Java AWT Event Handling PDF
Document Details
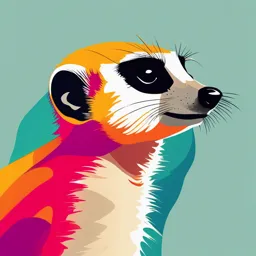
Uploaded by WorldFamousLife7260
Yuvakshetra Institute of Management Studies
Tags
Summary
This document introduces AWT event handling in Java, covering event types, event handling mechanisms, and a list of common event classes. The document is part of a BCA course material.
Full Transcript
BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING AWT EVENT HANDLING What is an Event? Change in the state of an object is known as event i.e. event describes the chang...
BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING AWT EVENT HANDLING What is an Event? Change in the state of an object is known as event i.e. event describes the change in state of source. Events are generated as result of user interaction with the graphical user interface components. For example, clicking on a button, moving the mouse, entering a character through keyboard, selecting an item from list, scrolling the page are the activities that causes an event to happen. Types of Event The events can be broadly classified into two categories: Foreground Events - Those events which require the direct interaction of user. They are generated as consequences of a person interacting with the graphical components in Graphical User Interface. For example, clicking on a button, moving the mouse, entering a character through keyboard, selecting an item from list, scrolling the page etc. Background Events - Those events that require the interaction of end user are known as background events. Operating system interrupts, hardware or software failure, timer expires, an operation completion are the example of background events. What is Event Handling? Event Handling is the mechanism that controls the event and decides what should happen if an event occurs. This mechanism have the code which is known as event handler that is executed when an event occurs. Java Uses the Delegation Event Model to handle the events. This model defines the standard mechanism to generate and handle the events. Let’s have a brief introduction to this model. The Delegation Event Model has the following key participants namely: Source - The source is an object on which event occurs. Source is responsible for providing information of the occurred event to its handler. Java provide as with classes for source object. Listener - It is also known as event handler. Listener is responsible for generating response to an event. From java implementation point of view the listener is also an object. Listener waits until it receives an event. Once the event is received , the listener process the event an then returns. 1 Event Object – the class object which describes the event. Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING The benefit of this approach is that the user interface logic is completely separated from the logic that generates the event. The user interface element is able to delegate the processing of an event to the separate piece of code. In this model, Listener needs to be registered with the source object so that the listener can receive the event notification. This is an efficient way of handling the event because the event notifications are sent only to those listener that want to receive them. Steps involved in event handling The User clicks the button and the event is generated. Now the object of concerned event class is created automatically and information about the source and the event get populated with in same object. Event object is forwarded to the method of registered listener class. the method is now get executed and returns. An event occurs (like mouse click, key press, etc) which is followed by the event is broadcasted by the event source by invoking an agreed method on all event listeners. The event object is passed as argument to the agreed-upon method. Later the event listeners respond as they fit, like submit a form, displaying a message / alert etc. Event Classes Event Classes: Following is the list of commonly used event classes. Sr. No. Control & Description 1 AWTEvent It is the root event class for all AWT events. This class and its subclasses supercede the original java.awt.Event class. 2 ActionEvent The ActionEvent is generated when button is clicked or the item of a list is double clicked. 2 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING 3 InputEvent The InputEvent class is root event class for all component-level input events. 4 KeyEvent On entering the character the Key event is generated. 5 MouseEvent This event indicates a mouse action occurred in a component. 6 TextEvent The object of this class represents the text events. 7 WindowEvent The object of this class represents the change in state of a window. 8 AdjustmentEvent The object of this class represents the adjustment event emitted by Adjustable objects. 9 ComponentEvent The object of this class represents the change in state of a window. 10 ContainerEvent The object of this class represents the change in state of a window. 11 MouseMotionEvent The object of this class represents the change in state of a window. 12 PaintEvent The object of this class represents the change in state of a window. Event Listeners The Event listener represent the interfaces responsible to handle events. Java provides us various Event listener classes but we will discuss those which are more frequently used. Every method of an event listener method has a single argument as an object which is subclass of EventObject class. For example, mouse event listener methods will accept instance of MouseEvent, where MouseEvent 3 derives from EventObject. Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING EVENTLISTNER INTERFACE It is a marker interface which every listener interface has to extend. This class is defined in java.util package. Class declaration Following is the declaration for java.util.EventListener interface: public interface EventListener AWT Event Listener Interfaces: Following is the list of commonly used event listeners. Sr. No. Control & Description 1 ActionListener This interface is used for receiving the action events. 2 ComponentListener This interface is used for receiving the component events. 3 ItemListener This interface is used for receiving the item events. 4 KeyListener This interface is used for receiving the key events. 5 MouseListener This interface is used for receiving the mouse events. 6 TextListener This interface is used for receiving the text events. 4 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING 7 WindowListener This interface is used for receiving the window events. 8 AdjustmentListener This interface is used for receiving the adjusmtent events. 9 ContainerListener This interface is used for receiving the container events. 10 MouseMotionListener This interface is used for receiving the mouse motion events. 11 FocusListener This interface is used for receiving the focus events. KeyListener Interface The class which processes the KeyEvent should implement this interface.The object of that class must be registered with a component. The object can be registered using the addKeyListener() method. Interface declaration Following is the declaration for java.awt.event.KeyListener interface: public interface KeyListener extends EventListener Interface methods S.N. Method & Description 1 void keyPressed(KeyEvent e) 5 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING Invoked when a key has been pressed. 2 void keyReleased(KeyEvent e) Invoked when a key has been released. 3 void keyTyped(KeyEvent e) Invoked when a key has been typed. MouseListener Interface The class which processes the MouseEvent should implement this interface.The object of that class must be registered with a component. The object can be registered using the addMouseListener() method. Interface declaration Following is the declaration for java.awt.event.MouseListener interface: public interface MouseListener extends EventListener Interface methods S.N. Method & Description 1 void mouseClicked(MouseEvent e) Invoked when the mouse button has been clicked (pressed and released) on a component. 2 void mouseEntered(MouseEvent e) Invoked when the mouse enters a component. 6 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING 3 void mouseExited(MouseEvent e) Invoked when the mouse exits a component. 4 void mousePressed(MouseEvent e) Invoked when a mouse button has been pressed on a component. 5 void mouseReleased(MouseEvent e) Invoked when a mouse button has been released on a component. WindowListener Interface The class which processes the WindowEvent should implement this interface.The object of that class must be registered with a component. The object can be registered using the addWindowListener() method. Interface declaration Following is the declaration for java.awt.event.WindowListener interface: public interface WindowListener extends EventListener Interface methods S.N. Method & Description 1 void windowActivated(WindowEvent e) Invoked when the Window is set to be the active Window. 2 void windowClosed(WindowEvent e) Invoked when a window has been closed as the result of calling dispose on the window. 7 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING 3 void windowClosing(WindowEvent e) Invoked when the user attempts to close the window from the window's system menu. 4 void windowDeactivated(WindowEvent e) Invoked when a Window is no longer the active Window. 5 void windowDeiconified(WindowEvent e) Invoked when a window is changed from a minimized to a normal state. 6 void windowIconified(WindowEvent e) Invoked when a window is changed from a normal to a minimized state. 7 void windowOpened(WindowEvent e) Invoked the first time a window is made visible. MouseMotionListener Interface The interfaceMouseMotionListener is used for receiving mouse motion events on a component. The class that process mouse motion events needs to implements this interface. Class declaration Following is the declaration for java.awt.event.MouseMotionListener interface: public interface MouseMotionListener extends EventListener Interface methods S.N. Method & Description 1 void mouseDragged(MouseEvent e) 8 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING Invoked when a mouse button is pressed on a component and then dragged. 2 void mouseMoved(MouseEvent e) Invoked when the mouse cursor has been moved onto a component but no buttons have been pushed. Example Program import java.awt.*; import java.awt.event.*; public class KeyListenerExample extends Frame implements KeyListener{ Label l; TextArea area; KeyListenerExample(){ l=new Label(); l.setBounds(20,50,100,20); area=new TextArea(); area.setBounds(20,80,300, 300); area.addKeyListener(this); add(l);add(area); setSize(400,400); setLayout(null); setVisible(true); } public void keyPressed(KeyEvent e) { l.setText("Key Pressed"); } public void keyReleased(KeyEvent e) { l.setText("Key Released"); } public void keyTyped(KeyEvent e) { l.setText("Key Typed"); } public static void main(String[] args) { new KeyListenerExample(); } } 9 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING JAVA ADAPTER CLASSES Adapter Classes in Java are nothing new. They are used to provide the implementation of Listener interfaces. The advantage of the adapter class is that it saves code. If we inherit the adapter class using the Adapter interface, we will not be forced to provide the implementation of all the methods of the listener interface. There are namely three packages in which the Java adapter classes are found. The three packages are: java.awt. event java.awt. dnd java.swing. event Also, another advantage of the Adapter Class is that they are useful when we want to process a few of the events that are handled by a particular event listener interface. Below are some of the Adapter classes and their corresponding listener interface. Advantages of the Adapter class Assists unrelated classes to work together. Provides a way to use classes in multiple ways. Increases the transparency of classes. Its provides a way to include related patterns in a class. It provides a pluggable kit for developing applications. It makes a class highly reusable. These are present in the Abstract Window Toolkit of the Java package. Adapter Class Listener Interface WindowAdapter WindowListener KeyAdapter KeyListener MouseAdapter MouseListener 10 MouseMotionAdapter MouseMotionListener Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING FocusAdapter FocusListener ComponentAdapter ComponentListener ContainerAdapter ContainerListener HierarchyBoundsAdapter HierarchyBoundsListener AWT - OVERVIEW Graphical User Interface Graphical User Interface (GUI) offers user interaction via some graphical components. For example our underlying Operating System also offers GUI via window,frame,Panel, Button, Textfield, TextArea, Listbox, Combobox, Label, Checkbox etc. These all are known as components. Using these components we can create an interactive user interface for an application. GUI provides result to end user in response to raised events.GUI is entirely based events. For example clicking over a button, closing a window, opening a window, typing something in a textarea etc. These activities are known as events.GUI makes it easier for the end user to use an application. It also makes them interesting. Basic Terminologies Term Description Component Component is an object having a graphical representation that can be displayed on the screen and that can interact with the user. For examples buttons, checkboxes, list and scrollbars of a graphical user interface. Container Container object is a component that can contain other components. Components added to a container are tracked in a list. The order of the list will define the components' front-to-back stacking order within the container. If no 11 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING index is specified when adding a component to a container, it will be added to the end of the list. Panel Panel provides space in which an application can attach any other components, including other panels. Window Window is a rectangular area which is displayed on the screen. In different window we can execute different program and display different data. Window provide us with multitasking environment. A window must have either a frame, dialog, or another window defined as its owner when it's constructed. Frame A Frame is a top-level window with a title and a border. The size of the frame includes any area designated for the border. Frame encapsulates window. It and has a title bar, menu bar, borders, and resizing corners. Canvas Canvas component represents a blank rectangular area of the screen onto which the application can draw. Application can also trap input events from the use from that blank area of Canvas component. Examples of GUI based Applications Following are some of the examples for GUI based applications. Automated Teller Machine (ATM) Airline Ticketing System Information Kiosks at railway stations Mobile Applications Navigation Systems 12 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING Advantages of GUI over CUI GUI provides graphical icons to interact while the CUI (Character User Interface) offers the simple text-based interfaces. GUI makes the application more entertaining and interesting on the other hand CUI does not. GUI offers click and execute environment while in CUI every time we have to enter the command for a task. New user can easily interact with graphical user interface by the visual indicators but it is difficult in Character user interface. GUI offers a lot of controls of file system and the operating system while in CUI you have to use commands which is difficult to remember. Windows concept in GUI allow the user to view, manipulate and control the multiple applications at once while in CUI user can control one task at a time. GUI provides multitasking environment so as the CUI also does but CUI does not provide same ease as the GUI do. Using GUI it is easier to control and navigate the operating system which becomes very slow in command user interface. GUI can be easily customized. 13 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING Java AWT Hierarchy The hierarchy of Java AWT classes are given below. Container The Container is a component in AWT that can contain another components like buttons, textfields, labels etc. The classes that extends Container class are known as container such as Frame, Dialog and Panel. Window The window is the container that have no borders and menu bars. You must use frame, dialog or another window for creating a window. Panel The Panel is the container that doesn't contain title bar and menu bars. It can have other components 14 like button, textfield etc. Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING Frame The Frame is the container that contain title bar and can have menu bars. It can have other components like button, textfield etc. Useful Methods of Component class Method Description public void add(Component c) inserts a component on this component. public void setSize(int width,int height) sets the size (width and height) of the component. public void setLayout(LayoutManager m) defines the layout manager for the component. public void setVisible(boolean status) changes the visibility of the component, by default false. WORKING WITH: COLOR, FONT The Color class states colors in the default sRGB color space or colors in arbitrary color spaces identified by a ColorSpace. public void paint(Graphics g) { Font plainFont = new Font("Serif", Font.PLAIN, 24); g.setFont(plainFont); g.setColor(Color.red); g.drawString("Welcome to TutorialsPoint", 50, 70); g.setColor(Color.GRAY); g.drawString("Welcome to TutorialsPoint", 50, 120); } 15 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING SIMPLE GRAPHICS AWT Button The button class is used to create a labeled button that has platform independent implementation. The application result in some action when the button is pushed. Java AWT Button Example import java.awt.*; public class ButtonExample { public static void main(String[] args) { Frame f=new Frame("Button Example"); Button b=new Button("Click Here"); b.setBounds(50,100,80,30); f.add(b); f.setSize(400,400); f.setLayout(null); f.setVisible(true); } } Output: 16 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING Java AWT Button Example with ActionListener import java.awt.*; import java.awt.event.*; public class ButtonExample { public static void main(String[] args) { Frame f=new Frame("Button Example"); final TextField tf=new TextField(); tf.setBounds(50,50, 150,20); Button b=new Button("Click Here"); b.setBounds(50,100,60,30); b.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ tf.setText("Welcome to Javatpoint."); } }); f.add(b);f.add(tf); f.setSize(400,400); f.setLayout(null); f.setVisible(true); } } Output: 17 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING AWT TextField The object of a TextField class is a text component that allows the editing of a single line text. It inherits TextComponent class. Java AWT TextField Example import java.awt.*; class TextFieldExample{ public static void main(String args[]){ Frame f= new Frame("TextField Example"); TextField t1,t2; t1=new TextField("Welcome to Javatpoint."); t1.setBounds(50,100, 200,30); t2=new TextField("AWT Tutorial"); t2.setBounds(50,150, 200,30); f.add(t1); f.add(t2); f.setSize(400,400); f.setLayout(null); f.setVisible(true); } } Output: 18 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING AWT Choice The object of Choice class is used to show popup menu of choices. Choice selected by user is shown on the top of a menu. It inherits Component class. Java AWT Choice Example import java.awt.*; public class ChoiceExample { ChoiceExample(){ Frame f= new Frame(); Choice c=new Choice(); c.setBounds(100,100, 75,75); c.add("Item 1"); c.add("Item 2"); c.add("Item 3"); c.add("Item 4"); c.add("Item 5"); f.add(c); f.setSize(400,400); f.setLayout(null); f.setVisible(true); } public static void main(String args[]) { new ChoiceExample(); } } Output: 19 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING Java AWT List The object of List class represents a list of text items. By the help of list, user can choose either one item or multiple items. It inherits Component class. Java AWT List Example import java.awt.*; public class ListExample { ListExample(){ Frame f= new Frame(); List l1=new List(5); l1.setBounds(100,100, 75,75); l1.add("Item 1"); l1.add("Item 2"); l1.add("Item 3"); l1.add("Item 4"); l1.add("Item 5"); f.add(l1); f.setSize(400,400); f.setLayout(null); 20 f.setVisible(true); Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING } public static void main(String args[]) { new ListExample(); } } Output: Java AWT Scrollbar The object of Scrollbar class is used to add horizontal and vertical scrollbar. Scrollbar is a GUI component allows us to see invisible number of rows and columns. Java AWT Scrollbar Example import java.awt.*; class ScrollbarExample{ ScrollbarExample(){ Frame f= new Frame("Scrollbar Example"); Scrollbar s=new Scrollbar(); s.setBounds(100,100, 50,100); f.add(s); f.setSize(400,400); 21 f.setLayout(null); Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING f.setVisible(true); } public static void main(String args[]){ new ScrollbarExample(); } } Output: Java AWT TextArea The object of a TextArea class is a multi line region that displays text. It allows the editing of multiple line text. It inherits TextComponent class. Java AWT TextArea Example import java.awt.*; public class TextAreaExample { TextAreaExample(){ Frame f= new Frame(); 22 TextArea area=new TextArea("Welcome to javatpoint"); Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING area.setBounds(10,30, 300,300); f.add(area); f.setSize(400,400); f.setLayout(null); f.setVisible(true); } public static void main(String args[]) { new TextAreaExample(); } } Output: Java AWT Checkbox The Checkbox class is used to create a checkbox. It is used to turn an option on (true) or off (false). Clicking on a Checkbox changes its state from "on" to "off" or from "off" to "on". Java AWT Checkbox Example 23 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING import java.awt.*; public class CheckboxExample { CheckboxExample(){ Frame f= new Frame("Checkbox Example"); Checkbox checkbox1 = new Checkbox("C++"); checkbox1.setBounds(100,100, 50,50); Checkbox checkbox2 = new Checkbox("Java", true); checkbox2.setBounds(100,150, 50,50); f.add(checkbox1); f.add(checkbox2); f.setSize(400,400); f.setLayout(null); f.setVisible(true); } public static void main(String args[]) { new CheckboxExample(); } } Output: 24 Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING Java AWT MenuItem and Menu he object of MenuItem class adds a simple labeled menu item on menu. The items used in a menu must belong to the MenuItem or any of its subclass. The object of Menu class is a pull down menu component which is displayed on the menu bar. It inherits the MenuItem class. Java AWT MenuItem and Menu Example import java.awt.*; class MenuExample { MenuExample(){ Frame f= new Frame("Menu and MenuItem Example"); MenuBar mb=new MenuBar(); Menu menu=new Menu("Menu"); Menu submenu=new Menu("Sub Menu"); MenuItem i1=new MenuItem("Item 1"); MenuItem i2=new MenuItem("Item 2"); MenuItem i3=new MenuItem("Item 3"); MenuItem i4=new MenuItem("Item 4"); MenuItem i5=new MenuItem("Item 5"); menu.add(i1); menu.add(i2); menu.add(i3); submenu.add(i4); submenu.add(i5); menu.add(submenu); mb.add(menu); f.setMenuBar(mb); f.setSize(400,400); f.setLayout(null); f.setVisible(true); 25 } public static void main(String args[]) Page BCA - JAVA YUVAKSHETRA INSTITUTE OF MANAGEMENT STUDIES BCA 2017 ONWARS BATCH PRORAMMING { new MenuExample(); } } Output: 26 Page