Web Programming Revision PDF
Document Details
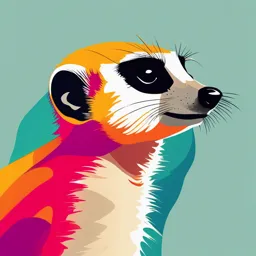
Uploaded by JudiciousYttrium5705
Tags
Related
- Buku Web Programming (PDF) - AQA 2019
- Unit 1. Web programming platforms in server environment. Characteristics of the PHP language V8.pdf
- Unit 1. Web programming platforms in server environment. Characteristics of the PHP language V8.pdf
- Web Programming Lec1 PDF
- BCA Web Programming Using PHP Past Paper (Nov 2022) PDF
- Module 1 - Web Programming Using PHP (CA4CRT13) PDF
Summary
This document provides revision material for web programming, covering topics such as binary representation, PHP concepts (variables, datatypes, functions, comments), and conditional statements. It is suitable for undergraduate students.
Full Transcript
Week 1 - Binary Numbers Binary is a way of representing informations using only 2 options: On (1), off ( 0). Computer use binary to process and store the data. By combining many one and offs into complex patterns. The patterns tells the computers What to preform. Computer language: - Computers d...
Week 1 - Binary Numbers Binary is a way of representing informations using only 2 options: On (1), off ( 0). Computer use binary to process and store the data. By combining many one and offs into complex patterns. The patterns tells the computers What to preform. Computer language: - Computers don't understand words of numbers like humans. Everything is represented by binary electrical signal that registers in one of two states: on or off. To make sense of complicated data, Week2- introduction to PHP coding Client side vs server side Client side (front-end): it's what happens in the browser. Includes - - layout design - interactivity of a website Main technologies used are (HTML, CSS, JavaScript) Server - side (back-end): it's what handled behind-the - scenes process. Includes - - managing databases - user authentication - server logic Technologies used (Server-side language (Python), PHP, Ruby, frameworks like node.js) Interaction between client-side and server-side 1. Request: When you type a URL in your browser and hit enter, your browser sends a request to the server. 2. Processing: The server processes this request, fetches the necessary data (like HTML, CSS, and JavaScript les), and sends it back to your browser. 3. Response: Your browser receives these les and renders the web page you see. Client-Side: Runs in the browser, handles user interface and interactivity. Example: Pop-up product details. Server-Side: Runs on the server, handles data processing and storage. Example: Processing an order at checkout. fi fi Types of PHP comments Single line comments // this is a single - line comment # Multi line comments PHP variable declaration rules 1- variables in PHP always begin with the ($) followed by the variable name. 2- The initial characters of a variable name should be a letter or an underscore. (Not a number or special character) 3- PHP variable names are case - sensitive,$ age and $ AGE represents 2 distinct variables. Assigning values to variables Assignment operator ( =) is used to assign a value to a variable. $ variablename = value; Example: $age = 25; or $ name = "Sara"; PHP datatypes overview Integer datatype: integers are whole numbers (positive or negative) without a decimal point. Example: $ intVar=42; $negIntVar=-42; Float datatype: oats are decimal numbers in PHP. Example: $ oatVar = 3.14; Boolean datatype: Boolean true or false in PHP coding. Example: $boolVar = true; String datatype: Strings: Sequences of characters in PHP A string is a non-numeric data type. It holds letters or any alphabets, numbers, and even special characters. String values must be enclosed either within single quotes or in double quotes Example: $stringVar = "Hello, World!"; Array datatype: array in PHP collection of indexed value. Example: $arrayVar=(1,2,3); Null datatype: $ nullvar = null; Printing variables : ?php $text = "Hello, World!"; $num1 = 10; $num2 = 20; // echoing the variables echo $text; echo $num1."+".$num2."="; echo $num1 + $num2; ? fl fl PHP Math Functions Content: 1. Addition: PHP $sum = 5 + 10; 2. Subtraction: PHP $difference = 10 - 5; 3. Multiplication: PHP $product = 5 * 10; 4. Division: PHP $quotient = 10 / 2; 5. Modulus: PHP $remainder = 10 % 3; PHP math function (coding) // Convert Decimal to Binary// echo decbin(8). ""; // Convert Binary to Decimal// echo bindec(1100). ""; // Find the maximum value// $numbers = array(10, 22, 43, 45, 32, 34); echo "The maximum value is: ". max($numbers). ""; // Math operations// echo 10 * 5. ""; echo 10 / 5. ""; echo 5 + 10. ""; // Random number between 1 and 100// echo rand(1, 100). ""; Research Activity 1. What is Client-Side scripting? 2. What is server-side scripting? 3. Client-side vs server-side (advantages and disadvantages)? 4. What are the programming languages that support each type? 5. When do we use Client-side and server-side, or do we need them together? Week3 - conditional statements and loops What are conditional statements? Conditional statements use ( if,then )logic to affect code execution based on certain conditions. If statements The 'if' statement enables selective code execution based on speci ed conditions, crucial for decision-making. How does it work if Statements Output will appear when only Condition must be true. Syntax Example if(condition) { code to be executed if condition is true; } Exercise 1 Description: Write a PHP program to check whether a number is positive, negative, or zero. Instructions: Use if... elseif... else conditions. You should use appropriate PHP Operators. Also check if it is not a numeric value. Hint: Use > 0 for checking if a number is positive. Use < 0 to check if a number is negative. Answer: fi Exercise 2 Description: Write a PHP program to check if a person is eligible to vote or not. Condition: Minimum age required to vote is 18. Use if... else statements. Exercise 3 Description: Write a PHP program to check if a customer is eligible for a discount or not. Conditions: Create a variable that has the value of the total purchase amount. Use if... else statements to check if the amount is more than 100. If the amount is more than 100, the customer will be eligible for a discount. Exercise 4 Description: Write a PHP program to check if the student should be under probation. Conditions: Create a variable that has the value of the student’s GPA. Use if... elseif... else statements. The if statement should check if the GPA is more than 3 and print the following message: “CONGRATS, YOU ARE DOING GREAT. KEEP IT UP”. The elseif statement should check if the GPA is more than 2.25 and print the following message: “Well Done”. The else statement should print the following message: “Study hard! You can do it”. 1- while loop Scenario: printing numbers from 1 to 10. Explanation: Initialization: The variable $num is initialized to 1. Condition Check: The loop continues as long as $num is less than or equal to 10. Code Execution: The current value of $num is printed, followed by a line break. Then, $num is incremented by 1. Additional Example: Scenario: Multiplying numbers by 2 until the result exceeds 100. Explanation: Initialization: The variable $multiply is initialized to 1. Condition Check: The loop continues as long as $multiply is less than or equal to 100. Code Execution: The current value of $multiply multiplied by 2 is printed, followed by a line break. Then, $multiply is incremented by 1. 2. Do-While Loop Scenario: Printing a message until a condition is met. Code Example: Explanation: Initialization: The variable $x is initialized to 1. Loop Execution: The do block is executed rst, printing the current value of $x and a message, followed by a line break. Then, $x is incremented by 1. Condition Check: After executing the do block, the while condition is checked. Since $xis not greater than 10, the loop stops after the rst iteration. 3. For Loop Scenario: Printing numbers from 1 to 3. Code Example: Explanation: Initialization: The loop starts by initializing the variable $i to 1. Condition Check: Before each iteration, the condition $i Explanation: Initialization: The loop starts by initializing the variable $i to 0. Condition Check: Before each iteration, the condition $i < $count is checked. If it’s true, the loop continues; otherwise, it stops. Code Execution: The code inside the loop prints the current fruit name, followed by a line break. Increment: After each iteration, the value of $i is incremented by 1. fi fi 4. Foreach Loop Scenario: Printing elements of an array. Code Example: Explanation: Initialization: The foreach loop starts by iterating over each element in the $fruits array. Code Execution: The code inside the loop prints the current fruit, followed by a line break. Additional Example: Scenario: Accessing elements of an associative array.