Write a C program to calculate the average of three numbers.
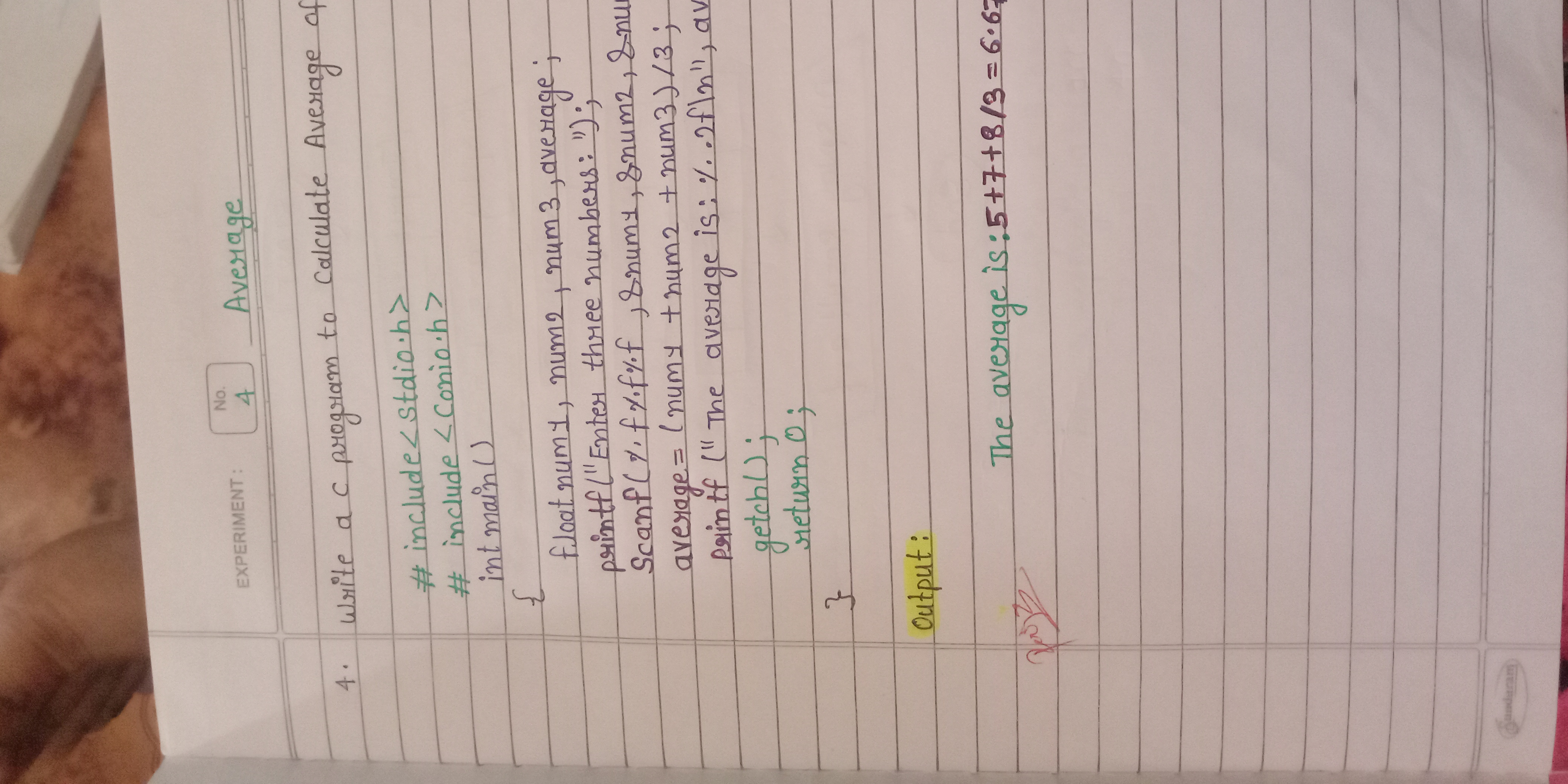
Understand the Problem
The question asks for a C program that calculates the average of three numbers, including instructions on input and output formatting.
Answer
```c #include <stdio.h> #include <math.h> int main() { float num1, num2, num3, average; printf("Enter three numbers: "); scanf("%f %f %f", &num1, &num2, &num3); average = (num1 + num2 + num3) / 3; printf("The average is: %.2f\n", average); return 0; } ```
Answer for screen readers
#include <stdio.h>
#include <math.h>
int main() {
float num1, num2, num3, average;
printf("Enter three numbers: ");
scanf("%f %f %f", &num1, &num2, &num3);
average = (num1 + num2 + num3) / 3;
printf("The average is: %.2f\n", average);
return 0;
}
Steps to Solve
- Include Necessary Libraries Include the standard input-output library and the math library to perform input and output operations.
#include <stdio.h>
#include <math.h>
- Declare Variables Declare variables to store the three numbers and the average.
float num1, num2, num3, average;
- Input the Numbers
Use
printf
to prompt the user to input three numbers andscanf
to read the numbers.
printf("Enter three numbers: ");
scanf("%f %f %f", &num1, &num2, &num3);
- Calculate the Average Calculate the average using the formula for the average of three numbers.
average = (num1 + num2 + num3) / 3;
- Output the Average Print the calculated average to the console.
printf("The average is: %.2f\n", average);
- Return Statement End the program with a return statement.
return 0;
#include <stdio.h>
#include <math.h>
int main() {
float num1, num2, num3, average;
printf("Enter three numbers: ");
scanf("%f %f %f", &num1, &num2, &num3);
average = (num1 + num2 + num3) / 3;
printf("The average is: %.2f\n", average);
return 0;
}
More Information
This C program calculates the average of three floating-point numbers entered by the user. The average is displayed with two decimal points for clarity.
Tips
- Forgetting to include necessary libraries: Always ensure
#include <stdio.h>
and#include <math.h>
are included in your code. - Incorrect format specifiers in
scanf
orprintf
: Ensure you use%f
for floating-point numbers and format the output correctly. - Forgetting the
return 0;
statement: This is essential to indicate that the program has finished executing successfully.
AI-generated content may contain errors. Please verify critical information