Python Programming Unit 1 (GE8151) PDF
Document Details
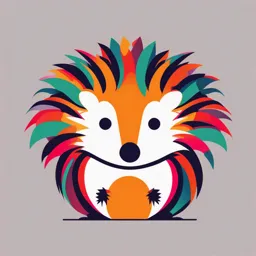
Uploaded by FamousObsidian499
Mrs.N.Jeevananthini
Tags
Summary
This document contains a syllabus for a Python programming course, specifically focusing on foundational concepts like algorithmic problem-solving and Python programming language elements.
Full Transcript
GE8151 PROBLEM SOLVING AND PYTHON PROGRAMMING N.J SYLLABUS OBJECTIVES: To know the basics of algorithmic problem solving To read and write simple Python progr...
GE8151 PROBLEM SOLVING AND PYTHON PROGRAMMING N.J SYLLABUS OBJECTIVES: To know the basics of algorithmic problem solving To read and write simple Python programs. To develop Python programs with conditionals and loops. To define Python functions and call them. To use Python data structures –- lists, tuples, dictionaries. To do input/output with files in Python. UNIT I ALGORITHMIC PROBLEM SOLVING 9 Algorithms, building blocks of algorithms (statements, state, control flow, functions), notation (pseudo code, flow chart, programming language), algorithmic problem solving, simple strategies for developing algorithms (iteration, recursion). Illustrative problems: find minimum in a list, insert a card in a list of sorted cards, guess an integer number in a range, Towers of Hanoi. UNIT II DATA, EXPRESSIONS, STATEMENTS 9 Python interpreter and interactive mode; values and types: int, float, boolean, string, and list; variables, expressions, statements, tuple assignment, precedence of operators, comments; modules and functions, function definition and use, flow of execution, parameters and arguments; Illustrative programs: exchange the values of two variables, circulate the values of n variables, distance between two points. UNIT III CONTROL FLOW, FUNCTIONS 9 Conditionals: Boolean values and operators, conditional (if), alternative (if-else), chained conditional (if-elif-else); Iteration: state, while, for, break, continue, pass; Fruitful functions: return values, parameters, local and global scope, function composition, recursion; Strings: string slices, immutability, string functions and methods, string module; Lists as arrays. Illustrative programs: square root, gcd, exponentiation, sum an array of numbers, linear search, binary search. UNIT IV LISTS, TUPLES, DICTIONARIES 9 Lists: list operations, list slices, list methods, list loop, mutability, aliasing, cloning lists, list parameters; Tuples: tuple assignment, tuple as return value; Dictionaries: operations and methods; advanced list processing - list comprehension; Illustrative programs: selection sort, insertion sort, mergesort, histogram. UNIT V FILES, MODULES, PACKAGES 9 Files and exception: text files, reading and writing files, format operator; command line arguments, errors and exceptions, handling exceptions, modules, packages; Illustrative programs: word count, copy file. TOTAL : 45 PERIODS TEXT BOOKS Allen B. Downey, ``Think Python: How to Think Like a Computer Scientist„„, 2nd edition, Updatedfor Python 3,Shroff/O„Reilly Publishers, 2016 (http://greenteapress.com/wp/thinkpython/) Guido van Rossum and Fred L. Drake Jr, ―An Introduction to Python – Revised and updated for Python 3.2, Network Theory Ltd., 2011. REFERENCES: John V Guttag, ―Introduction to Computation and Programming Using Python„„, Revised and expanded Edition, MIT Press , 2013 Robert Sedgewick, Kevin Wayne, Robert Dondero, ―Introduction to Programming in Python: An Inter-disciplinary Approach, Pearson India Education Services Pvt. Ltd., 2016. 1 Mrs.N.Jeevananthini Assistant Professor Department of CS and BCA N.J UNIT I ALGORITHMIC PROBLEM SOLVING INTRODUCTION PROBLEM SOLVING Problem solving is the systematic approach to define the problem and creating number of solutions. The problem solving process starts with the problem specifications and ends with a correct program. PROBLEM SOLVING TECHNIQUES Problem solving technique is a set of techniques that helps in providing logic for solving a problem. Problem solving can be expressed in the form of 1. Algorithms. 2. Flowcharts. 3. Pseudo codes. 4. Programs 5.ALGORITHM It is defined as a sequence of instructions that describe a method for solving a problem. In other words it is a step by step procedure for solving a problem Should be written in simple English Each and every instruction should be precise and unambiguous. Instructions in an algorithm should not be repeated infinitely. Algorithm should conclude after a finite number of steps. Should have an end point Derived results should be obtained only after the algorithm terminates. Qualities of a good algorithm The following are the primary factors that are often used to judge the quality of the algorithms. Time – To execute a program, the computer system takes some amount of time. The lesser is the time required, the better is the algorithm. Memory – To execute a program, computer system takes some amount of memory space. The lesser is the memory required, the better is the algorithm. Accuracy – Multiple algorithms may provide suitable or correct solutions to a given problem, some of these may provide more accurate results than others, and such algorithms may be suitable Building Blocks of Algorithm As algorithm is a part of the blue-print or plan for the computer program. An algorithm is constructed using following blocks. Statements States Control flow Function 2 N.J Statements Statements are simple sentences written in algorithm for specific purpose. Statements may consists of assignment statements, input/output statements, comment statements Example: Read the value of „a‟ //This is input statement Calculate c=a+b //This is assignment statement Print the value of c // This is output statement Comment statements are given after // symbol, which is used to tell the purpose of the line. States An algorithm is deterministic automation for accomplishing a goal which, given an initial state, will terminate in a defined end-state. An algorithm will definitely have start state and end state. Control Flow Control flow which is also stated as flow of control, determines what section of code is to run in program at a given time. There are three types of flows, they are 1. Sequential control flow 2. Selection or Conditional control flow 3. Looping or repetition control flow Sequential control flow: The name suggests the sequential control structure is used to perform the action one after another. Only one step is executed once. The logic is top to bottom approach. Example Description: To find the sum of two numbers. 1. Start 2. Read the value of „a‟ 3. Read the value of „b‟ 4. Calculate sum=a+b 5. Print the sum of two number 6. Stop Selection or Conditional control flow Selection flow allows the program to make choice between two alternate paths based on condition. It is also called as decision structure Basic structure: IFCONDITION is TRUE then perform some action ELSE IF CONDITION is FALSE then perform some action The conditional control flow is explained with the example of finding greatest of two numbers. Example Description: finding the greater number 1. Start 2. Read a 3 N.J 3. Read b 4. If a>b then 1. Print a is greater else 2. Print b is greater 5. Stop Repetition control flow Repetition control flow means that one or more steps are performed repeatedly until some condition is reached. This logic is used for producing loops in program logic when one one more instructions may need to be executed several times or depending on condition. Basic Structure: Repeat untilCONDITIONis true Statements Example Description: to print the values from 1 to n 1. Start 2. Read the value of „n‟ 3. Initialize i as 1 4. Repeat step 4.1 until i< n 1. Print i 5. Stop Function A function is a block of organized, reusable code that is used to perform a single, related action. Function is also named as methods, sub-routines. Elements of functions: 1. Name for declaration of function 2. Body consisting local declaration and statements 3. Formal parameter 4. Optional result type. Basic Syntax function_name(parameters) function statements end function Algorithm for addition of two numbers using function Main function() Step 1: Start Step 2:Call the function add() Step 3: Stop sub function add() Step1:Functionstart 4 N.J Step2:Geta,bValues Step 3: add c=a+b Step 4: Printc Step 5: Return 2.NOTATIONS OF AN ALGORITHM Algorithm can be expressed in many different notations, including Natural Language, Pseudo code, flowcharts and programming languages. Natural language tends to be verbose and ambiguous. Pseudocode and flowcharts are represented through structured human language. A notation is a system of characters, expressions, graphics or symbols designs used among each others in problem solving to represent technical facts, created to facilitate the best result for a program Pseudocode Pseudocode is an informal high-level description of the operating principle of a computer program or algorithm. It uses the basic structure of a normal programming language, but is intended for human reading rather than machine reading. It is text based detail design tool. Pseudo means false and code refers to instructions written in programming language. Pseudocode cannot be compiled nor executed, and there are no real formatting or syntax rules. The pseudocode is written in normal English language which cannot be understood by the computer. Example: Pseudocode: To find sum of two numbers READ num1,num2 sum=num1+num2 PRINT sum Basic rules to write pseudocode: 1. Only one statement per line. Statements represents single action is written on same line. For example to read the input, all the inputs must be read using single statement. 2. Capitalized initial keywords The keywords should be written in capital letters. Eg: READ, WRITE, IF, ELSE, ENDIF, WHILE, REPEAT, UNTIL Example: Pseudocode: Find the total and average of three subjects RAED name, department, mark1, mark2, mark3 Total=mark1+mark2+mark3 Average=Total/3 WRITE name, department,mark1, mark2, mark3 3. Indent to show hierarchy Indentation is a process of showing the boundaries of the structure. 4. End multi-line structures Each structure must be ended properly, which provides more clarity. Example: 5 N.J Pseudocode: Find greatest of two numbers READ a, b IF a>b then PRINT a is greater ELSE PRINT b is greater ENDIF 5. Keep statements language independent. Pesudocode must never written or use any syntax of any programming language. Advantages of Pseudocode Can be done easily on a word processor Easily modified Implements structured concepts well It can be written easily It can be read and understood easily Converting pseudocode to programming language is easy as compared with flowchart Disadvantages of Pseudocode It is not visual There is no standardized style or format Flowchart A graphical representation of an algorithm. Flowcharts is a diagram made up of boxes, diamonds, and other shapes, connected by arrows. Each shape represents a step in process and arrows show the order in which they occur. Table 1: Flowchart Symbols S.No Name of Symbol Type Description symbol 1. Terminal Oval Represent the start and Symbol stop of the program. 2. Input/ Output Parallelogram Denotes either input or symbol output operation. 3. Process symbol Rectangle Denotes the process to be carried 4. Decision symbol Diamond Represents decision making and branching 5. Flow lines Arrow lines Represents the sequence of steps and direction of flow. Used to connect symbols. 6 N.J 6. Connector Circle A connector symbol is represented by a circle and a letter or digit is placed in the circle to specify the link. This symbol is used to connect flowcharts. Rules for drawing flowchart 1. In drawing a proper flowchart, all necessary requirements should be listed out in logical order. 2. The flow chart should be clear, neat and easy to follow. There should not be any room for ambiguity in understanding the flowchart. 3. The usual directions of the flow of a procedure or system is from left to right or top to bottom. Only one flow line should come out from a process symbol. 4. Only one flow line should enter a decision symbol, but two or three flow lines, one for each possible answer, cap leave the decision symbol. 5. Only one flow line is used in conjunction with terminal symbol. 6. If flowchart becomes complex, it is better to use connector symbols to reduce the number of flow lines. 7. Ensure that flowchart has logical start and stop. Advantages of Flowchart Communication: Flowcharts are better way of communicating the logic of the system. Effective Analysis With the help of flowchart, a problem can be analyzed in more effective way. Proper Documentation Flowcharts are used for good program documentation, which is needed for various purposes. Efficient Coding 7 The flowcharts act as a guide or blue print during the system analysis and program N.J development phase. Systematic Testing and Debugging The flowchart helps in testing and debugging the program Efficient Program Maintenance The maintenance of operating program becomes easy with the help of flowchart. It helps the programmer to put efforts more efficiently on that part. Disadvantages of Flowchart Complex Logic: Sometimes, the program logic is quite complicated. In that case flowchart becomes complex and difficult to use. Alteration and Modification: If alterations are required the flowchart may require re- drawing completely. Reproduction: As the flowchart symbols cannot be typed, reproduction becomes problematic. Control Structures using flowcharts and Pseudocode Sequence Structure Pseudocode Flow Chart General Structure Process 1 …. Process 1 Process 2 … Process 2 Process 3 Process 3 Example READ a Start READ b Result c=a+b PRINT c a=10,b=20 c=a+b print c Stop 8 N.J Conditional Structure Conditional structure is used to check the condition. It will be having two outputs only (True or False) IF and IF…ELSE are the conditional structures used in Python language. CASE is the structure used to select multi way selection control. It is not supported in Python. Pseudocode Flow Chart General Structure IF condition THEN Yes Process 1 if(condition) ENDIF Process 1 No Process 2 Example READ a Start READ b IF a>b THEN PRINT a is greater a=10,b=20 Yes if (a>b) Print a is greater No Stop IF… ELSE IF…THEN…ELSE is the structure used to specify, if the condition is true, then execute Process1, else, that is condition is false then execute Process2 Pseudocode Flow Chart General Structure IF condition THEN Process 1 Yes if(condition) ELSE Process 2 ENDIF Process 1 No Process 2 Example 9 N.J READ a Start READ b IF a>b THEN a=10,b=20 PRINT a is greater Yes if (a>b) Print a is greater No Print b is greater Stop Iteration or Looping Structure Looping is generally used with WHILE or DO...WHILE or FOR loop. WHILE and FOR is entry checked loop Pseudocode Flow Chart General Structure WHILE condition Body of the loop No ENDWHILE if(condition) Yes Body of the loop Example DO…WHILE is exit checked loop, so the loop will be executed at least once. 10 N.J INITIALIZE a=1 Start WHILE a