Graphics Programming Using AWT PDF
Document Details
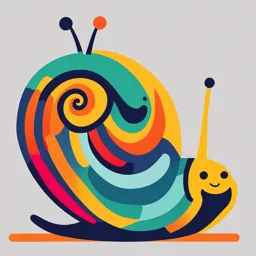
Uploaded by ProudAndradite
Tags
Summary
This document provides an introduction to Graphics Programming using AWT. It explains the concept of GUI and its advantages over CUI. It covers topics like creating frames, drawing shapes, and displaying images in Java.
Full Transcript
# GRAPHICS PROGRAMMING USING AWT ## CHAPTER 27 Whenever an application (or software) is created, the user can work with it in two ways. The first way is where the user can remember some commands on which the application is built and type those commands to achieve the respective tasks. For example,...
# GRAPHICS PROGRAMMING USING AWT ## CHAPTER 27 Whenever an application (or software) is created, the user can work with it in two ways. The first way is where the user can remember some commands on which the application is built and type those commands to achieve the respective tasks. For example, the user may have to type a PRINT command to send a file contents to a printer. Here, the user should know the syntax and correct usage of the PRINT command. Then only he can interact with the application properly. This is called CUI (Character User Interface) since the user has to use characters or commands to interact with the application. The main disadvantage in CUI is that the user has to remember several commands and their use with correct syntax. Hence, CUI is not user-friendly. A person who does not know any thing about computers will find this CUI very difficult. The second way is where the user need not remember any commands but interacts with any application by clicking on some images or graphics. For example, if the user wants to print a file, he can click on a printer image and the rest of the things will be taken care of by the application. The user has to tell how many copies he wants and printing continues. This is very easy for the user since the user remembers only some symbols or images, like a magnifying glass symbol for searching, a briefcase symbol for a directory, etc. This environment where the user can interact with an application through graphics or images is called GUI (Graphics User Interface). GUI has the following advantages: - It is user-friendly. The user need not worry about any commands. Even a layman will be able to work with the application developed using GUI. - It adds attraction and beauty to any application by adding pictures, colors, menus, animation, etc. We can observe almost all websites lure their visitors on Internet since they are developed attractively using GUI. - It is possible to simulate the real life objects using GUI. For example, a calculator program may actually display a real calculator on the screen. The user feels that he is interacting with a real calculator and he would be able to use it without any difficulty or special training. So, GUI eliminates the need of user training. - GUI helps to create graphical components like push buttons, radio buttons, check boxes, etc. and use them effectively in our programs. ## AWT Abstract Window Toolkit (AWT) represents a class library to develop applications using GUI. The java.awt package got classes and interfaces to develop GUI and let the users interact in a more friendly way with the applications. Figure 27.1 shows some important classes of java.awt package. ## Creating a Frame A frame becomes the basic component in AWT. The frame has to be created before any other component. The reason is that all other components can be displayed in a frame. There are three ways to create a frame, which are as follows: - Create a Frame class object, ```java Frame f = new Frame(); ``` - Create a Frame class object and pass its title also, ```java Frame f = new Frame("My frame"); ``` - The third way is to create a subclass MyFrame to the Frame class and create an object to the subclass as: ```java class MyFrame extends Frame MyFrame f = new MyFrame(); ``` Since, MyFrame is a subclass of Frame class, its object f contains a copy of Frame class and hence f represents the frame. In all these cases, a frame with initial size of 0 pixels width and 0 pixels height will be created, which is not visible on the screen. You may be wondering what these pixels are. A pixel (short for picture element) represents any single point or dot on the screen. Any data or pictures which are displayed on the screen are composed of several dots called pixels. Nowadays, monitors can accommodate 800 pixels horizontally and 600 pixels vertically. So the total pixels seen on one screen would be 800x600 = 480,000 pixels. This is called 'screen resolution'. See Figure 27.3. The more the screen resolution, the more clarity a picture will have on the screen when displayed. This is the reason most laptops use 1024x768 pixels resolution. Since, the size of our frame would be 0px width and 0px height, it is not visible and hence should increase its size so that it would be visible to us. This is done by setSize() method, as: ```java f.setSize(400,350); ``` Here, the frame's width is set to 400 px and height to 350 px. Then, we can display the frame, using setVisible() method, as: ```java f.setVisible(true); ``` ## Program 1: Create a frame by creating an object to Frame class. ```java //Creating a frame - version 1 import java.awt.*; class MyFrame { public static void main(String args[]) { //create a frame Frame f = new Frame("My AWT frame"); //set the size of the frame f.setSize(300,250); //display the frame f.setVisible(true); } } ``` ## Program 2: Create a frame by creating an object to the subclass of Frame class. ```java //Creating a frame - version 2 import java.awt.*; class MyFrame extends Frame { //call super class constructor to store title MyFrame (String str) { super(str); } public static void main(String args[]) { //create a frame with title MyFrame f = new MyFrame("My AWT frame"); //set the size of the frame f.setSize(300,250); //display the frame f.setVisible(true); } } ``` This frame can be minimized, maximized and resized, but cannot be closed. Even if we click on close button of the frame, it will not perform any closing action. Now the question is how to close the frame? Closing a frame means attaching action to the component. To attach actions to the components, we need 'event delegation model'. Let us discuss what it is. ## Event Delegation Model When we create a component, generally the component is displayed on the screen but is not capable of performing any actions. For example, we created a push button, which can be displayed but cannot perform any action, even when someone clicks on it. But user expectation will be different. A user wants the push button to perform some action. Hence, he clicks on the button. Clicking like this is called event. An event represents a specific action done on a component. Clicking, double clicking, typing data inside the component, mouse over, etc. are all examples of events. When an event is generated on the component, the component will not know about it because it cannot listen to the event. To let the component understand that an event is generated.on it, we should add some listener to the components. A listener is an interface which listens to an event coming from a component. A listener will have some abstract methods which need to be implemented by the programmer. When an event is generated by the user on the component, the event is not handled by the component. On the other hand, the component sends (delegates) that event to the listener attached to it. The listener will not handle the event. It hands over (delegates) the event to an appropriate method. Finally, the method is executed and the event is handled. This is called 'event delegation model'. See Figure 27.4. ## Important Interview Question **What is event delegation model?** Event delegation model represents that when an event is generated by the user on a component, it is delegated to a listener interface and the listener calls a method in response to the event. Finally, the event is handled by the method. **Which model is used to provide actions to AWT components?** Event delegation model. So, the following steps are involved in event delegation model: - We should attach an appropriate listener to a component. This is done using addxxxListener() method. Similarly, to remove a listener from a component, we can use removexxxListener() method. - Implement the methods of the listener, especially the method which handles the event. - When an event is generated on the component, then the method in step 2 will be executed and the event is handled. **What is the advantage of event delegation model?** In this model, the component is separated from the action part. So there are two advantages: - The component and the action parts can be developed in two separate environments. For example, we can create the component in Java and the action logic can be developed in VisualBasic. - We can modify the code for creating the component without modifying the code for actions of the component. Similarly, we can modify the action part without modifying the code for the component. Thus, we can modify one part without effecting any modification to other parts. This makes debugging and maintenance of code very easy. ## Closing the Frame We know Frame is also a component. We want to close the frame by clicking on its close button. Let us follow these steps to see how to use event delegation model to do this: - We should attach a listener to the frame component. Remember, all listeners are available in java.awt.event package. The most suitable listener to the frame is 'window listener'. It can be attached using addWindowListener() method as: ```java f.addwindowListener(windowListener obj); ``` Please note that the addWindowListener() method has a parameter that is expecting object of WindowListener interface. Since it is not possible to create an object to an interface, we should create an object to the implementation class of the interface and pass it to the method. - Implement all the methods of the WindowListener interface. The following methods are found in WindowListener interface: ```java public void windowActivated (windowEvent e) public void windowClosed (WindowEvent e) public void windowClosing (WindowEvent e) public void windowDeactivated (windowEvent e) public void windowDeiconified (windowEvent e) public void windowIconified (windowEvent e) public void windowOpened (windowEvent e) ``` In all the preceding methods, WindowListener interface calls the public void windowClosing() method when the frame is being closed. So, implementing this method alone is enough, as: ```java public void windowClosing (windowEvent e) { //close the application System.exit(0); } ``` For the remaining methods, we can provide empty body. So, when the frame is closed, the body of this method is executed and the application gets closed. In this way, we can handle the frame closing event. ## Program 3: Create a frame and close it on clicking the close button. ```java //Creating a frame and closing it. import java.awt.*; import java.awt.event.*; class MyFrame extends Frame { public static void main(String args[]) { //create a frame Frame f = new Frame("My AWT frame"); //set a title for the frame f.setTitle("My AWT frame"); //set the size of the frame f.setSize(300,250); //display the frame f.setVisible(true); //close the frame f.addwindowListener(new Myclass()); } class Myclass implements WindowListener { public void windowActivated (WindowEvent e) {} public void windowClosed (windowEvent e) {} public void windowClosing (WindowEvent e) { System.exit(0); } public void windowDeactivated (WindowEvent e) {} public void windowDeiconified (windowEvent e){} public void windowIconified (windowEvent e){} public void windowOpened (windowEvent e){} } } ``` In this program, we not only create a frame but also close the frame when the user clicks on close button. For this purpose, we use WindowListener interface. Here, we had to mention all the methods of WindowListener interface, just for the sake of method. This is really cumbersome. There is another way to escape this. There is a class WindowAdapter in java.awt.event package, that contains all the methods of the WindowLister interface with an empty implementation (body). If we can extend Myclass from this WindowAdapter class, then we need not write all the methods with empty implementation. We can write only the method which interests us. This is shown in Program 4. ## Program 4: Close the frame using WindowAdapter class. ```java //Creating a frame and closing it. import java.awt.*; import java.awt.event.*; class MyFrame extends Frame { public static void main(String args[]) { //create a frame with title MyFrame f = new MyFrame(); //set a title for the frame f.setTitle("My AWT frame"); //set the size of the frame f.setSize(300,250); //display the frame f.setVisible(true); //close the frame f.addwindowListener(new Myclass()); } class Myclass extends windowAdapter { public void windowClosing (windowEvent e) { System.exit(0); } } } ``` ## Important Interview Question **What is an adapter class?** An adapter class is an implementation class of a listener interface which contains all methods implemented with empty body. For example, WindowAdapter is an adapter class of WindowListener interface. Adapter classes reduce overhead on programming while working with listener interfaces. Please observe Program 4. In this, even the code of Myclass can be copied directly into addWindowListener() method, as: ```java f.addwindowListener(new windowAdapter() { public void windowClosing(windowEvent e) { System.exit(0); } }); ``` This looks a bit confusing, but it is correct. We copy the code of Myclass into the method of MyFrame class. But, in the preceding code, we cannot find the name of Myclass anywhere in the code. It means the name of Myclass is hidden in MyFrame class and hence Myclass is an inner class in MyFrame class whose name is not mentioned. Such an inner class is called 'anonymous inner class'. ## Important Interview Question **What is anonymous inner class?** Anonymous inner class is an inner class whose name is not mentioned, and for which only one object is created. Let us now rewrite Program 4 again using anonymous inner class concept to close the frame. ## Program 5: Close the frame using an anonymous inner class. ```java //Creating a frame and closing it. import java.awt.*; import java.awt.event.*; class MyFrame extends Frame { public static void main(String args[]) { //create a frame with title MyFrame f = new MyFrame(); //set a title for the frame f.setTitle("My AWT frame"); //set the size of the frame f.setSize(300,250); //display the frame f.setVisible(true); //close the frame. Here Myclass name is not mentioned //but its object is passed to the method. f.addwindowListener(new windowAdapter() { public void windowClosing (WindowEvent e) { System.exit(0); } }); } } ``` So far, we discussed the following three ways to close the frame: - By implementing all the methods of WindowListener interface. - By using WindowAdapter class and by implementing only the required method. - By directly copying the code of an anonymous inner class. ## Uses of a Frame Once, the frame is created, we can use it for any of the purposes mentioned here: - To draw some graphical shapes like dots, lines, rectangles, etc. in the frame. - To display some text in the frame. - To display pictures or images in the frame. - To display components like push buttons, radio buttons, etc. in the frame. ## Drawing in the Frame Graphics class of java.awt package has the following methods which help to draw various shapes. - `drawLine (int x1, int y1, int x2, int y2)` This method is useful to draw a line connecting (x1,y1) and (x2,y2). - `drawRect(int x, int y, int w, int h)` This method draws outline of a rectangle. The left top corner of the rectangle starts at (x,y), the width is w, and the height is h. - `drawRoundRect (int x, int y, int w, int h, int arcw, int arch)`: This method draws the outline of a rectangle with rounded corners. The rectange's top left corner starts at (x,y), the width is w, and height is h. The rectangle will have rounded corners. The rounding is specified by arcw and arch. arcw represents horizontal diameter of the arc at the corner and arch represents vertical diameter of the arc at the corner. - `drawOval (int x, int y, int w, int h)`: This method draws a circle or ellipse bounded in the region of a rectangle starting at (x,y), with width w and height h. - `drawArc(int x, int y, int w, int h, int sangle, int aangle)`: Draws an arc bounded by a rectangle region of (x,y), with width w and height h. Here, sangle represents the beginning angle measured from 3 o'clock position in the clock. aangle represents the arc angle relative to start angle. sangle + aangle = end angle. If the sangle is positive, the arc is drawn counter clock wise and if it is negative, then it is drawn clockwise. To understand the arcs, see Figure 27.5. - `drawPolygon (int x[], int y [], int n)`: This method draws a polygon that connects pairs of coordinates mentioned by the arrays x[] and y[]. Here, x [] is an array which holds x coordinates of points and y[] is an array which holds y coordinates. n represents the number of pairs of coordinates. To draw any of these shapes, we need paint () method of Component class, which refreshes the frame contents automatically when a drawing is displayed. This method is useful whenever we want to display some new drawing or text or images in the frame. The paint() method is automatically called when a frame is created and displayed. ## Program 6: Draw a smiling face using the methods of Graphics class. ```java //Drawing a smiling face in a frame import java.awt.*; import java.awt.event.*; class Drawl extends Frame { Draw1() { //close the frame this.addwindowListener(new windowAdapter() { public void windowClosing (WindowEvent e) { System.exit(0); } }); } //to refresh the frame contents public void paint(Graphics g) { //set blue color for drawing g.setColor(Color.blue); //display a rectangle to contain drawing g.drawRect(40,40,200,200); //face g.drawoval (90,70,80,80); //eyes g.drawoval (110,95,5,5); g.drawoval (145,95,5,5); //nose g.drawLine(130,95,130,115); //mouth g.drawArc(113,115,35,20,0,-180); } public static void main(String args[]) { //create the frame Draw1 d = new Drawl(); //set the size and title d.setSize(400,400); d.setTitle("My drawing"); //display the frame d.setVisible(true); } } ``` ## Filling with Colors To fill any shape with a desired color, first of all we should set a color using setColor() method. Then any of the following methods will draw those respective shapes by filling with the color. - `fillRect(int x, int y, int w, int h)`: This method draws a rectangle and fills it with the specified color. - `fillRoundRect (int x, int y, int w, int h, int arcw, int arch)`: This method draws filled rectangle with rounded corners. - `filloval (int x, int y, int w, int h)`: This method is useful to create an oval (circle or ellipse) which is filled with a specified color. - `fillArc(int x, int y, int w, int h, int sangle, int aangle)`: This method draws an arc and fills it with a specified color. - `fillPolygon (int x[], int y [], int n)`: This method draws and fills a polygon with a specified color. Let us rewrite Program 6, using filled shapes and see the output. ## Program 7: Fill the shapes with some colors. ```java //Drawing a smiling face in a frame with filled colors import java.awt.*; import java.awt.event.*; class Draw2 extends Frame { Draw2 () { //close the frame this.addwindowListener(new windowAdapter() { public void windowClosing (windowEvent e) { System.exit(0); } }); } public void paint(Graphics g) { //set blue color g.setColor(Color.blue); //display a rectangle to contain drawing g.fillRect(40,40, 200, 200); //set yellow color g.setColor(Color.yellow); //face g.filloval (90,70,80,80); //set black color g.setColor(Color.black); //eyes g.filloval (110,95,5,5): g.filloval (145,95,5,5); //nose g.drawLine(130,95,130,115); //set red color g.setColor(Color.red); //mouth g.fillArc(113,115,35,20,0,-180); } public static void main(String args[]) { //create the frame Draw2 d = new Draw2(); //set the size and title d.setSize(400,400); d.setTitle("My drawing"); //display the frame d.setVisible(true); } } ``` Let us write a Java program to see how to create a polygon. Now, a polygon has several sides and create each side, we need x and y coordinates to connect by a straight line. All the x coordinat and y coordinates can be stored in two arrays as: ```java int x[] = {40,200,40,100}; int y[] = {40,40,200,200}; ``` Now, if a polygon is drawn, it starts at (40, 40) and connects it with (200, 40), from there a line connects it to (40, 200) and finally ends at (100,200). So here, totally 4 pairs of coordinates are there, and hence the polygon can be drawn using: ```java g.drawPolygon(x, y, 4); ``` Or, to get a filled polygon, we can use: ```java g.fillPolygon(x, y, 4); ``` ## Program 8: Create a polygon that is filled with green color. This polygon must be created inside a rounded rectangle which is filled with red color. ```java //Drawing a smilie in a frame import java.awt.*; import java.awt.event.*; class DrawPoly extends Frame { DrawPoly() { //close the frame this.addwindowListener(new windowAdapter() { public void windowClosing (windowEvent e) { System.exit(0); } }); } public void paint(Graphics g) { //set red color g.setColor(Color.red); //display a filled rounded rectangle g.fillRoundRect(30,30,250,250,30,30); //set green color g.setColor(Color.green); //take x and y coordinates in arrays int x[] = {40,200,40,100}; int y[] = {40,40,200,200}; //there are 4 pairs of x, y coordinates int num = 4; //create filled polygon connecting the coordinates g.fillPolygon(x, y, num); } public static void main(String args[]) { //create the frame DrawPoly d = new DrawPoly(); //set the size and title d.setSize(400,400); d.setTitle("My Polygon"); //display the frame d.setVisible(true); } } ``` Finally, another Java program to depict a small figure is shown in Program 9. ## Program 9: Draw a home with moon at back ground. ```java //My Home import java.awt.*; import java.awt.event.*; class Home extends Frame { Home () { this.addwindowListener(new windowAdapter() { public void windowClosing (WindowEvent e) { System.exit(0); } }); } public void paint(Graphics g) { //store x, y coordinates in x[] and y[] int x[] = {375,275,475}; int y[] = {125,200,200}; int n = 3; //no. of pairs //set gray background for frame this.setBackground(Color.gray); //set yellow color for rectangle house g.setColor(Color.yellow); g.fillRect(300,200,150,100); //set blue color for another rectangle door g.setColor(Color.blue); g.fillRect(350,210,50,60); //draw a line g.drawLine(350,280,400,280); //set dark gray for polygon - roof g.setColor(Color.darkGray); g.fillPolygon(x,y, n); //set cyan color for oval moon g.setColor(Color.cyan); g.filloval (100, 100, 60, 60); //set green for arcs g.setColor(Color.green); g.fillArc(50,250,150,100,0,180); g.fillArc(150, 250, 150, 100,0,180); g.fillArc(450,250,150,100,0,180); //draw a line the bottom most line of drawing g.drawLine(50,300,600,300); //display some text g.drawString("My Happy Home", 275, 350); } public static void main(String args[]) { //create the frame Home h = new Home(); //set the size and title h.setSize(500,400); h.setTitle("My Home"); //display the frame h.setVisible(true); } } ``` ## Displaying Dots To display a dot or point on the screen, we can take the help of drawLine() method. For example, to display a point at (300,300) coordinates, we should use drawLine() method in such a way that the line is drawn starting from the same point and ending at the same point, as: ```java drawLine (300,300,300,300); ``` This will display a dot at (300,300). The following program illustrates how to draw several white dots on the black screen. ## Program 10: Display several dots on the screen continuously. ```java //Displaying a group of dots on black screen import java.awt.*; import java.awt.event.*; class Points extends Frame { public void paint (Graphics g) { //set white color for dots g.setColor(Color.white); for (;;) //display dots forever { //generate x, y coordinates randomly. Maximum 800 and 600 px int x = (int) (Math.random() * 800); int y = (int) (Math.random() * 600); //Use drawLine() to display a dot g.drawLine(x, y, x, y); try{ //make a time delay of 20 milliseconds Thread.sleep(20); }catch(InterruptedException ie){} } } public static void main(String args[]) { //create frame Points obj = new Points(); //set black background color for frame obj.setBackground(Color.black); //set the size and title for frame obj.setSize(500,400); obj.setTitle("Random dots"); //display the frame obj.setVisible(true); } } ``` ## Displaying text in the frame To display some text or strings in the frame, we can take the help of drawstring() method of Graphics class, as: ```java g.drawString("Hello", x,y); ``` Here, the string "Hello" will be displayed starting from the coordinates (x, y). If we want to set some color for the text, we can use setColor() method of Graphics class, as: ```java g.setColor(Color.red); ``` There are two ways to set a color in AWT. The first way is by directly mentioning the needed color name from Color class, as Color.red, Color.yellow, Color.cyan, etc. All the standard colors are declared as constants in Color class, as shown in the Table 27.1: | Color | |---|---| | Color.black | Color.blue | Color.cyan | | Color.pink | Color.red | Color.orange | | Color.magenta | Color.darkGray | Color.gray | | Color.lightGray | Color.green | Color.yellow | | Color.white | The second way to mention any color is by combining the three primary colors: red, green, and blue while creating Color class object, as: ```java Color c = new Color(r,g,b); ``` Here, r,g,b values can change from 0 to 255. 0 represents no color. 10 represents low intensity whereas 200 represents high intensity of color. Thus, ```java Color c = new Color(255,0,0); //red color Color c = new Color(255,255,255); //white color Color c = new Color(0,0,0); //black color ``` This Color class object c should be then passed to setColor() method to set the color. To set some font to the text, we can use setFont() method of Graphics class, as: ```java g.setFont(Font object); ``` This method takes Font class object, which can be created as: ```java Font f = new Font("SansSerif", Font.BOLD, 30); ``` Here, "SansSerif" represents the font name, Font. BOLD represents the font style and 30 represents the font size in pixels. There are totally 3 styles that we can use: - Font.BOLD - Font.ITALIC - Font.PLAIN We can also combine any two styles, for example, to use bold and italic, we can write Font.BOLD + Font.ITALIC ## Program 11: Display some text in the frame using drawString() method. ```java //Frame with background color and message import java.awt.*; import java.awt.event.*; class Message extends Frame { Message() { //close the frame when close button clicked addwindowListener(new windowAdapter() { public void windowClosing (WindowEvent we) { System.exit(0); } }); }//end of constructor public void paint(Graphics g) { //set background color for frame this.setBackground(new Color(100,20,20)); //set font for the text Font f = new Font("Arial", Font.BOLD+Font.ITALIC, 30); g.setFont(f); //set foreground color g.setColor(Color.green); //display the message g.drawString("Hello, How are u? ", 100,100); } public static void main(String args[]) { Message m = new Message(); m.setSize(400,300); m.setTitle("This is my text"); m.setVisible(true); } } ``` ## Knowing the Available Fonts It is possible to know which fonts are available in our system. When we know the available fonts, we can use any of the font names to create Font class object. First of all, we should get the local graphics environment, as: ```java GraphicsEnvironment ge = GraphicsEnvironment.getLocalGraphicsEnvironment(); ``` Now, this ge contains the available font names which can be retrieved as: ```java String fonts[] = ge.getAvailableFontFamilyNames(); ``` ## Program 12: Know which fonts are available in a local system. ```java //knowing the available fonts import java.awt.*; class Fonts { public static void main(String args[]) { //get the local graphics environment information into //GraphicsEnvironment object ge GraphicsEnvironment ge = GraphicsEnvironment.getLocal GraphicsEnvironment(); //From ge, get available font family names into fonts [] String fonts[] = ge.getAvailableFontFamilyNames(); System.out.println("Available fonts on this system: "); //retreive one by one the font names from fonts [] and display for(int i=0; i<fonts.length; i++) System.out.println(fonts[i]); } } ``` ## Displaying Images in the Frame We can display images like .gif and .jpg files in the frame. For this purpose, we should follow these steps: - **Load the image into Image class object using getImage() method of Toolkit class.** ```java Image img = Toolkit.getDefaultToolkit().getImage("diamonds.gif"); ``` Here, Toolkit.getDefaultToolkit () method creates a default Toolkit class object. Using this object, we call getImage() method and this method loads the image diamonds.gif into img object. But, loading the image into img will take some time. JVM uses a separate thread to load the image into img and continues with the rest of the program. So, there is a possibility of completing the program execution before the image is completely loaded into img. In this case, a blank frame without any image will be displayed. To avoid this, we should make JVM wait till the image is completely loaded into img object. For this purpose, we need MediaTracker class. Add the image to MediaTracker class and allot an identification number to it starting from 0,1,... ```java MediaTracker track = new MediaTracker(this); track.addImage(img, 0); //0 is the id number of image ``` Now, MediaTracker keeps JVM waiting till the image is loaded completely. This is done using waitForID() method. ```java track.waitForID(0); ``` This means wait till the image with the id number 0 is loaded into img object. Similarly, if several images are there, we can use waitForID (1), waitForID (2), etc., - **Once the image is loaded and available in img, then we can display the image using drawImage() method of Graphics class, as:** ```java g.drawImage(img, 50,50,null); ``` Here, img is the image that is displayed at (50,50) coordinates and 'null' represents ImageObserver class object which is not required. ImageObserver is useful to store history of how the image is loaded into the object. Since, this is not required, we can use just null in its place. Another alternative for drawImage() is: ```java g.drawImage(img,50,50, 200, 2