Input and Output PDF
Document Details
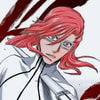
Uploaded by Kennzueee
Our Lady of Fatima University
Soma, T. & Streib, J.
Tags
Summary
This document explains input and output concepts using Java. It demonstrates how to display output to a screen and takes input from a keyboard using the Java programming language. The examples provided cover basic input and output operations in Java and explain the role of the `println` and `print` methods.
Full Transcript
IT2402 Input and Output Input is the process of entering data in software running on a computer through typed text, various types of files, or mouse commands. Word processing programs such as Microsoft Word often take its input as alphanumerical data acco...
IT2402 Input and Output Input is the process of entering data in software running on a computer through typed text, various types of files, or mouse commands. Word processing programs such as Microsoft Word often take its input as alphanumerical data according to a user’s keystroke. Output is the data processed by the software that can be directed at printers, screens, monitors, and other devices that display output. Programmers’ output is usually a working application or software. Output (Soma, T. & Streib, J., 2023) A program must perform or produce any type of output to be considered useful. The output can be in any form such as output to a screen, a disk, a printer, or even in a form of movement found in robots in a manufacturing site. In programming, only output to a screen is expected. The “Hello World!” program is one of the most common first programs when learning a new language, as it ensures that the beginner is writing a program correctly and using the compiler properly. 1 class OutputSample { 2 public static void main(String[] args){ 3 System.out.println("Hello World!"); 4 } 5 } To refresh, the line 3 is a Java statement. This statement outputs the string within the double quotation marks to the screen. The standard output device, by default, is the computer monitor. The Java statement is terminated with a semi-colon (;). Java uses System.out to refer to the console output, the output sent to the console. Console output is performed by simply using the println method to display a primitive value or a string to the monitor. The println method is pronounced as “print line,” although it is not spelled that way. The println method is part of the Java API, a predefined set of classes used in any Java program that are not part of the language. The print in println causes the string in the parenthesis to be the output to the computer screen. The ln, however, causes the cursor on the screen to move down to the next line. This sample program displays Hello World! with a _ (cursor) on the second line, provided there are no errors. The double quotation marks are not considered output to the screen, and the cursor (_) appears on the next line. Using the same sample program, if ln is removed, such as: System.out.println("Hello World!"); The output becomes: Hello World! The difference this time is the cursor is placed next to or at the end of the string rather than displayed on the second line. There are instances where the cursor does not appear on the screen and the difference could not be observed. It is still important to know where the cursor is located so that the output is correct. The following statements display the strings on two lines and the cursor on the next line. 05 Handout 1 *Property of STI [email protected] Page 1 of 3 IT2402 Hello System.out.println("Hello"); World! System.out.println("World!"); The following statements display the strings on the same line since neither included ln, and no space at the end of the strings was indicated. System.out.print("Hello"); HelloWorld! System.out.print("World!"); The following statements can mimic the proper format of the output, but this one requires three (3) statements. System.out.print("Hello "); Hello World! System.out.print("World! "); System.out.println(); Adding a System.out.println(); statement does not always produce a blank line. The only time a blank line is produced is when there is not a preceding System.out.print(); statement on the same code segment. Input (Soma, T. & Streib, J. 2023) Inputs, much like outputs, can come from different sources, such as a mouse, a keyboard, a disk, or even sensors on a robot in the industrial industries. The input process is vital as it is the way to change the output of a program anytime the programmer wants to modify it through editing and recompiling it before executing it again. The import statement is added to use a predefined method for input. All predefined classes and methods in Java API are organized into packages. The packages that will be used in a program are identified by the import statement. The import.java.util.Scanner; statement can be used to import the Scanner class of the java.util package. 1 import java.util.*; 2 class InputOutput { 3 public static void main(String[] args) { 4 int num; 5 6 Scanner scanner; 7 scanner = new Scanner(System.in); 8 9 num = scanner.nextInt(); 10 System.out.println("The integer is " + num); 11 } 12 } The program above uses an asterisk (*) to indicate that any class inside the java.util package can be used and referenced in the program. It includes classes that support collections, data, and calendar operations. Another package is java.lang, which includes the System and Math classes. This package is automatically imported into all Java programs so this package does not need to be imported. 05 Handout 1 *Property of STI [email protected] Page 2 of 3 IT2402 In the given program, for input to work properly, a place to store the data entered is needed. The first statement declared in the main method (line 4) is the variable num as type int. The next statement (line 6) is the declaration of the variable scanner of type Scanner, which is not a primitive data type but a class. Line 7, which has the scanner = new Scanner(System.in); statement, creates a new instance of the Scanner class, in other words, a Scanner object. Java uses System.in to refer to the keyboard, which is considered the standard input device. Input is not directly supported by Java, unlike output. The Scanner class can be used to create an object to get input from the keyboard. The statement on line 7 assigns a reference to the new object to the variable scanner. The import statement and the statements in lines 6 and 7 are a must in any program that needs to input data. The statement on line 9, shows the Scanner object is used to scan the input for the next integer. The nextInt() method makes the system wait until an integer is entered from the keyboard. Once an integer is entered, it is assigned to the variable num. Side note, nextDouble() is used to read double data type numbers, the next() method reads a word of a String type that ends before a space, and the nextLine() method reads an entire line of text of type String. If the code above is executed, a blinking cursor will appear on the console output or the monitor. For regular people who are not familiar with programming, they might not know what to do when they see this. A System.out.print("Enter an integer: "); statatemet before the nextInt() method can be useful. It will serve as a prompt which is an output message to understand what is expected to be entered. A prompt must also be user-friendly and not aggressive and must state what value needs to be entered. The final code will look like this: 1 import java.util.*; 2 class InputOutput { 3 public static void main(String[] args) { 4 int num; 5 6 Scanner scanner; 7 scanner = new Scanner(System.in); 8 9 System.out.print("Enter an integer: "); //new added prompt 10 num = scanner.nextInt(); 11 System.out.println("The integer is " + num); 12 } 13 } The result of the program should be similar to the following: Enter an integer: 8 The integer is 8 _ References: Agarwal, S. & Bansal, H. (2023). Java in depth. BPB Publications. Ciesla, R. (2021). Programming basics: Getting started with Java, C#, and Python. Apress. Soma, T. & Streib, J. (2023). Guide to Java: A concise introduction to programming. Springer. 05 Handout 1 *Property of STI [email protected] Page 3 of 3