Given the variables int x and int y declare and initialize a string named values that is of the format x hyphen y. Example: "22-13" (Without spaces)
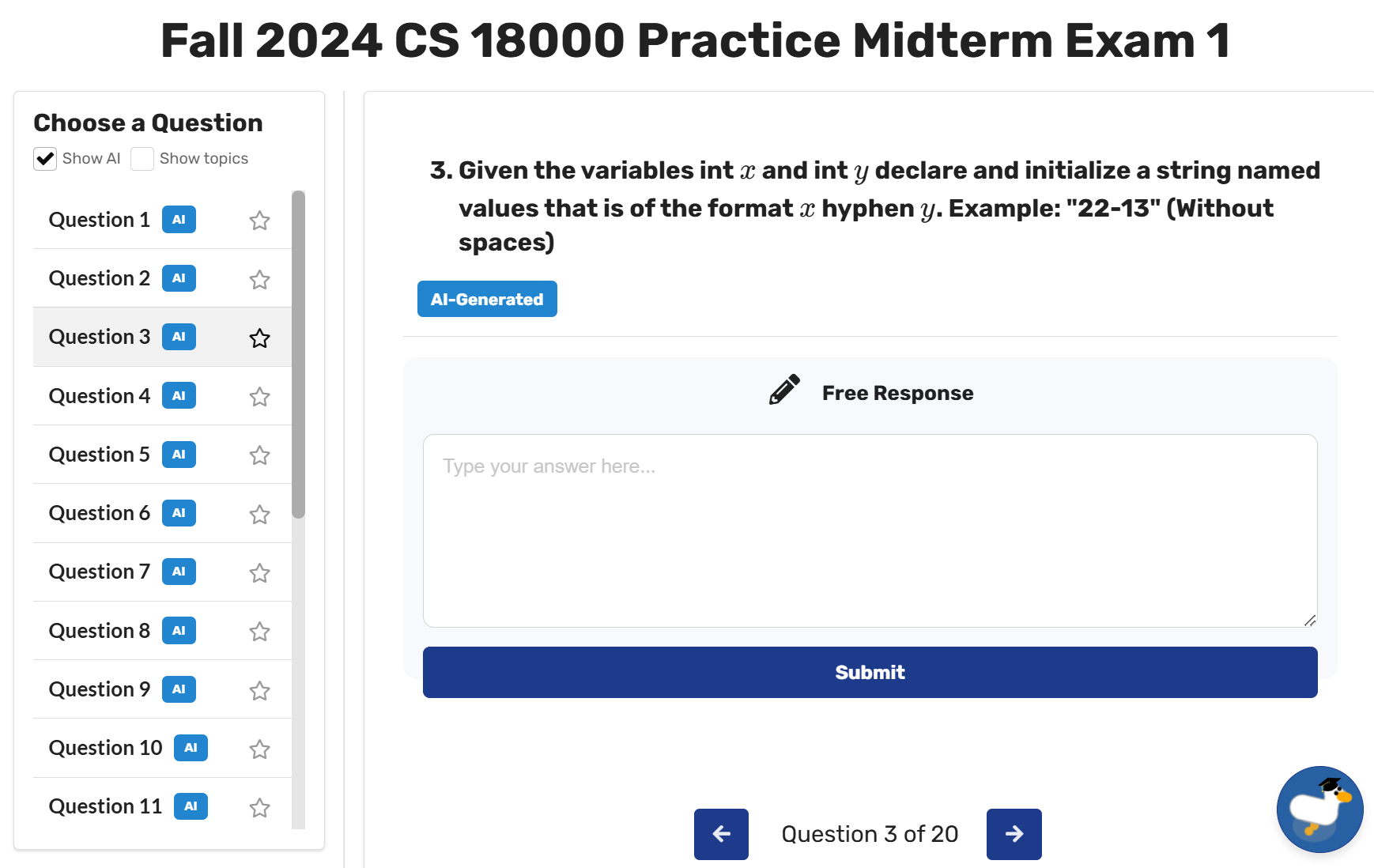
Understand the Problem
The question is asking us to declare and initialize a string variable in a programming context, formatted as 'x-y' where 'x' and 'y' are integer variables. We need to focus on how to correctly format and assign these values to a string variable.
Answer
The string can be initialized as $result = \text{String.valueOf}(x) + "-" + \text{String.valueOf}(y);$
Answer for screen readers
The formatted string variable can be initialized as:
String result = String.valueOf(x) + "-" + String.valueOf(y);
Steps to Solve
-
Declare Integer Variables
Start by declaring the integer variables
x
andy
and assign them some values. For this example, let’s use:
int x = 22;
int y = 13;
-
Convert Integer to String
To create a string in the desired format, we need to convert these integers into a string representation. You can use
String.valueOf()
to convert integers to strings:
String xString = String.valueOf(x);
String yString = String.valueOf(y);
-
Concatenate with Hyphen
Now concatenate the two string representations with a hyphen in between. This can be done using the
+
operator:
String result = xString + "-" + yString;
-
Final Result
At this point, you can print or use the
result
string:
System.out.println(result); // This will output: 22-13
The formatted string variable can be initialized as:
String result = String.valueOf(x) + "-" + String.valueOf(y);
More Information
This approach combines string concatenation in Java with integer conversion. The resulting string will display as "22-13" when x
is set to 22 and y
is set to 13.
Tips
null
AI-generated content may contain errors. Please verify critical information