Programming 1 - Introduction to Programming PDF
Document Details
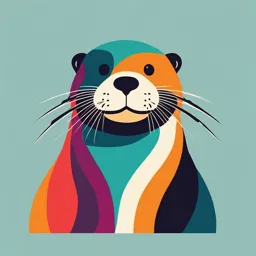
Uploaded by EntrancedMagnolia1583
Dr/ Mahmoud Gamal
Tags
Related
- Introduction to Java Programming and Data Structures (2019) by Y. Daniel Liang - PDF
- Computer Programming Concepts PDF
- Class of 2025 CS Notes PDF
- A Concise Introduction to Programming Algorithms in Java PDF
- GC 101 Programming Essentials - Java Programming Questions PDF
- COS 101: First Class Summary - Computer Science PDF
Summary
These lecture notes are an introduction to programming concepts. They cover topics such as what programming is, the steps in the program development life cycle, the basics of programming language, and give important examples, including some examples using the Java programming language.
Full Transcript
Programming 1 Chapter 1: Introduction to Programming Dr/ Mahmoud Gamal 1 Course Outlines: Chapter 1: Introduction to Programming. Chapter 2: Introduction to Java Programming. Chapter 3: Controlling Program flow. Chapter 4: Methods. Chapter 5: Ar...
Programming 1 Chapter 1: Introduction to Programming Dr/ Mahmoud Gamal 1 Course Outlines: Chapter 1: Introduction to Programming. Chapter 2: Introduction to Java Programming. Chapter 3: Controlling Program flow. Chapter 4: Methods. Chapter 5: Arrays. 2 What is Programming? Programming is a process of problem solving. Program Development Life Cycle? Step 1: Analyze the problem Understand the overall problem. Define problem requirements Does program require user interaction? ▪ If yes, what is the input? What is the expected output? Design steps (algorithm) to solve the problem. Algorithm: Step-by-step problem-solving process. 3 Step 2: Implement the algorithm Implement the algorithm in code. Verify that the algorithm works. Step 3: Maintenance Use and modify the program if the problem domain changes. Note: If the problem is complex, divide it into sub-problems. Analyze each sub-problem as above. 4 Example: Write a program to find the area of rectangle. area = length * width Input:…………..>> length, width Processing: …….>> area = length * width Output: ………..>> print out the area. 5 The Language of a computer: Machine Language: Language of a computer. Binary digit (bit): The digit 0 or 1 Binary code: A sequence of 0s and 1s 6 The Evolution of Programming Languages: Programming Language: It is a set of rules used to write a program. ❑ Low-Level Languages. Machine Language Assembly Language ❑ High-Level Languages. In order to write a program using a programming language you need: An application named Integrated Development Environment (IDE). The IDE helps you to write the program and correct the errors. An application to transform the program into machine language (Assembler or Compiler) 7 The Evolution of Programming Languages: 1- Machine Language (Low-level language): Early computers were programmed in machine language. Example: To calculate wages = rate* hours in machine language: 100100 010001 // Load 100110 010010 // Multiply 100010 010011 // Store 8 The Evolution of Programming Languages: 2- Assembly Language:(Low-level language): Assembly language instructions are mnemonic. Assembler: Translates a program written in assembly language into machine language. 9 Examples of instructions in Assembly Language and Machine Language: Assembly Language Machine Language LOAD 100100 STORE 100010 MULT 100110 ADD 100101 SUB 100011 10 Assembly Language-Low Level: Limitations: Each processor type has its own Assembly language. You must be fully aware of the processor design. Very detailed and needs long time and big effort to write. Advantages Programs written using it executes faster. 11 The Evolution of Programming Languages: 3- High Level Languages: Its commands are English like (e.g., print, input, copy) High-level languages include Basic, C, C++, C#, and Java. Compiler: Translates a program written in a high-level language into machine language Example: The equation wages = rate * hours can be written in Java as: wages = rate * hours; 12 The Problem Analysis - Coding – Execution Cycle: 13 Programming 1 Lecture 2 Chapter 2: Introduction to Java Programming Dr/ Mahmoud Gamal 1 A Java Program: Output: 2 A Java Program: Package: Group of classes. Class: 1. Data items 2. Methods ( or functions or operations) The main method is the entry point to the program. Java is case sensitive language. Console window: Text box into which the program's output is printed. 3 Structure of a Java program class: a program public class name { public static void main(String[] args) { Body of statement; statement; main method: a named group method... statement; of statements } } statement: a command to be executed Every executable Java program consists of a class, that contains a method named main, that contains the statements (commands) to be executed. System.out.println Statement: A statement that prints a line of output on the console. Two ways to use System.out.println : System.out.println("text"); Prints the given message as output. System.out.println(); Prints a blank line of output. 5 Example: public class Hello { public static void main(String[] args) { System.out.println("Hello, world!"); System.out.println(); System.out.println("This program produces"); System.out.println("four lines of output"); } } Output: Hello, world! This program produces four lines of output 6 Compile/run a program 1. Write it. code or source code: The set of instructions in a program. 2. Compile it. COMPILER javac: translates the program from Java to bytecode bytecode: runs on many computer types (any computer with JVM) 3. Run (execute) it. output: whatever the programmer instructs the program to do source code byte code output compile run Hello, World! javac Hello.java java Hello Identifier: A name given to an item in your program. Must start with a letter or _ or $ Do not use space or any special character. Do not use any reserved name like (void, class, int) Do not start with a number. legal: _myName TheCure ANSWER_IS_42 $bling$ illegal: me+u 49ers side-swipe Ph.D’s Example: public class MyClass { 8 Syntax: Syntax: The set of legal structures and commands that can be used in a particular language. Every basic Java statement ends with a semicolon ; The contents of a class or method occur between { and } 9 Programming Errors: 1- Syntax Errors: Detected by the compiler. 2- Runtime Errors: Causes the program to abort. 10 Programming Errors: 3- Logic Errors: Produces incorrect results. 11 Strings: string: A sequence of characters to be printed. Starts and ends with a " quote " character. The quotes do not appear in the output. Examples: "hello" "This is a string. It's very long!" Restrictions: May not span multiple lines. "This is not a legal String." May not contain a " character. "This is not a "legal" String either."12 Escape sequence: Escape Sequence: A special sequence of characters used to represent certain special characters in a string. \t tab character \n new line character \" quotation mark character \\ backslash character Example: System.out.println("\\hello\nhow\tare \"you\"?\\\\"); Output: \hello how are "you"?\\ 13 Questions What is the output of the following println statements? System.out.println("\ta\tb\tc"); System.out.println("\\\\"); System.out.println("'"); System.out.println("\"\"\""); System.out.println("C:\nin\the downward spiral"); Answer: a b c \\ ' """ C: in he downward spiral Questions Write a println statement to produce this output: / \ // \\ /// \\\ Answer: System.out.println("/ \\ // \\\\ /// \\\\\\"); Questions What println statements will generate this output? This program prints a quote from the Gettysburg Address. "Four score and seven years ago, our 'fore fathers' brought forth on this continent a new nation." Answer: System.out.println("This program prints a"); System.out.println("quote from the Gettysburg Address."); System.out.println(); System.out.println("\"Four score and seven years ago,"); System.out.println("our 'fore fathers' brought forth on"); System.out.println("this continent a new nation.\""); Questions What println statements will generate this output? A "quoted" String is 'much' better if you learn the rules of "escape sequences." Also, "" represents an empty String. Don't forget: use \" instead of " ! '' is not the same as “ Answer: System.out.println("A \"quoted\" String is"); System.out.println("'much' better if you learn"); System.out.println("the rules of \"escape sequences.\""); System.out.println(); System.out.println("Also, \"\" represents an empty String."); System.out.println("Don't forget: use \\\" instead of \" !"); System.out.println("'' is not the same as \""); Comments: Comment: A note written in source code by the programmer to describe or clarify the code. Comments are not executed when your program runs. Syntax: // comment text, on one line or, Examples: // This is a one-line comment. Using comments Where to place comments: at the top of each file (a "comment header") at the start of every method (seen later) to explain complex pieces of code Comments are useful for: Understanding larger, more complex programs. Multiple programmers working together, who must understand each other's code. Comments Example public class BaWitDaBa { public static void main(String[] args) { // first verse System.out.println("Bawitdaba"); System.out.println("da bang a dang diggy diggy"); System.out.println(); // second verse System.out.println("diggy said the boogy"); System.out.println("said up jump the boogy"); } } print vs println: println print Programming 1 Lecture 3 Chapter 2: Introduction to Java Programming Dr/ Mahmoud Gamal 1 Data Types: Type: A name for a category or set of data values that are related, as in type int in java, which used to represent integer values. Examples: integer, real number, string 2 BYTE --> SHORT Java Data Types: --> INT --> LONG 1 --> 2 --> 4 --> 8 3 Expressions: 4 Arithmetic Operator: 5 Integer division with / 6 Integer remainder with % 14 / 4 = 3.5 4 *.5 = 2 7 Precedence: 8 Precedence: Summary: 9 Precedence Examples: 10 Precedence Questions: 11 Real numbers ( Type double or float): 12 Real Number Example: 13 Mixing Types: 14 String Concatenation: 15 Variables: Receipt Example: 16 Variables: 17 Declaration: 18 Assignment: 19 Declaration/initialization: 20 Using Variables: 21 Assignment and algebra: 22 Assignment and Types: Division is done first 23 Compile Errors: 24 Printing a variable's Value: 25 Programming 1 Lecture 4 Chapter 2: Introduction to Java Programming Dr/ Mahmoud Gamal 1 Increment and decrement: 2 Modify-and-assign: 3 Increment- Decrement Pre increment - Post increment post pre Pre decrement - Post decrement Example: Example: Evaluate the expression: 7 Receipt Question: 8 Receipt Answer: 9 Summary of Variables: 10 Trace: 11 Type casting: 12 Examples: PARENTHESES ACTUALLY MATTER 13 Example: Example: All integers Casting ( change the data type) casting char data type char : A primitive data type representing single characters of text (e.g., 'a', 'b', '@', ' ', etc.). public static void main(String[] args) { char a = 's'; System.out.println ("student" + a); } Output: students char vs. int Each char is mapped to an integer value internally Called an ASCII value 'A' is 65 'B' is 66 ' ' is 32 'a' is 97 'b' is 98 '*' is 42 Mixing char and int causes automatic conversion to int. 'a' + 10 is 107, 'A' + 'A' is 130 To convert an int into the equivalent char, type-cast it. (char) ('a' + 2) is 'c' Example public static void main(String[] args) { Be Careful: int x = 1; char x = 67; //correct since it char letter1 = 'a'; takes the value as the ASCII char letter2 = (char) (letter1 + 4); code 67 System.out.println(letter2); int y = 67; char x = y; // incorrect since x = 'a' + 3; here it takes it as integer y System.out.println(x); } Output: e 100 19 Programming 1 Lecture 5 Chapter 2: Introduction to Java Programming Dr/ Mahmoud Gamal 1 أأ Accepting Input from the User (Scanner): To call Scanner: import java.util.Scanner; Java.util ……>> Package Scanner……>> Class To create object (Data + Methods) from class scanner: Scanner Name = new Scanner (System.in) System.in …..>> refer to accepting input from keyboard Example: Scanner input = new Scanner (System.in) 2 Scanner methods Method Description nextInt() reads an int from the user nextDouble() reads a double from the user nextFloat() reads a float from the user next() reads a one-word String from the user next().charAt(0) reads one char from the user Each method waits until the user presses Enter. The value typed by the user is returned. Example: System.out.print("How old are you? "); int age = input.nextInt(); System.out.println("You typed " + age); Scanner Example import java.util.Scanner; // so that I can use Scanner public class UserInputExample { public static void main(String[] args) { Scanner console = new Scanner(System.in); System.out.print("How old are you? "); int age = console.nextInt(); age 29 years 36 int years = 65 - age; System.out.println(years + " years to retirement!"); } } Console (user input underlined): How old are you? 29 36 years until retirement! Example: A program to calculate the sum of two numbers Output: 5 Example: A Program to calculate the area of rectangle. Output: 6 Example: A Program to calculate the net salary 7 Java's Math class Method name Description Math.abs(value) absolute value Math.ceil(value) rounds up Math.floor(value) rounds down Math.log10(value) logarithm, base 10 Math.max(value1, value2) larger of two values Math.min(value1, value2) smaller of two values Math.pow(base, exp) base to the exp power Math.random() random double between 0 and 1 Math.round(value) nearest whole number Math.sqrt(value) square root Math.sin(value) sine/cosine/tangent of Math.cos(value) an angle in radians Constant Description Math.tan(value) Math.E 2.7182818... Math.toDegrees(value) convert degrees to Math.PI 3.1415926... Math.toRadians(value) radians and back Calling Math methods Math.methodName(parameters) Examples: double squareRoot = Math.sqrt(121.0); System.out.println(squareRoot); // 11.0 int absoluteValue = Math.abs(-50); System.out.println(absoluteValue); // 50 System.out.println(Math.min(3, 7) + 2); // 5 double exp = Math.pow(2, 4); System.out.println(exp); // 16.0 The Math methods do not print to the console. Each method produces ("returns") a numeric result. The results are used as expressions (printed, stored, etc.). Example: 10 Remember: Type casting type cast: A conversion from one type to another. To promote an int into a double to get exact division from / To truncate a double from a real number to an integer Syntax: (type) expression Examples: double result = (double) 19 / 5; // 3.8 int result2 = (int) result; // 3 int x = (int) Math.pow(10, 3); // 1000 Calling Math methods Parameters send information in from the caller to the method. Return values send information out from a method to its caller. A call to the method can be used as part of an expression. -42 Math.abs(-42) 42 main 2.71 3 Math.round(2.71) Example: Java program to find the Hypotenuse of a right-angled triangle 13 Programming 1 Lecture 6 & 7 Chapter 3: Controlling Program Flow Dr/ Mahmoud Gamal 1 Relational Operators: A relational operator checks the relationship between two operands. If the relation is true, it returns true. If the relation is false, it returns value false. Relational operators are used in decision making and loops. 2 Relational Operators: Note: a = b (This will put the value of b in a) a ==b (This will check if a equals b or not) 3 Logical operators: An expression containing logical operator returns either true or false depending upon whether expression results true or false. Logical operators are commonly used in decision making. 4 Logical operators: Operator Meaning of operator Example && AND C = 5, d = 2 True only if all operands are ((c ==5)&& (d > 5) true Equals to false || OR C = 5, d = 2 True only if either one ((c ==5)|| (d > 5) operand is true Equals to true ! NOT C = 5, d = 2 True only if the operand is !(c ==5) false Equals to false 5 Example: Example Result (2 == 3) && (-1 < 5) false (2 == 3) || (-1 < 5) true !(2 == 3) true Evaluating logic expressions Relational operators cannot be "chained" as in algebra. 2 = 2.0) { System.out.println(“Application accepted"); } else { System.out.println("Application denied."); } 14 Syntax (c):(Nested If – else Statement) 15 Nested If – else Statement: Syntax (c): Example: if (x > 0) { System.out.println("Positive"); } else if (x < 0) { System.out.println("Negative"); } else { System.out.println("Zero"); } 16 Nested if structures exactly 1 path 0 or 1 path if (test) { if (test) { statement(s); statement(s); } else if (test) { } else if (test) { statement(s); statement(s); } else { } else if (test) { statement(s);} statement(s);} 0, 1, or many paths (independent tests) if (test) { statement(s); } if (test) { statement(s); } if (test) { statement(s); } Example : Write a program that accepts an integer number from the user, then checks and prints out whether it is an even or an odd number. 18 Output: 19 Example: Write a program that accepts an integer number from the user and, in case the number is positive, checks and prints out whether it is an even or odd number. 20 Output: 21 Example: Write a program that accepts two integer numbers from the user and compares them. 22 Output: 23 Example: Write a program that will print: Excellent for exam grades greater than or equal to 90, Verry Good for grades greater than or equal to 80, Good with grades greater than or equal to 70, Pass with grades greater than or equal to 60, and Fail for all other grades. 24 Answer: 25 Output: 26 Combining more than one condition Example: Write a program that accepts an integer number from the user and checks the number between 0 and 100. 27 Output: Note: If number of statements equal 1, we can remove curly braces { } 28 Switch Statement: It is used with multiple selections: Syntax: 29 Example: 30 Example: Write a program to enable the student to enter the grade’s letter to get the corresponding message as the following: A : Excellent B : Very Good C: Good F: Fail Other: Invalid grade 31 Answer: 32 Output: 33 Notes: Program without break 34 Output: 35 Example: Write a program to ask the user for the brightness of a light bulb (in Watts) and print out the expected lifetime: Brightness Lifetime in hours 25 2500 40,60 1000 75, 100 750 otherwise Wrong Input 36 Answer: 37 Answer: 38 Example: Write a program that displays the following menu, then calculates the required operation according to the menu. The program should give an error message for invalid inputs. Enter your choice: 1. Add the two numbers 2. Subtract the two number 3. Multiplication the two numbers. 39 Answer: 40 Answer: 41 Programming 1 Lecture 8 & 9 Chapter 3: Controlling Program Flow Dr/ Mahmoud Gamal 1 Program Execution Flow: 2 Loop (Iteration) Statements: Loop statements allow repeatedly executing a statement or a sequence of statements one or more times as long as some condition remains true. There are three loop statements in Java 1) for loop statement 2) while loop statement 3) do-while loop statement 3 Types of Loops: 1- Count-Controlled loops: Repeat a specified number of times For Loop 2- Sentinel-Controlled (indefinite) loops: Some condition within the loop body changes and this causes the repeating to stop While Do… While 4 1- for Loop Statement: Repetition with for loops: So far, repeating a statement is redundant: System.out.println(“Ahmed says:"); System.out.println("I am so smart"); System.out.println("I am so smart"); System.out.println("I am so smart"); System.out.println("I am so smart"); System.out.println("S-M-R-T... I mean S-M-A-R-T"); Java's for loop statement performs a task many times. System.out.println("Homer says:"); for (int i = 1; i