Programming Languages PDF
Document Details
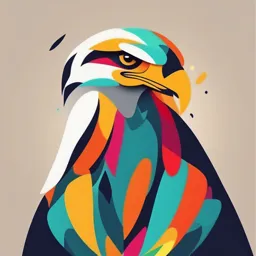
Uploaded by VerifiableKraken
Tags
Summary
This document provides an introduction to programming languages. It covers fundamental concepts like algorithms, syntax, semantics, and debugging. The document also introduces different types of programming languages such as assembly language and high-level language.
Full Transcript
## 11 Programming Languages ### 1. Introduction A language is the main medium of communicating between the Computer systems and the most common are the programming languages. As we know a Computer only understands binary numbers that is 0 and 1 to perform various operations but the languages are d...
## 11 Programming Languages ### 1. Introduction A language is the main medium of communicating between the Computer systems and the most common are the programming languages. As we know a Computer only understands binary numbers that is 0 and 1 to perform various operations but the languages are developed for different types of work on a Computer. They are based on certain syntactic and semantic rules, which define the meaning of each of the programming language constructs. ### 1.1 Some Terms Related to Programming Language - **Algorithm:** An algorithm is a well-defined procedure that allows a computer to solve a problem. Another way to describe an algorithm is a sequence of unambiguous instructions. The use of the term 'unambiguous' indicates that there is no room for subjective interpretation. Every time you ask your computer to carry out the same algorithm, it will do it in exactly the same manner with the exact same result. - **Syntax:** The syntax of a computer language is the set of rules that defines the combinations of symbols that are considered to be a correctly structured document or fragment in that language. Text-based computer languages are based on sequences of characters, while visual programming languages are based on the spatial layout and connections between symbols (which may be textual or graphical). Documents that are syntactically invalid are said to have a syntax error. - **Semantics:** Semantics is the field concerned with the rigorous mathematical study of the meaning of programming languages. It does so by evaluating the meaning of syntactically legal strings defined by a specific programming language, showing the computation involved Syntax is about the structure or the grammar of the language. It answers the question: how do I construct a valid sentence? All languages, even English and other human languages have grammars, that is, rules that define whether or not the sentence is properly constructed. Semantics is about the meaning of the sentence. It answers the questions: is this sentence valid? If so, what does the sentence mean? In summary, syntax is the concept that concerns itself only whether or not the sentence is valid for the grammar of the language. Semantics is about whether or not the sentence has a valid meaning. - **Source Code and Object Code:** Source code is the fundamental component of a computer program that is created by a programmer. It can be read and easily understood by a human being. When a programmer types a sequence of C language statements into Windows Notepad, for example, and saves the sequence as a text file, the text file is said to contain the source code. Source code and object code refer to the "before" and "after" versions of a computer program that is compiled (see compiler) before it is ready to run in a computer. Object code, or sometimes an object module, is what a computer compiler produces. In a general sense object code is a sequence of statements or instructions in a computer language. - **Debugging:** Debugging is the art of diagnosing errors in programs and determining how to correct them. "Bugs" come in a variety of forms, including: coding errors, design errors, complex interactions, poor user interface designs, and system failures. Debugging is the routine process of locating and removing computer program bugs, errors or abnormalities, which is methodically handled by software programmers via debugging tools. Debugging checks, detects and corrects errors or bugs to allow proper program operation according to set specifications. - **Patch:** A patch is a piece of software designed to update a computer program or its supporting data, to fix or improve it. This includes fixing security vulnerabilities and other bugs, with such patches usually called bugfixes or bug fixes, and improving the usability or performance. Patch (sometimes called a "fix") is a quick-repair job for a piece of programming. During a software product's beta test distribution or try-out period and later after the product is formally released, problems (called bug) will almost invariably be found. A patch is the immediate solution that is provided to users. ### 1.2 Types of Languages - **Low Level Language:** Low-level languages those languages which are extremely close to machine language. A low-level programming language is a programming language that provides little or no abstraction from a computer's instruction set architecture - commands or functions in the language map closely to processor instructions. Generally, this refers to either machine code or assembly language. Low-level languages are more appropriate for developing new operating systems or writing firmware codes for micro-controllers. - **Assembly Language:** An assembly language is a group of languages that implements a symbolic representation of the machine code required to program certain CPU architecture. It is a programming language for microprocessors and other programming devices, and it is the most basic programming language available for any processor. Generally, assemblers produce object files, and most provide macros. Unlike high-level languages, assembly languages lack variables and functions, but they have the same structure and set of commands, much like machine languages. This programming language is helpful to programmers when speed is required and when they need to perform an operation that cannot be done in high-level languages. Machine code is the only language a computer can process directly without a previous transformation. - **High Level Language:** A high-level language is a programming language such as C, FORTRAN, or Pascal that enables a programmer to write programs that are more or less independent of a particular type of computer. Such languages are considered high-level because they are closer to human languages and further from machine languages. In contrast, assembly languages are considered low-level because they are very close to machine languages. The main advantage of high-level languages over low-level languages is that they are easier to read, write, and maintain. It is important to know that a "computer language" and a "programming language" are not quite the same. A computer language is code that can be read by a computer. A programming language (also called software languages) is used to make a program. For instance, HTML, CSS, XML, SQL, and Latex are examples of computer languages that are not programming languages. C/C++, Python, Lua, Scala, and Java are examples of computer languages that are programming languages. Some programming languages are scripting languages. Scripting languages are computer languages that are not compiled (converted to binary/machine-code). In other words, the computer reads the plain text, usually by using a program called an interpreter. Examples of scripting languages include Python, Lua, Perl, Ruby, and JavaScript. Markup languages are computer languages that are not compiled (like scripting languages). However, markup languages are not used to make programs. Rather, they can be used to make web-pages (like HTML) or databases (like XML). Markup languages use "tags" instead of commands (such as "<br />"). - **Procedural Language:** Procedural language is a type of computer programming language that specifies a series of well-structured steps and procedures within its programming context to compose a program. It contains a systematic order of statements, functions, and commands to complete a computational task or program. Procedural language is also known as imperative language. Procedural programming languages include C, Go, Fortran, Pascal, Ada, and BASIC. - **Object-Oriented Programming Language:** Object-oriented programming (OOP) is a programming paradigm based on the concept of "objects", which may contain data, in the form of fields, often known as attributes; and code, in the form of procedures, often known as methods. A feature of objects is that an object's procedures can access and often modify the data fields of the object with which they are associated. Significant object-oriented languages include Java, C++, C#, Python, PHP, Ruby, Perl, Delphi, Objective-C, Swift, Common Lisp, and Smalltalk. In your examination they can ask a question that which among the following is a type of object oriented programming language. So it's beneficial for you to know that which follow OOPS concept and which do not does so. ### 1.3 Compiler Interpreter and Assembler Both compiler and interpreter convert human readable high level language like Java, C++ etc into machine language but there is difference in the way both function. - **Compiler** scans the entire program once and then converts it into machine language which can then be executed by computer's processor. In short compiler translates the entire program in one go and then executes it. - **Interpreter**, on the other hand, first converts high level language into an intermediate code and then executes it line by line. This intermediate code is executed by another program. The execution of program is faster in compiler than interpreter as in interpreter code is executed line by line. Compiler generates error report after translation of entire code whereas in case of interpreter once an error is encountered it is notified and no further code is scanned. - **Assembler** is used for converting the code of low level language (assembly language) into machine level language. ### 2. C Language #### 2.1 Introduction: C is a programming language developed at AT & T's Bell Laboratories of USA in 1972. It was designed and written by Dennis Ritchie. It is one of the most popular computer languages today because of its structure, high-level abstraction, machine independent feature. C language was developed with UNIX operating system, so it is strongly associated with UNIX, which is one of the most popular network operating system. #### 2.2. C Keyword Keywords are the words whose meaning has already been explained to the C compiler. The keywords cannot be used as variable names because if we do so we are trying to assign a new meaning to the keyword, which is not allowed by the computer. Some C compilers allow you to construct variable names that exactly resemble the keywords. However, it would be safer not to mix up the variable names and the keywords. The keywords are also called 'Reserved words'. There are only 32 keywords available in C: | Keyword | Keyword | Keyword | Keyword | Keyword | Keyword | Keyword | Keyword | Keyword | Keyword | | :--------- | :------ | :------ | :------ | :------ | :------ | :------ | :------ | :------ | :------ | | auto | double | int | struct | break | else | long | switch | case | float | | enum | short | register | typedef | char | extern | return | union | const | signed | | volatile | do | if | static | while | void | default | goto | sizeof | unsigned | #### 2.3. Data Types in C Data types simply refer to the type and size of data associated with variables and functions. There are five basic data types use in C: - **double**: a double-precision floating point value. - **int**: integer - a whole number. - **float**: floating point value - ie a number with a fractional part. - **char**: a single character. - **void**: valueless special purpose type #### 2.4. Header files in C Header files contain the set of predefined standard library functions that we can include in our c programs. But, to use these various library functions, we have to include the appropriate header files: | Header File | Description | | :---------- | :----------------------------------------------- | | stdio.h | Input/Output Functions | | conio.h | console input/output | | assert.h | Diagnostics Functions | | ctype.h | Character Handling Functions | | locale.h | Localization Functions | | math.h | Mathematics Functions | | setjmp.h | Nonlocal Jump Functions | | signal.h | Signal Handling Functions | | stdarg.h | Variable Argument List Functions | | stdlib.h | General Utility Functions | | string.h | String Functions | | time.h | Date and Time Functions | #### 2.5. Decision making in C Decision making is about deciding the order of execution of statements based on certain conditions or repeat a group of statements until certain specified conditions are met. C language handles decision-making by supporting the following statements: **1. If Statement:** If statements in C is used to control the program flow based on some condition. it's used to execute some statement code block if expression is evaluated to true, otherwise it will get skipped. This is an simplest way to modify the control flow of the program. There are four different types of if statement in C: - **Simple if Statement** - **if-else statement** - **Nested if-else Statement** - **else-if Ladder** **2. Switch Statement:** Switch statement is used when you have multiple possibilities for the if statement. The basic format of switch statement is: ```c switch(variable) { case 1: //execute your code break; case n: //execute your code break; default: //execute your code break; } ``` After the end of each block it is necessary to insert a break statement because if the programmers do not use the break statement, all consecutive blocks of codes will get executed from each and every case onwards after matching the case block. **3. Goto Statement:** C supports a special form of statement that is the goto Statement which is used to branch unconditionally within a program from one point to another. goto statement is used by programmers to change the sequence of execution of a C program by shifting the control to a different part of the same program. **4. Conditional Operator Statement:** Conditional Operator returns the statement depends upon the given expression result. #### 2.6. C Loops Loops are used to execute a set of statements repeatedly until a particular condition is satisfied. A loop statement allows us to execute a statement or group of statements multiple times. There are 3 type of Loops in C language: - **While Loop:** The most basic loop is the while loop and it is used is to repeat a block of code. The while loop has on control expression (a specific condition) and executes as long as the given expression is true. Here is the syntax: ```c variable initialization; while (condition) { statements; variable increment or decrement; } ``` - **For Loop:** For loop is used to execute a set of statements repeatedly until a particular condition is satisfied. We can say this is an open ended loop. ```c for(initialization; condition; increment/decrement) { statement-block; } ``` - **Do-while Loop:** In some situations it is necessary to execute body of the loop before testing the condition. Such situations can be handled with the help of do-while loop. do statement evaluates the body of the loop first and at the end, the condition is checked using while statement. It means that for at least one time, the body of the loop will be executed, even though the starting condition inside while is initialized to false. ```c do { } while(condition) ``` #### 2.6.1 C Loop Control Statements: Loop control statements are used to change normal sequence of execution of loop. | Statement | Syntax | Description | | :------------- | :-------------- | :------------------------------------------------------------------------------------------------------------------------ | | break statement | break; | Is used to terminate loop or switch statements. | | continue statement | continue; | Is used to suspend the execution of current loop iteration and transfer control to the loop for the next iteration. | | goto statement | labelName: statement; | It's transfer current program execution sequence to some other part of the program. | #### 2.7. Functions in C Programming Functions are used to provide modularity to the software. By using functions, you can divide complex tasks into small manageable tasks. The use of functions can also help avoid duplication of work. For example, if you have written the function for calculating the square root, you can use that function in multiple programs. - **Syntax of function:** ``` Arguments list Function header Function (argl, arg2, arg3) type argl, arg2, arg3 Arguments declarations { } statement 1; statement2; statement3; Function body statement4; ``` - **The general format of a function is:** ```` <Return type> <Function name> <Parameter list> { <local definitions> executable statements; Return (expression); } ```` - **Example:** ```c int f1 (int j, float f) { int k; k = 1; return (k); } ``` - **Formal Arguments:** The arguments specify in function header is called as formal arguments because they represent the names of data items that are transferred in to the function from the calling portion of the program. They are also called as formal parameters. - **Actual Arguments:** The arguments specify in function call is called as actual arguments. Since they define the data items that are actually transferred. They are also called as actual parameters or simply arguments. - **Example:** ```c main () { int i, j, k; i = 10; j = 20; k = add(i, j); //D /* This is the function call and 'i' and 'j' are the actual parameters */ } ``` #### 2.7.1 Function call Functions cannot execute themselves with exception to main (). In a C program only main is executable. All other functions are not. If this is true, then there must be some way by which the functions executes. This is known as function call. A function can be called from another function with exception to main() function. This means main() functions can call other functions but other functions cannot call main(). If by curiosity you happen to call main() function and it compiled well then you may end up in infinite loop. A function is called from within another function by just putting its name inside that function with proper parameters and if it returns anything then you must supply a proper variable to catch the value. Before you can call the function, it should be defined or at least prototype. #### 2.8. Parameter Passing Information can be passed from one function to another using parameters. - **Example:** ```c Main () { int i; i = 0; printf (" The value of i before call %d \n", i); /* The value of 'i' is 0 */ f1 (i); printf (" The value of i after call %d \n", i); /* The value of 'i' is still 0 */ } void f1 (int k) { k = k + 10; /* the value of 'k' is now 10 */ } ``` - **Explanation:** - The parameter used for writing the function is called the formal parameter, k in this case. - The argument used for calling the function is called the actual parameter. - The actual and formal parameters may have the same name. - When the function is called, the value of the actual parameter is copied into the formal parameter. Thus k gets the value 0. This method is called parameter passing by value. - Since only the value of i is passed to the formal parameter k, and k is changed within the function, the changes are done in k and the value of i remains unaffected. - Thus i will equal 0 after the call; the value of i before and after the function call remains the same. #### 2.9. Categories of function A Function in C belongs to one of the following categories: - Function with no arguments and no return values - Function with arguments and no return values - Function with no arguments and return values - Function with arguments and return values #### 2.10. Scope rules: - **Local Variables:** A local variable is a variable that is given local scope. Such variables are accessible only from the function or block in which it is declared. Local variables are contrasted with global variables. Local variables are special because in most languages they are automatic variables stored on the call stack directly. This means that when a recursive function calls itself, local variables in each instance of the function are given separate memory address space. Hence variables of this scope can be declared, written to, and read, without any risk of side-effects to processes outside of the block in which they are declared. - **Global variables:** These variables can be accessed (i.e. known) by any function comprising the program. They are implemented by associating memory locations with variable names. They do not get recreated if the function is recalled. Global variables are used extensively to pass information between sections of code that don't share a caller/callee relation like concurrent threads and signal handlers. In Other word Global variables are those, which are available throughout the program's execution. They are not defined inside any block. They exist in memory until the program is in memory. #### 2.11. Parameter passing technique Parameters are the values upon which we want the function should operate. These are passed to functions within parenthesis. The mechanism by which parameters are passed to function is known as parameter passing. There are basically 2-types of techniques - **Call by value** - **Call by reference** **Call by value:** In call by value method if the formal parameters are changed then the values in calling function will not change. This is because formal parameters are local to the function, and actual parameters are local to the calling function. But there are certain cases we need to change the value of the variable from inside a function. This is usually done in pass by reference method or else it can be done only in case of global variable. Only global variables can be changed from within function. Here an interesting thing comes to mind. If we have defined a global variable and a local variable with same name then if we change the value then whose value will be changed, let us see it. Consider the following examples. - **Example:** ```c main () { int a = 10, b=20; swapy (a,b); /* Pass by value occurs here */ printf ("\na = % d b = % d", a,b); /* The values of a and b remain the same as 10 and 20 respectively */ swapy (int x, int y) { int t; t = x; x = y; /* The value of 'x' changes to 20 */ y = t; /* The value of 'y' changes to 10 */ printf ("\n x = % d y = % d", x, y); /* Prints x as 20 and y as 10 */ } } ``` - **Output:** ``` a = 10 b = 20 x = 20 y = 10 ``` **Call by Reference:** Call by reference is implemented indirectly by passing the address of the variable. implement the function indirectly. This is done by passing the address of the variable and changing the value of the variable through its address. - **Example:** ```c main () { int a = 10, b =20, /* The '&' symbol indicates that the address of the variable is being passed */ swapr (&a, &b); printf ("\n a = %d b= %d", a, b); /* Prints 'a' as 20 and 'b' as 10 */ swapr (int *x, int * y) /* 'x' and 'y' are pointers to the addresses of the variables in 'main()' */ { int t; t= *x /* 't' stores the value stored in the address pointed to by 'x' */ *x = *y; /* The value stored in the address pointed to by 'y' is copied into the address pointed to by 'x' */ *y = t; /* The value stored in 't' is copied into the address pointed to by 'y' */ printf ("\n x = % d y = % d", *x, *y); /* Prints 'x' as 20 and 'y' as 10 */ } /* We are working with the addresses of the variables, not their actual values */ } ``` - **Output:** ``` a = 20 b= 10 x = 20 y = 10 ``` #### 2.12. Recursion Recursion is the phenomenon of function calling itself repeatedly. Consider a function calls itself from within itself. Recursion is useful in the situation, where some series of similar and dependent calculations are needed. The best example is factorial. Factorial of n(n!) can be written as n*(n-1)*(n-2)*(n-3)*......*2*1 So, it is a continued product from n to 1. Hence, we can rewrite it as n*factorial of(n-l). From this we can write a function to calculate the factorial of a number ```c int factorial(int n) { if(n<=l) return(l); else return(n*factorial(n-1)); } ``` - **Example:** ```c /* to calculate the factorial through recursion */ #include<stdio.h> #include<conio.h> int fact(int); void main() { int n,result; clrscr(); printf("Enter the number"); scanf("%d",&n); result=fact(n); printf("\n The factorial of %d is %d",n,result); getch(); } int fact(int x) { int f; if(x==1) { return(x); } else { return(x*fact(x-1)); } } ``` #### 2.13. Pointer A pointer is a variable that holds a memory address, usually the location of another variable in memory. Pointer is a variable, which contains memory address. This address is the location of another variable in memory. Consider 2 variables. If the first variable contains the address of second variable, then it is said the first variable points to second variable. The declaration of a variable consists of type (any valid type), '*' and variable name. The syntax for this purpose is as follows `<type> * <variable name>;` The type defines here which type of data the pointer is going to point. This means which type of variables address this pointer will point to. The "&" operator gets the address of any variable and the '*' operator counters the '&' operator. That is gets the value at that address taking into consideration the base type. Let us see an example ```c int a, b; int *ptr1, *ptr2; /*Here ptr1 and ptr2 are two pointer type variables, which can contain the address of 2 integer variables*/ ptr1=&a; ptr2=&b; ``` In the above example ptr1 contains the address of a and ptr2 contains the address of b. By address it is meant the memory location of the variable at the run time. Suppose at run time a is at location 1004 and it has a value of say 50 then ptr1 will contain 1004 not 50. Let us now check the * operator. This operator gets the value. So, to get the value stored at any location we can write `m=*ptr1;` Here type of m and base type of ptr1 must be same. Now contains the value stored at address pointed by ptr1, that is value of a. A pointer can be assigned to another pointer type variable of same base type Consider the following code. ```c int a int *ptr1, *ptr2; ptrl=&a; /* ptr1 is assigned the address of a */ ptr2=ptr1; /* ptr2 also stores the address of a */ printf("%p%p", ptr1, ptr2); /* prints the addresses of ptr1 and ptr2 */ ``` The above code shows assigning of the address of a variable in to a pointer type variable. Then we store the address stored in one pointer type variable in another pointer type variable. Then in last statement we are printing the address stored in those pointers. You can notice we are using a %p a here to print the address. #### Array of Pointer If array of any data type is possible then array of pointers is also possible. In this case each element of the array is a pointer to any data type. Suppose we want to create an array of integer pointer then we should write in following manner. ```c int k,l,m,n; int *a[4]; /* here a is an array of pointe to integer */ /*hence we can write*/ a[0]=&k; a[1]=&l a[2]=&m a[3]=&n; ``` #### Multidimensional Array We read earlier how to represent multi-dimensional arrays using pointers. Now we will look into how to access the elements of multi-dimensional array. Follow the example bellow. ```c Int a[3][3][3]; /* is a multi-dimensional array*/ Int p,x,y,z,; Int ***k; /* k is a pointer to pointe to pointer to integer */ /* hence k can hold the address of 3-diensional array */ k=a; /*now we will store values into the array*/ for(x=0;x<3;x++) { for(y=0;y<3;y++) { for(z=0;z<3;z++) printf'("Enter element %d %d %d\n", x, y, z); scanf("%d",&p); /* read the element */ *(*(*(k+x)+y)+z)=p; /* store the element in array */ } /* now to print the values */ for(x=0;x<3;x++) { for(y=0;y<3;y++) { for(z=0; z<3;z++) { p=*(*(*(k+x)+y)+z); printf ("The Element %d %d %d= %d\n", x, y,z, p); } } } } ``` #### Function and pointer We have discussed pass by value method in functions. In that case the value is passed to the function. But in case of pass by reference we pass the address of variables to the called function. As the address of variable is passed, any modification of value at that address will be reflected in the calling function. Hence this should be considered seriously. The pass by reference is advantageous when we pass array, or we need the variables value should be changed by the function. Consider the case of a swapping function we need to alter the values and that should be available in calling function. We know a function can return only one value hence pass by value is not possible. So, it is done using pass by reference. Consider the following swapping example ```c #include<stdio.h> } void swap (int *p, int *q) /* we take two pointers */ int * temp /* temporary pointer variable */ *temp= * /*'store the value stored in p*/ *p= *q; /* store value stored in p into q*/ *q= *temp; main() { int a=10 b=30; printf("value of a =%d and of b = %d before swap( ) \ n", a, b ); swap (&a, &b); /* passing addresses*/ printf("value of a = %d and of b = %d before swap( ) \ n", a, b ); } ``` #### 2.14. Arrays Array means a group of similar type of things. In C programming it means a collection of similar type of data in consecutive memory locations, as an example array of integers array of floats etc. - **Types of Arrays:** - **Single-dimensional array:** the simplest form of an array is a single dimensional array. The array is given a name and is elements are referred to by their subscripts or indices. - **Introduction to Array Variables** - Arrays are a data structure which hold multiple values of the same data type. Arrays are an example of a structured variable in which 1) there are a number of pieces of data contained in the variable name, and 2) there is an ordered method for extracting individual data items from the whole. - Consider the case where a programmer needs to keep track of the ID numbers of people within an organization. Her first approach might be to create a specific variable for each user. This might look like ```c int id1 = 101; int id2 = 232; int id3 = 231; ``` It becomes increasingly more difficult to keep track of the IDs as the number of variables increase. Arrays offer a solution to this problem. - **Array Variables Example** - An array is a multi-element box, a bit like a filing cabinet, and uses an indexing system to find each variable stored within it. In C, indexing starts at zero. Arrays, like other variables in C, must be declared before they can be used. - The replacement of the previous example using an array looks like this: ```c int id[3]; /* declaration of array id */ id[0] = 101; id[1] = 232; id[2] = 231; ``` - In the first line, we declared an array called id, which has space for three integer variables. Each piece of data in an array is called an element. Thus, array id has three elements. After the first line, each element of id is initialized with an ID number. - **Memory Representation of Single Dimension Arrays** - Single -dimension arrays are essentially lists of information of the same type and their elements are stored in contiguous memory location in their index order. For instance, an array grade of type char with 8 elements declared as char grade[8]; will have the element grade[0] at the first allocated memory location, grade[1] at the next contiguous memory location, grade[2] at the next, and so forth. Since grade is a char type array, each element size is 1 byte(A character size is 1 byte) and it will be represented in memory as shown below ```c grade[0] grade[1]. Address 101 102 103 104 105 106 107 108 ......grade[7] ``` If you have an int array age with 5 elements declared as int age[5]; Its each element will have size of(int)1 bytes for its storage. On a system with 2 bytes integer size, the array age's each element (being an integer) will be having 2 bytes for its storage. If the starting memory location of array age is 100, then it will be represented in the memory as shown below ```c age[0] age[1] age[2] age[3] age[4] Address 100 102 104 106 108 ``` - **Declaring Arrays** - Arrays may consist of any of the valid data types. Arrays are declared along with all other variables in the declaration section of the program and the following syntax is used ```c type array_name[n]; grade age ``` where n is the number of elements in the array. Some examples are ```c int final[160]; float distance[66]; ``` - During declaration consecutive memory locations are reserved for the array and all its elements. After the declaration, you cannot assume that the elements have been initialized to zero. Random junk is at each element's memory location. - **Initializing Arrays during Declaration** - If the declaration of an array is preceded by the word static, then the array can be initialized at declaration. The initial values are enclosed in braces. e.g., static int value[9] = {1,2,3,4,5,6,7,8,9}; static float height[5]={6.0,7.3,2.2,3.6,19.8}; - Some rules to remember when initializing during declaration - If the list of initial elements is shorter than the number of array elements, the remaining elements are initialized to zero. - If a static array is not initialized at declaration manually, its elements are automatically initialized to zero. - If a static array is declared without a size specification, its size equals the length of the initialization list. In the following declaration, a has size 5. ```c static int a[]={-6,12,18,2,323}; ``` - **Using Arrays** - Recall that indexing is the method of accessing individual array elements. Thus grade[89] refers to the 90th element of the grades array. A common programming error is out-of-bounds array indexing. Consider the following code: ```c int grade[3]; grade[5] = 78; ```