Lecture (5).ppt.pdf
Document Details
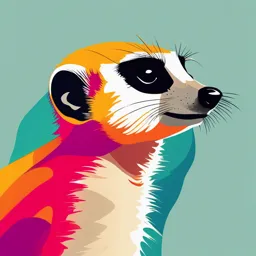
Uploaded by GracefulConnemara4342
W.P. Wagner School
Tags
Full Transcript
Lecture (5) Why do we need sockets? Provides an abstraction for interprocess communication Socket Socket: The services provided (often by the operating system) that provide the interface between application and protocol software. Network APIs provide a way for net...
Lecture (5) Why do we need sockets? Provides an abstraction for interprocess communication Socket Socket: The services provided (often by the operating system) that provide the interface between application and protocol software. Network APIs provide a way for network platforms to communicate with applications Application Network API Protocol A Protocol B Protocol C Socket Functions –Define an “end- point” for communication –Initiate and accept a connection –Send and receive data –Terminate a connection gracefully Examples File transfer apps (FTP), Web browsers (HTTP), Email (SMTP/ POP3), etc… Operational statuses of sockets Two different types of sockets : – stream vs. datagram Stream socket :( a. k. a. connection- oriented socket) the socket on the server process waits for requests from a client. To do this, the server first establishes (binds) an address that clients can use to find the server. When the address is established, the server waits for clients to request a service – It provides reliable, connected networking service – Error free; no out- of- order packets (uses TCP) – applications: telnet, http, … Datagram socket :( a. k. a. connectionless socket) Connectionless sockets do not establish a connection over which data is transferred. Instead, the server application specifies its name where a client can send requests. Connectionless sockets use User Datagram Protocol (UDP) instead of TCP/IP – It provides unreliable, best- effort networking service – Packets may be lost; may arrive out of order (uses UDP) – applications: streaming audio/ video (realplayer), … Addressing Client Server Addresses, Ports and Sockets Like apartments and mailboxes – You are the application – Your apartment building address is the address – Your mailbox is the port – The post-office is the network – The socket is the key that gives you access to the right mailbox Client – high level view Create a socket Setup the server address Connect to the server Read/write data Shutdown connection Server – high level view Create a socket Bind the socket Listen for connections Accept new client connections Read/write to client connections Shutdown connection UDP Protocol This simple protocol provides transport layer addressing in the form of UDP ports and an optional checksum capability. UDP is a very simple protocol. Messages, so called datagrams, are sent to other hosts on an IP network without the need to set up special transmission channels or data paths beforehand. The UDP socket only needs to be opened for communication. It listens for incoming messages and sends outgoing messages on request. UDP Segment in Transport Layer Checksum The final two bytes of the UDP header is the checksum, a field that's used by the sender and receiver to check for data corruption. Before sending off the segment, the sender: Computes the checksum based on the data in the segment: checksum is a string of numbers and letters that act as a fingerprint for a message/file against which later comparisons can be made to detect errors in the data. They are important because we use them to check files for integrity. Pseudo header helps to find transfer bit errors and also to protect against other types of network errors like the possibility of IP datagram reaching a wrong host Sending UDP Data UDP is a byte stream service. It does not know anything about the format of the data being sent. It simply takes the data, encapsulates it into a UDP packet, and sends it to the remote peer. The UDP protocol does not wait for any acknowledgement and is unable to detect any lost packets. When acknowledgement or detection is required, it must be done by the application layer. However, it is better to use a TCP Socket for communication when acknowledgement is necessary. Example for Sending UDP Data Sending / Receiving Data With a connection (SOCK_STREAM): – int count = send(sock, &buf, len, flags); count: # bytes transmitted (-1 if error) buf: char[], buffer to be transmitted (64000 byte) len: integer, length of buffer (in bytes) to transmit (512 byte) flags: integer, special options, usually just 0 (acknowledgement) – int count = recv(sock, &buf, len, flags); count: # bytes received (-1 if error) buf: void[], stores received bytes len: # bytes received flags: integer, special options, usually just 0 socket() TCP Server bind() Well-known port TCP Client listen() Socket() accept() connect() Connection establishment blocks until connection from client write() read() process request write() read() close() read() close() Dealing with blocking calls Types of connections…. For simple connections this is fine Many functions ….. – accept(), connect(), – All recv() What means complex connection routines – Multiple connections – Simultaneous sends and receives – Simultaneously doing non-networking processing Dealing with blocking (cont..) Options were took into consideration… – Create multi-process or multi-threaded code – Turn off blocking feature (fcntl() system call) – Use the select() function What does select() do? Can be permanent blocking, time-limited blocking or non- blocking – Input: a set of file descriptors – Output: info on the file-descriptors’ status Therefore, can identify sockets that are “ready for use”: calls involving that socket will return immediately select function call int status = select() – Status: # of ready objects, -1 if error – nfds: 1 +largest file descriptor to check – readfds: list of descriptors to check if read-ready – writefds: list of descriptors to check if write-ready – Timeout: time after which select returns