Data Types and Variables PDF
Document Details
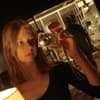
Uploaded by ObtainableHyperbolic
Freie Universität Berlin
Tags
Summary
This document provides an overview of data types and variables in Python. It describes primitive data types such as integers, floating-point numbers, and booleans, as well as composite data types like strings, lists, and dictionaries. The document explains the characteristics of each data type and offers examples, which provides a good introduction to programming with Python.
Full Transcript
# Data Types and Variables ## 3.1 The five facets of a variable - A variable in Python is a name associated with an object in memory. - A variable can be associated with different objects of different types. - In most imperative programming languages, a variable is characterized by at least five di...
# Data Types and Variables ## 3.1 The five facets of a variable - A variable in Python is a name associated with an object in memory. - A variable can be associated with different objects of different types. - In most imperative programming languages, a variable is characterized by at least five different properties: - **Content:** The specific object with which the variable is associated. For example, the content of x = 42 would be the binary sequence 101010. - **Address:** The location of the object. In Python, it is not possible to get the address of an object, but languages close to hardware can show the address of variables and objects. - **Size:** The number of bytes used to describe the object. - **Data type:** A collection of values of the same kind. It tells us what operations are possible to apply on these values. The data type of a variable corresponds to the type of the associated object. - **Scope:** The range of the program in which the variable can be used. It starts with the declaration, which is the first appearance of an assignment. ## 3.2 Primitive data types ### 3.2.1 NoneType - The data type NoneType has only one possible value, which is called None. - It represents the absence of a result or value. - There are no operations for this data type. ### 3.2.2 Booleans - The data type bool represents truth values in Boolean logic. - There are two possible values: True and False. - Operations include: - and: True if and only if both operands are True. - or: True if and only if at least one operand is True. - not: Reverses the truth value of the operand. ### 3.2.3 Integers - The data type int represents integer numbers with a sign. - Examples are 0, 1, -1, 2, 122, 42, -1234. - Some operations on integer numbers are: - (+), (-), (*): Classical numerical operations. - (/): Divides two integers and returns a floating-point number. - (//): Divides two integers and returns an integer. - (%): The modulo operation. When dividing an integer by another integer, it returns the remainder. - abs(-): Returns the absolute value of a number. ### 3.2.4 Floating-point numbers - Floating-point numbers (float) represent a subset of the rational numbers. - They are represented in a special binary format (called IEEE754). - Some operations on floating-point numbers are: - (+), (-), (*), (/): Classical numerical operations. - int(-): Converts a floating-point number into an integer, by removing the digits after the decimal point. ## 3.3 Composite data types - Allow us to collect several simple data items together and to treat them as a unit. - This allows us to group data in our program, and to manipulate arbitrarily large data sets. - There are several composite data types in Python: ### 3.3.1 Characters and Strings - The data type str represents sequences/strings of characters. - Python uses UNICODE-strings. - Strings are represented using single or double quotes ("Hallo", 'Welt', "Hallo 'Welt"", ""Hallo" Welt'). - Some operations on strings are: - (+) Concatenates two strings. - len(-): Returns the length of a string, i.e., it returns the number of characters. - ord(-): Returns the ordinal number of a character. The function produces an error if its input is not of length 1. - chr(-): The inverse function of ord(-). It takes an integer and returns the character according to the UNICODE-assignment. - In Python, a character is the same as a string of length 1. - Strings are immutable, i.e., we cannot change them. ### 3.3.2 Lists - A list consists of a sequence of data objects. - The objects can be of distinct types. - The order of the objects matters, and the same data object can appear repeatedly in a list. - Lists are mutable, that means we can change entries of a list by using the index notation. ### 3.3.3 Tuples - Tuples are written with round parentheses instead of square brackets. - The index operator for tuples also uses square brackets. - Lists are mutable, whereas tuples are immutable. ### 3.3.4 Dictionaries - Dictionaries store key-value pairs, where a key can appear at most once and can be used to look up a value. - A key-value pair is called in item. - Dictionaries are mutable. ## 3.4 Number representation ### 3.4.1 Natural numbers - Typically, we represent numbers in the decimal system. - A number in the decimal system can be represented as a sum. - Instead of 10 we can use any other natural number (larger than 1) as a basis. - In computer science, we typically use bases 2, 8, or 16. ### 3.4.2 Integer numbers - In order to represent negative numbers, we use the two's complement representation. - The integer numbers in [0, \(2^{b-1}\) - 1] are represented binary as described above. - The integer number - \(2^{b-1}\) is represented by the binary string 10...0. - Finally, we can get the representation of the integer numbers in [- \(2^{b-1}\)-1, -1] as follows: - Start from the representation of the corresponding positive number. - Invert all the bits. - Increment the result by 1. - This representation has the following advantages and disadvantages: - Each number in the interval has a unique representation. - Subtracting a number is the same as adding the inverse number. - The interval is not symmetric. (There is a negative number more.)