*3) Output : HelloHelloByeHelloHelloByeHelloHelloBye Dr. Shakti Singh |16 Exercise – Example Write a Python function that takes a string as input and returns a new string with the first and last characters of the input string exchanged. If the input string has less than two characters, the function should return the original string. def exchange_chars(input_str): if len(input_str) < 2: return input_str else: return input_str[-1] + input_str[1:-1] + input_str #Function Call print(exchange_chars('Hello')) Output : oellH Dr. Shakti Singh |17 Strings Strings are immutable. Once defined, they cannot be changed. You can modify strings. However, the modification doesn’t happen on the original string. A copy of a string is created, modified, and returned to the caller. For example, if you have a string str1='Hello', and you try to change it, like with str1+='Bye’, it creates a new string "Hello Bye" and assigns it to str1, while the original string 'Hello' stays the same in memory. Dr. Shakti Singh |18 Strings str1='Hello' Output : str2='to' print('ID of str1 is:',id(str1)) ID of str1 is: 1632230062768 print('ID of str2 is:',id(str2)) ID of str2 is: 1632200660976 str1+=str2 print('str1 after concat. is:',str1) str1 after concat. is: Helloto print('ID of str1 is:',id(str1)) ID of str1 is: 1632230063152 print('ID of str2 is:',id(str2)) ID of str2 is: 1632200660976 str3=str1 print('str3 is:',str3) str3 is: Helloto print('ID of str3 is:',id(str3)) ID of str3 is: 1632230063152 What if str3=str1 is placed before the concatenation? Dr. Shakti Singh |19 Strings – Formatting Strings Formatting Review – %s,.format, f-strings etc.- Please Refer to Slide Set 2-3 and all subsequent slide sets Dr. Shakti Singh |20 Strings – Comparison Strings Comparison Review – Please Refer to Slide Set 2-3 and all subsequent slide sets Dr. Shakti Singh |21 Strings – Functions String Functions Review-.upper(),.lower(),.isupper() etc. – Please refer slide set T5 Dr. Shakti Singh |22 Strings – Functions capitalize( ) Syntax: string.capitalize() The capitalize() function returns a string where only the first character is the upper case. title( ) Syntax: string.title() The title() function returns a string where the first character in every word of the string is an upper case. mystr="hello How are you?" x=mystr.capitalize() Output : y=mystr.title() x is Hello how are you? print('x is {} \ny is {}'.format(x,y)) y is Hello How Are You? Dr. Shakti Singh |23 Strings – Functions find( ) Syntax: string.find(value, start, end) The find() function finds the first occurrence of the specified value. It returns -1 if the value is not found in that string. index( ) Syntax: string.index(value, start, end) The find() function finds the first occurrence of the specified value. It raises an exception if the value is not found. mystr="hello How are you?" x=mystr.find('r') Output : y=mystr.index('r') x is 11 print('x is {} \ny is {}'.format(x,y)) y is 11 Dr. Shakti Singh |24 Strings – Functions mystr="hello How are you?" Output : x=mystr.find('are',1,9) x is -1 y=mystr.index('are',4,14) y is 10 print('x is {} \ny is {}'.format(x,y)) mystr="hello How are you?" Output : x=mystr.find('bat') -1 print(x) mystr="hello How are you?" Output : x=mystr.index('bat') builtins.ValueError: substring print(x) not found Dr. Shakti Singh |25 Strings – Functions startswith( ) Syntax: string.startswith(character) It checks if a string starts with the given character. endswith( ) Syntax: string.endswith(character) It checks if a string ends with the given character. mystr="hello How are you?" Output : print(mystr.startswith('H')) False print(mystr.startswith('he')) True print(mystr.endswith('ou?')) True fruits=['apple','banana','kiwi','apricot','mango'] Output : newfruits=[i for i in fruits if i.startswith('a')] ['apple', 'apricot'] print(newfruits) Dr. Shakti Singh |26 Strings – Functions swapcase( ) Syntax: string.swapcase() The swapcase() function returns a string where all the upper-case letters are lower-case and vice versa. mystr="hello How are you?" mystr='Hello Today is 10th of feburary' x=mystr.swapcase() print(mystr.swapcase()) print(f'x is {x}') Output : Output : x is HELLO hOW ARE YOU? hELLO tODAY IS 10TH OF FEBURARY Dr. Shakti Singh |27 Strings – Functions replace( ) Syntax: string.replace(oldvalue, newvalue, optional count) The replace() function replaces a specified phrase with another phrase. mystr="hello How are you?" # replace all instances of o with u x=mystr.replace('o','u') # replace only first two instances of o with u y=mystr.replace('o','u',2) print(f'x is {x} \ny is {y}') Output : x is hellu Huw are yuu? y is hellu Huw are you? Dr. Shakti Singh |28 Strings – Functions split( ) Syntax: string.split(separator, maxsplit) The split() function splits a string into a list. mystr1="hello How are you?" mystr2="apple, orange, banana, watermelon" # split all words of a string x=mystr1.split() # split all words with comma as a seperator y=mystr2.split(",") What is the output of print(f'x is {x} \ny is {y}') print(mystr2.split())? Output : x is ['hello', 'How', 'are', 'you?'] y is ['apple', ' orange', ' banana', ' watermelon'] Dr. Shakti Singh |29 Strings – Functions mystr2="apple$orange$banana$watermelon" # split the string only once at the first $ seperator x=mystr2.split("$",1) # split the string twice at the first two $ seperators y=mystr2.split("$",2) print(f'x is {x} \ny is {y}') Output : x is ['apple', 'orange$banana$watermelon'] y is ['apple', 'orange', 'banana$watermelon'] Dr. Shakti Singh |30 Strings – Functions strip( ) Syntax: string.strip(characters) The strip() function removes any leading, and trailing whitespaces or a set of characters (optional). mystr1=" hello How are you? " Output: print(mystr1) hello How are you? print(mystr1.strip()) hello How are you? mystr1=" hello How are you?" Output: print(mystr1.strip('?')) hello How are you mystr1="*hello How are you?" Output: print(mystr1.strip('?*')) hello How are you mystr1="*hello How are you?" Output: print(mystr1.strip('h?*')) ello How are you Dr. Shakti Singh |31 Strings – Functions mystr2="apple,orange,banana\n" Output: mystr3="\n,tomato\t\t" apple,orange,banana mystr4=",cherry " print(mystr2+mystr3+mystr4) ,tomato ,cherry print(mystr2.strip()+mystr3.strip()+mystr4.strip()) Output: apple,orange,banana,tomato,cherry print((mystr2.strip()+mystr3.strip()+mystr4.strip()).split(',')) Output: ['apple', 'orange', 'banana', 'tomato', 'cherry'] Dr. Shakti Singh |32 Exercise – Example Write a Python function called reverse_words that takes in a string as input and returns the string with the order of the words reversed. def reverse_words(input_string): # split the input string into individual words list words = input_string.split() # reverse the order of the words reversed_words = words[::-1] # join the reversed words together reversed_string = " ".join(reversed_words) # return the final reversed string Output : return reversed_string you? are how Hello #Function Call print(reverse_words('Hello how are you?')) Dr. Shakti Singh |33 Exercise – Example Count the number of upper-case characters, lower-case characters, and all others in a string. mystr='Hello How are you?' count_up=count_low=count_others=0 for i in mystr: if i.isupper(): count_up+=1 elif i.islower(): count_low+=1 else: count_others+=1 print(f"Total upper-case characters: {count_up}, lower-case characters:{count_low}, Other characters: {count_others}") Output : Total upper-case characters: 2, lower-case characters:12, Other characters: 4 Dr. Shakti Singh |34 Exercises Write a Python function that takes in two strings as arguments and returns a new string that combines the two strings in the following way: The first letter of the first string, followed by the first letter of the second string, followed by the second letter of the first string, followed by the second letter of the second string, and so on, until all the letters of both strings have been used. If one string is longer than the other, the remaining letters of the longer string should be appended to the end of the combined string. Dr. Shakti Singh |35 Exercises Write a Python function that takes a string as input and returns a message indicating whether the password is weak, medium, or strong. A weak password is one that contains only letters or only numbers or is fewer than eight characters long. A medium password contains letters and numbers and is at least eight characters long. A strong password contains letters, numbers, and special characters and is at least eight characters long. Your function should return one of the following strings: "Password is weak" "Password is medium" "Password is strong" Dr. Shakti Singh |36 Exercises You are given a list of lists representing information about employees: employees = [["John Doe", 30, "Manager"],["Jane Smith", 25, "Developer"],["Alice Johnson", 35, "Designer"]] Write a Python function to print this information in a formatted table. The table should have three columns: "Name", "Age", and "Position", and each employee's information should be printed on a separate row. Dr. Shakti Singh |37 FYI Dr. Shakti Singh |38 Strings – Functions ord()function returns the ASCII code of the character and chr() function returns character represented by an ASCII number. ch='R' Output : 82 print(ord(ch)) print(chr(82)) Output : R Output : print(chr(112)) p print(ord('p')) 112 Dr. Shakti Singh |39 List of Built-in String Methods and Functions Dr. Shakti Singh |40 List of Built-in String Methods and Functions Dr. Shakti Singh |41 List of Built-in String Methods and Functions Dr. Shakti Singh |42