Computational Thinking and Programming PDF
Document Details
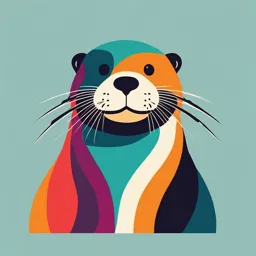
Uploaded by NavigableMetaphor
Department of Computer Science and Engineering
Tags
Related
- M5-CONDITIONALS PDF - If and If-Else Statements
- Lecture 03: Flow Control PDF
- Programming Operators and Control Statements (PDF)
- SYSC 2006: Imperative Programming Lecture 3 - Flow of Control (Carleton University)
- Control Statements If - Theory Notes PDF
- Hyrje në Shkenca Kompjuterike dhe Programim PDF
Summary
This document provides an introduction to computational thinking and programming, focusing on conditional statements and control flow. It covers different types of conditional statements, including if, if-else, nested if-else, else-if ladders, and switch case statements.
Full Transcript
Computational Thinking and Programming UNIT-II Syllabus: _________________________________________________________________________________________________________ Computational Problems: if, if-else, nested if, switch-case, ternary operator, got...
Computational Thinking and Programming UNIT-II Syllabus: _________________________________________________________________________________________________________ Computational Problems: if, if-else, nested if, switch-case, ternary operator, goto, Iteration with for, while, do- while, break, continue Standard problems GCD, finding roots, generating factors of a number, Checking for leap year Number series problems Fibonacci series, natural numbers, even numbers, prime numbers, multiplication table, palindrome numbers. __________________________________________________________________________________________________________________ Introduction: basically a C program is a set of statements which are usually executed successively. But in practice, we have a number of situations where we may have to change the order of execution of statements based on certain conditions, or replicate a group of statements until certain specified circumstances are met. The general form of a standard decision making structure found in most of the programming languages like C is as follows: C language handles decision-making by supporting the following Conditional Control statements: 1) if statement 2) if-else statement 3) nesting if-else statement 4) else-if ladder statement 5) switch case statement Depending on the complexity of conditions to be tested we can implement if-stmt in different forms they are: - simple if, if-else, nested if-else, else-if ladder. Department of Computer Science & Engineering Page: 1 Computational Thinking and Programming 1. Simple if: the general syntax to use simple if statement is: Syntax: - if (Test Expression) { Statement-Block; } Statement-n; Flow diagram of simple if statement Working: If the Expression is True the Stmt block will be Executed. If the Expression is False, the Stmt –block will be skipped and the Execution will jump to the Statement-n Note: - In this case if the condition is true both the Statement-Block & Statement- X gets Executed in Sequence. 2. if else statement: the general syntax of using if else statement: syntax: if(Test Expression) { True Statements-Block; } else { False Statements-Block; } Statement-n; Department of Computer Science & Engineering Page: 2 Computational Thinking and Programming Flow diagram of if-else statement Working: If Expression is True, then the True Statements-Block will be Executed which are immediately followed by if. If the Expression is False, then the False Statements-Block are Executed. However, the Statement-n gets Executed after that. 3. Nesting if-else statement In practical, sometimes, a choice has to be made from more than two possibilities. When sequences of decisions are concerned, we may have to apply more than one if-else statement in nested form. Nested if-else statement is similar to if-else statement, where new block of if-else statement is defined in existing if or else block statement. The nested if-else statement allows us to verify for multiple test expressions and execute different codes for more than two conditions. Department of Computer Science & Engineering Page: 3 Computational Thinking and Programming syntax: if(Test Expression1) { if(Test Expression2) { Block of Statement-1; } else { Block of Statement-2; } } else { Block of Statement-3; } Statement-n; Working: Expression1 will be Evaluated first. If Expression1 is Evaluated to true then we enter into Outer if- Block, then Expression2 will be Evaluated: - o if Expression2 is Evaluated to True then the Inner if-block statemets (i.e) Block of Statement-1 will be Executed. o if Expression2 is Evaluated to False then the Inner else-block Statements (i.e) Block of Statement-2 will be Executed. If Expression1 is Evaluated to False then we enter into Outer else-block Statements (i.e) Block of Statement-3 will be Executed. At last Statement-n will be Executed After that. Department of Computer Science & Engineering Page: 4 Computational Thinking and Programming Flow diagram of nesting if-else statement 4. else-if ladder when we need to test different conditions with different statements, where multipath decisions are involved, this is called else-if ladder. Actually multipath decision is a sequence of ifs in which the statements related with each else is an if. If-else ladder statement is used to test set of conditions in sequence that is if we have different test conditions with different statements then we need else-if ladder statements. The general syntax of if-else ladder is as follows: if(Test_Expression1) Statement-1; else if(Test_Expression2) Statement-2; else if(Test_Expression3) Statement-3; else if(Test_Expression4) Statement-4; ……… else if(Test_Expression N) Department of Computer Science & Engineering Page: 5 Computational Thinking and Programming Statement-N; else default-Statement; Statement-X; Flow diagram of else-if ladder statement Working: First Test_Expression1 will be Evaluated first. If that Test_Expression1 is True then, Statement-1 will be Executed. If the Test_Expression1 is Evaluated to false then, Test_Expression2 is Evaluated. If Test_Expression2 is Evaluated to True then, Statement-2 will be Executed Otherwise We test Test_ Expression3 so on up to Test_Expression N. If N Expressions are False then, the default-Statement of else block will be Executed. However Statement-X will be executed after that. Department of Computer Science & Engineering Page: 6 Computational Thinking and Programming 5. Switch case statement If we are checking on the value of a single variable in nested if –else statement, in this situation it is better to use switch case statement. It is frequently faster than nested if-else but not always. Fortunately, C has built-in multi-way decision statement known as switch statement. A switch statement tests the value of a given variable or expression against a list of case values. In this statement, when case is matched or found then block of statements associated with that case is executed. This type of statement is mostly used when we have number of options or choices or cases and we may need to perform a different task for each choice or case. The general syntax of switch case statement is as follows: switch(Test_Expression) { case value/constant-1; block1; break; case value/constant-2 block2; break; case value/constant-3 block3; break; case value/constant-N blockN; break; ………. default default-Block; break; } Statement-X; Department of Computer Science & Engineering Page: 7 Computational Thinking and Programming Flowchart of switch case statement Working: The expression is an integer or characters. Value-1,value-2,…..value-N are constant or constant Expression. Also known as CASE LABELS. o each case labels should be unique in a switch statement. When switch is executed the value of the expression is successfully compared against the values value-1, value-2,…value-N. if case values match with the value of the expression then the block of statements that follows the case are executed. The break statement at the end of each block signals the end of a particular case and causes an exit from the switch statement, transferring the control to the Statement-X following the switch. The default gets executed if the case value doesn’t match with expression value. Note:- Nearly 257 case labels can be used with a switch case Statement. Department of Computer Science & Engineering Page: 8 Computational Thinking and Programming Unconditional Control Statements: (1) The break Statement: A break statement terminates the execution of the loop and the control is transferred to the statement immediately following the loop. i.e., the break statement is used to terminate loops or to exit from a switch. It can be used within for, while, do-while, or switch statement. The break statement is written simply as break; In case of inner loops, it terminates the control of inner loop only. Syntax: Jump-statement; break; The jump statement in c break syntax can be while loop, do while loop, for loop or switch case. Flowchart: (2) The continue Statement: The continue statement is used to bypass the remainder of the current pass through a loop. The loop does not terminate when a continue statement is encountered. Instead, the remaining loop statements are skipped and the computation proceeds directly to the next pass through the loop. The continue statement can be included within a while, do-while, for statement. It is simply written as “continue”. The continue statement tells the compiler “Skip the following Statements and continue with the next Iteration”. In “while” and “do” loops continue causes the control to go directly to the test –condition and then to continue the iteration process. In the case of “for” loop, the updation section of the loop is executed before test-condition, is evaluated. Department of Computer Science & Engineering Page: 9 Computational Thinking and Programming Syntax: Jump-statement; Continue; The jump statement can be while, do while and for loop. Flowchart: Flow chart of continue statement (3) The “goto” Statement: C supports the “goto” statement to branch unconditionally from one point to another in the program. Although it may not be essential to use the “goto” statement in a highly structured language like “C”, there may be occasions when the use of goto is necessary. The goto requires a label in order to identify the place where the branch is to be made. A label is any valid variable name and must be followed by a colon (: ). The label is placed immediately before the statement where the control is to be transferred. The label can be anywhere in the program either before or after the goto label statement. Syntax : goto label;....................................... label: statement; Department of Computer Science & Engineering Page: 10 Computational Thinking and Programming In this syntax, label is an identifier. When, the control of program reaches to goto statement, the control of the program will jump to the label: and executes the code below it. goto label;....................................... label: statement; Forward Jump label: Statement;....................................... goto label; Backward Jump Flow chart of goto statement (4) Return Statement: The return statement terminates the execution of a function and returns control to the calling function. Execution resumes in the calling function at the point immediately following the call. A return statement can also return a value to the calling function. Syntax: Jump-statement: return expression; Department of Computer Science & Engineering Page: 11 Computational Thinking and Programming Looping/ Iterative Statements: Executing a single statement or block of statements repeatedly for the required number of times. Such kind of problems can be solved using looping statements. The looping statements are used to execute a single statement or block of statements repeatedly until the given condition is FALSE C language provides three looping statements... while statement do-while statement for statement While Statement: The while statement is used to execute a single statement or block of statements repeatedly as long as the given condition is TRUE. The while statement is also known as Entry control looping statement. The while statement has the following syntax. Syntax: while(condition) { ------; Block of statements; ------; } Flow of while loop is as follows At first, the given condition is evaluated. If the condition is TRUE, the single statement or block of statements gets executed. Once the execution gets completed the condition is evaluated again. If it is TRUE, again the same statements get executed. The same process is repeated until the condition is evaluated to FALSE. Department of Computer Science & Engineering Page: 12 Computational Thinking and Programming Whenever the condition is evaluated to FALSE, the execution control moves out of the while block. while is a keyword so it must be used only in lower case letters. If the condition contains a variable, it must be assigned a value before it is used. The value of the variable used in condition must be modified according to the requirement inside the while block. In a while statement, the condition may be a direct integer value, a variable or a condition. A while statement can be an empty statement. Do-while statement: The do-while statement is used to execute a single statement or block of statements repeatedly as long as given the condition is TRUE. The do-while statement is also known as the Exit control looping statement. The do-while statement has the following syntax Syntax: do { ------; Block of statements; ------; } While(condition); Flow of do-while loop is as follows Department of Computer Science & Engineering Page: 13 Computational Thinking and Programming At first, the single statement or block of statements which are defined in do block are executed. Once the execution gets completed again the condition is evaluated. If it is TRUE, again the same statements are executed. The same process is repeated until the condition is evaluated to FALSE. If the condition contains a variable, it must be assigned a value before it is used. In a do-while statement, the condition may be a direct integer value, a variable or a condition. A do-while statement can be an empty statement. In do-while, the block of statements is executed at least once. For statement: The for statement is used to execute a single statement or a block of statements repeatedly as long as the given condition is TRUE. The for statement has the following syntax and execution flow diagram... Syntax: for(initialization; condition;modification) { ------; Block of statements; ------; } Flow of for loop is as follows Department of Computer Science & Engineering Page: 14 Computational Thinking and Programming for is a keyword so it must be used only in lower case letters. Every for statement must be provided with initialization, condition, and modification (They can be empty but must be separated with ";") Ex: for ( ; ; ) or for ( ; condition ; modification ) or for ( ; condition ; ) In for statement, the condition may be a direct integer value, a variable or a condition. The for statement can be an empty statement. Standard Problems: 1. Write a C Program to find GCD of two numbers. Program: #include void main() { int num1,num2,gcd=0,i; printf("Enter two numbers num1 & num2 to find GCD: "); scanf("%d%d",&num1,&num2); for(i=1;i