Java Swing and AWT: Chapter 1 PDF PDF
Document Details
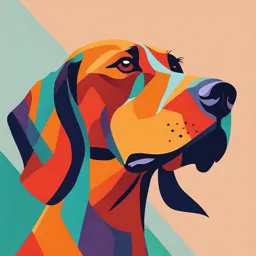
Uploaded by FastForgetMeNot7181
Haramaya University
2025
Tags
Summary
This document is Chapter 1 on Java Swing and AWT from Haramaya University. This chapter discusses key concepts in graphical user interface GUI programming, including components, and layout managers. The document also includes descriptions of AWT and Swing.
Full Transcript
Haramaya University College of Computing and Informatics Department of Software Engineering Advanced Programming January 2025 Chapter One AWT & Swing Advanced programming Chapter 1 2 What is GUI(graphical user interface)? A graphical user interface (GUI)...
Haramaya University College of Computing and Informatics Department of Software Engineering Advanced Programming January 2025 Chapter One AWT & Swing Advanced programming Chapter 1 2 What is GUI(graphical user interface)? A graphical user interface (GUI) presents a user-friendly mechanism for interacting with an application. GUIs are built from GUI components. These are sometimes called controls or widgets. A GUI component is an object with which the user interacts via the mouse, the keyboard or another form of input, such as voice recognition. Advanced programming Chapter 1 3 Java Swing and AWT Java provides two sets of components for GUI programming: ▪ AWT: classes in the java.awt package ▪ Swing: classes in the javax.swing package Advanced programming Chapter 1 4 Abstract Windowing Toolkit (AWT): Sun's initial effort to create a set of cross-platform GUI classes. (JDK 1.0 - 1.1) Maps general Java code to each operating system's real GUI system. Problems AWT components depend on native code counterparts to handle their functionality. Thus, these components are often called heavyweight components. Advanced programming Chapter 1 5 AWT cont... Component: A GUI widget that resides in a window. Also called controls in many other languages. Awt supports the following components: Labels Buttons Check boxes Choice lists Lists Scroll bars Text Editing Advanced programming Chapter 1 6 Swing ▪ Swing implements GUI components that build on AWT technology. ▪ Versions (JDK 1.2+) ▪ Swing is implemented entirely in Java. ▪ Swing components do not depend on operating system’s native code to handle their functionality. Thus, these components are often called lightweight components. ▪ Add J prefix to awt component ▪ Eg. JButton, Jlist, Jcheckbox…etc. Advanced programming Chapter 1 7 Pros Portability: Pure Java implementation. Features: Not limited by native components. Look and Feel: Pluggable look and feel. Swing: Pros Components automatically have the look and feel of the OS their running on. and Cons Cons Performance: Swing components handle their own painting (instead of using APIs like DirectX on Windows). Look and Feel: May look slightly different than native components. Advanced programming Chapter 1 8 Pros Speed: native components speed performance. AWT: Pros Look and feel: AWT components more closely reflect the look and feel of the and Cons OS they run on. Cons Portability: use of native peers(OS component) creates platform specific limitations. Advanced programming Chapter 1 9 Components (Swing) Advanced programming Chapter 1 10 Components cont.. All user interface elements Component is an abstract that are displayed on the class that encapsulates all screen and that of the attributes of a visual interact with the user are component. subclasses of Component. Advanced programming Chapter 1 11 Containers Is a subclass of Component(It is Other Container objects can be A container is responsible for Two Containers also an instance of a subclass of stored inside of a Container. laying out any components that java.awt.Container). it contains. Window Frame dialog panel Advanced programming Chapter 1 12 java.lang.Object java.awt.Component Containers cont.. java.awt.Container javax.swing.JComponent java.awt.Window javax.swing.JPanel javax.swing.JFileChooser java.awt.Frame javax.swing.JPopupMenu javax.swing.JToolbar javax.swing.JFrame javax.swing.JLabel Advanced programming Chapter 1 13 Every container has a LayoutManager that determines how different components are positioned within the container. Containers cont.. Components are positioned inside the container according to a LayoutManager. Advanced programming Chapter 1 14 Containers cont.. The AWT defines Panels are Windows are two different kinds subclasses of subclasses of of containers, java.awt.Panel. java.awt.Window. A panel is A window is a contained inside Panels and free-standing, another native window. container. Panels do not Windows. stand on their own. Advanced programming Chapter 1 15 Containers cont.. A frame is an instance There are two kinds of A dialog is a subclass of of a subclass of windows: java.awt.Dialog. java.awt.Frame. Frames and It represents a A dialog is a transitory Dialogs. normal, native window that exists window. merely to impart some information or get some input from the user. Advanced programming Chapter 1 16 Panel A PANEL IS A FAIRLY GENERIC SINCE EACH PANEL CAN HAVE ITS OWN PANEL IS A WINDOW THAT DOES NOT OTHER COMPONENTS CAN BE ADDED TO CONTAINER WHOSE PRIMARY PURPOSE LAYOUTMANAGER, YOU CAN DO MANY CONTAIN A TITLE BAR, MENU BAR, OR A PANEL OBJECT BY ITS ADD( ) METHOD IS TO SUBDIVIDE THE DRAWING AREA THINGS WITH PANELS THAT YOU CAN'T BORDER. (INHERITED FROM CONTAINER). INTO SEPARATE RECTANGULAR PIECES. DO WITH A SINGLE LAYOUTMANAGER. Advanced programming Chapter 1 17 Panel cont.. If you want to add new Panel rather than the default one, use this syntax: removeAll(); // to remove all remove(controlObj); // for controls from the current Panel panelName = new Panel(); single controls panels. then add any control you need If you want to remove controls to it using add() method: for panel: finally add this panel to the panelName.add(controlObj); default one by using add() method. i.e add(panelName); Advanced programming Chapter 1 18 Panel Example.. public class FrameDemo extends Frame{ public FrameDemo(){ Panel panel=new Panel();// creating new panel panel.setLayout(new FlowLayout()); panel.setBackground(Color.red); panel.add(new TextField(12));// adding comp to created panel panel.add(new Button("Ok")); this.add(panel); //adding created panel to default panel }} Advanced programming Chapter 1 19 Windows The java.awt.Window class and its subclasses let you create free- standing windows. That is, a Window is not itself contained. A Window is a subclass of java.awt.Container that is independent of other containers. Since Window extends java.awt.Container you can add components like Buttons and TextFields to Windows. Advanced programming Chapter 1 20 Windows ‒ you Don't use the Window class directly. Instead you use one of its subclasses, either java.awt.Frame or java.awt.Dialog depending on your need. ‒ A Frame is what most people think of as a window in their native environment. It can have a menu bar, titles, borders and resizing corners. Advanced programming Chapter 1 21 ‒ A Dialog will not have a menu bar. ▪ It can be moved. ▪ Its purpose is to get some particular information from the user (input) or to impart some particularly Windows important information to the cont.. user (output). ▪ It is normally visible on the screen only until it gets the input or receives acknowledgement from the user about its output. Advanced programming Chapter 1 22 Windows cont.. Frames are very useful Frame f = new in more complex Frame("My Window"); applications. Frame f = new Frame(); Frames let you separate More commonly you'll want different functions or to name the frame so pass data into different the constructor a string that windows. specifies the window's title. Everything you needAdvanced to programming Chapter 1 To create a new frame 23 create and work with Windows cont.. Unlike panels and applets, the However you can change this default LayoutManager for a using the frame's setLayout() frame is BorderLayout, not method like this: FlowLayout. f.setLayout(new FlowLayout()); Frames inherit from java.awt.Component so they have paint() and update() methods. If you want to draw in the frame and process events manually, just create a subclass of Frame and add listener objects to it. Advanced programming Chapter 1 24 Frame cont.. f.add(new this.add(new Button("OK")); Button(“Ok”)); If you're calling add() Since the default To add a component to from one of the layout for a frame is Frame subclass's BorderLayout, you a frame call the frame's own methods you should specify add() method. won't need to do whether you want this. the component added to the North, South, East, West or Center. Advanced programming Chapter 1 25 Frame cont.. Here's how you'd add a centered label to the center of Frame f: f.add("Center", new Label("frame")); or f.add(new Label(“frame”),BorderLayout.CENTER); You move a Frame with the setLocation(int x, int y) method. The size and position of any However x and y are relative to the given frame is unpredictable screen unless you specify it. Advanced programming Chapter 1 26 Specifying the size is easy. Just When you're done adding components, resizing and moving the Frame, make it visible by calling its show() method like so: Frame cont.. Frame f=new Frame(“this is frame title”); or f.setVisible(true); f.show(); //since it inherits from java.awt.Component Advanced programming Chapter 1 27 Frame Example import java.awt.*; public class FrameTester { public static void main(String[] args) { Frame myFrame = new Frame("My AWT Frame"); myFrame.setSize(250, 250); myFrame.setLocation(300,200); myFrame.add("Center", new Label(“Hello awt”)); myFrame.show(); }} This window cannot be closed because it does not yet respond to the WINDOW_CLOSING event. Advanced programming Chapter 1 28 import javax.swing.*; public class HelloWorldSwing { public static void main(String[] args) { JFrame frame = new JFrame("HelloWorldSwing"); final JLabel label = new JLabel("Hello World"); frame.getContentPane().add(label); frame.setDefaultCloseOperation( JFrame.EXIT_ON_CLOSE); frame.pack(); frame.setVisible(true); } } Frame ‒ JFrames (and Swing containers in general) differ from AWT frames (and AWT containers in general) in that you must add Example2 component's to the JFrame's content pane rather than directly to the frame itself. Furthermore JFrames are closeable by default whereas AWT frames are not. Advanced programming Chapter 1 29 import java.awt.*; public class CenteredFrameTester { public static void main(String[] args) { Frame myFrame = new Frame("My Frame"); myFrame.setSize(250, 250); Centering a Toolkit kit = myFrame.getToolkit(); Dimension screenSize = kit.getScreenSize(); frame on int screenWidth = screenSize.width; int screenHeight = screenSize.height; screen Dimension windowSize = myFrame.getSize(); int windowWidth = windowSize.width; int windowHeight = windowSize.height; int upperLeftX = (screenWidth - windowWidth)/2; int upperLeftY = (screenHeight - windowHeight)/2; myFrame.setLocation(upperLeftX, upperLeftY); myFrame.show(); } } Advanced programming Chapter 1 30 Main Steps in GUI Programming To make any graphic program work we must be able to create windows and add content to them. To make this happen we must: Fill the container Import the awt or Set up a top-level Install listeners for Display the with GUI swing packages. container. GUI Components. container. components. Advanced programming Chapter 1 31 Dialogs ‒ Dialogs are more transitory. They're used for simple user input or for quick alerts to the user. ‒ java.awt.Dialog is a subclass of java.awt.Window and hence of java.awt.Container and java.awt.Component. ‒ Therefore a lot of what you learned about frames applies to dialogs as well. You move them, resize them and add to them almost exactly as you do for frames. ‒ There are two differences between dialogs and frames: ▪ A frame can have a menu bar. A dialog cannot. ▪ A dialog can be modal. A frame cannot. Advanced programming Chapter 1 32 ‒ A modal dialog blocks all other use of the application until the user responds to it. ‒ A modal dialog cannot be moved and does not allow the user to switch to another window in the same program. Dialogs ▪ On some platforms the user may not even be able to switch to another program cont.. ‒ Non-modal dialogs pop-up but they don't prevent the user from doing other things while they're visible. ‒ Because modal dialogs inconvenience users by forcing them to respond when the computer wants them to rather than when they want to, their use should be kept to a minimum. Advanced programming Chapter 1 33 Dialogs cont.. Dialog d = new Dialog(new Frame(), false); The second argument is a There are two boolean which specifies The only methods that are whether or not the dialog constructors for Dialog significantly different between a should be modal. which differ in whether Modal dialogs are only modal dialog and a frame are the or not the dialog is relative to their parent frame, constructors. given a title. which is passed as the first argument to the constructor. That is they block input to the parent frame but not to other frames. Advanced programming Chapter 1 34 ‒ You can make a dialog resizable and movable by calling its setResizable() method with a boolean value of true like this: d.setResizable(true); Dialogs ‒ You can give a dialog a title bar by adding the title cont.. string to the constructor: Dialog d = new Dialog(new Frame(), "My Dialog Window", false); ‒ All the other methods of the Dialog class are exactly the same as they are for Frames. Advanced programming Chapter 1 35 import java.awt.*; public class DialogDemo { public DialogDemo(){ Dialog d=new Dialog(new Frame(),"Title",false); Dialog d.setSize(200,400); d.add("South",new Button("Click Here")); Example d.add("North",new TextField(23)); d.pack(); d.show(); } public static void main(String arg[]){ new DialogDemo(); }} Advanced programming Chapter 1 36 Create your own Dialogs For example one of the simpler common dialogs is a create your own dialog by notification dialog that gives the extending it to Dialog class and user a message to which they instantiate that subclass from can say OK to signify that the main program. they've read it. The next slide describes Dialog subclass. Advanced programming Chapter 1 37 Dialog create yoimport java.awt.*; public void import java.awt.event.*; actionPerformed(ActionEvent e) { public class DialogDemo extends Dialog implements subclass ActionListener this.setVisible(false); { this.dispose(); public DialogDemo(Frame parent,String message){ Example super(parent,message); Panel panel=new Panel(); Button ok=new Button("Ok"); } } ok.addActionListener(this); class DialogCheck { panel.setLayout(new FlowLayout()); public static void main(String s[]){ panel.add("Center",new Label("This is to Notify User DialogDemo demo=new using Dialog")); DialogDemo(new panel.add("South",ok); Frame(),"Notification Dialog Box"); this.add(panel); this.pack(); demo.show(); this.setSize(200,100); } this.setLocation(100,100); } this.show(); } Advanced programming Chapter 1 38 Layout Manager All of the components that we have shown so far have been positioned by the default layout manager. First, it is very tedious to manually layout a large number of components. It is possible to lay out Java controls by hand, too, you generally won’t want to, for two main reasons. Second, sometimes the width and height information is not yet available when you need to arrange some control, because the native toolkit components haven’t been realized. Advanced programming Chapter 1 39 ‒ A layout manager is an instance of any class that implements the Layout Manager interface. The layout manager is set by the Layout setLayout( )method. ‒ If no call to setLayout( )is made, then the Manager cont.. default layout manager is used. Theset Layout( )method has the following general form: void setLayout(LayoutManagerlayoutObj) ‒ If you wish to disable the layout manager and position components manually, pass null for layoutObj. Advanced programming Chapter 1 40 Layout Manager Type ‒ Flow Layout ‒ Grid Layout ‒ Border Layout ‒ GridBag Layout ‒ Card Layout ‒ Box Layout Advanced programming Chapter 1 41 Flow Layout ‒ FlowLayout is the default layout manager for panels. ‒ FlowLayout implements a simple layout style, which is similar to how words flow in a text editor, which by default is left to right, top to bottom. Therefore by default, components are laid out line-by-line beginning at the upper-left corner. ‒ In all cases, when a line is filled, layout advances to the next line. Advanced programming Chapter 1 42 Flow Layout Here are the constructors for FlowLayout: FlowLayout( ) FlowLayout(int how) FlowLayout(int how, int horz, int vert) Instantiate it this way FlowLayout() flow=new FlowLayout(); flow.setAlignment(FlowLayout.CENTER);// flow.setHgap(5); // to set horizontal space flow.setVgap(10); // to set vertical space in place of center you can also use between controls between controls RIGHT,LEFT,LEADING AND TRAILING Advanced programming Chapter 1 43 ‒ FlowLayout(int how) use it this way FlowLayout flow=new FlowLayout(FlowLayout.LEFT); // for Flow Layout FlowLayout.LEFT you can use 0 for left,1 for center 2 for right ‒ FlowLayout(int how,int hor, int ver) use it this way FlowLayout flow=new FlowLayout(1,5,10); In all case after you declare and intialize it provide it as argument to setLayout() method. i.e: setLayout(flow); Advanced programming Chapter 1 44 public BorderLayout() ‒ Divides container into five regions: ▪ NORTH and SOUTH regions expand to fill region horizontally, and use the component's preferred size vertically. Border Layout ▪ WEST and EAST regions expand to fill region vertically, and use the component's preferred size horizontally. ▪ CENTER uses all space not occupied by others. myFrame.setLayout(new BorderLayout()); myFrame.add(new JButton("Button 1"), BorderLayout.NORTH); ▪ This is the default layout for a JFrame. Advanced programming Chapter 1 45 ‒ GridLayoutlays out components in a two-dimensional grid. When you instantiate a GridLayout, you define the number of rows and columns. Grid Layout ‒ The constructors supported by GridLayout are shown here: ▪ GridLayout( ) Use it as GridLayout grid=new GridLayout(); grid.setRows(3); grid.setColumns(3); grid.setHgap(2); grid.setVgap(3); Advanced programming Chapter 1 46 Grid Layout cont.. GridLayout(intnumRows, intnumColumns) Use it as GridLayout grid=new GridLayout(3,3); GridLayout(intnumRows, intnumColumns, inthorz, intvert) Use it as GridLayout(3,3,10,5); Finally add to SetLayout, using setLayout(grid); Remember if want to add Layout to layout manager you can do it as: setLayout(new GridLayout(2,3,2,4)); // the same for flow and border layout Advanced programming Chapter 1 47 Card Layout The CardLayout class is unique among the other This can be useful for user interfaces with optional layout managers in that it stores several different components that can be dynamically enabled and layouts. Each layout can be thought of as being on disabled upon user input. CardLayout provides a separate index card in a deck that can be these two constructors: shuffled so that any card is on top at a given time. CardLayout( ) CardLayout(int horz, int vert) Advanced programming Chapter 1 48 Card Layout cont.. 1 2 3 Use of a card layout requires a This panel must have Thus, you must create a panel bit more work than the other CardLayout selected as its that contains the deck and a layouts. The cards are typically layout manager. The cards that panel for each card in the deck. held in an object of type Panel. form the deck are also typically Next, you add to the objects of type Panel. appropriate panel the components that form each card. Finally, you add this panel to the window. Advanced programming Chapter 1 49 Card Layout cont.. ‒ When card panels are added to a panel, they are usually given a name. Thus, most of the time, you will use this form of add( ) when adding cards to a panel: ▪ void add(ComponentpanelObj, Objectname) Eg. Panel p=new Panel(); CardLayout c=new CardLayout(5,10); p.setLayout(c); Panel p1=new Panel(); Panel p2=new Panel(); p.add(p1,”Panel 1”); p.add(p2,”Panel 2”); add(p); Advanced programming Chapter 1 50 ‒ After you have created a deck, your program activates a card by calling one of the following methods defined by Card Layout CardLayout: ▪ void first(Container deck) cont.. ▪ void last(Container deck) ▪ void next(Container deck) ▪ void previous(Container deck) ▪ void show(Container deck, String cardName) Advanced programming Chapter 1 51 Card Layout cont.. To show the last card, call last(). The Here deck is a reference to the same for previous and next card. Both container (usually a panel) that holds next( )and previous( )automatically the cards, and cardName is the name cycle back to the top or bottom of the of a card. Calling first( )causes the first deck, respectively. The show( card in the deck to be shown. )method displays the card whose name is passed in cardName. Advanced programming Chapter 1 52 Components Advanced programming Chapter 1 53 A component is an object having a graphical representation that can be displayed on the screen. like checkboxes, menus, windows, buttons, text fields, applets, and more. A container is a special component that can hold other components. Example of containers in typical GUI applications include: Components panels, windows, applets, frames Functionality of most GUI components derive from the Component and Container classes. Advanced programming Chapter 1 54 Component Elements Advanced programming Chapter 1 55 Label is the easiest control which is used to display string on screen. A label is an object of type Label. Labels are passive controls that do not support any interaction with the user(i.e It do not support any event handler). Label defines the following constructors: Label( ) JLabel Label(Stringstr) Label(Stringstr, inthow) Advanced programming Chapter 1 56 The first version creates a blank label. The second version creates a label that contains the string specified by str. This string is left-justified. The third version creates a label that contains the string specified by str using the alignment specified by JLabel cont.. how. The value of how must be one of these three constants: Label.LEFT, Label.RIGHT, or Label.CENTER. E.g. Label labelObj=new Label(“this is Label Demo”,Label.RIGHT); Advanced programming Chapter 1 57 We can set or change the text in a label by using the setText( ) method. We can obtain the void setText(String str) JLabel cont.. current label by calling getText( ). String getText( ) You can set the alignment of the string within the label by calling setAlignment( ).To obtain the current alignment, call getAlignment( ). Advanced programming Chapter 1 58 ‒ The most widely used control is button. button is a component that contains a label and that generates an event when it is pressed. Buttons are objects of type Button. Button defines two constructors: ▪ Button( ) ▪ Button(String str) JButton ▪ Advanced programming Chapter 1 59 For Button() use it this way: Button buttonObj=new Button(); buttonObj.setLabel(“This is button Demo”); // setLabel() method JButton cont.. For Button(String str) use it this Way Button buttonObj=new Button(“this is button Demo”); Advanced programming Chapter 1 60 JButton import java.awt.*; import javax.swing.*; Example public class GuiExample1 { public static void main(String[] args) { JFrame frame = new JFrame(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(new Dimension(300, 100)); frame.setTitle("A frame"); JButton button1 = new JButton(); button1.setText("Click me!"); button1.setBackground(Color.RED); frame.add(button1); frame.setVisible(true); } } ▪ Advanced programming Chapter 1 61 Handling Buttons JButton cont.. ‒ Each time a button is pressed, an action event is generated. This is sent to any listeners that previously registered an interest in receiving action event notifications from that component. ‒ Each listener implements the ActionListener interface. That interface defines the actionPerformed( )method, which is called when an event occurs. ‒ An ActionEvent object is supplied as the argument to this method. It contains both a reference to the button that generated the event and a reference to the action command string associated with the button. Advanced programming Chapter 1 62 ‒ You can Add ActionListener to Button using two ways: // the first way By Implementing the ActionListener Interface button =new Button(“Demo”); add(button); button.addActionListener(this); // do some actions Public void actionPerformed(ActionEvent a){ JButton cont.. If(a.getSource()==button){ / / what ever you want here } Advanced programming Chapter 1 63 ‒ You can Add ActionListener to Button using the following ways as well. Import java.awt.event.ActionListener; // import other class here us you program Public class ButtonDemo extends Applet { Button button; //other declaration here; Public void init(){ button =new Button(“Demo”); add(button); button.addActionListener(new ActionListener(){ Public void actionPerformed(ActionEvent a){ JButton cont.. If(a.getSource()==button){ // what ever you want here } Or if(a.getActionCommand().equals(“Demo”)){ }}}); // }} Advanced programming Chapter 1 64 JButton cont.. The label of a button is obtained by calling The Object of button is obtained by calling So to get Label of Button use this method. the getActionCommand( )method on the the getSource() method on the actionevent ActionEvent object passed to object passed to actionPerformed() actionPerformed( ). method. a.getActionCommand().equals(“button”); // to get Object of button a.getSource()==button. // depending on the previous example Advanced programming Chapter 1 65 TextField The TextField class implements a single-line text-entry area, usually called an edit control. Text fields allow the user to enter strings and to edit the text using the arrow keys, cut and paste keys, and mouse selections. TextField is a subclass of TextComponent. TextField defines the following constructors: TextField( ) TextField(intnumChars) TextField(Stringstr) TextField(Stringstr, intnumChars) Advanced programming Chapter 1 66 ▪ TextField() use it this way: TextField field=new TextField(); field.setColumns(15);// to limit number of character to be displayed field.setText(“Initial value of textfield”); TextField Advanced programming Chapter 1 67 TextField TextField(int numChar) use it this way TextField field=new TextField(15); field.setText(“Initial value of textfield”); TextField(String str) use it this TextFeild field=new TextField(“initial value of textfield”); way TextField(String str,int numChar) use this way field.setEditable(true);// Editable textField, if false not editable TextField field=new field.setEchoChar(‘@’); // to hide plain character of fields TextField(“initail value of field.select(2,6);// to select text start for index 2 upto index 6 textfield”, 15); field.getSelectedText(); // capture the selected text from the field Advanced programming Chapter 1 68 Handling Events Every time the user types a character or pushes a mouse button, an event occurs. Any object can be notified of the event. All the object has to implement the appropriate interface and be registered as an event listener on the appropriate event source. Advanced programming Chapter 1 69 ‒ Every event handler requires three pieces of code: 1. declaration of the event handler class that implements a listener interface or extends a class that implements a listener interface public class MyClass implements ActionListener { ……} How to 2. registration of an instance of the event handler class as a listener Implement an 3. someComponent.addActionListener(instan ceOfMyClass); providing code that implements the methods in Event Handler the listener interface in the event handler class public void actionPerformed(ActionEvent e) {...//code that reacts to the action... } Advanced programming Chapter 1 70 How to Implement an Event Handler 1 public class ButtonClickExample extends JFrame implements ActionListener { JButton b = new JButton("Click me!"); public ButtonClickExample() { b.addActionListener(this); 2 getContentPane().add(b); pack(); setVisible(true); } public void actionPerformed(ActionEvent e) { 3 b.setBackground(Color.CYAN); } public static void main(String[] args) { Advanced programming Chapter 1 71 ‒ Since text fields perform their own editing Text Field functions, your program generally will not respond to individual key events that Event occur within a text field. However, you may want to respond when the user Handler presses ENTER key. It use ActionListener event handler Advanced programming Chapter 1 72 ‒ Sometimes a single line of text input is not enough for a given task. To handle these situations, the AWT includes a simple multiline editor called TextArea. Following are the constructors for TextArea: ▪ TextArea( ) ▪ TextArea(int numLines, int numChars) // no of columns and char to be displayed ▪ TextArea(String str) // Initial value ▪ TextArea(String str, intnumLines, int numChars) Text Area ▪ TextArea(String str, intnumLines, int numChars, int sBars) // int sBars type of Scrollbar, eg Scrollbar.VERTICAL,.HORIZONTAL,.VE RTIVAL_ONLY and the like Advanced programming Chapter 1 73 ‒ Most of the methods are similar to TextField but there is some new feature for TextArea only. void append(String str) void insert(String str, int index) void replaceRange(String str, int startIndex, int endIndex) area.append(“this value”) // it add at the end index of the area but setText() method replace the existing Text Area cont.. value on the area. area.insert(3,6); // this insert new string starting from index 3 to index 6 area.replaceRange(“Replace”,3,12); // replace the existing text from 3 to 12 index Advanced programming Chapter 1 74 Checkbox A checkbox is a control that is used to turn an option on or off. It consists of a small box that can either contain a check mark or not. There is a label associated with each check box that describes what option the box represents. You change the state of a check box by clicking on it. Check boxes can be used individually or as part of a group. Check boxes are objects of the Checkbox class. Checkbox(String str, boolean on, CheckboxGroup cbGroup) Checkbox( ) Checkbox(String str) Checkbox(String str, boolean on) ,Checkbox(String str, CheckboxGroup cbGroup, boolean on) Advanced programming Chapter 1 75 Checkbox cont.. Checkbox() use it this way Checkbox check=new Checkbox(); check.setLabel(“Default”); // to set Label of checkbox check.setCheckboxGroup(cbGroupName); check.setState(true); // checkbox created with checked state Checkbox(String str) use it this way Checkbox check=new Checkbox(“LabelofCheckbox”); // with label Checkbox (String str,boolean on) use it this way Checkbox check=new Checkbox(“Label”,true);// the state is on Checkbox(String str,boolean on, CheckboxGroup cbGroup) use it this way Checkbox check=new Checkbox(“label”,false,cbGroup);// if checkboxGroup is their unless use null on the place of cbGroup Advanced programming Chapter 1 76 Event handler for Checkbox Each time a check box is selected or deselected, an item event is generated. We can add Item event to checkbox by implementing the ItemListener interface. That interface defines the itemStateChanged( )method, with argument ItemEvent object. You can apply this ItemListener in similar way for actionListener. To execute any activities depending on checkbox state use getState() method. Like below: Inside public void //what ever you want to execute If(check.getState()==true/false){ } } itemStateChanged(ItemEvent e) { here Advanced programming Chapter 1 77 CheckboxGroup It is possible to create a set of mutually exclusive check boxes in which one and only one check box in the group CheckboxGroup cbGroup=new CheckboxGroup(); can be checked at any one t ime. These check boxes are often called radio buttons. How to use Check boxGroup Check box check=new Checkbox(“label”,cbGroup,true); You can det ermine which check box in a group i s currently selected by cal ling getSel ectedCheck box(). You can set a Checkbox check1=new Checkbox(“l abel”,cbGroup,true); check box by cal ling setSelect edCheckbox(). So depending on the above: if(cbGroup.getSelectedCheckbox().getLabel()==check){ // operation t o be execut ed here} Advanced programming Chapter 1 78 Choice Control The Choice class is used to create a pop-up list of items from which the user may choose. Thus, a Choice control is a form of menu. When inactive, a Choice component takes up only enough space to show the currently selected item. When the user clicks on it, the whole list of choices pops up, and new selection can be made. Create Choice control using the default constructor i.e Choice(). Choice combobox=new Choice(); To add item to it use add(String name) method; combobox.add(“first”); combobox.add(“second”); To determine which item is currently selected, you may call either getSelectedItem() or getSelectedIndex(). The getSelectedItem( )method returns a string containing the name of the item. getSelectedIndex( ) returns the index of the i tem. Advanced programming Chapter 1 79 Choice cont… int getItemCount( ) // return number of item found in choice void select(int index) // select item whose index is supplied void select(String name) // select item whose label is supplied String getItem(int index) // capture item whose index is provided Based on the previous slide combobox.getItemCount(); // return 2 combobox.selet(1); // second selected Advanced programming Chapter 1 80 Use same Event handler as Checkbox.i.e itemListener interface To execute anything depending on the current selected item of choice inside itemStateChanged() method or inside other applet method use this statements Event If(combobox.getSelectedItem()==first){ // what ever here} handler for Choice Or if(combobox.getSelectedIndex()==1){ // what ever here } Or if (combobox.getItem(combobox.getSelectedIndex())==second){ // what ever here } Advanced programming Chapter 1 81 The List class provides a compact, multiple-choice, scrolling selection list. Unlike the Choice object, which shows only the single selected item in the menu, a List object can be constructed to show any number of choices in the visible window. List provides these constructors: List(int numRows, boolean List(int numRows) // limit List( ) multipleSelect) // decide item to be displayed single or multi selection To add item to List use add(string str) or add(string str, int index) methods. List you can determine which item is currently selected by calling either getSelectedItem( )or getSelectedIndex( ) for single selection. Advanced programming Chapter 1 82 you can determine which item is currently selected by calling either getSelectedItems( )or getSelectedIndexes( ) for multiple selection. List cont… To process list events, you will need to implement the Event Handling in List ActionListener interface. Each time a List item is double-clicked, an ActionEvent object is generated. Advanced programming Chapter 1 83 To respond to an event, a component receives, you register an event listener for the event type with the component. EventListeners The AWT defines eleven sub-interfaces of java.util.EventListener. java.awt.event.ComponentListener Event listeners are java.awt.event.ContainerListener java.awt.event.FocusListener objects which java.awt.event.KeyListener java.awt.event.MouseListener implement a java.awt.event.MouseMotionListener java.util.EventListener java.awt.event.WindowListener java.awt.event.ActionListener interface. java.awt.event.AdjustmentListener java.awt.event.ItemListener java.awt.event.TextListener Advanced programming Chapter 1 84 Mouse Events A java.awt.event.MouseEvent is sent to a component when the mouse state changes over the component. There are seven types of mouse events, each represented by an integer constant: MouseEvent.MOUSE_DRAGGED: MouseEvent.MOUSE_PRESSED: MouseEvent.MOUSE_CLICKED: A MouseEvent.MOUSE_ENTERED: MouseEvent.MOUSE_EXITED: MouseEvent.MOUSE_MOVED: MouseEvent.MOUSE_RELEASED: The mouse was moved over the The mouse button was pressed mouse button was pressed, then component while a mouse The cursor entered the The cursor left the component's The mouse moved in the (but not released) over the The mouse button was released released component's space space component's space over the component. button was held down component Advanced programming Chapter 1 85 Mouse Events A java.awt.event.MouseEvent is sent to a component when the mouse state changes over the component. There are seven types of mouse events, each represented by an integer constant: MouseEvent.MOUSE_CLICKED: A mouse button was pressed, then released MouseEvent.MOUSE_DRAGGED: The mouse was moved over the component while a mouse button was held down MouseEvent.MOUSE_ENTERED: The cursor entered the component's space MouseEvent.MOUSE_EXITED: The cursor left the component's space MouseEvent.MOUSE_MOVED: The mouse moved in the component's space MouseEvent.MOUSE_PRESSED: The mouse button was pressed (but not released) over the component MouseEvent.MOUSE_RELEASED: The mouse button was released over the component. Advanced programming Chapter 1 86