SIN 3011 / SIM 3025 Scientific Computing Chapter 1 PDF
Document Details
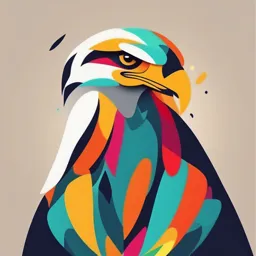
Uploaded by GraciousTellurium9246
Universiti Malaya
Noor Fadiya Mohd Noor
Tags
Summary
This document is a chapter on Basic Elements of C++ in Scientific Computing course. It covers basic concepts and rules in C++ programming, how to create a C++ program, statements, preprocessor directives, simple data types, string data type, variable and constant, operators and arithmetic expressions, and precedence order.
Full Transcript
SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing SIN 3011 / SIM 3025 Chapter 1: Basic Elements of C++...
SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing SIN 3011 / SIM 3025 Chapter 1: Basic Elements of C++ Objectives In this chapter, you will learn/review: – Basic concepts and rules in C++ programming – How to create a C++ program – Statements – Preprocessor directives – Simple data types – string data type – Variable and constant – Operators and arithmetic expressions – Precedence order Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 2 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 1 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 1.0 Introduction Programming language: – A set of rules, symbols and special words Programming – Process of planning and creating a program Computer program – Sequence of statements whose objective is to accomplish a task Function (or subprogram): – Collection of statements; when executed, accomplishes something – May be predefined or standard Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 3 1.1 Syntax Syntax: - Rules that specify which statements (instructions) are legal/valid or illegal/invalid Errors in syntax are found in compilation int x; //Line 1 int y //Line 2: error double z; //Line 3 y = w + x; //Line 4: error Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 4 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 2 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing Debugging: Fixing Syntax Errors During program compilation – Compiler will identify the syntax errors – Specifies the line numbers where the errors occur Example2_Syntax_Errors.cpp c:\chapter 2 source code\example2_syntax_errors.cpp(9) : error C2146: syntax error : missing ';' before identifier 'num' c:\chapter 2 source code\example2_syntax_errors.cpp(11) : error C2065: 'tempNum' : undeclared identifier Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 5 1.2 Semantics Semantics: – Set of rules that gives meaning to a language – Determine the meaning of the instructions – All syntax errors might be removed but the program fails to produce desired output Ex: 2 + 3 * 5 and (2 + 3) * 5 are both syntactically correct expressions, but have different meanings Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 6 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 3 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 2.0 Creating a C++ Program A C++ program is a collection of functions, one of which is the function main The first line of the function main is called the heading of the function: – int main() The statements enclosed between the curly braces ({ and }) form the body of the function Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 7 2.1 Statements All C++ statements end with a semicolon ; (statement terminator) { and } are not C++ statements but regarded as delimiters A C++ program contains two types of statements: – Declaration statements: To declare things, such as variables – Executable statements: To perform calculations, manipulate data, create output, accept input, etc. Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 8 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 4 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 2.2 Parts of C++ Program C++ program has two parts: – Preprocessor directives – The program Preprocessor directives and program statements constitute C++ source code (.cpp) Compiler generates object code (.obj) Executable code is produced and saved in a file with the file extension.exe Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 9 2.3 Preprocessor Directives C++ has a small number of operations Many functions and symbols needed to run a C++ program are provided as collection of libraries Every library has a name and is referred to by a header file Preprocessor directives are commands supplied to the preprocessor program All preprocessor commands begin with # No semicolon at the end of these commands Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 10 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 5 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing Preprocessor Directives (cont’d.) Syntax to include a header file: For example: #include – Causes the preprocessor to include the header file iostream in the program Preprocessor commands are processed before the program goes through the compiler Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 11 std namespace cin and cout are declared in the header file iostream, but within std namespace To use cin and cout in a program, use the following two statements: #include using namespace std; Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 12 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 6 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 3.0 A C++ Program Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 13 A C++ Program (cont’d.) Sample output: Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 14 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 7 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing A C++ Program (cont’d.) Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 15 A C++ Program (cont’d.) Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 16 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 8 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 3.1 Comments A well-documented program is easier to understand and modify Comments can be used to document programs Comments should appear in a program to: – Explain the purpose of the program – Identify who wrote it – Explain the purpose of particular statements Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 17 Comments (cont’d.) Comments are for the reader, not the compiler Two types: – Single line: begin with // // This is a C++ program. // Welcome to C++ Programming. – Multiple line: enclosed between Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 18 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 9 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 3.2 Reserved Words (Keywords) Reserved word symbols (or keywords): – Cannot be redefined within program – Cannot be used for anything other than their intended use Examples: – int – float – double – char – const – void – return Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 19 3.3 Special Characters Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 20 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 10 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 3.4 Special Symbols Token: The smallest individual unit of a program written in any language C++ tokens include special symbols, word symbols and identifiers Special symbols in C++ include: Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 21 3.5 Identifiers Identifier: Name of something that appears in a program – Consists of letters, digits, and the underscore character (_) – Must begin with a letter or underscore C++ is case sensitive – NUMBER is not the same as number Examples of predefined identifiers: cout and cin Unlike reserved words, predefined identifiers may be redefined, but it is not a good idea Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 22 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 11 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing Identifiers (cont'd.) Legal identifiers in C++: – first – conversion – payRate Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 23 Naming Identifiers Identifiers can be self-documenting: – CENTIMETERS_PER_INCH Avoid run-together words: – annualsale – Solution: Capitalizing the beginning of each new word: annualSale Inserting an underscore just before a new word: annual_sale Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 24 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 12 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 3.6 Whitespaces Every C++ program contains whitespaces; blanks, tabs and newline characters Used to separate special symbols, reserved words, identifiers and numbers (when data is input) Blanks must never appear within a reserved word or identifier Proper utilization of whitespaces is important to make the program more readable Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 25 4.0 Data Types Data type: Set of values together with a set of operations C++ data types fall into three categories: – Simple data type eg. int, bool, float, enum – Structured data type eg. array, struct, class – Pointers Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 26 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 13 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 4.1 Simple Data Types Three categories of simple data – Integral: integers (numbers without a decimal) Can be further categorized: – char, short, int, long, bool, unsigned char, unsigned short, unsigned int, unsigned long – Floating-point: decimal numbers – Enumeration type: user-defined data type Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 27 Simple Data Types (cont’d.) Different compilers may allow different ranges of values Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 28 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 14 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 4.2 int Data Type Examples: -6728 0 78 +763 Cannot use a comma within an integer – Commas are only used for separating items in a list Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 29 4.3 bool Data Type bool type – Two values: true and false – Manipulate logical (Boolean) expressions true and false – Logical values bool, true, and false – Reserved words Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 30 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 15 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 4.4 char Data Type The smallest integral data type Used for single characters: letters, digits, and special symbols Each character is enclosed in single quotes – 'A', 'a', '0', '*', '+', '$', '&' A blank space is a character – Written ' ', with a space left between the single quotes Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 31 char Data Type (cont’d.) Different character data sets exist ASCII: American Standard Code for Information Interchange – Each of 128 values in ASCII code set represents a different character – Characters have a predefined ordering based on the ASCII numeric value Collating sequence: Ordering of characters based on the character set code Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 32 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 16 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing ASCII Table Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 33 4.5 Floating-Point Data Types C++ uses scientific notation to represent real numbers (floating-point notation) Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 34 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 17 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing Floating-Point Data Types (cont’d.) float: represents any real number – Range: -3.4E+38 to 3.4E+38 (four bytes) double: represents any real number – Range: -1.7E+308 to 1.7E+308 (eight bytes) Minimum and maximum values of data types are system dependent Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 35 Floating-Point Data Types (cont’d.) Maximum number of significant digits (decimal places) for float values: 6 or 7 Maximum number of significant digits for double: 15 Precision: maximum number of significant digits – Float values are called single precision – Double values are called double precision Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 36 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 18 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 4.5 Data Types and Variables To declare a variable, must specify the data type it will store Syntax: dataType identifier; Examples: int counter; double interestRate; char grade; Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 37 4.6 string Type Programmer-defined type supplied in ANSI/ISO Standard C++ library Sequence of zero or more characters enclosed in double quotation marks Null (or empty): a string with no characters Each character has a relative position in the string – Position of first character is 0 Length of a string is number of characters in it – Example: length of "William Jacob" is 13 Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 38 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 19 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing Using the string Data Type To use the string type, you need to access its definition from the header file string Include the following preprocessor directive: #include or #include Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 39 5.0 Variable and Constant Variable: A memory location whose contents can be changed Memory allocation before initialization Memory spaces after the statement length = 6.0; executes Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 40 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 20 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 5.1 Defined Constant 2 types of constants: - A defined constant - A declared constant Defined Constant: A fixed value created using a preprocessor directive and works on textual substitution before program compilation. Syntax to define a constant: #define identifier value Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 41 5.2 Declared Constant Declared constant: A memory location whose content can’t be changed during execution. Syntax for a declared constant: In C++, const is a reserved word Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 42 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 21 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 5.3 Variables Declaration Consider two ways of declaring variables: – Method 1 int feet, inch; double x, y; – Method 2 int feet,inch; double x,y; Both are correct; however, the second is hard to read Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 43 5.4 Variables Initialization Not all types of variables are initialized automatically Variables can be initialized when declared: int first=13, second=10; char ch=' '; double x=12.6; All variables must be initialized before they are used – But not necessarily during declaration Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 44 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 22 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 6.0 Storing Data Into A Variable Data must be loaded into main memory before it can be manipulated Storing data in memory is a two-step process: – Instruct computer to allocate memory – Include statements to put data into memory Ways to place data into a variable: – Use C++’s assignment statement – Use input (read) statements Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 45 6.1 Assignment Statement The assignment statement takes the form: Expression is evaluated and its value is assigned to the variable on the left side A variable is said to be initialized the first time a value is placed into it In C++, = is called the assignment operator Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 46 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 23 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing Assignment Statement (cont’d.) Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 47 6.2 Input (Read) Statement cin is used with >> to gather input This is called an input (read) statement The stream extraction operator is >> For example, if miles is a double variable cin >> miles; – Causes computer to get a value of type double and places it in the variable miles Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 48 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 24 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing Input (Read) Statement (cont’d.) Using more than one variable in cin allows more than one value to be read at a time Example: if feet and inches are variables of type int, this statement: cin >> feet >> inches; – Inputs two integers from the keyboard – Places them in variables feet and inches respectively Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 49 Example Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 50 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 25 SIN 3011 / SIM 3025: 10/15/2024 Scientific Computing 7.0 Output The syntax of cout and