Pyrthon Unit 1.pdf
Document Details
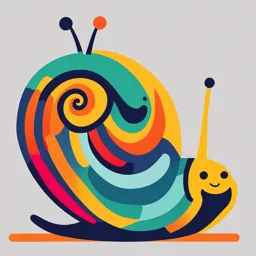
Uploaded by ThriftySandDune
Chhattisgarh Swami Vivekanand Technical University
Related
- A Concise History of the World: A New World of Connections (1500-1800)
- Medication Review PDF
- Lg 5 International Environmental Laws, Treaties, Protocols, and Conventions
- Ziraat Finans Grubu Uygulamaları
- Psychoanalytic Diagnosis: Understanding Personality Structure
- Dynamics of the Cell Membrane PDF - Istanbul Atlas University
Full Transcript
RSR RUNGTA COLLEGE OF ENGINEERING AND TECHNOLOGY Rungta Knowledge City ,Kohka – Kurud ,Bhilai (C.G)-490024 Department of Computer Science & Engineering DIPLOMA AI&ML – 5th Sem Subject: Python Programming...
RSR RUNGTA COLLEGE OF ENGINEERING AND TECHNOLOGY Rungta Knowledge City ,Kohka – Kurud ,Bhilai (C.G)-490024 Department of Computer Science & Engineering DIPLOMA AI&ML – 5th Sem Subject: Python Programming Subject code: 2109571(022) Unit – 1 Python's main features: 1. Simple and Easy to Learn - Python has a clean and easy-to-understand syntax that mimics natural language, making it beginner-friendly and reducing the learning curve. 2. Interpreted Language - Python is an interpreted language, meaning that code is executed line by line, allowing for dynamic testing and debugging without the need for compilation. 3. Dynamically Typed - Python doesn’t require explicit declaration of variable types. The type is determined at runtime based on the variable's value, which provides flexibility in coding. 4. Extensive Standard Library - Python includes a vast standard library that covers many areas like string operations, web service tools, operating system interfaces, and protocols. This rich set of modules helps reduce the need for external dependencies. 5. Cross-Platform Compatibility - Python is cross-platform, meaning it can run on different operating systems such as Windows, macOS, and Linux without requiring modifications to the codebase. 6. Object-Oriented and Procedural Programming Support - Python supports both object-oriented and procedural programming paradigms, allowing developers to choose the approach that best suits their needs. 7. Extensible and Embeddable - Python can be extended with code written in C or C++ and can also be embedded within applications as a scripting language, making it highly versatile. 8. High-Level Language - Python abstracts away most of the complex details of the computer system, enabling developers to focus more on problem-solving rather than low-level programming details. 9. Large and Active Community - Python has a large, active, and supportive community that contributes to a wealth of resources, libraries, frameworks, and documentation. This makes finding help and libraries for almost any application much easier. 10. Open Source - Python is open source, meaning it is free to use and distribute, even for commercial purposes. The open-source nature allows for continuous improvements and updates from the community. 11. Robust Frameworks and Tools - Python has a variety of frameworks and tools like Django, Flask (for web development), Pandas, NumPy (for data analysis), and TensorFlow, PyTorch (for machine learning) that streamline development processes and enhance functionality. 12. Strong Support for Integration - Python can easily integrate with other languages and technologies. It supports integration with C, C++, Java, and.NET components, and also has strong support for various web services and data formats (JSON, XML). Abhishek Singh(Subject Teacher) RSR RUNGTA COLLEGE OF ENGINEERING AND TECHNOLOGY Rungta Knowledge City ,Kohka – Kurud ,Bhilai (C.G)-490024 Department of Computer Science & Engineering DIPLOMA AI&ML – 5th Sem Subject: Python Programming Subject code: 2109571(022) Working modes: Python supports various working modes that cater to different types of development workflows, from quick interactive testing to building large-scale applications. These working modes are designed to offer flexibility, ease of use, and efficiency depending on the needs of the developer. 1. Interactive Mode:- Interactive mode, also known as the Python Shell or REPL(Read-Eval-Print Loop), is a command-line environment where you can execute Python code one line at a time. This mode is primarily used for quickly testing code, learning Python, or experimenting with small code snippets. Every line of code you write is immediately evaluated, and the result is displayed instantly. This mode is excellent for debugging and checking the behavior of small code sections. How to Use: - Open a terminal (Command Prompt in Windows, Terminal in macOS/Linux) and type `python` or `python3` (depending on the version installed). - You will enter the interactive shell, where you can type Python commands and see their results. Example: Advantages: - Instant feedback for every line of code. - Very convenient for testing small code snippets and learning Python. - No need to create or save files. Disadvantages: - Not suitable for large programs or projects. - Code is not saved, so you will lose it after exiting the session unless you manually copy it. Abhishek Singh(Subject Teacher) RSR RUNGTA COLLEGE OF ENGINEERING AND TECHNOLOGY Rungta Knowledge City ,Kohka – Kurud ,Bhilai (C.G)-490024 Department of Computer Science & Engineering DIPLOMA AI&ML – 5th Sem Subject: Python Programming Subject code: 2109571(022) 2. Script Mode:- In Script Mode, you write Python code in a file with a `.py` extension, and you can run the entire script in one go. This mode is used for creating programs or scripts that are intended to be reused or distributed. Script Mode is typically used for larger projects where multiple lines of code or modules are involved. The script can be saved, edited, and reused, making it ideal for writing applications, automation scripts, or even large-scale software systems. How to Use: 1. Write your Python code in any text editor (such as Notepad, Sublime Text, VS Code, PyCharm, etc.). 2. Save the file with a `.py` extension (e.g., `my_script.py`). 3. Run the script from the terminal using the command `python filename.py`. Example: (`hello.py`): Advantages: - Great for writing full programs and reusable code. - The script is saved and can be executed multiple times. - Can be used for automation and larger software development. Disadvantages: - No immediate feedback like in interactive mode; you need to save and run the entire file. - For quick testing, this mode can be slower since the script must be executed each time. Variables and Data type A Variable is a named location in memory used to store data. Variables can hold different types of data and can be changed during the execution of a program. Python is a dynamically-typed language, which means you don't need to declare the type of a variable when you create it; Python automatically assigns the data type based on the value assigned to the variable. Abhishek Singh(Subject Teacher) RSR RUNGTA COLLEGE OF ENGINEERING AND TECHNOLOGY Rungta Knowledge City ,Kohka – Kurud ,Bhilai (C.G)-490024 Department of Computer Science & Engineering DIPLOMA AI&ML – 5th Sem Subject: Python Programming Subject code: 2109571(022) Key Features of Variables in Python: - Dynamic Typing: You don't need to declare the type of a variable explicitly. - Mutable and Immutable Variables: Some types of data can be changed (mutable), while others cannot (immutable). - Naming Convention: Variable names should start with a letter or an underscore, followed by letters, numbers, or underscores. They are case-sensitive (`myVar` and `myvar` are different variables). Declaring a Variable You declare a variable by simply assigning a value to it using the assignment operator (`=`). A Data type is an attribute that defines the kind of data a variable can hold. Python provides a variety of built-in data types, and each type serves a specific purpose. The major categories of data types are: Numeric Types These are the basic building blocks in Python. 1. Integer (`int`):- Represents whole numbers, positive or negative, without any decimal point. Example: Range: Python integers can be arbitrarily large as it supports automatic memory management. 2. Float (`float`):- Represents numbers with decimal points. These are real numbers. Example: 3. Boolean (`bool`):- Represents one of two values: `True` or `False`. It is often used in conditional statements and comparisons. Abhishek Singh(Subject Teacher) RSR RUNGTA COLLEGE OF ENGINEERING AND TECHNOLOGY Rungta Knowledge City ,Kohka – Kurud ,Bhilai (C.G)-490024 Department of Computer Science & Engineering DIPLOMA AI&ML – 5th Sem Subject: Python Programming Subject code: 2109571(022) Example: 4. Complex Numbers (`complex`):- Represents numbers in the form `a + bj`, where `a` is the real part and `b` is the imaginary part. Example: Sequence Types These are used to store collections or more complex data structures. 1. List (`list`):- Represents an ordered collection of items, which can be of any type (integers, strings, floats, etc.). Lists are mutable, meaning the elements can be changed after creation. Syntax: Lists are defined using square brackets `[]`. Example: Operations on Lists: - Accessing elements: - Modifying elements: 2. Tuple (`tuple`):- Similar to lists, but tuples are immutable, meaning they cannot be changed after they are created. Syntax: Defined using parentheses `()`. Abhishek Singh(Subject Teacher) RSR RUNGTA COLLEGE OF ENGINEERING AND TECHNOLOGY Rungta Knowledge City ,Kohka – Kurud ,Bhilai (C.G)-490024 Department of Computer Science & Engineering DIPLOMA AI&ML – 5th Sem Subject: Python Programming Subject code: 2109571(022) Example: 3. String (`str`):- Represents sequences of characters enclosed in single (`'`) or double (`"`) quotes. Strings are immutable, which means once a string is created, it cannot be modified. Example: String Operations: Concatenation: Combining strings using `+`. Slicing: Accessing parts of the string. Keywords Keywords are reserved words that have special meaning in the language. They can't be used as identifiers (like variable names or function names). These keywords are part of the syntax and structure of Python. Python keywords are special reserved words that have specific meanings and purposes in Python. These keywords form the foundation of Python's syntax and functionality. You cannot use them as identifiers (such as variable names or function names) because they are reserved by the language. Below is a detailed explanation of some of the most important Python keywords: 1. `False` and `True`:- These are Boolean values representing truth. `True` evaluates to 1, and `False` evaluates to 0. They are often used in conditions and logical expressions. Example: Abhishek Singh(Subject Teacher) RSR RUNGTA COLLEGE OF ENGINEERING AND TECHNOLOGY Rungta Knowledge City ,Kohka – Kurud ,Bhilai (C.G)-490024 Department of Computer Science & Engineering DIPLOMA AI&ML – 5th Sem Subject: Python Programming Subject code: 2109571(022) 2. `None`:- This keyword is used to define a null value or no value at all. It is similar to `null` in other languages. Example: 3. `and`, `or`, `not`:- These are logical operators used to combine conditional statements. - `and` returns `True` if both statements are `True`. - `or` returns `True` if at least one statement is `True`. - `not` negates the truth value. Example: 4. `if`, `else`, `elif`:- These keywords are used for conditional statements. `if` checks a condition, `else` runs if no conditions are `True`, and `elif` (else if) checks other conditions if the `if` is `False`. Example: Abhishek Singh(Subject Teacher) RSR RUNGTA COLLEGE OF ENGINEERING AND TECHNOLOGY Rungta Knowledge City ,Kohka – Kurud ,Bhilai (C.G)-490024 Department of Computer Science & Engineering DIPLOMA AI&ML – 5th Sem Subject: Python Programming Subject code: 2109571(022) 5. `for`:- Used to iterate over a sequence (such as a list, tuple, or string). Example: 6. `while`:- A loop that repeats as long as a condition is `True`. Example: 7. `break`:- Terminates the current loop prematurely. Example: 8. `continue`:- Skips the rest of the loop iteration and moves to the next iteration. Example: 9. `def`: Defines a function. Abhishek Singh(Subject Teacher) RSR RUNGTA COLLEGE OF ENGINEERING AND TECHNOLOGY Rungta Knowledge City ,Kohka – Kurud ,Bhilai (C.G)-490024 Department of Computer Science & Engineering DIPLOMA AI&ML – 5th Sem Subject: Python Programming Subject code: 2109571(022) Example: 10. `return`:- Exits a function and optionally returns a value. Example: 11. `class`:- Used to define a new user-defined class. Example: 12. `try`, `except`, `finally`:- These are used for handling exceptions. The `try` block tests a block of code for errors, the `except` block handles the error, and the `finally` block is executed regardless of the result. Example: 13. `import`:- Used to import a module (external code or libraries). Abhishek Singh(Subject Teacher) RSR RUNGTA COLLEGE OF ENGINEERING AND TECHNOLOGY Rungta Knowledge City ,Kohka – Kurud ,Bhilai (C.G)-490024 Department of Computer Science & Engineering DIPLOMA AI&ML – 5th Sem Subject: Python Programming Subject code: 2109571(022) Example: 14. `from`, `as`:- - `from`: Used to import specific parts of a module. - `as`: Used to assign an alias to a module. Example: 15. `in`, `not in`:- Used to check membership in sequences like lists, tuples, or strings. Example: 16. `is`:- Used to check if two variables point to the same object in memory. Example: 17. `global`:- Used to declare that a variable inside a function is global, meaning it can be modified inside the function. Example: Abhishek Singh(Subject Teacher) RSR RUNGTA COLLEGE OF ENGINEERING AND TECHNOLOGY Rungta Knowledge City ,Kohka – Kurud ,Bhilai (C.G)-490024 Department of Computer Science & Engineering DIPLOMA AI&ML – 5th Sem Subject: Python Programming Subject code: 2109571(022) 18. `nonlocal`:- Used to declare that a variable inside a nested function is not local to the function but belongs to the enclosing function. Example: 19. `pass`:- A null operation, used when a statement is required syntactically but no action is needed. Example: 20. `with`:- Used for resource management, such as working with files or managing locks. Example: 21. `lambda`:- Used to create anonymous functions (small functions without a name). Example: 22. `yield`:- Used in a function like `return`, but instead of exiting the function, it pauses the function and returns a value. The function can later be resumed. Example: Abhishek Singh(Subject Teacher) RSR RUNGTA COLLEGE OF ENGINEERING AND TECHNOLOGY Rungta Knowledge City ,Kohka – Kurud ,Bhilai (C.G)-490024 Department of Computer Science & Engineering DIPLOMA AI&ML – 5th Sem Subject: Python Programming Subject code: 2109571(022) 23. `assert`:- Used for debugging purposes. It tests if a condition is `True`; if not, it raises an `AssertionError`. Example: 24. `del`:- Used to delete an object, variable, or an item from a list. Example: Operators Operators are symbols in programming languages that perform operations on variables and values. 1. Logical Operators Logical operators are used to combine conditional statements. They return a boolean value (`True` or `False`) based on the logic they perform. Types of Logical Operators - AND (`and`): Returns `True` if both the operands (conditions) are true. - OR (`or`): Returns `True` if at least one of the operands (conditions) is true. - NOT (`not`): Returns `True` if the operand (condition) is false, and vice versa. Detailed Examples: Abhishek Singh(Subject Teacher) RSR RUNGTA COLLEGE OF ENGINEERING AND TECHNOLOGY Rungta Knowledge City ,Kohka – Kurud ,Bhilai (C.G)-490024 Department of Computer Science & Engineering DIPLOMA AI&ML – 5th Sem Subject: Python Programming Subject code: 2109571(022) Explanation: - `and` Operator: `a > b` and `b > c` both are `True`, so the result is `True`. - `or` Operator: `a > b` is `True`, but `c > b` is `False`. Since one condition is `True`, the result is `True`. - `not` Operator: `not (a > b)` inverts the `True` to `False`. 2. Relational (Comparison) Operators Relational or comparison operators are used to compare two values. They return a boolean value (`True` or `False`) based on the comparison. Types of Relational Operators - Equal to (`==`): Checks if the values of two operands are equal. - Not equal to (`!=`): Checks if the values of two operands are not equal. - Greater than (`>`): Checks if the value of the left operand is greater than the value of the right operand. - Less than (`=`): Checks if the value of the left operand is greater than or equal to the value of the right operand. - Less than or equal to (``: `a` is not greater than `b`, so it returns `False`. - `=`: `a` is not greater than or equal to `b`, so it returns `False`. - `