Week 6 Exercises (C Programming)
Document Details
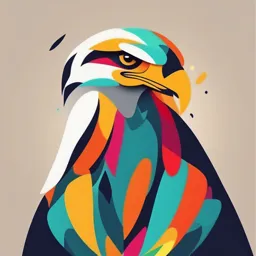
Uploaded by SnazzyEarthArt8280
Istanbul Atlas Üniversitesi
Tags
Summary
These slides cover various C programming exercises, including calculating sums of even and odd numbers, manipulating matrices, swapping array elements, using functions and loops for calculations, identifying vowels and spaces, finding the largest elements in arrays, and other fundamental C programming concepts related to algorithms.
Full Transcript
Exercises Write a C program that asks the user to enter 10 numbers and calculates the sum of odd and even numbers separately. Create a multidimensional matrix according to the number of rows and columns taken from the keyboard, assign elements and print the sum of the elements to the screen....
Exercises Write a C program that asks the user to enter 10 numbers and calculates the sum of odd and even numbers separately. Create a multidimensional matrix according to the number of rows and columns taken from the keyboard, assign elements and print the sum of the elements to the screen. Sample Output Write a program that swaps the first three elements of a 10-element array with the last three elements. Sample Output First, create a 2x3 matrix, take the elements of the array from outside and display them on the screen. Then, swap the elements you entered as rows and columns, that is, turn the rows into columns and the columns into rows and display them on the screen. (Subject in Mathematics: Transpose) Functions in Maths Functions in C Function Declation Why we use the functions? -Some of the key benefits of using functions are: Enables reusability and reduces redundancy. Makes a code modular. Once a function is defined, it can be used over and over and over again. Types of Functions 1.Library Functions 2.User Defined Functions) (built-in function) (tailor-made functions) A compiler package already exists that contains these functions, each of which has a User-defined functions can be specific meaning and is included in the improved and modified according package. to the need of the programmer. pow(), sqrt(), strcmp(), strcpy() etc. Let Remember! The void function is used to indicate that a function does not return any value. This means that the function performs an action but does not return a result after executing that action. What is the output ? -Be careful –> Global/local Local Variable: variable Variables defined inside a function or block (for example, an if or for block). They can only be used inside the block or function in which they are defined. These variables are recreated each time the function is called and their place in memory is released when the function ends. Global Variable: Variables defined outside of functions that all functions and code can access. They can be used anywhere in the program and can be shared between functions. They are in memory when the program starts running and Write a program using function add two numbers Solution 1 Solution 2 Solution 3 Calculate the Addition and Subtraction of Two Numbers Entered from the Keyboard Using the Function. Sample Output Program to calculate the area and circumference of a circle-II Solution 2: Using built-in function -> pow() Solution 1 Program to calculate the area and circumference of a circle-II pow( ) Solution 3: By creating your own Solution 4: By creating your own method (function) and function using the pow() method, which is a ready-made function in the class you created. Let's write a program that calculates the factorial How many different ways can you find a solution? Solution 2: Using Solution 1: Using for while loop loop Solution 3: Using Solution 4: Using if+for function Write a Program to Find the Largest Element in an Array Using an Array and a Function (Get the Array Size from the Keyboard) C Program to Find Number of Letters 'a' in a Text How to write a function that counts the number of vowels in a text? The clue: strlen() Write a program using a function to find the number of spaces in the entered text. Sample Output