Given the variables int x and int y declare and initialize a string named values that is of the format x hyphen y. Example: '22-13' (Without spaces)
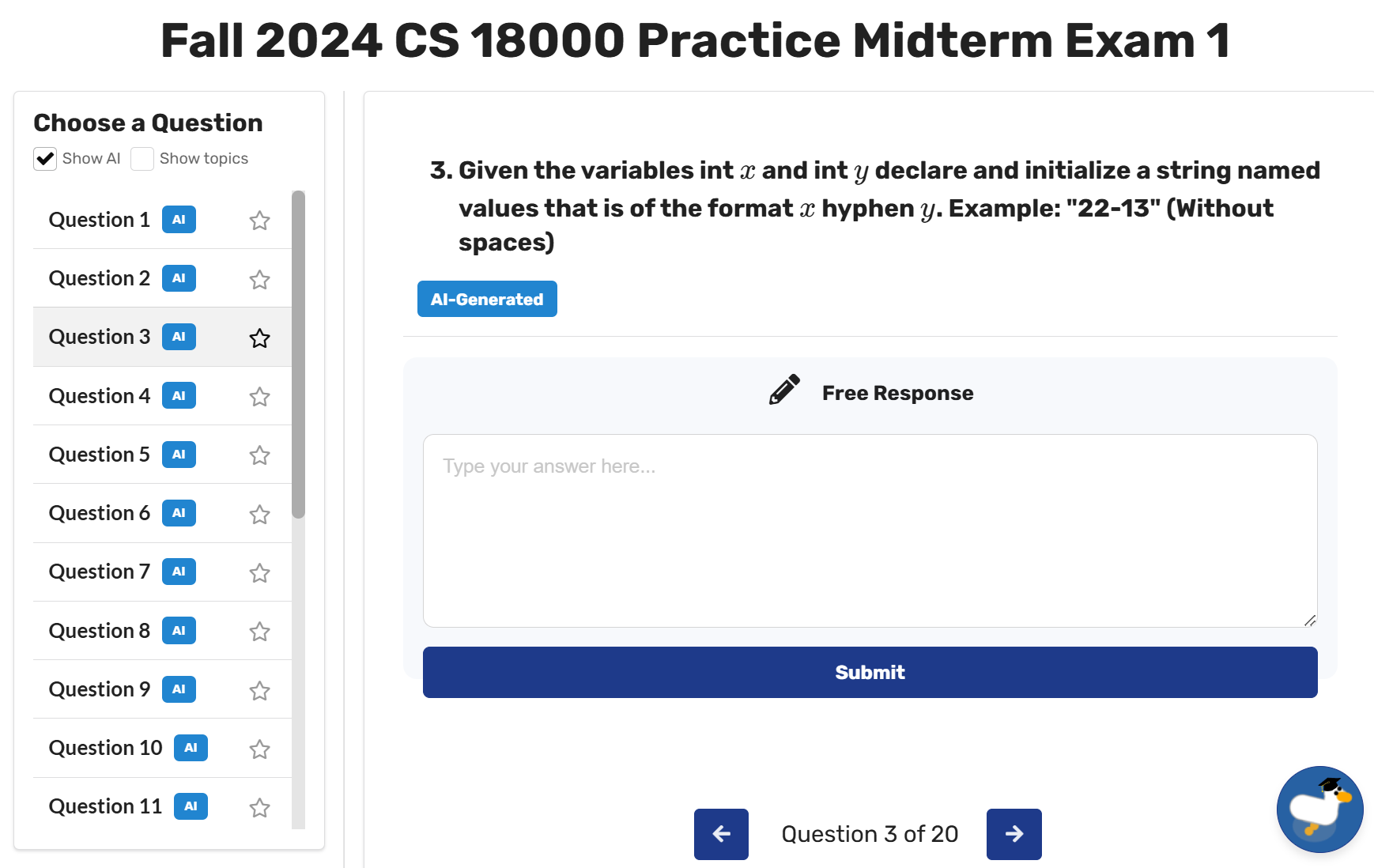
Understand the Problem
The question is asking to declare and initialize a string variable with a specific format using two integer variables, x and y. The format should include a hyphen between the two integers. For example, if x is 22 and y is 13, the output should be the string '22-13'.
Answer
```java String formattedString = String.valueOf(x) + "-" + String.valueOf(y); ```
Answer for screen readers
The final string variable declaration and initialization would be:
String formattedString = String.valueOf(x) + "-" + String.valueOf(y);
Steps to Solve
-
Declare integer variables
Start by declaring two integer variables
x
andy
. For example:
int x = 22; // Example value
int y = 13; // Example value
-
Convert integers to strings
Next, convert both integer values to strings. In many programming languages, this can be done using methods like
String.valueOf()
. For example:
String strX = String.valueOf(x);
String strY = String.valueOf(y);
- Concatenate with a hyphen Now, concatenate the two string representations with a hyphen in between. In Java, this can be done straightforwardly:
String formattedString = strX + "-" + strY;
- Complete string initialization You can also create the final string in a single line without intermediate variables:
String formattedString = String.valueOf(x) + "-" + String.valueOf(y);
The final string variable declaration and initialization would be:
String formattedString = String.valueOf(x) + "-" + String.valueOf(y);
More Information
This method is commonly used in Java and other programming languages to format strings dynamically using variable values. It allows for easy creation of formatted outputs based on variable content.
Tips
- Forgetting to convert integers to strings before concatenation will cause errors.
- Misspelling method names or using incorrect syntax for the chosen programming language can lead to compilation issues.
AI-generated content may contain errors. Please verify critical information