Introduction to Programming and Problem Solving (CS101) Lecture 9 PDF
Document Details
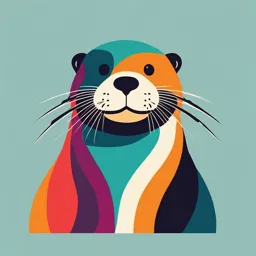
Uploaded by ComelySeal8346
Ibrahim Shawky
Tags
Summary
This document covers programming fundamentals, particularly loops and some C# examples. Various aspects of programming loops are investigated, and example solutions are provided, including examples of calculating salary increments and showing multiplication tables.
Full Transcript
Introduction to Programming and Problem Solving (CS101) Lecture 9 Prepared by/ Ibrahim Shawky while Loop Structure hile loop structure evaluates the condition (i.e. Expression2) first then perform or he action(s) based on the value of the condition. body of...
Introduction to Programming and Problem Solving (CS101) Lecture 9 Prepared by/ Ibrahim Shawky while Loop Structure hile loop structure evaluates the condition (i.e. Expression2) first then perform or he action(s) based on the value of the condition. body of the loop may not be executed if the value of the condition evaluated to be Write a program using while loop to print the ello world 10 times on screen Write a program using while loop to print the umber from 1 to 10 on screen Write a program using while loop to print the ven numbers from 1 to 10 on screen Write a program using while loop to print the ven numbers from 10 to 1 on screen do …while Loop Structure The do … while loop structure perform statement first then evaluates the condition (i.e. Expression2). The body of the loop will be executed at least once even if the value of the condition evaluates false. Write a program using do while loop to print he hello world 10 times on screen Write a program using do while loop to print he number from 1 to 10 on screen Write a program using do while loop to print he even numbers from 1 to 10 on screen Write a program using do while loop to print he even numbers from 10 to 1 on screen xample 6.9: Write code segment that prints the summation of the first n numbers. Example 6.10: Write statement that prints the factorial of a number. Write a code segment that calculates the esult of for any integer numbers x and y. break vs. continue Statement Continue example Continue output reak example Break output lculates the sum of an unspecified number of tegers. When the user enters non positive mber, the loop has to terminate its processing. xample 6: write a program to calculate the alary for the employee for next five years with increment 10% per year? Nested Loop Nested loops refer to the process of placing one or more loop constructs inside the body of another loop. It consists of an outer loop that contains one or more inner loops. Each time the outer loop iterates once, the inner loops execute their complete iterations. Nested for Loop Write the program that show the ollowing result Solution Example 7: Write a program which produces a simple multiplication table of the following format for integers in the range 1 to 9: 1x1=1 1x2=2... 9 x 9 = 81 Solution Example 7: Write a program which produces a simple multiplication table of the following format for integers in the range 1 to 9: Solution Example 7: Write a program which produces a simple multiplication table of the following format for integers in the range 1 to 9: Solution Example 7: Write a program which produces a simple multiplication table of the following format for integers in the range 1 to 9: Solution Write the program that show the ollowing result Solution Solution Exercise Write a program that print the summation of odd numbers from 1 to 100 using: (a) for loop structure. (b) while loop structure. (c) do… while loop structure. Exercise rite a program which produces a simple ultiplication table of the following format for tegers in the range 1 to 9: Exercise Write the program that show the following result Exercise write a program to calculate the salary for the employee for next five years with increment 10% per year? Exercise Write C# Program to check if a number is prime or not Exercise Write a program that print all prime number between 1 and 1000 Introduction to Programming and Problem Solving (CS101) Lecture 6 Prepared by/ Ibrahim Shawky How to add two specific number الخالصه في انك تعمل الجورزم جوه دماغك How to add two specific number using System; namespace MyApplication { class Program { static void Main(string[] args) { int x ; x= 5; int y; x = 6; int z; z = x + y; Console.WriteLine(z); // Print the sum of x + y }}} How to add two specific number using System; namespace MyApplication { class Program { static void Main(string[] args) { int x,y,z ; x= 5; x = 6; z = x + y; Console.WriteLine(z); // Print the sum of x + y } } } Basic Structure of C# Program The program made up of one or more namespace. Each namespace contains one or more class. Each class contains one or more method. Each method contains one or more statement Basic Structure of C# Program Line 1 of the program using System; The using keyword is used to include the System namespace in the program. It causes the compiler to include some functionality needed to build the program. Line 2 has the namespace declaration. A namespace is a collection of classes. The HelloWorldApplication namespace contains the class HelloWorld. Line 3 contains the opening brace ({) marks the beginning of the namespace. Basic Structure of C# Program Line 4 has a class declaration. Classes generally would contain more than one method. However, the HelloWorld class has only one Main method. Line 5 contains the opening brace ({) marks the beginning of the class. Line 6 defines the Main method, which is the entry point for all C# programs. All programs must have the main method. Line 7 contains the opening brace ({) marks the beginning of the method. Basic Structure of C# Program Line 8 will be ignored by the compiler and it has been put to add additional comments in the program. Comments can be created using: // …….. for creating single line comment for creating Multi-lines comments Line 9 Console.WriteLine("Hello World"); This statement causes the message "Hello, World!" to be displayed on the screen. Basic Structure of C# Program Line 10 Console.ReadKey(); makes the program wait for a key press and it prevents the screen from running and closing quickly. Line 11 contains the closing brace (}) marks the end of the method. Line 12 contains the closing brace (}) marks the end of the class. Line 13 contains the closing brace (}) marks the end of the namespace. Declaration Statement Declaration Statement examples Data Type We consider primitive data types that are built into C# (string, char, int, long, float, double, bool). Each data type name is a C# keyword. The following table lists the available value types in C#. Data Type Variable Variables represent memory locations that hold values. These values may be changed in the program. Variable name is an identifier. All identifiers must follow the following rules: It is a sequence of characters of any length. It consists of letters, digits, underscores (_). It must start with a letter or an underscore (_). It cannot start with a digit. It cannot include space or special character between characters. It cannot be a reserved word. legal identifiers and illegal identifier Variable When the variable is declared it represented as a memory location. Variable is declared in terms of its name, type, size and value. When a new value is entered the old value is lost. Printing Statement It prints a text message or displays the value of a variable on the screen. Write a program that read two integer numbers and display their summation Using system namespace hello class hell static void main(String [] args) { int x,y,z; x=3; y=4; z=x+y; Console.Write(z); Console.ReadKey(); } } } Assignment statement Output devices Introduction to Programming and Problem Solving (CS101) Lecture 7 Prepared by/ Ibrahim Shawky Write a program that read two integer numbers and display their summation, then trace the output of the program statements. }}} Example explanation Suppose that the values of the number1 and number2 are 9 and 3 respectively. The following table illustrates tracing the program statements. Reading Statement Reading statement used to get value from the user input. Write a program that convert temperature in Celsius to degrees Fahrenheit. Implement the algorithm that calculates the net salary of an employee }} Write c# code for Computing the area of rectangle Constant Constants are permanent and immutable values. A constant represents a read only variable that never change along the life cycle of the program. A constant must be declared and initialized in the same statement. Here is the syntax for declaring a constant. In this example, the constant PI is always 3.14, and it cannot be changed. The constant Week always has the value of 7. Write c# code for Computing the area of circle // Program to implement area of circle in C# using System; class Circle { static void Main(string[] args) { Const float pi=3.14; Console.Write("Enter Radius: "); double rad = Convert.ToDouble(Console.ReadLine()); double area = pi * rad * rad; Console.WriteLine("Area of circle is: " + area); } } Type Conversion Type conversion converts one type of data to another type. Type conversion can be implicit or explicit. Implicit conversions are performed by converting a small data type to larger type. For example, converting from int to long is legal implicit type conversion, where converting from long to int is illegal implicit type conversion. Type Conversion Explicit conversions are performed by explicitly using the predefined functions: Casting. Convert class. Parse Method (convert from string). Here is an example for casting double to int. Type Conversion Convert class provides the following built-in type conversion methods. Type Conversion Assignment statement Operators C# provides a set of built-in operators. C# operators decomposed into several types include the following: Arithmetic operators. Relational operators. Logical operators. Bitwise operators. Assignment operators. Arithmetic Operators Arithmetic operators can be classified as binary operators such as (+, -, *, / and %) and unary operators such as (++ and --). Suppose that variables x, y and z holds the values 10, 2 and 3 respectively Output devices Introduction to Programming and Problem Solving (CS101) Lecture 7 Prepared by/ Ibrahim Shawky Operators C# provides a set of built-in operators. C# operators decomposed into several types include the following: Arithmetic operators. Relational operators. Logical operators. Bitwise operators. Assignment operators. Arithmetic Operators Arithmetic operators can be classified as binary operators such as (+, -, *, / and %) and unary operators such as (++ and --). Suppose that variables x, y and z holds the values 10, 2 and 3 respectively Increment and Decrement Operators The increment (++) and the decrement (--) operators are used for increasing and decreasing the value of a variable by 1 respectively. Example Relational Operators Relational operators are known as comparison operators. All relational operators are binary operators that study the relationship between two expressions. Suppose that variables x and y holds the values 10 and 2 respectively. Relational Operators Logical Operators Logical operators can be classified as binary operators such as (&& and ||) and one unary operator (!). Suppose that the Boolean variables x and y holds the values true and false respectively. e Bitwise Operators Bitwise operators work on bits and perform bit by bit operation. It can be classified as binary operators such as (&, |, ^, >) and only one unary operator (~). Bitwise Operators Compound Assignment Operators C# allows you to combine assignment and various preceding mentioned operators using compound assignment operators. For example, the arithmetic expression (count = count + 1) increases the variable count by 1 can be written as (count += 1). Operators Precedence Operator precedence determine the order in which operators are evaluated. Certain operators have higher precedence than others; for example, the division operator has higher precedence than the subtraction operator. Size of method To get the exact size of a type or a variable on a particular platform, we can use the sizeof method. When the following code is compiled and executed, it produces the following result, Size of int: 4 Exercise Write a program that converts the distance entered in meters to kilometer? Write the program that take minimum and maximum temperature and calculate the average temperature? Output devices Introduction to Programming and Problem Solving (CS101) Lecture 8 part 1 Prepared by/ Ibrahim Shawky Example Decision Decision making structure is a conditional structure that causes the program to make a selection based on evaluating a certain expression. If the expression evaluates to be true, it causes the program to execute a statement or a set of statement C# provides three conditional statements (if, if..else and switch..case) beside a conditional operator. if Statement If Syntax Example The following two statements are equivalent that check if the value of the variable i greater than 0, then display the message "i is positive". Example: write a program that display youth on the screen if the age of the person is less than or equal 20 Example: Write code segment that estimates the grade of the student based on his input degree. if... else Statement Example Even or odd Example: write a program that display youth on the screen if the age of the person is less than or equal 20 other wise display older on the display Nested if … else Structure Example represents the nested if… else version of the code segment that print the grade of the student according to his degree. Example Example 5.6: Develop a program that accept two integer numbers from the user and then perform one operation (+ for summation, - for Subtraction, * for multiplication, / for Division). Example Example Example 2: write the program that find whether the input is positive, negative or zero? Example: Write a program to find the maximum of two numbers? Output devices Introduction to Programming and Problem Solving (CS101) Lecture 8 part 2 Prepared by/ Ibrahim Shawky Example Develop a program that compute the net salary if you know: If salary greater than or equal 1000, the tax = 5%. If salary between 1000 & 500, the tax = 2%. Otherwise No tax. Example: Write a program to find the maximum of three numbers? switch … case Structure Switch syntax Example Write a program that accept two numbers from the user and one mathematical operator (+ to Add, - to Subtract, * to multiply, and / to Division), then perform only one of the four mathematical operation. Example Example Write a code segment that accept an integer number (1-9) from the user and display its equivalent alphabetic. Conditional Assignment Syntax example : Use switch to write a program to find the country international phone code that match the name of the country Exercise Write a program which inputs a person’s height (in centimeters) and weight (in kilograms) and outputs one of the messages: underweight, normal, or overweight, using the criteria: Underweight: weight < height/2.5 Normal: height/2.5