ITNT313 Lecture 4 - Network Programming PDF
Document Details
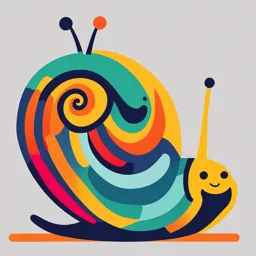
Uploaded by FestiveOpossum9360
University of Tripoli
Marwa Ebrahim Elwakedy
Tags
Summary
This document is a lecture on network programming using sockets, covering topics such as socket programming, datagram and stream communication, and TCP programming with Python. It features diagrams and code snippets for a better understanding
Full Transcript
04 TCP Programming Network Programming University of Tripoli Faculty of Information Technology Department of Networking Assistant Lecturer / Marwa Ebrahim Elwakedy Socket Programming: â—¼ Sockets are programming abstraction that represent end-p...
04 TCP Programming Network Programming University of Tripoli Faculty of Information Technology Department of Networking Assistant Lecturer / Marwa Ebrahim Elwakedy Socket Programming: ◼ Sockets are programming abstraction that represent end-points of network comm. ◼ Sockets encapsulate the implementation of network and transport layer protocols. ◼ Programs create socket objects and send/receive data using the sockets API. ◼ Developed by Bill Joy for the Berkeley UNIX. ◼ Also known as Berkeley(BSD) Sockets. ◼ Now sockets are everywhere. 04 TCP Programming Sockets in Python: 04 TCP Programming Datagram vs. Stream: ◼ Network communication abstraction: connectionless (datagram/packet) connection-based (stream) ◼ Socket interfaces also reflect the two types of network communication: For connectionless comm(UDP), create datagram sockets(on both side) and sendto/recvfrom each other. For connection-based comm(TCP), create stream sockets, server waits(listen) for conn request, set up a connection, and then sendall/recv. 04 TCP Programming Socket Programming: ◼ Port addressing is another abstraction for multiple services on the same host. ◼ Every service is associated with (bound-to) one or more ports. ◼ To contact a service, must provide both the IP address and the port number it is using (listening-to). ◼ Both UDP and TCP use 16-bit port number in the range 1 to 65535. 04 TCP Programming Port Assignment ◼The Internet uses well-known port numbers to avoid the need for a port directory service ◼Every standard Internet application must request a port number from the Internet Assigned Numbers Authority (IANA; http://www.iana.org/) ◼ FTP tcp:21 SMTP tcp:25 SNMP udp:161 DNS udp:53, tcp:53 HTTP tcp:80 04 TCP Programming Stream Sockets 04 TCP Programming TCP Socket Flow 04 TCP Programming Python Socket Basics ◼To create a socket import socket s = socket.socket(addr_family, type) ◼ Address families socket.AF_INET Internet protocol (IPv4) socket.AF_INET6 Internet protocol (IPv6) ◼ Socket types socket.SOCK_STREAM Connection based stream (TCP) socket.SOCK_DGRAM Datagrams (UDP) ◼ Example: from socket import * s = socket(AF_INET,SOCK_STREAM) 04 TCP Programming Socket Types ◼Almost all code will use one of following from socket import * s1 = socket(AF_INET, SOCK_STREAM) s2 = socket(AF_INET, SOCK_DGRAM) ◼Most common case: TCP connection : s = socket(AF_INET, SOCK_STREAM) 04 TCP Programming Using a Socket ◼Creating a socket is only the first step s = socket(AF_INET, SOCK_STREAM) ◼ Further use depends on application ◼Server Listen for incoming connections ◼Client Make an outgoing connection 04 TCP Programming TCP Client ◼How to make an outgoing connection from socket import * s = socket(AF_INET,SOCK_STREAM) address = ('www.ndhu.edu.tw',80) s.connect(address) # Connect s.send(b'GET / HTTP/1.0\n\n') # Send request(in byte) data = s.recv(10000) # Get response s.close() # Close the socket 04 TCP Programming TCP Client ◼s.connect(addr) makes a connection ◼ Once connected, use send(), recv() to transmit and receive data ◼close() shuts down the connection 04 TCP Programming Socket Functions ◼socket.socket(family=AF_INET, type=SOCK_STREAM, proto=0) ◼ socket.getaddrinfo(host, port, family=0, type=0, proto=0, flags=0) ◼socket.getfqdn([name]) ◼ socket.gethostbyname(hostname) ◼ socket.gethostname() ◼socket.gethostbyaddr(ip_address) 04 TCP Programming Socket Functions ◼socket.getprotobyname(protocolname) ◼ socket.getservbyname(servicename[, protocolname]) ◼ socket.getservbyport(port[, protocolname]) ◼ socket.getdefaulttimeout() ◼ socket.setdefaulttimeout(timeout) ◼socket.sethostname(name) 04 TCP Programming TCP Server ◼Network servers are a bit more tricky ◼Must listen for incoming connections on a well-known(or agreed upon) port number ◼Typically run forever in a server-loop ◼ May have to service multiple clients (currency) at the same time 04 TCP Programming TCP Server ◼ A simple server: create a server socket, accept a connection and say hello from socket import * s = socket(AF_INET, SOCK_STREAM) s.bind(("",9000)) s.listen(2) while True: c,a = s.accept() print("Received connection from", a) c.send(b"Hello " + a.encode()) c.close() 04 TCP Programming Address Binding ◼ First execute the server program ◼ The server must bind the socket to a specific address s.bind(("",9000)) ◼ The bind method bind the created socket s to a specific (address, port). s.bind(("",9000)) #Binds to localhost s.bind(("localhost",9000)) s.bind(("192.168.2.1",9000)) s.bind(("104.21.4.2",9000)) 04 TCP Programming Listening for Connection ◼ After binding, the server starts listening for connections s.listen(2) s.listen(backlog) ◼ backlog is # of pending connections to allow ◼Note: not related to max number of clients 04 TCP Programming Server Loop & Accept New Connections ◼The server starts a loop of serving clients. ◼ The loop starts with an accept() to new connection while True: c,a = s.accept() ◼ s.accept() blocks until connection received ◼ Server sleeps if nothing is happening 04 TCP Programming Client Socket & Address ◼accept() returns a pair (client_socket, addr) ◼ The new client socket is used for further communication with the client. ◼ The client address includes IP and port no. c,a = s.accept() 04 TCP Programming Sending Data ◼Server uses the client socket for transmitting data(in bytes). c,a = s.accept() print("Received connection from", a) c.send(b"Hello " + a.encode()) ◼The server socket is only used for accepting new connections. 04 TCP Programming Closing the Connection ◼After finishing the service, server should close() the connection to release resources. c.close() ◼ Server can keep client connection alive as long as it wants ◼Can repeatedly receive/send data before closing 04 TCP Programming Wait for the next Connection ◼After Current service iteration ends with close(). ◼ New iteration starts with accept() again. while True: c,a = s.accept() print("Received connection from", a) c.send(b"Hello " + a.encode()) c.close() ◼Original server socket is reused to listen for more connections ◼ Server runs forever in a loop like this 04 TCP Programming TCPEchoServer import socket import sys # Create a TCP/IP socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Bind the socket to the port server_address = ('localhost', 10000) print('starting up on port ', server_address) sock.bind(server_address) # Listen for incoming connections sock.listen(1) 04 TCP Programming TCPEchoServer (2) while True: print('waiting for a connection') connection, client_address = sock.accept() # Wait for a connection try: print('connection from', client_address) while True: # Receive the data in small chunks and retransmit it data = connection.recv(16) print('received , data) if data: print('sending data back to the client') connection.sendall(data) else: print('no data from', client_address) break finally: # Clean up the connection connection.close() 04 TCP Programming TCPEchoClient iimport socket import sys # Create a TCP/IP socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Connect the socket to the server listening port server_address = ('localhost', 10000) sock.connect(server_address) 04 TCP Programming TCPEchoClient (2) try: # Send data message = 'This is the message.' print('sending ', message) sock.sendall(message.encode()) # Look for the response amount_received = 0 amount_expected = len(message) msg = [] while amount_received < amount_expected: data = sock.recv(16) amount_received += len(data) msg.append(data) print('received ', b''.join(msg).decode()) finally: print('closing socket') sock.close() 04 TCP Programming Advanced Sockets ◼Socket programming is often a mess ◼ Huge number of options ◼ Many corner cases ◼ Many failure modes/reliability issues ◼ Will briefly cover a few critical issues 04 TCP Programming Partial Reads/Writes ◼Be aware that reading/writing to a socket may involve partial data transfer ◼ send() returns actual bytes sent ◼ recv() length is only a maximum limit len(data) s.send(data) #Send partial data data = s.recv(10000) len(data) #Received less than max 04 TCP Programming Partial Reads/Writes ◼Be aware that for TCP, the data stream is continuous- --no concept of records, etc. # Client... s.send(data) s.send(moredata)... # Server... data = s.recv(maxsize)... ◼A lot depends on OS buffers, network bandwidth, congestion, etc. 04 TCP Programming Sending All Data ◼To wait until all data is sent, use: sendall() s.sendall(data) ◼ Blocks until all data is transmitted ◼ For most normal applications, this is what you should use ◼Exception : You don’t use this if networking is mixed in with other kinds of processing (e.g., screen updates, multitasking, etc.) 04 TCP Programming End of Data ◼How to tell if there is no more data? ◼recv() will return empty string s.recv(1000) ◼ This means that the other end of the connection has been closed (no more sends) 04 TCP Programming Data Reassembly ◼Receivers often need to reassemble messages from a series of small chunks ◼Here is a programming template for that fragments = [] # List of byte chunks while not done: chunk = s.recv(maxsize) # Get a chunk if not chunk: break # EOF. No more data fragments.append(chunk) # Reassemble the message message = b"".join(fragments).decode() ◼Don't use string concat (+=). It's slow. 04 TCP Programming Timeouts ◼Most socket operations block indefinitely ◼ Can set an optional timeout s = socket(AF_INET, SOCK_STREAM) s.settimeout(5.0) # Timeout of 5 seconds ◼Will get a timeout exception s.recv(1000) Traceback (most recent call last): File "", line 1, in socket.timeout: timed out ◼ Disabling timeouts s.settimeout(None) 04 TCP Programming Non-blocking Sockets ◼Instead of timeouts, can set non-blocking s.setblocking(False) ◼ Future send(), recv() operations will raise an exception if the operation would have blocked s.setblocking(False) s.recv(1000) Traceback (most recent call last): File "", line 1, in socket.error: (35, 'Resource temporarily unavailable') s.recv(1000) 'Hello World\n' ◼Sometimes used for polling 04 TCP Programming Socket Options ◼Sockets have a large number of parameters ◼ Can be set using s.setsockopt() ◼Example: Reusing the port number s.bind(("",9000)) Traceback (most recent call last): File "", line 1, in File "", line 1, in bind socket.error: (48, 'Address already in use') s.setsockopt(socket.SOL_SOCKET,... socket.SO_REUSEADDR, 1) s.bind(("",9000)) ◼ Consult socket reference for more options 04 TCP Programming