Explain the purpose of the break statement in C programming. Give an example. Define an array and give an example. Explain the purpose of the strlen() function in C. State any two... Explain the purpose of the break statement in C programming. Give an example. Define an array and give an example. Explain the purpose of the strlen() function in C. State any two modes in which a file can be opened in C. What are the limitations of static arrays in C? Write the syntax of the ternary (conditional) operator and explain its usage. Differentiate between static arrays and dynamic arrays in C. What is the syntax for opening a file in C? Give an example.
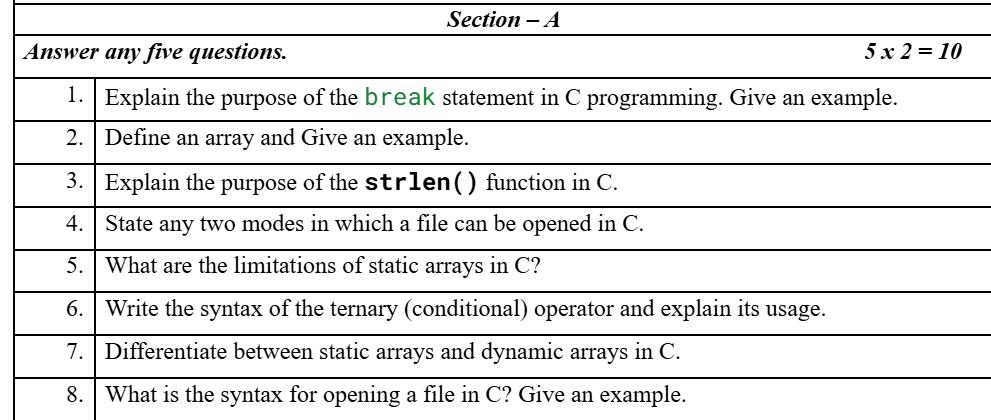
Understand the Problem
The question is asking for explanations and examples related to various concepts in C programming, including the break statement, arrays, file modes, limitations of static arrays, ternary operators, and differences between static and dynamic arrays.
Answer
1. Exits loops/switch. 2. Stores elements. 3. Returns string length. 4. Modes: 'r', 'w'. 5. Fixed size. 6. `condition ? result1 : result2`. 7. Static: fixed, dynamic: resizable. 8. `FILE *fptr = fopen("filename", "mode");`
- The
break
statement exits loops or switch statements. Example:
for(int i = 0; i < 10; i++) {
if(i == 5)
break;
}
- An array stores multiple elements of the same type. Example:
int numbers[5] = {1, 2, 3, 4, 5};
-
strlen()
returns the length of a string. -
Two modes to open a file:
- 'r' (read mode)
- 'w' (write mode)
-
Limitations of static arrays:
- Fixed size
- Potential memory waste
-
Syntax of the ternary operator:
condition ? result1 : result2;
Usage example:
int max = (a > b) ? a : b;
- Static arrays have a fixed size; dynamic arrays can be resized during runtime.
- Syntax to open a file:
FILE *fptr = fopen("filename", "mode");
Example:
FILE *fptr = fopen("data.txt", "r");
Answer for screen readers
- The
break
statement exits loops or switch statements. Example:
for(int i = 0; i < 10; i++) {
if(i == 5)
break;
}
- An array stores multiple elements of the same type. Example:
int numbers[5] = {1, 2, 3, 4, 5};
-
strlen()
returns the length of a string. -
Two modes to open a file:
- 'r' (read mode)
- 'w' (write mode)
-
Limitations of static arrays:
- Fixed size
- Potential memory waste
-
Syntax of the ternary operator:
condition ? result1 : result2;
Usage example:
int max = (a > b) ? a : b;
- Static arrays have a fixed size; dynamic arrays can be resized during runtime.
- Syntax to open a file:
FILE *fptr = fopen("filename", "mode");
Example:
FILE *fptr = fopen("data.txt", "r");
More Information
The break
statement is useful for stopping loops early, such as when a condition is met. Arrays are a foundational data structure in C, useful for spatial locality and predictable memory allocation.
Tips
Common mistakes include forgetting to properly define array sizes or attempting to use break
outside of loops or switch statements.
Sources
- Break Statement in C - GeeksforGeeks - geeksforgeeks.org
- strlen() function in C - GeeksforGeeks - geeksforgeeks.org
- Array in C: Declaration, Advantages, & Examples - Simplilearn.com - simplilearn.com
AI-generated content may contain errors. Please verify critical information