Boolean Data Types PDF
Document Details
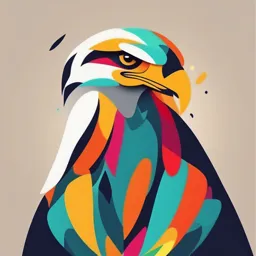
Uploaded by FirstRatePluto1290
Tags
Related
- Chapter 6 Working with Different Types of Data (PDF)
- Programming in Python for Business Analytics (BMAN73701) Lecture Notes PDF
- Python Data Types PDF
- VCE Applied Computing Units 3&4 Software Development - Introduction to Programming PDF
- Data Types and Variables PDF
- Lecture 3 - Programming 2 - 2024-2035 PDF
Summary
This document explains Boolean data types and relational operators in Python programming. It includes examples and demonstrates how to use these concepts in code.
Full Transcript
Boolean Data Types Often in a program you need to compare two values, such as whether x is greater than y. ◦ Or if an inputted value, such as radius, is less than zero. There are six comparison operators (also known as relational operators) that can be used to compare two v...
Boolean Data Types Often in a program you need to compare two values, such as whether x is greater than y. ◦ Or if an inputted value, such as radius, is less than zero. There are six comparison operators (also known as relational operators) that can be used to compare two values. The result of the comparison is a Boolean value: True or False. For example: 1 x = 10 > 20 2 y = 10 < 20 3 print ("x =", x) # output: False 4 print ("y =", y) # output: True x = False y = True 4.2 13 Relational Operators 4.2 14 Caution The equal to comparison operator is two equal signs (==), not a single equal sign (=). The latter symbol is for assignment. >>> 20 == 30 False >>> 50 == 50 True >>> 50.0 == 50 True >>> 50 = 30 SyntaxError: can't assign to literal >>> 60.0001 == 60 False 4.2 15 Boolean Variables A variable that holds a Boolean value is known as a Boolean variable. The Boolean data type is used to represent Boolean values (True or False). A Boolean variable can hold one of the two values: True or False. For example, the following statement assigns the value True to the variable lightsOn: lightsOn = True True and False are literals, just like a number such as 10. They are reserved words and cannot be used as identifiers in a program. 4.2 16 Convert Boolean Value to Integer Internally, Python uses 1 to represent True and 0 for False. You can use the int function to convert a Boolean value to an integer. >>> int(True) 1 >>> int(False) 0 >>> print(int(True)) 1 >>> print(int(20 > 50)) 0 >>> print(int(True == False)) 0 >>> print(int(20 * 2 > 30)) 1 4.2 17 Convert Numeric Value to Boolean You can also use the bool function to convert a numeric value to a Boolean value. The function returns False if the value is 0; otherwise, it always returns True. >>> bool(1) True >>> bool(0) False >>> bool(15) True >>> bool(-20) True >>> bool(10 + 10 - 20) False 4.2 18 Puzzle What is the output of the following script? 1 x = int(True) + int(True) + int(int(True) - int(True)) 2 y = int(True) * int(bool(50) * 3) 3 r = x + y 4 print("x = ", x) 5 print("y = ", y) 6 print("r = ", r) 7 print("bool(r) = ", bool(r)) 8 print("bool(r - r) = ", bool(r - r)) x = 2 y = 3 r = 5 bool(r) = True bool(r - r) = False 4.2 19 4.3. Generating Random Numbers ▪ Generating Random Integer Numbers ▪ Program 1: Math Learning Tool ▪ randrange function ▪ Generating Random Float Numbers ▪ Check Point #1 - #4 20 Generating Random Integer Numbers randint function To generate a random number, you can use the randint(a, b) function in the random module. This function returns a random integer between a and b, inclusively. To obtain a random integer between 0 and 9, use randint(0, 9): 1 import random 2 x = random.randint(0, 9) 3 # x could be 0,1,2,3,4,5,6,7,8, or 9 4 print(x) x = 2 x = 9 x = 7 x = 3 4.3 21 Math Learning Tool Program 1 Write a program that helps a first-grader practice addition. The program should randomly generate two single-digit integers and should then ask the user for the answer. The program will then display a message stating if the answer is true or false. What is 9 + 4? 12 9 + 4 = 12 is False What is 3 + 1? 4 3 + 1 = 4 is True 4.3 Program 1 22 Math Learning Tool Phase 1: Problem-solving What does mean single-digit integers? 0-9 1-digit (single) integer → [0 - 9] 1 import random 2 number = random.randint(0, 9) 0–9 0–9 2-digit integer → [10 - 99] 1 import random 2 number = random.randint(10, 99) 0–9 0–9 0–9 3-digit integer → [100 - 999] 1 import random 2 number = random.randint(100, 999) 4.3 Program 1 23 Math Learning Tool Phase 1: Problem-solving Design your algorithm: 1. Generate two single-digit integers for number1 and number2. ▪ Use randint(0, 9) ▪ Example: number1 = 2 and number2 = 6 2. Ask the user to answer a question ▪ Example: “What is 2 + 6 ?” 3. Print whether the answer is true or false 4.3 Program 1 24 Math Learning Tool Phase 2: Implementation LISTING 4.1 AdditionQuiz.py 1 import random Run 2 3 # Generate random numbers 4 number1 = random.randint(0, 9) 5 number2 = random.randint(0, 9) 6 7 # Prompt the user to enter an answer 8 answer = eval(input("What is " + str(number1) + " + " 9 + str(number2) + "? ")) 10 11 # Display result 12 print(number1, "+", number2, "=", answer, 13 "is", number1 + number2 == answer) What is 1 + 7? 8 1 + 7 = 8 is True What is 4 + 8? 9 4 + 8 = 9 is False 4.3 Program 1 25 Math Learning Tool Trace The Program Execution What is 4 + 8? 9 4 + 8 = 9 is False 4.3 Program 1 26 Math Learning Tool Discussion The program uses the randint function defined in the random module. The import statement imports the module (line 1). Lines 4-5 generate two numbers, number1 and number2. Line 8 obtains an answer from the user. The answer is graded in line 12 using a Boolean expression number1 + number2 == answer. 4.3 Program 1 27 randrange function Python also provides another function, randrange(a, b), for generating a random integer between a and b – 1, which is equivalent to randint(a, b – 1). For example, randrange(0, 10) and randint(0, 9) are the same. Since randint is more intuitive, the book generally uses randint in the examples. >>> import random >>> random.randrange(1, 3) # the value could be: 1 or 2 1 >>> random.randint(1, 3) # the value could be: 1, 2 or 3 2 >>> random.randint(0, 1) # the value could be: 0 or 1 1 >>> random.randrange(0, 1) # This will always be 0 0 4.3 28 Generating Random Float Numbers random function You can also use the random() function to generate a random float r such that 𝟎 ≤ 𝒓 < 𝟏 For example: >>> import random >>> random.random() 0.3362800598715141 >>> random.random() 0.886713208073315 >>> random.random() 0.9735731618649538 Note: the random() function returns a random float number between 0.0 and 1.0 (excluding 1.0). 4.3 29 Check Point #1 How you can generate a float number with n-digit before the decimal point? We can do that by multiplying the generated number with 10𝑛. For example: >>> import random >>> random.random() * 10 ** 1 # 1-digit float number 8.573088600232266 >>> random.random() * 10 ** 2 # 2-digits float number 99.56489589285628 >>> random.random() * 10 ** 3 # 3-digit float number 428.6688384440885 Formula: number = random.random() * 10 ** n n for the number of the digits before the decimal point 4.3 30 Check Point #2 How you can generate a float number with n-digit before the decimal point and d-digit after the decimal point? We can do that by using the round function as the following: >>> import random >>> round(random.random() * 10 ** 1, 2) 7.66 >>> round(random.random() * 10 ** 2, 2) 79.95 >>> round(random.random() * 10 ** 4, 3) 2969.055 Formula: number = round(random.random() * 10 ** n, d) n for the number of the digits before the decimal point d for the number of the digits after the decimal point 4.3 31 Check Point #3 How you can generate a random float number that is equal or greater than a and less than b (𝑎 ≤ 𝑛𝑢𝑚𝑏𝑒𝑟 < 𝑏). We can do that as the following: number = a + (random.random() * (b - a)) Examples: >>> import random >>> a, b = 1, 3 >>> a + (random.random() * (b - a)) # a = 1, b = 3 1.6393718672389215 >>> a, b = 10, 20 >>> a + (random.random() * (b - a)) # a = 10, b = 20 15.046056155663972 4.3 32 Check Point #4 How do you generate a random integer i such that 𝟎 ≤ 𝒊 < 𝟐𝟎 ? 1 import random 2 i = random.randint(0, 19) 3 # Or -> 4 i = random.randrange(0, 20) How do you generate a random integer i such that 𝟏𝟎 ≤ 𝒊 ≤ 𝟓𝟎? 1 import random 2 i = random.randint(10, 50) 3 # Or -> 4 i = random.randrange(10, 51) 4.3 33 4.4. if Statements ▪ Types of Selection Statements ▪ One-way if Statements ▪ if Block ▪ Program 2: Simple if Demo ▪ Check Point #5 34 Types of Selection Statements The preceding program (Program 1) displays a message such as 6 + 2 = 7 is False. If you wish the message to be 6 + 2 = 7 is incorrect, you have to use a selection statement to make this minor change. Python has several types of selection statements: ◦ one-way if statements ◦ two-way if-else statements ◦ nested if statements ◦ multi-way if-elif-else statements ◦ conditional expressions 4.4 35 One-way if Statements A one-way if statement executes an action if and only if the condition is true. The syntax for a one-way if statement is: if boolean-expression: statement(s) The flowchart to the right demonstrates the syntax of an if statement. Example: 1 lightOn = True 2 3 if lightOn: 4 print("Light ON") 4.4 36 Note Also, the following syntax is valid for one-way if statement with a one statement on one line: if boolean-expression: statement Example: 1 lightOn = True 2 if lightOn: print("Light ON") 3 if lightOn == False: print("Light OFF") ◦ It is equivalent to: 1 lightOn = True 2 3 if lightOn: 4 print("Light ON") 5 6 if lightOn == False: 7 print("Light OFF") 4.4 37 Remember A flowchart is a diagram that describes an algorithm or process, showing the steps as boxes of various kinds, and their order by connecting these with arrows. Process operations are represented in these boxes, and arrows connecting them show flow of control. A diamond box is used to denote a Boolean condition and a rectangle box is for representing statements. 4.4 38 if Block If the boolean-expression evaluates to true, the statements in the if block are executed. The if block contains the statements indented after the if statement. For example: if radius >= 0: area = radius * radius * math.pi print("The area for the circle of radius", radius, "is", area) ▪ If the value of radius is greater than or equal to 0, then the area is computed and the result is displayed; otherwise, these statements in the block are not executed. 4.4 39 if Block if radius >= 0: area = radius * radius * math.pi print("The area for the circle of radius", radius, "is", area) 4.4 40 Note The statements in the if block must be indented in the lines after the if line and each statement must be indented using the same number of spaces. For example, the following code is wrong, because the print statement in line 3 is not indented using the same number of spaces as the statement for computing area in line 2. 1 if radius >= 0: 2 area = radius * radius * math.pi 3 print("The area for the circle of radius", radius, "is", area) 4.4 41 Note 1 if i > 0: 1 if i > 0: 2 print("i is positive.") 2 print("i is positive.") (A) Wrong ❌ (B) Correct ✔ 1 if i > 0: print("Positive") 1 if i > 0: print("Positive") 2 print("Not in the if block") 2 print("Not in the if block") (C) Wrong ❌ (D) Correct ✔ 1 if i > 0: 1 if i > 0: 2 print("Positive") 2 print("Positive") 3 print("Not in the if block") 3 print("Not in the if block") (E) Wrong ❌ (F) Correct ✔ 1 if i > 0 # missing : 1 if i > 0 : 2 print("i is positive.") 2 print("i is positive.") (G) Wrong ❌ (H) Correct ✔ 4.4 42 Simple if Demo Program 2 Write a program that prompts the user to enter an integer. If the number is a multiple of 5, the program displays the result HiFive. If the number is divisible by 2, the program displays HiEven. Enter an integer: 4 HiEven Enter an integer: 15 HiFive Enter an integer: 30 HiFive HiEven 4.4 Program 2 43 Simple if Demo Phase 1: Problem-solving Design your algorithm: 1. Prompt the user to enter an integer (number). 2. If number is a multiple of 5, print HiFive. ▪ if (number % 5 == 0) 3. If number is divisible by 2, print HiEven. ▪ if (number % 2 == 0) 4.4 Program 2 44 Simple if Demo Phase 2: Implementation LISTING 4.1 SimpleIfDemo.py 1 number = eval(input("Enter an integer: ")) Run 2 3 if number % 5 == 0: 4 print("HiFive") 5 6 if number % 2 == 0: 7 print("HiEven") Enter an integer: 4 HiEven Enter an integer: 15 HiFive Enter an integer: 30 HiFive HiEven 4.4 Program 2 45 Check Point #5 Write an if statement that assigns 1 to x if y is greater than 0. 1 if y > 0: 2 x = 1 Write an if statement that increases pay by 3% if score is greater than 90. 1 if score > 90: 2 pay = pay + ( pay * (3 / 100) ) 4.4 46