LAB ASSIGNMENT 04 real PDF
Document Details
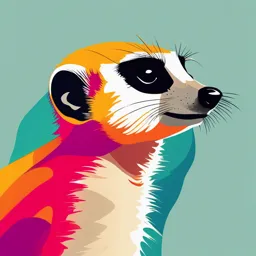
Uploaded by AbundantIntegral
unknown
Sawan Parmar
Tags
Summary
This document contains C programming code for converting infix notation to postfix notation and evaluating postfix expressions. It also demonstrates the implementation of stacks and queues using linked lists. The provided code implements basic operations on these data structures.
Full Transcript
SAWAN PARMAR 0801IT231117 LAB ASSIGNMENT-04 Q.14: Write a program to convert infix notation to postfix notation. #include #include #include #define MAX 100 struct Stack { int top; char...
SAWAN PARMAR 0801IT231117 LAB ASSIGNMENT-04 Q.14: Write a program to convert infix notation to postfix notation. #include #include #include #define MAX 100 struct Stack { int top; char items[MAX]; }; void initStack(struct Stack *s) { s->top = -1; } int isEmpty(struct Stack *s) { return (s->top == -1); } void push(struct Stack *s, char value) { if (s->top == MAX - 1) { printf("Stack Overflow\n"); } else { s->items[++(s->top)] = value; } } SAWAN PARMAR 0801IT231117 char pop(struct Stack *s) { if (isEmpty(s)) { printf("Stack Underflow\n"); return '\0'; } else { return s->items[(s->top)--]; } } char peek(struct Stack *s) { if (isEmpty(s)) { return '\0'; } else { return s->items[s->top]; } } int isOperator(char ch) { return (ch == '+' || ch == '-' || ch == '*' || ch == '/' || ch == '^'); } int precedence(char ch) { switch (ch) { case '^': return 3; case '*': case '/': return 2; case '+': SAWAN PARMAR 0801IT231117 case '-': return 1; default: return 0; } } void infixToPostfix(char infix[], char postfix[]) { struct Stack s; initStack(&s); int i, j = 0; for (i = 0; infix[i] != '\0'; i++) { char token = infix[i]; if (isalnum(token)) { postfix[j++] = token; } else if (token == '(') { push(&s, token); } else if (token == ')') { while (!isEmpty(&s) && peek(&s) != '(') { postfix[j++] = pop(&s); } pop(&s); } else if (isOperator(token)) { while (!isEmpty(&s) && precedence(peek(&s)) >= precedence(token)) { postfix[j++] = pop(&s); } push(&s, token); } } while (!isEmpty(&s)) { SAWAN PARMAR 0801IT231117 postfix[j++] = pop(&s); } postfix[j] = '\0'; } int main() { char infix[MAX], postfix[MAX]; printf("Enter an infix expression: "); scanf("%s", infix); infixToPostfix(infix, postfix); printf("Postfix expression: %s\n", postfix); printf("SAWAN PARMAR\n0801IT231117\n"); return 0; } SAWAN PARMAR 0801IT231117 Q.15:Write a program to evaluate postflix expression using Stack. #include #include #include #define MAX 100 struct Stack { int top; int items[MAX]; }; void initStack(struct Stack* s) { s->top = -1; } int isFull(struct Stack* s) { return s->top == (MAX - 1); } int isEmpty(struct Stack* s) { return s->top == -1; } void push(struct Stack* s, int value) { if (!isFull(s)) { s->items[++(s->top)] = value; } SAWAN PARMAR 0801IT231117 } int pop(struct Stack* s) { if (!isEmpty(s)) { return s->items[(s->top)--]; } return -1; } int evaluatePostfix(char* expression) { struct Stack s; initStack(&s); for (int i = 0; expression[i] != '\0'; i++) { if (isdigit(expression[i])) { push(&s, expression[i] - '0'); } else { int operand2 = pop(&s); int operand1 = pop(&s); switch (expression[i]) { case '+': push(&s, operand1 + operand2); break; case '-': push(&s, operand1 - operand2); break; case '*': push(&s, operand1 * operand2); SAWAN PARMAR 0801IT231117 break; case '/': push(&s, operand1 / operand2); break; } } } return pop(&s); } int main() { char expression[MAX]; printf("Sawan Parmar\n0801IT231117\n\n"); printf("Enter postfix expression: "); scanf("%s", expression); printf("Result: %d\n", evaluatePostfix(expression)); return 0; } SAWAN PARMAR 0801IT231117 Q.16: Write a program to implement QUEUE using link list that performs following operations (a) INSERT (b) DELETE (e) DISPLAY. #include #include struct Node { int data; struct Node* next; }; struct Queue { struct Node* front; struct Node* rear; }; struct Node* createNode(int data) { struct Node* newNode = (struct Node*)malloc(sizeof(struct Node)); newNode->data = data; newNode->next = NULL; return newNode; } void initQueue(struct Queue* q) { q->front = q->rear = NULL; SAWAN PARMAR 0801IT231117 } int isEmpty(struct Queue* q) { return (q->front == NULL); } void insert(struct Queue* q, int data) { struct Node* newNode = createNode(data); if (q->rear == NULL) { q->front = q->rear = newNode; } else { q->rear->next = newNode; q->rear = newNode; } printf("%d inserted into the queue.\n", data); } void delete(struct Queue* q) { if (isEmpty(q)) { printf("Queue is empty, cannot delete.\n"); return; } struct Node* temp = q->front; q->front = q->front->next; if (q->front == NULL) { q->rear = NULL; } printf("Deleted element: %d\n", temp->data); SAWAN PARMAR 0801IT231117 free(temp); } void display(struct Queue* q) { if (isEmpty(q)) { printf("Queue is empty.\n"); return; } struct Node* temp = q->front; printf("Queue elements: "); while (temp != NULL) { printf("%d ", temp->data); temp = temp->next; } printf("\n"); } int main() { struct Queue q; initQueue(&q); int choice, value; do { printf("\nQueue Operations:\n"); printf("1. Insert\n"); printf("2. Delete\n"); printf("3. Display\n"); printf("4. Exit\n"); printf("Enter your choice: "); SAWAN PARMAR 0801IT231117 scanf("%d", &choice); switch (choice) { case 1: printf("Enter value to insert: "); scanf("%d", &value); insert(&q, value); break; case 2: delete(&q); break; case 3: display(&q); break; case 4: printf("Exiting...\n"); break; default: printf("Invalid choice, please try again.\n"); } } while (choice != 4); printf("SAWAN PARMAR\n0801IT231117\n"); return 0; } SAWAN PARMAR 0801IT231117 Q.17: Write a program to implement Queue (FIFO) using link list. #include #include struct Node { int data; struct Node* next; }; struct Queue { struct Node *front, *rear; }; struct Node* newNode(int data) { struct Node* temp = (struct Node*)malloc(sizeof(struct Node)); temp->data = data; temp->next = NULL; return temp; } struct Queue* createQueue() { struct Queue* q = (struct Queue*)malloc(sizeof(struct Queue)); q->front = q->rear = NULL; return q; } void enqueue(struct Queue* q, int data) { struct Node* temp = newNode(data); SAWAN PARMAR 0801IT231117 if (q->rear == NULL) { q->front = q->rear = temp; return; } q->rear->next = temp; q->rear = temp; } int dequeue(struct Queue* q) { if (q->front == NULL) return -1; struct Node* temp = q->front; int data = temp->data; q->front = q->front->next; if (q->front == NULL) q->rear = NULL; free(temp); return data; } void displayQueue(struct Queue* q) { struct Node* temp = q->front; if (temp == NULL) { printf("Queue is empty\n"); return; } printf("Queue: "); while (temp) { SAWAN PARMAR 0801IT231117 printf("%d -> ", temp->data); temp = temp->next; } printf("NULL\n"); } int main() { printf("SAWAN PARMAR\n0801IT231117\n\n"); struct Queue* q = createQueue(); enqueue(q, 10); enqueue(q, 20); enqueue(q, 30); displayQueue(q); printf("Dequeued: %d\n", dequeue(q)); displayQueue(q); enqueue(q, 40); displayQueue(q); return 0; } SAWAN PARMAR 0801IT231117 Q.18: Write a program to implement circular Queue using array that perforoms following operations a) INSERT b) DELETE c) DISPLAY. #include #include #define SIZE 5 struct CircularQueue { int items[SIZE]; int front, rear; }; void initQueue(struct CircularQueue* q) { q->front = -1; q->rear = -1; } int isFull(struct CircularQueue* q) { if ((q->front == 0 && q->rear == SIZE - 1) || (q->rear == (q->front - 1) % (SIZE - 1))) { return 1; } return 0; } int isEmpty(struct CircularQueue* q) { if (q->front == -1) { return 1; } SAWAN PARMAR 0801IT231117 return 0; } void insert(struct CircularQueue* q, int value) { if (isFull(q)) { printf("Queue is full!\n"); return; } if (q->front == -1) q->front = 0; q->rear = (q->rear + 1) % SIZE; q->items[q->rear] = value; printf("Inserted %d\n", value); } void delete(struct CircularQueue* q) { if (isEmpty(q)) { printf("Queue is empty!\n"); return; } int value = q->items[q->front]; if (q->front == q->rear) { q->front = -1; q->rear = -1; } else { q->front = (q->front + 1) % SIZE; } printf("Deleted %d\n", value); SAWAN PARMAR 0801IT231117 } void display(struct CircularQueue* q) { if (isEmpty(q)) { printf("Queue is empty!\n"); return; } printf("Queue: "); int i = q->front; while (i != q->rear) { printf("%d ", q->items[i]); i = (i + 1) % SIZE; } printf("%d\n", q->items[q->rear]); } int main() { printf("SAWAN PARMAR\n0801IT231117\n\n"); struct CircularQueue q; initQueue(&q); insert(&q, 10); insert(&q, 20); insert(&q, 30); insert(&q, 40); insert(&q, 50); display(&q); delete(&q); delete(&q); SAWAN PARMAR 0801IT231117 display(&q); insert(&q, 60); insert(&q, 70); display(&q); return 0; } SAWAN PARMAR 0801IT231117 Q.19: Write a program to implement circular queue using link list. #include #include struct Node { int data; struct Node* next; }; struct CircularQueue { struct Node *front, *rear; }; struct Node* createNode(int value) { struct Node* newNode = (struct Node*)malloc(sizeof(struct Node)); newNode->data = value; newNode->next = NULL; return newNode; } struct CircularQueue* createQueue() { struct CircularQueue* queue = (struct CircularQueue*)malloc(sizeof(struct CircularQueue)); queue->front = queue->rear = NULL; return queue; } void enqueue(struct CircularQueue* queue, int value) { SAWAN PARMAR 0801IT231117 struct Node* newNode = createNode(value); if (queue->rear == NULL) { queue->front = queue->rear = newNode; queue->rear->next = queue->front; } else { queue->rear->next = newNode; queue->rear = newNode; queue->rear->next = queue->front; } printf("Enqueued %d\n", value); } int dequeue(struct CircularQueue* queue) { if (queue->front == NULL) { printf("Queue is empty, cannot dequeue.\n"); return -1; } int value; struct Node* temp = queue->front; if (queue->front == queue->rear) { value = queue->front->data; free(queue->front); queue->front = queue->rear = NULL; } else { value = queue->front->data; queue->front = queue->front->next; queue->rear->next = queue->front; free(temp); SAWAN PARMAR 0801IT231117 } printf("Dequeued %d\n", value); return value; } void displayQueue(struct CircularQueue* queue) { if (queue->front == NULL) { printf("Queue is empty.\n"); return; } struct Node* temp = queue->front; printf("Queue elements are: "); do { printf("%d ", temp->data); temp = temp->next; } while (temp != queue->front); printf("\n"); } int main() { printf("SAWAN PARMAR\n0801IT231117\n\n"); struct CircularQueue* queue = createQueue(); enqueue(queue, 10); enqueue(queue, 20); enqueue(queue, 30); enqueue(queue, 40); displayQueue(queue); dequeue(queue); SAWAN PARMAR 0801IT231117 displayQueue(queue); enqueue(queue, 50); displayQueue(queue); return 0; } SAWAN PARMAR 0801IT231117 Q.20: Write a program to implement priority queue using link list. #include #include struct Node { int data; int priority; struct Node* next; }; struct Node* createNode(int value, int priority) { struct Node* newNode = (struct Node*)malloc(sizeof(struct Node)); newNode->data = value; newNode->priority = priority; newNode->next = NULL; return newNode; } void enqueue(struct Node** head, int value, int priority) { struct Node* newNode = createNode(value, priority); if (*head == NULL || (*head)->priority > priority) { newNode->next = *head; *head = newNode; } else { struct Node* temp = *head; while (temp->next != NULL && temp->next->priority next; } SAWAN PARMAR 0801IT231117 newNode->next = temp->next; temp->next = newNode; } printf("Enqueued %d with priority %d\n", value, priority); } int dequeue(struct Node** head) { if (*head == NULL) { printf("Priority Queue is empty, cannot dequeue.\n"); return -1; } int value = (*head)->data; struct Node* temp = *head; *head = (*head)->next; free(temp); printf("Dequeued %d\n", value); return value; } void displayQueue(struct Node* head) { if (head == NULL) { printf("Priority Queue is empty.\n"); return; } struct Node* temp = head; printf("Priority Queue elements (value, priority): "); while (temp != NULL) { printf("(%d, %d) ", temp->data, temp->priority); SAWAN PARMAR 0801IT231117 temp = temp->next; } printf("\n"); } int main() { printf("SAWAN PARMAR\n0801IT231117\n\n"); struct Node* priorityQueue = NULL; enqueue(&priorityQueue, 10, 2); enqueue(&priorityQueue, 30, 1); enqueue(&priorityQueue, 20, 3); enqueue(&priorityQueue, 40, 0); displayQueue(priorityQueue); dequeue(&priorityQueue); displayQueue(priorityQueue); enqueue(&priorityQueue, 50, 1); displayQueue(priorityQueue); return 0; }