DigiPen CSD1130 Lecture 3: Game State Manager and Function Pointers PDF
Document Details
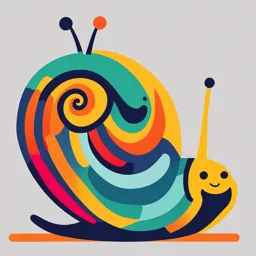
Uploaded by DarlingMagic
DigiPen Institute of Technology
Tags
Summary
This document presents lecture notes on game state management and function pointers, focusing on the design and implementation within a game engine. It uses pseudo code and flow charts to describe the game loop, state switching, and component management. Developed at DigiPen Institute of Technology, it covers essential concepts for the CSD1130 course.
Full Transcript
Game State Manager Lecture 3_2 Function Pointers CSD1130 1. Game Engine Design 2 1.1. Game engine flow 2...
Game State Manager Lecture 3_2 Function Pointers CSD1130 1. Game Engine Design 2 1.1. Game engine flow 2 Game Implementation Techniques © DigiPen Institute of Technology. All Rights Reserved. 1 Lecture 3 |Game State Manager – Function Pointers CSD1130 – Game Implementation Techniques 1. Game Engine Design 1.1. Game engine flow 2 © DigiPen Institute of Technology. All Rights Reserved. CSD1130 – Game Implementation Techniques Lecture 3 | Game State Manager – Function Pointers Any application consists of a general initialization part, followed by a loop that keeps running until the user decides to quit the application. This loop should handle all user input, handle how the user is interacting with the application, execute all game logic and finally reflect the overall result on the screen. In a game, this loop is called the “game loop”. When the game loop breaks (The player decided to quit the game or any other possible reason), the application will go to a “freeing” part, which basically releases all the data that was loaded during the initialization part. Note that all data that was loaded inside the game loop should be released before exiting it. As a general rule, all data that was loaded in one component should be released in the same component upon exit, which will make the code more consistent, the application more stable and memory leaks much easier to trace. Pseudo Code INITIALIZE system components INITIALIZE frame rate controller INITIALIZE game state manager WHILE Current game state is not equal to QUIT IF Current game state is not equal to RESTART CALL “Update()” game state manager CALL “Reset()” frame rate controller CALL “Load()” Current game state ELSE SET Next game state to be equal to Previous game state SET Current game state to be equal to Previous game state END IF CALL “Init()” Current game state WHILE Next game state is equal to Current game state CALL “Start()” frame rate controller UPDATE input status CALL “Update()” for the Current game state CALL “Render()” for the Current game state CALL “End()” frame rate controller END WHILE CALL “Free()” for the Current game state IF Next game state is not equal to RESTART CALL “Unload()” for Current game state END IF © DigiPen Institute of Technology. All Rights Reserved. 3 Lecture 3 |Game State Manager – Function Pointers CSD1130 – Game Implementation Techniques SET Previous game state to be equal to Current game state SET Current game state to be equal to Next game state END WHILE TERMINATE system components 4 © DigiPen Institute of Technology. All Rights Reserved. CSD1130 – Game Implementation Techniques Lecture 3 | Game State Manager – Function Pointers Managing system components Any game code (or application in general) has to initialize some system components before actually going into the game code and the game loop. These system components usually set up some necessary hardware related functionalities which will be later used within the game. Example: o Setting up an input device o Setting up video device o Setting up an audio device o Allocating video buffers o Deciding if some parts of the pipeline should be done using the hardware or software. This kind of system component initialization should be done just once before entering the game loop, and if any component fails to initialize properly, the application or the game usually quits with the appropriate error, since the application won't be able to run. If all system components are initialized properly, we initialize few arguments like the frame rate controller and the previous/current/next game state and the game loop is started. Note that the previous code should never be reached again. Upon exiting the game loop, all the devices that were allocated should be released before exiting the application. For example, if a video device was created, it should be released in order to free all its allocated resources on the video card. Also, if an input device was allocated during the initialization stage, it should be released upon exiting the application. This would be the final code part of the game or the application, and care must be taken to make sure every allocated resource is released. Game loop After initializing all the hardware related components, the application will enter the game loop, and will obviously keep looping until the user decides to quit the application (or for any other game logic reason). This loop is where all the actual game logic resides. It consists of: o Loading the current state: Loading all the state's data (unless it is being restarted.) o Resetting the frame rate controller o Initializing the current state (Make all the state's data ready to be used for the first time) o State loop, which keeps looping until the game switches to a different state or if the current state is reset. o Freeing the current state upon exiting the state loop o Unloading the state data in case it isn't being restarted Please refer them to the game state manager for a detailed description of states' functionalities. © DigiPen Institute of Technology. All Rights Reserved. 5 Lecture 3 |Game State Manager – Function Pointers CSD1130 – Game Implementation Techniques Handling game states o State switching When the game switches to a new state, it remains in this state until it needs to switch to another one. There are many reasons why a game should switch to another state, like the completion of a level, losing, or simply by pressing a cheat key. Restarting the same state is also considered a state switch, but special care is taken not to unload/reload the same data when a state is restarted (Please refer to the “Restarting” section). In order to do that, a state should have a loop on its own which is embedded inside the game loop. This loop is called the state loop. The condition to break out of the state loop is to set the next wanted state indicator to a state different than the current one. By doing so, the state loop will break the next time it restarts and checks the loop's condition. Note that when the next wanted state indicator is changed, the game continues running the current state until the end of the state loop (or in other words until the end of the current frame). The state switch will only occur at the next state loop condition check. o Loading/Unloading state data Each state uses different backgrounds, sprites, animations and sound effects... Therefore each state has its own set of data which should be loaded upon entering and exiting a state (and not restarting it). Data loading/unloading is usually slow, because it needs to load disk files which will leads to excessive access to the hard disk. Therefore it shouldn't be done during gameplay or during “interactive time” where having a constant and relatively high frame rate is extremely important, which can be in a loading or introduction level. By looking at the general game flow, notice that loading/unloading states' data is done outside the state loop. During the state loop, all the CPU and GPU power should be devoted to update and render the game, and none of it should be wasted on loading/unloading data like textures and sound files etc. o State restarting Restarting a state is treated the same way as switching to a different state during the first steps. The next state indicator can be set to “RESTART” for example, which is obviously different than the name of the current state. This will cause the state loop to break. Unlike switching to a different state, some condition checks will prevent the game from unloading the state's data upon exiting the state loop, and eventually they will also prevent reloading the same state data at the beginning of the game loop. These simple checks will save lots of time when restarting the same state, because loading/unloading 6 © DigiPen Institute of Technology. All Rights Reserved. CSD1130 – Game Implementation Techniques Lecture 3 | Game State Manager – Function Pointers can take a considerably great amount of time, especially if the state contains lots of animations and sound effects... The only thing we have to do when restarting a state is calling its free and initialize functions, which make the state's data ready again for use for the first time. Note that resetting the state's data is not specific to restarting a state, so it doesn't need any special condition checks. (Refer to the game state manager for more info about states' functionalities) © DigiPen Institute of Technology. All Rights Reserved. 7 Lecture 3_1 Frame Rate Controller 1. Game Engine Design 2 CSD1130 1.1. System Components 2 Game Implementation Techniques © DigiPen Institute of Technology. All Rights Reserved. 1 Lecture 3 | Frame Rate Controller CSD1130 – Game Implementation Techniques 1. Game Engine Design 1.1. System Components Game state manager o (Explained in previous session) Frame rate controller o In games, the amount of time needed to complete 1 frame (That is updating the objects, updating AI, checking for collision, reflection, physics, checking/updating input, updating general gameplay, rendering...) is extremely inconsistent. It depends on many factors like: ▪ Detail level ▪ Number of visible objects ▪ Number of effects (lights, normal mapping, shadows...) ▪ AI depth ▪ Collision types (Precise collision checks take more time than basic collision checks) ▪ Physics iterations ▪ Etc... o The main job of a frame rate controller is to make sure the game is running at a consistent speed. o This is done by making sure each frame is taking a certain pre-set amount of time. o Simply, whenever a frame is completed and it's time to go back to the beginning of the game loop (which is the beginning of the next frame or loop), we check how much time the current frame took. o If it's less than the pre-set amount, then we keep the game “waiting” - which is basically creating an empty loop – until that amount of time is reached. o On the other hand, if the amount of time taken to complete 1 frame is already greater than the wanted amount, then the way to fix it is by doing some optimizations (Like avoiding rendering objects outside the viewport's boundaries), reduce the number of effects used.. 2 © DigiPen Institute of Technology. All Rights Reserved. CSD1130 – Game Implementation Techniques Lecture 3 | Frame Rate Controller The frame rate controller can have other secondary functionalities like: o The number of frames since the game started o The number of frames since the current game state started o How much time the last frame used (This info could be used by time dependent objects, like animations and physics) Since the frame rate controller needs to know the time at the beginning and end of each loop, its functions should be called at these points. © DigiPen Institute of Technology. All Rights Reserved. 3 Lecture 3 | Frame Rate Controller CSD1130 – Game Implementation Techniques Frame rate controller pseudo code: Initialization Frames counter =0 Max Frame Rate = 60 Frame Rate = Max Frame Rate Frame Time = 1 / Frame Rate Min Frame Time = 1 / Max Frame Rate Frame Controller Start Frame Start = Current Time Frame Controller End While((Current Time – Frame Start) < (Min Frame Time)) Frame End = Current Time Frame Time = Frame End – Frame Start Increment Frame Counter Note that the time received from the system (Current Time in the pseudo code) should have at least a 1 millisecond precision in order for the frame rate controller to work. This is the game flow chart after implementing a frame rate controller: 4 © DigiPen Institute of Technology. All Rights Reserved. CSD1130 – Game Implementation Techniques Lecture 3 | Frame Rate Controller © DigiPen Institute of Technology. All Rights Reserved. 5