Software Engineering PDF
Document Details
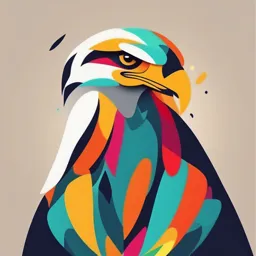
Uploaded by SweetNovaculite3606
Maastricht University
Tags
Summary
This document provides an introduction to software engineering and architecture. It covers concepts such as the engineering design process, and differences between science and engineering. Software engineering is introduced as a way to solve real-world problems using science and mathematics.
Full Transcript
Software Engineering 1 Introduction to Software Engineering and Architectures 2 Introduction This lecture introduces key aspects of software engineering and architecture. The course focuses on understanding how companies manage large-scale software projects, rather than on coding practices...
Software Engineering 1 Introduction to Software Engineering and Architectures 2 Introduction This lecture introduces key aspects of software engineering and architecture. The course focuses on understanding how companies manage large-scale software projects, rather than on coding practices or architecture design. 2.1 Class Structure Lectures: Held on Monday, Tuesday, and Friday, focusing on methodologies for large-scale projects. No Labs: Theoretical material doesn’t translate well into a lab format; hands-on experience will come from other courses. 3 What is Engineering? Definition: Engineering applies science and math to solve real-world problems, aiming to create practical solutions. Engineering vs. Science: – Science is about answering questions through investigation. – Engineering focuses on building solutions based on learned principles. – Informally: ”Scientists build to learn, while engineers learn to build.” 3.1 The Engineering Design Process Engineers often follow a loosely structured process to develop solutions: 1. Define the Problem: Identify the specific problem or need. 2. Do Background Research: Gather relevant information and context. 3. Specify Requirements: Define the requirements the solution must meet. 4. Create Alternative Solutions, Choose the Best, and Develop It: Explore different ap- proaches and select the most viable. 5. Build a Prototype: Develop a working model to test the concept. 6. Test and Redesign as Necessary: Refine the prototype based on feedback and testing results. 7. Communicate Results: Share the solution and findings with stakeholders. 1 4 What is Software Engineering? Definition: Software engineering applies engineering principles to software development, aiming to solve problems through the creation of software solutions. Differences from Traditional Engineering: – Software lacks physical constraints, making it infinitely adaptable and complex. – Unlike physical sciences, there are no natural laws governing software behavior. – Software is ”invisible” and malleable, lacking models that simplify its structure (e.g., ball- and-stick models in chemistry). Challenge: The flexibility of software leads to endless potential solutions, making it hard to compare alternatives or reject changes during development. 5 What is Professional Software Engineering? Definition: Professional software engineering occurs within a for-profit organization, focusing on project profitability within business constraints. Contextual Factors: – Software engineering in companies is influenced by hierarchy, budget, legal issues, and orga- nizational structure. – A key goal is to create profitable, maintainable software within a fixed timeframe. Challenges: – Large teams and projects require coordination across many roles and departments. – Issues like people management, budgeting, and deadline adherence add complexity beyond the technical aspects of software creation. 6 The Challenges of Large-Scale Software Engineering Scale and Complexity: – Managing a project with 500 developers differs vastly from managing one with five. – Large codebases (e.g., millions of lines) are harder to coordinate, maintain, and ensure quality in. Lack of Formal Theory: – Software engineering lacks a strict, unified theory, relying instead on ”rules of thumb” and empirical guidelines. – This lack of rigor can be frustrating, especially for those used to exact answers and logical proofs common in computer science. Informal Approach: While software engineering may seem imprecise, these methods have proven effective in real-world applications, similar to early architecture (e.g., Notre Dame Cathedral) built without modern physics. 7 Conclusion and Key Takeaways This course will explore how companies structure and manage software projects for scalability and profitability. While software engineering may lack a strict theory, it is essential for producing viable, scalable systems. The field combines elements of art, science, and business, preparing students to navigate complex, real-world projects. 2 8 Lifecycle Families 9 Introduction This lecture covers the key aspects of software lifecycle models, exploring the common lifecycle families and how they structure software projects. Topics include the roles of project members, phases of software development, and rules governing lifecycle transitions. 10 Projects Definition: A project consists of a group of people working together to achieve a specific goal. Role Assignment: Project membership is generally determined by accounting; if you’re paid under a project’s budget, you’re considered part of it. Project Activities: Members perform various tasks based on project requirements, contributing to different phases of development. Focus of the Course: This course will detail: – Phases: What phases exist, and what happens during each. – Lifecycles: Different models showing how phases are combined in project execution. 11 Phases Phases represent grouped activities with specific goals, timing, and roles within a project. Definition: A phase, or stage, is a set of related activities happening around the same time, involving specific team members. Characteristics: Phases are defined by: – Activities: The actions that occur within the phase. – Participants: The team members involved at each stage. Example - School Project: 1. Planning: Define objectives, read problem descriptions, assign tasks. 2. Coding: Write code, use version control. 3. Testing: Ensure the demo or final project functions as expected. Project Phase Control: – Not everyone on a project has to be in the same phase at once. – Phases provide control, preventing disorganized or unproductive work. 12 Lifecycles A software lifecycle is defined as a set of phases and rules governing transitions between them. Purpose: Lifecycles define the current project focus and indicate the possible next steps. Lifecycle Families: Lifecycles are grouped into families, or models, sharing similar phases and transition rules. Practical Application: Few projects use a pure lifecycle model; adaptations are common to meet specific needs. 3 13 The Waterfall Model The Waterfall model represents a linear approach to project management, where phases follow a strict, sequential order. Structure: The project is always in one defined phase at a time, progressing forward without returning to previous phases. Common Phases: – Initial Planning: Define requirements, timeline, and resources. – Design: Outline large-scale solution elements. – Implementation: Build the solution. – Delivery: Deploy the solution and provide ongoing support. Rules for Phase Changes: – Each phase must complete before the next begins. – No phase is revisited once it’s completed. – The project progresses according to a defined schedule. Outcome: At the end, a complete solution is delivered. 14 Iterative Models Iterative models allow for more flexibility by enabling transitions between phases, including backward movement. Characteristics: – Iterative models permit moving back to previous phases, especially useful for incremental development. – This flexibility is often used when revisiting previous stages to refine or improve parts of the system. Example: Iterative models may involve returning from later stages (like testing) back to earlier phases to adjust features. Visual Example: Circular iterative models visually emphasize this flexibility and the cyclical nature of development. 15 Agile Models Agile models focus on adaptability, customer feedback, and frequent, incremental improvements. Characteristics: – Agile models prioritize minimal upfront planning. – Phases are dynamic, and coding and testing are often intermingled. Agile Philosophy: – Develop a system based on current understanding. – Gather feedback and use it to refine the system. – Continue until the customer is satisfied or resources are exhausted. Rules for Phase Changes: – Phases shift as needed to make progress. – Different teams may work in separate phases simultaneously. – Projects often lack a defined endpoint; they end once the customer is content with the product. 4 16 Summary This lecture provided a high-level overview of project phases and lifecycle families. Future sessions will: – Detail individual phases in the order of the Waterfall model. – Explore issues in Waterfall and how incremental models attempt to address them. – Investigate Agile and its distinctive approach to flexibility and iterative improvements. 17 Requirements 18 Introduction This lecture covers the fundamentals of requirements and requirements engineering, detailing what re- quirements are, their types, and the end goal of defining and refining these requirements. 19 What Are Requirements? Definition: Requirements specify what the system must accomplish and the constraints within which it must operate. Requirements Document: – Requirements are gathered into a formal document, often used as a contract basis. – Example: The customer agrees to pay an amount if the system is delivered by a particular date with specified functionalities. Detailed View of Requirements: – Requirements should be abstract enough to allow multiple contractors to offer solutions but clear enough to meet client needs. – Once a contract is awarded, the contractor refines the system definition to confirm under- standing with the client. 19.1 The Process of Gathering Requirements Requirements Gathering: Initial ideas may be vague and involve extensive discussions with the client. Example - McDonald’s Kiosks: A vague idea like “allow customers to place orders on a screen instead of waiting in line” evolves through refinement to specify actual functionalities. 20 Requirements Engineering Definition: The process of refining vague ideas into a concrete, agreed-upon set of requirements that the customer and provider both accept. Participants: Senior team members, management, accountants, and lawyers are typically in- volved. Challenges: This phase requires technical skill, negotiation, and people skills due to the complex task of understanding and documenting what the client wants. Risk of Failure: A poorly executed requirements phase may lead to contract issues, unmet deliverables, or lawsuits. 5 21 Types of Requirements Requirements are classified into functional and non-functional, each with distinct implications. 21.1 Functional Requirements Definition: Statements of what the system should provide, including responses to inputs and particular behaviors. Examples: – “The system must display available menu items.” – “Items should be grouped into categories like ‘burgers’ and ‘chicken’.” – “Users can choose one item at a time.” 21.2 Non-Functional Requirements Definition: Constraints on how the system operates, such as timing or standards compliance. Examples: – “Response time must be under 0.5 seconds.” – “System must not crash when a user cancels an order.” 22 Distinguishing Functional and Non-Functional Requirements Ambiguity in Distinction: – Some requirements, like “System must process MasterCard transactions within 5 seconds,” may appear as both functional (process transactions) and non-functional (time constraint). Rule of Thumb: If it describes system behavior, it’s likely functional; if it’s a limitation on implementation, it’s non-functional. Example: – Accepting credit cards is functional. – Processing within 5 seconds is non-functional. Complex Scenarios: – Example: Integrating with McDonald’s existing order system could be functional (system interaction) and non-functional (compatibility constraint). – Note: When distinctions are unhelpful, drop them as they may reflect design or implementa- tion decisions. 23 Metrics for Non-Functional Requirements Non-functional requirements can be measured even if they don’t align with specific code locations. Examples of Non-Functional Metrics: – Speed: Transactions per second, response time. – Size: Megabytes, number of ROM chips. – Ease of Use: Training time, number of help frames. – Reliability: Mean time to failure, rate of failure. – Robustness: Time to restart after failure, probability of data corruption. – Portability: Percentage of platform-dependent statements. 6 24 Use Cases Use cases are central to the requirements document, outlining possible system interactions. Definition: A use case describes how a user can interact with the system to accomplish a task. Components: Include actors, interactions, and scenarios. Formats: Can be simple text or formal UML diagrams. Examples: – A mobile banking app use case: actors (customers, underwriters), goal (account setup), trigger (new account selection). 25 Use Case Templates and Scenarios Template Elements: – Primary Actor: Main user initiating the action. – Goal: Desired outcome for the actor. – Preconditions: Conditions required before the use case. – Trigger: Action that initiates the use case. – Scenario: Steps and interactions. – Exceptions: Errors and corrective actions. Filled Example: – System: Mobile banking app. – Primary Actor: Customer opening an account. – Goal: Simplify account registration. – Stakeholders: CEO and VP of Product. 26 The End Result Purpose of Requirements Phase: – Define a testable understanding of system functionality and constraints. – Validation differs for functional (e.g., visible actions) and non-functional requirements (e.g., time performance). Use Case Variants: – Use cases often evolve, adding scenarios for error handling and edge cases. – Example: A banking app includes normal (successful account creation) and error scenarios (network failure). 27 Requirements Engineering 28 Introduction This lecture explores the process of requirements engineering, covering specifications, requirements gath- ering methods, and the phases involved in refining and validating requirements. 7 29 Specifications Definition: Specifications are constraints that apply to the system, often unrelated to specific behaviors but impacting possible solutions. Distinction from Requirements: Requirements focus on what the system should do (behavior), while specifications limit how it can operate. Example: McDonald’s kiosks might require a specific display size, constraining design choices but not affecting the functional behavior. Additional Notes: – Specifications may include legal or technical requirements that impact design. – Distinguishing specifications from requirements can prevent design limitations from being overlooked. 30 Phases of Requirements Engineering Requirements engineering is often iterative, revisiting phases to gather more detail: Elicitation: Collect initial requirements from stakeholders. Analysis: Refine and evaluate requirements for completeness and consistency. Validation: Confirm with decision-makers that requirements match system needs. Documentation: Record the final requirements in a structured document. Management: Address inevitable changes to requirements over time. 31 Elicitation Purpose: Identify stakeholders and gather context on the application domain, system require- ments, and constraints. Types of Stakeholders: – Direct: Business owners, customers. – Indirect: Maintenance staff, employees interacting with the system. Methods: – Interviews: Direct discussions with stakeholders to capture requirements. – Ethnography: Observing business processes to understand real-world needs. 32 Analysis Objective: Resolve conflicts between stakeholder ideas and derive a cohesive set of requirements. Challenges: – Stakeholders often have conflicting goals or views. – Example: Cashiers may follow informal processes that differ from formal rules, leading to inconsistencies. Business Process Reengineering: – Examining workflows to align real-world practices with system requirements. Tools for Analysis: – Use Cases: Define actors and their interactions with the system. – User Stories: Describe requirements from the user’s perspective. 8 32.1 Use Cases and User Stories Use Cases: – Format: Actors, interactions, goals. – Example: Customer selects items on a kiosk, views a menu, and places an order. User Stories: – Format: ”As a [role], I want to [action] because [reason].” – Example: ”As a customer, I want to order a Big Mac because I am hungry.” – User stories provide a simple, benefit-focused view of each feature. 33 Validation Purpose: Confirm requirements with stakeholders to ensure they reflect system goals. Validation Criteria: – Comprehensibility: Is the requirement clear? – Verifiability: Can the requirement be realistically tested? – Traceability: Can the requirement’s origin be tracked? – Adaptability: Can changes be made with minimal impact? 34 Documentation Version Control: Document requirements with updates to capture changes over time. Purpose: Serves as a permanent record of agreed requirements. Final Document: Includes functional and non-functional requirements, specifications, and stake- holder agreements. 35 Requirements Management Goal: Handle inevitable changes in requirements as the project evolves. Approach: Requirements management controls additions or removals to the requirements docu- ment in response to changing customer needs or project constraints. 36 The Final Document Definition: The software requirements document, also called “reqs,” provides a clear statement of system requirements. Purpose: Clearly defines functional and non-functional requirements for developers and stake- holders, focusing on what the system should do rather than how it should do it. 37 Users of a Requirements Document The requirements document is used by multiple project stakeholders: Developers: Understand system requirements for implementation. Testers: Define testing criteria based on requirements. Project Managers: Track project progress and ensure alignment with goals. Clients: Ensure the final product aligns with their needs. 9 38 Management 39 Introduction This lecture covers the fundamentals of software project management, including its purpose, the resources involved, the organizational setup, and common challenges faced during the process. 40 What is Software Project Management? Definition: Software project management is the process of planning, implementing, monitoring, and controlling software projects to achieve project goals within constraints. Understanding Project Management: – Project management involves supervising a team to reach project goals within given con- straints. – Management primarily deals with people and organizational challenges rather than technical issues. Key Aspects of Management: – Teams: Management is team-based, involving different personalities and goals, leading to interpersonal conflicts. – Supervision: Managers have authority to assign tasks, discipline, reward, and make hiring or firing decisions. – Orders from Above: Managers receive goals and constraints (scope, time, and budget) from higher management, often without being part of the requirements creation. Goals and Constraints: – The goal is to deliver software that meets requirements, also known as the final deliverable. – Constraints include scope, time, and budget, all outlined in project requirements and plans. 41 Management Tools Managers have access to resources like budget, personnel, and company facilities to help achieve project goals. 41.1 Budget Role of Budget: Defines the project’s scope and feasibility; without a budget, it’s a hobby. Budget Allocation: – Managers receive periodic budgets. – Expenditures are justified in quarterly and yearly reports. 41.2 Personnel Hiring: – Managers hire based on project requirements, although regulations and commitments compli- cate hiring. – Internal approvals may be needed, and finding the right skills can be challenging. 10 41.3 Access to Company Resources Departments: Legal, marketing, HR, and other company departments can support the project. Facilities: Managers also have access to equipment, desks, and necessary spaces, often within budget constraints. 42 Organization Setup Phase: Team organization is established, including choosing a lifecycle model (e.g., Agile, Waterfall) and assigning roles. Scheduling: An initial project schedule is set, defining milestones and early deadlines. 43 Work Initial Phase: – A small team (manager and senior technical staff) begins foundational work. – Early tasks may involve creating prototypes or demos to test ideas and lock down core func- tionalities. Full Team Engagement: – As the project progresses, the full team works on implementing all required functionalities. – Senior members tackle technical decisions and unforeseen challenges. Progress Monitoring: – Measuring progress is difficult in software projects, as there are no physical metrics like in other fields. – Managers submit frequent status reports, despite challenges in assessing true progress. Challenges of Managing Programmers: – Programmers may resist direction due to their expertise, making management complex. – Technical conflicts are hard to resolve, especially if managers lack the technical knowledge to mediate effectively. Motivating Teams: – Managers often rely on team members’ desire to succeed and fear of failure to keep the project on track. – Success is often driven by the team’s investment in the project, with a common belief that projects succeed if “willed or loved” into existence. 44 Results High Failure Rate: A significant percentage of software projects fail to meet criteria like on-time delivery, budget adherence, scope completion, and bug-free operation. Flexible Success Criteria: – Projects are more likely to be deemed successful if criteria are relaxed (e.g., allowing minor bugs or delays). – Large projects, like games, often fail to meet strict success criteria even under ideal conditions (experienced teams, clear deadlines). Statistics on Project Failure Rates: – Informal estimates put failure rates as high as 83 – Studies by Gartner and Standish Group suggest failure rates around 66-80 – IEEE data reports lower rates (10-15 11 45 Management 46 Introduction This lecture covers the fundamentals of software project management, including its purpose, the resources involved, the organizational setup, and common challenges faced during the process. 47 What is Software Project Management? Definition: Software project management is the process of planning, implementing, monitoring, and controlling software projects to achieve project goals within constraints. Understanding Project Management: – Project management involves supervising a team to reach project goals within given con- straints. – Management primarily deals with people and organizational challenges rather than technical issues. Key Aspects of Management: – Teams: Management is team-based, involving different personalities and goals, leading to interpersonal conflicts. – Supervision: Managers have authority to assign tasks, discipline, reward, and make hiring or firing decisions. – Orders from Above: Managers receive goals and constraints (scope, time, and budget) from higher management, often without being part of the requirements creation. Goals and Constraints: – The goal is to deliver software that meets requirements, also known as the final deliverable. – Constraints include scope, time, and budget, all outlined in project requirements and plans. 48 Management Tools Managers have access to resources like budget, personnel, and company facilities to help achieve project goals. 48.1 Budget Role of Budget: Defines the project’s scope and feasibility; without a budget, it’s a hobby. Budget Allocation: – Managers receive periodic budgets. – Expenditures are justified in quarterly and yearly reports. 48.2 Personnel Hiring: – Managers hire based on project requirements, although regulations and commitments compli- cate hiring. – Internal approvals may be needed, and finding the right skills can be challenging. 12 48.3 Access to Company Resources Departments: Legal, marketing, HR, and other company departments can support the project. Facilities: Managers also have access to equipment, desks, and necessary spaces, often within budget constraints. 49 Organization Setup Phase: Team organization is established, including choosing a lifecycle model (e.g., Agile, Waterfall) and assigning roles. Scheduling: An initial project schedule is set, defining milestones and early deadlines. 50 Work Initial Phase: – A small team (manager and senior technical staff) begins foundational work. – Early tasks may involve creating prototypes or demos to test ideas and lock down core func- tionalities. Full Team Engagement: – As the project progresses, the full team works on implementing all required functionalities. – Senior members tackle technical decisions and unforeseen challenges. Progress Monitoring: – Measuring progress is difficult in software projects, as there are no physical metrics like in other fields. – Managers submit frequent status reports, despite challenges in assessing true progress. Challenges of Managing Programmers: – Programmers may resist direction due to their expertise, making management complex. – Technical conflicts are hard to resolve, especially if managers lack the technical knowledge to mediate effectively. Motivating Teams: – Managers often rely on team members’ desire to succeed and fear of failure to keep the project on track. – Success is often driven by the team’s investment in the project, with a common belief that projects succeed if “willed or loved” into existence. 51 Results High Failure Rate: A significant percentage of software projects fail to meet criteria like on-time delivery, budget adherence, scope completion, and bug-free operation. Flexible Success Criteria: – Projects are more likely to be deemed successful if criteria are relaxed (e.g., allowing minor bugs or delays). – Large projects, like games, often fail to meet strict success criteria even under ideal conditions (experienced teams, clear deadlines). Statistics on Project Failure Rates: – Informal estimates put failure rates as high as 83 – Studies by Gartner and Standish Group suggest failure rates around 66-80 – IEEE data reports lower rates (10-15 13 52 Implementation 53 Introduction This lecture explores the implementation phase in software development, focusing on key concepts such as reuse, coupling, cohesion, configuration management, and software as a communication tool. 54 What is Implementation? Definition: Implementation is the phase where the design document is translated into working code. The groundwork from previous phases (design, requirements) simplifies coding by specifying components, APIs, error conditions, and processes. Simplification of Coding: Previous phases intentionally reduce the complexity of the coding process. Hierarchy in Decision-Making: – Skilled and creative roles (like design) are more complex and better paid within corporate hierarchies. – Design documents leave some decision-making for developers to implement directly in code. – Good coding practices (e.g., SOLID principles, meaningful variable names) remain essential, but the context expands to business needs. 55 Reuse Reuse involves decisions on using existing software components or developing new ones. Also known as ”build-vs-buy.” Importance: Most modern software relies heavily on reused components. Decision Factors: Deciding on reuse hinges on cost-effectiveness and project requirements. Levels of Reuse: – Abstraction Reuse: Reusing design patterns or algorithms, like tree traversals. – System Reuse: Adopting entire systems, often via APIs (e.g., open-source software). – Component Reuse: Reusing libraries or modules (e.g., JavaFX for graphics). – Object Reuse: Reusing specific objects or methods (e.g., Java’s List class). – Code Reuse: Copying code, such as from Stack Overflow, with attention to licensing. Pros and Cons of Reuse: – Benefits: Saves time, utilizes tested solutions, and often provides fixed costs. – Drawbacks: Risks include compatibility issues, dependency on third-party updates, and potential for hidden bugs. 56 Coupling Coupling measures how dependent components are on each other. Low coupling is preferred to enhance modularity. Types of Coupling (from least to most coupled): – Data Coupling: Modules share data solely through parameters. – Stamp Coupling: Modules share a composite data structure, but each module only uses part of it. 14 – Control Coupling: One module influences another’s behavior through control flags. – External Coupling: Dependencies on external protocols or formats. – Common Coupling: Modules access shared global data. – Content Coupling: A module directly modifies or relies on another module’s data, creating the highest dependency. 57 Cohesion Cohesion measures how well elements within a module fit together, with high cohesion being preferable for maintainability. Types of Cohesion (from highest to lowest): – Functional Cohesion: Elements perform a single, focused task. – Sequential Cohesion: Output of one element serves as input for another. – Communicational Cohesion: Elements operate on the same data or contribute to a single goal. – Procedural Cohesion: Elements must occur in a specific order for the desired outcome. – Temporal Cohesion: Elements execute together due to timing needs rather than functional connection. – Logical Cohesion: Elements are related logically, not necessarily by function. – Coincidental Cohesion: Elements are unrelated, grouped without a clear purpose. 58 Summary of Coupling and Cohesion Guidelines: High cohesion and low coupling are generally ideal. Rule of Thumb: The more external elements needed to describe a module, the more coupled it is; multiple explanations for a module’s purpose often indicate low cohesion. 59 Implementation (Part 2) 60 Introduction This lecture delves into advanced aspects of implementation, focusing on configuration management, the complexity of building large systems like Windows, versioning, and software as a communication tool. 61 Configuration Management Definition: Configuration management involves managing code changes and ensuring consistency across a team, especially in large projects. Necessity of Configuration Management: – For individual projects, regular backups are typically sufficient. – For group projects, a single repository (e.g., Git) is helpful. – For large-scale projects, configuration management is essential as not all team members can communicate directly. Example - Windows Development: – Windows codebase contains approximately 3.5 million files and 50 million lines of code. – Maintained by around 4,000 engineers, with daily averages of 8,421 pushes, 2,500 pull requests, and 1,760 builds. – Microsoft developed a custom file system to support this repository. 15 62 Building Windows Process: – Engineers work on local branches, submitting pull requests to team branches. – Team branches are tested rigorously before merging into higher-level branches. Challenges: – With over 8,000 pushes daily, even a small bug rate would introduce numerous bugs, compli- cating builds and releases. – High potential for merge conflicts and build failures. Branch Hierarchy: – Layered branches isolate changes, reducing risks before merging into the main codebase. – Approval for pull requests and rigorous testing are required before changes move up the branch hierarchy. – If issues persist, Microsoft may assign a specialist team to resolve branch problems. Challenges with Multi-Repository Bug Fixes: – Complex bug fixes can require changes across multiple repositories, adding coordination com- plexity. Similar Systems: – Google manages its codebase using a custom version control system, Piper, with an estimated 15 million weekly changes across 250,000 files by around 25,000 developers. 63 Versioning Version Differences: – Different versions of Windows (e.g., Home, Pro, Education) are built from the same codebase, with variations based on included libraries and features. Importance of Versioning IDs: – Every change creates a new version ID, allowing exact reproduction of builds for debugging. Complexity: – Compatibility across multiple hardware platforms and software versions requires continuous testing and coordination. – Build engineers focus on maintaining version consistency across platforms. 64 Software as Communication Code serves as a communication tool within large organizations, conveying intent, functionality, and usage to other team members. Purpose of Code as Communication: – Helps team members understand the purpose, usage, and functionality of the code, even if they never meet. – Allows future developers to use, modify, and troubleshoot the code effectively. Key Aspects for Readability: – Usage Clarity: Indicates inputs, outputs, and error conditions. 16 – Functionality: Describes what the code accomplishes. – Mechanism: Explains how the code achieves its function, aiding debugging and extension. Importance Increases With: – Company Size: Less likelihood of direct communication with other developers. – Code Longevity: The original developer may not be available for future updates. – Code Reuse: Code may be sold or reused by third parties. 65 Challenges of Code Documentation Understanding Code Goals: – As with learning algorithms (e.g., quicksort), understanding code requires grasping both the purpose and mechanism. Updating Documentation: – Documentation should match code changes, but it often falls out of sync due to lack of updates. Forms of Documentation: – Plain Language Descriptions: Documentation for developers. – User Manuals, Javadocs, Examples: Help illustrate functionality and facilitate under- standing. Challenges with Non-Code Documentation: – Non-code documentation must be maintained alongside code. – Documentation often becomes outdated as code evolves, due to oversight or separation be- tween coders and documenters. 66 Design 67 Introduction This lecture covers the process of design in software development, exploring types of design, architecture, detailed design, and the qualities that define good design. 68 What is Design? Definition: Design transforms requirements into a blueprint guiding implementation, decomposing the system into components and organizing them through interfaces. Role of the Designer: – Interprets requirements and creates structured plans for developers. – Facilitates the transition from ambiguous requirements to a viable system plan. – Acts as a problem-solver, providing clarity and direction. – Example: ”The Wolf” character in popular culture as an effective problem-solver. 69 Types of Design Software design can encompass various levels, but this lecture focuses on two main types: Architectural Design: High-level decisions made by software architects, impacting the overall structure. Detailed Design: Component-level planning performed by designers, adding specificity to the architecture. 17 70 Architecture Enterprise Architects: – Establish company-wide standards, impacting operating systems, infrastructure, and lan- guages. – Rarely involved in individual projects; decisions influence multiple systems across the com- pany. Project Architects (Lead Architect): – Responsible for a project’s structure, addressing non-functional requirements and providing the “big picture.” – Key roles include balancing security, responsiveness, and stability. – Often liaises with managers and external representatives, especially when issues arise. 70.1 Architectural Views (4+1 Model) Architectural design is organized into views to highlight different aspects of the system: Logical View: Focuses on functionality, using class, sequence, and activity diagrams. Dominates the structure of complex projects. Physical View: Depicts hardware and network interconnections, useful for cloud or embedded systems. Process View: Illustrates dynamic behavior and component interactions, particularly valuable in parallel and distributed systems. Development View: Describes the software’s organization from a developer’s perspective, in- cluding layers, packages, and communication paths. Scenarios: Tests views against use cases to verify they meet functional and non-functional re- quirements. 71 Detailed Design Purpose: Expands on architecture, providing enough specificity for developers to begin coding. Role of Designers: – Define detailed aspects of components, interfaces, and APIs. – Work under the lead architect to complete the design document, especially in complex sub- systems like payment modules. Example: In a kiosk, the lead architect structures components like the UI, credit card reader, and server communications, while designers specify their interactions. 72 The Design Document Process: – Begins with high-level requirements, refined by enterprise and lead architects, and detailed further by designers. – Accumulates architecture views, scenarios, and detailed descriptions, providing a comprehen- sive implementation guide. Contents: – Modules, interfaces, testing guidelines, data structures, and global data cross-references. – Completeness ensures developers have a clear roadmap, reducing ambiguity and error poten- tial. 18 73 Desired Qualities of Good Design A good design should demonstrate: Correctness: Meets requirements, handling errors and unexpected situations. Understandability: Minimizes ambiguity, providing developers with clear guidance. Efficiency: Demonstrates acceptable performance without excessive reliance on coding optimiza- tions. Maintainability: Supports easy debugging, expansion, and adaptability, with modularity, low coupling, and high cohesion as best practices. 74 Software Measurement 75 Introduction This lecture covers the concept of software measurement, its purposes, and methods used to quantify aspects of the software process and product. Measurement is divided across three phases: planning, development, and testing. 76 What is Software Measurement? Definition: Software measurement assigns numeric values to attributes of a software product or process, helping manage resources, estimate costs, and allocate efforts. Purpose: – Like in manufacturing, software measurement aids budgeting, estimating process changes, and efficient resource allocation. – Managers use metrics to transition from intuition-based decisions to informed actions. Phases of Measurement: – Planning: Estimation before coding. – Development: Progress and complexity assessment. – Testing/QA: Quality evaluation. 77 Planning Goal: Estimate effort based on factors like team size, timeline, and system complexity. Estimation Methods: – Predict by Analogy: Uses historical data from similar projects for guidance. – Function Points (FPs): Estimation method based on system inputs, outputs, and com- plexity factors. 78 Function Points (FPs) Definition: FPs estimate development effort by assigning points to various system components. Counting Points: – External Inputs (EIs), External Outputs (EOs), External Inquiries (EQs), Internal Logical Files (ILFs), and External Interface Files (EIFs). Value Adjustment Factor (VAF): 19 – Adjusts FPs based on system factors like backup needs, data communication requirements, performance, and usability. P14 Calculation: F P = IO points × 0.65 + 0.01 × i=1 V AFi. Uses of FPs: – FPs can estimate at various levels (e.g., project, module, method) and at different project stages. Benefits and Drawbacks: – Benefits: Provides measurable, transparent, and revisable metrics. – Drawbacks: Pre-coding values may be arbitrary and lead to a false sense of certainty. 79 Development FPs in Development: FPs mainly estimate effort, but they can also measure completed code. Direct Measurement Methods: – Measures include fan-in/fan-out, Lines of Code (LOC), cyclomatic complexity, depth of con- ditional nesting, and inheritance depth. 79.1 Cyclomatic Complexity Definition: Measures the number of independent paths in the code, created by Thomas McCabe. Components: – Decision Points: Control flow points like if-statements and loops. – Sections: Continuous code lines without decision points. Control Flow Graph: Visual representation for calculating complexity. Formula: M = E − N + 2, where E is edges, N is nodes, and M is complexity. Example Calculations: Demonstrated with graphs for varying complexity. Implications: Higher complexity suggests more effort for writing and debugging. 80 Testing Testing Metrics: Guide resource allocation and track testing progress. Applications of FPs and Complexity: – FPs highlight high-functionality code for more intensive testing. – Complexity measures support basis-path testing and prioritize more complex functions. Testing Progress: – Monitored through metrics like bugs per line of code and bug frequency trends. 20