DrawPanel Implementation Guide PDF
Document Details
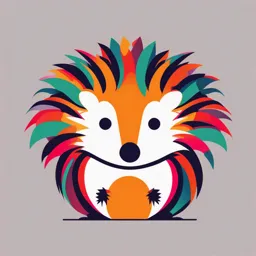
Uploaded by PositiveArtInformel8115
Lycée classique d'Echternach
Tags
Summary
This document provides a guide to using the DrawPanel class in Java for creating graphics. It covers how to create and customize DrawingPanels, including different drawing methods and techniques. The guide also discusses the use of colors, fonts, advanced features, and implementation with Object-Oriented Programming (OOP) and MVC
Full Transcript
How to Use and Implement DrawPanel in Java with MVC or OOP ========================================================== The DrawingPanel class provides a simple way to create graphics in Java. It is not part of the standard Java library, so you will need to download it separately. You can find the Dr...
How to Use and Implement DrawPanel in Java with MVC or OOP ========================================================== The DrawingPanel class provides a simple way to create graphics in Java. It is not part of the standard Java library, so you will need to download it separately. You can find the DrawingPanel class and its documentation online ^1^. This article will provide a comprehensive guide on how to use and implement DrawingPanel in Java programs, especially when following the Model-View-Controller (MVC) or Object-Oriented Programming (OOP) paradigms. Understanding the Basics of DrawPanel ------------------------------------- DrawingPanel is a subclass of javax.swing.JPanel that provides a drawing surface for your Java programs. It simplifies the process of creating a window and drawing graphics by handling the underlying window management and providing a Graphics object to draw on. ### Creating a DrawingPanel To create a DrawingPanel object, you can use one of its constructors: - - - - To make the DrawingPanel file work on Java 7 and lower, you might need to make two small modifications to its source code. Search for the two occurrences of the annotation \@FunctionalInterface and comment them out or remove those lines ^1^. Here\'s an example of creating a simple DrawingPanel: Java import java.awt.\*;\ \ public class Main {\ public static void main(String args) {\ DrawingPanel panel = new DrawingPanel(400, 400); // Creates a panel with width=400 and height=400\ Graphics g = panel.getGraphics(); // Gets the Graphics object to draw on the panel\ g.setColor(Color.RED); // Sets the drawing color to red\ g.drawRect(50, 50, 100, 100); // Draws a rectangle\ }\ } ### Drawing on the Panel Once you have a DrawingPanel object, you can obtain the Graphics2D object using the getGraphics() method. The Graphics2D object provides methods for drawing various shapes, lines, and text on the panel. Some common methods include: - - - - - - - - - - ### Working with Colors You can use the setColor() method of the Graphics2D object to set the color for drawing shapes and text. You can use predefined colors from the Color class (e.g., Color.RED, Color.BLUE, Color.GREEN) or create your own colors using the Color(int r, int g, int b) constructor, where r, g, and b represent the red, green, and blue components of the color, respectively ^2^. ### Working with Fonts To change the font for drawing text, you can use the setFont() method of the Graphics2D object. You can create a new Font object using the Font(String name, int style, int size) constructor, where name is the font name, style is the font style (e.g., Font.PLAIN, Font.BOLD, Font.ITALIC), and size is the font size in points. ### Advanced DrawingPanel Features DrawingPanel offers several advanced features that can be useful in various applications: - - - - ### Custom Drawing with paintComponent() The paintComponent() method is crucial for drawing on a DrawingPanel. It is called automatically whenever the panel needs to be redrawn, such as when the window is resized or another window overlaps it. To perform custom drawing, you need to override the paintComponent() method in your DrawingPanel subclass ^3^. Here\'s an example of how to override paintComponent() to draw a simple line: Java import java.awt.\*;\ \ public class MyDrawingPanel extends DrawingPanel {\ \ \@Override\ public void paintComponent(Graphics g) {\ super.paintComponent(g); // Always call the superclass method first\ Graphics2D g2d = (Graphics2D) g; // Cast to Graphics2D for more features\ g2d.drawLine(50, 50, 200, 200); // Draw a line\ }\ } In this example, we create a subclass of DrawingPanel called MyDrawingPanel. We override the paintComponent() method, making sure to call the superclass method (super.paintComponent(g)) first. Then, we cast the Graphics object to Graphics2D to access more advanced drawing features and use drawLine() to draw a line on the panel. Common Use Cases for DrawPanel ------------------------------ DrawingPanel can be used in a variety of applications, including: - - - Drawing Techniques with DrawingPanel ------------------------------------ DrawingPanel provides a rich set of tools for creating various graphical elements. Here are some common drawing techniques: - - - Connecting DrawPanel to the Main Frame and Other Classes -------------------------------------------------------- In a typical Java GUI application, you\'ll have a main frame (a JFrame object) that contains other components, including your DrawingPanel. To add your DrawingPanel to the main frame, you can use the add() method of the JFrame\'s content pane. Here\'s an example: Java import javax.swing.\*;\ import java.awt.\*;\ \ public class Main {\ public static void main(String args) {\ JFrame frame = new JFrame(\"My Drawing App\");\ frame.setDefaultCloseOperation(JFrame.EXIT\_ON\_CLOSE);\ frame.setSize(500, 500);\ \ DrawingPanel panel = new DrawingPanel(400, 400);\ frame.add(panel); // Adds the panel to the frame\'s content pane\ \ frame.setVisible(true);\ }\ } In this example, we create a JFrame and a DrawingPanel. Then, we add the DrawingPanel to the frame using frame.add(panel). This will place the DrawingPanel inside the frame\'s content pane, making it visible to the user. To connect DrawingPanel to other classes, you can use various techniques, such as: - - Using DrawPanel with ArrayLists ------------------------------- You can use ArrayLists to store and manage graphical objects that you want to draw on your DrawingPanel. For example, you can create an ArrayList of Shape objects and then iterate over the list to draw each shape on the panel. This approach is particularly useful when you have a dynamic number of objects to draw or when you need to manipulate the objects individually. Here\'s an example of how to use an ArrayList to draw and update multiple circles: Java import javax.swing.\*;\ import java.awt.\*;\ import java.awt.event.MouseAdapter;\ import java.awt.event.MouseEvent;\ import java.util.ArrayList;\ \ public class Main {\ public static void main(String args) {\ JFrame frame = new JFrame(\"ArrayList Example\");\ frame.setDefaultCloseOperation(JFrame.EXIT\_ON\_CLOSE);\ frame.setSize(500, 500);\ \ DrawingPanel panel = new DrawingPanel(400, 400);\ frame.add(panel);\ \ ArrayList\ circles = new ArrayList\();\ \ panel.addMouseListener(new MouseAdapter() {\ \@Override\ public void mouseClicked(MouseEvent e) {\ circles.add(new Circle(e.getX(), e.getY(), 20, Color.BLUE));\ panel.repaint();\ }\ });\ \ panel.addMouseMotionListener(new MouseAdapter() {\ \@Override\ public void mouseMoved(MouseEvent e) {\ for (Circle circle : circles) {\ if (circle.contains(e.getX(), e.getY())) {\ circle.setColor(Color.RED);\ } else {\ circle.setColor(Color.BLUE);\ }\ }\ panel.repaint();\ }\ });\ \ frame.setVisible(true);\ }\ \ static class Circle {\ private int x;\ private int y;\ private int radius;\ private Color color;\ \ public Circle(int x, int y, int radius, Color color) {\ this.x = x;\ this.y = y;\ this.radius = radius;\ this.color = color;\ }\ \ public void draw(Graphics2D g2d) {\ g2d.setColor(color);\ g2d.fillOval(x - radius, y - radius, radius \* 2, radius \* 2);\ }\ \ public boolean contains(int x, int y) {\ return Math.sqrt(Math.pow(x - this.x, 2) + Math.pow(y - this.y, 2)) \